# Config简介
随着微服务数量不断增加,需要系统具有可伸缩性和可扩展性,能够管理相当的的服务实例的配置管理,系统需要建立一个统一的配置管理中心。Spring Cloud 提供了分布式配置中心Spring Cloud Config,服务端默认的存储实现是Git,支持配置环境的标签版本。配置服务还支持多种仓库实现,如文件系统、Svn等。
本系统配置中心包含3个部分,配置服务器、客户端、Git仓库。
## 1、客户端
配置依赖,引入starter
```
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
```
启动配置类文件bootstrap.yml
```
eureka:
client:
service-url:
defaultZone: http://127.0.0.1:8092/eureka
spring:
cloud:
config:
discovery:
service-id: CITP-CONFIG-SERVER # 指定配置中心服务端的server-id
enabled: true #是否开启
label: master #指定仓库分支
profile: dev #指定版本
username: xxx
password: xxx
```
2.配置仓库
在建立配置服务器之前,需要现在git建立一个配置仓库,配置仓库如图:
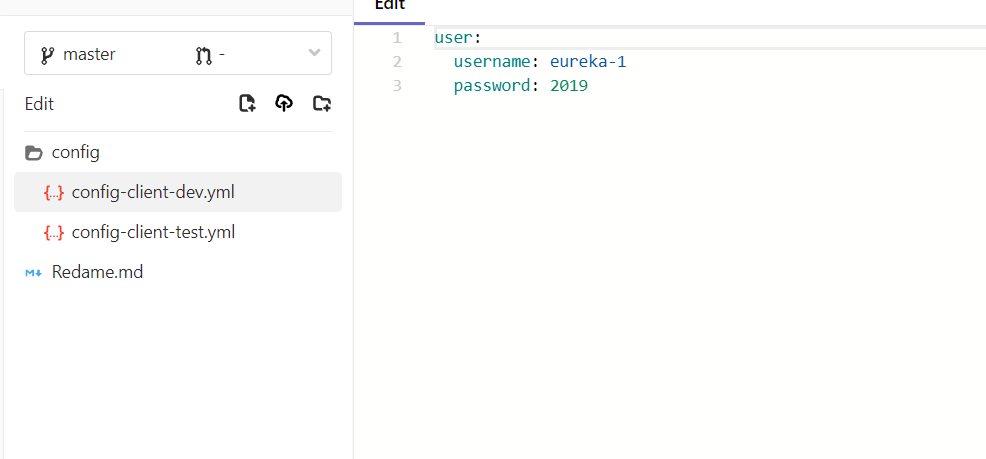
3.配置服务端
添加以下依赖:
```
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-config-server</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<!-- 安全保护 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
在入口application加入@EnableConfigServer、@EnableEurekaClient注解。
启动类配置文件bootstrap.yml配置Git信息:
```
spring:
application:
name: citp-config-server
cloud:
config:
server:
git:
uri: http://36.112.138.44:8081/CITPGroup/citp/config-demo.git #git配置地址
username: #git账号
password: #git密码
default-label: master #分支信息
search-paths: config #配置文件所在根目录
```
4.客户端验证
新建配置类读取git配置信息
```
@Component
@ConfigurationProperties(prefix = "user")
public class UserConfig {
private String username;
private String password;
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
}
```
新建controller验证读取信息
```
@RestController
public class ConfigController {
@Autowired
private UserConfig userConfig;
@GetMapping("/getValue")
public String getConfig(){
return userConfig.getUsername();
}
}
```