## 颜色
* 在Android中,颜色值由透明度alpha和RGB(红、绿、蓝)三原色定义,有八位十六进制数与六位十六进制数两种编码。
* 例如八位编码FFEEDDCC,FF表示透明度,EE表示红色的浓度,DD表示绿色的浓度,CC表示蓝色的浓度。透明度为FF表示完全不透明,为00表示完全透明。
* 六位十六进制编码有两种情况,在XML文件中默认不透明(透明度为FF),在代码中默认透明(透明度为00)。
所以在代码中要使用八位的十六进制数来表示颜色,因为六位的十六进制数在代码中是透明的。
下面的代码分别给两个文本控件设置六位编码和八位编码的背景色。
```
// 从布局文件中获取名叫tv_code_six的文本视图
TextView tv_code_six = findViewById(R.id.tv_code_six);
// 给文本视图tv_code_six设置背景为透明的绿色,透明就是看不到
tv_code_six.setBackgroundColor(0x00ff00);
// 从布局文件中获取名叫tv_code_eight的文本视图
TextView tv_code_eight = findViewById(R.id.tv_code_eight);
// 给文本视图tv_code_eight设置背景为不透明的绿色,即正常的绿色
tv_code_eight.setBackgroundColor(0xff00ff00);
```
XML文本布局的代码如下图
~~~
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="5dp"
android:orientation="vertical">
<TextView
android:id="@+id/tv_xml"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="XML设置六位背景颜色"
android:textSize="18sp"
android:background="#00ff00" />
<TextView
android:id="@+id/tv_code_six"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="代码设置六位背景颜色"
android:textSize="18sp" />
<TextView
android:id="@+id/tv_code_eight"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="代码设置八位背景颜色"
android:textSize="18sp" />
</LinearLayout>
~~~
如图所示
:-: 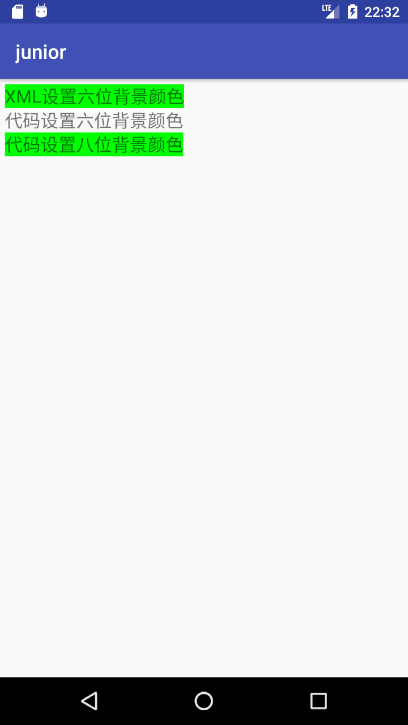
图1 不同方式设置颜色编码的效果图
在Android中使用颜色有下列3种方式:
1. 使用系统已定义的颜色常量。
Android系统有12种已经定义好的颜色,具体的类型定义在Color类中,详细的取值说明见表2-1。
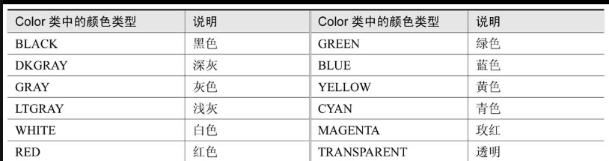
2. 使用十六进制的颜色编码。
在布局文件中设置颜色需要在色值前面加“#”,如`android:textColor="#000000"`。在代码中设置颜色可以直接填八位的十六进制数值(如`setTextColor(0xff00ff00);`),也可以通过`Color.rgb(int red, int green, int blue)`和`Color.argb(int alpha, int red, int green, int blue)`这两种方法指定颜色。在代码中一般不要用六位编码,因为六位编码在代码中默认透明,所以代码用六位编码跟不用没什么区别。
3. 使用colors.xml中定义的颜色。
res/values目录下有个colors.xml文件,是颜色常量的定义文件。如果要在布局文件中使用XML颜色常量,可引用“@color/常量名”;如果要在代码中使用XML颜色常量,可通过这行代码获取:`getResources().getColor(R.color.常量名)`。
在 XML 中定义的颜色值。颜色使用 RGB 值和 alpha 通道指定。您可以在接受十六进制颜色值的任何地方使用颜色资源。当 XML 中需要可绘制资源时,您也可以使用颜色资源(例如,`android:drawable="@color/green"`)。
该值始终以井号 (#) 字符开头,后跟以下某种格式的“透明度、红、绿、蓝”(Alpha-Red-Green-Blue) 信息:
* #RGB
* #ARGB
* #RRGGBB
* #AARRGGBB
**注意**:颜色是使用`name`属性中提供的值(而不是 XML 文件的名称)引用的简单资源。因此,您可以在一个 XML 文件中将颜色资源与其他简单资源合并到一个`<resources>`元素下。
**文件位置**:
`res/values/colors.xml`
该文件名可以任意设置。`<color>`元素的`name`将用作资源 ID。
**资源引用**:
在 Java 中:`R.color.*color_name*`
在 XML 中:`@[*package*:]color/*color_name*`
**语法**:
```
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color
name="color_name"
>hex_color</color>
</resources>
```
**元素**:
* `<resources>`
**必需**。该元素必须是根节点。
没有属性。
* `<color>`
以十六进制表示的颜色,如上所述。
属性:
* `name`
字符串。颜色的名称。该名称将用作资源 ID。
**示例**:
保存在`res/values/colors.xml`的 XML 文件:
~~~
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="opaque_red">#f00</color>
<color name="translucent_red">#80ff0000</color>
</resources>
~~~
以下应用代码会检索颜色资源:
~~~
Resources res = getResources();
int color = res.getColor(R.color.opaque_red);
~~~
以下布局 XML 会将该颜色应用到属性:
~~~
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:textColor="@color/translucent_red"
android:text="Hello"/>
~~~