## Spring Framework的发展和Spring Boot
* Spring 3 支持Java注解
* Spring 4 支持 Java 8, 同时推出Spring Boot
Spring Boot 简化了Spring配置。
## Spring Boot是什么?
Boot 翻译有靴子,启动的意思, 在计算机领域有引导的意思, 比如计算机启动引导或是Linux 的引导程序。
Spring Boot就是Spring的快速引导, 也就是引导快速搭建Spring 框架。
早期的Spring项目基于XML文件进行配置, 类似Bean,数据源等都在XML中配置, 配置繁琐,初始化一个项目需要耗费较长时间, 后来Java的注解使用逐渐增多, 很多配置就可以从XML转到Java类本身了。
在此继承上, Spring Boot简化配置, 很容易就可以创建一个项目, 大大减少了项目初始化的时间。
** Spring Boot 不是一个全新的框架, 其是Spring的一种简化配置方式。**
## Spring Boot版本
2014 年首次发布。
* 版本历史 https://github.com/spring-projects/spring-boot/releases
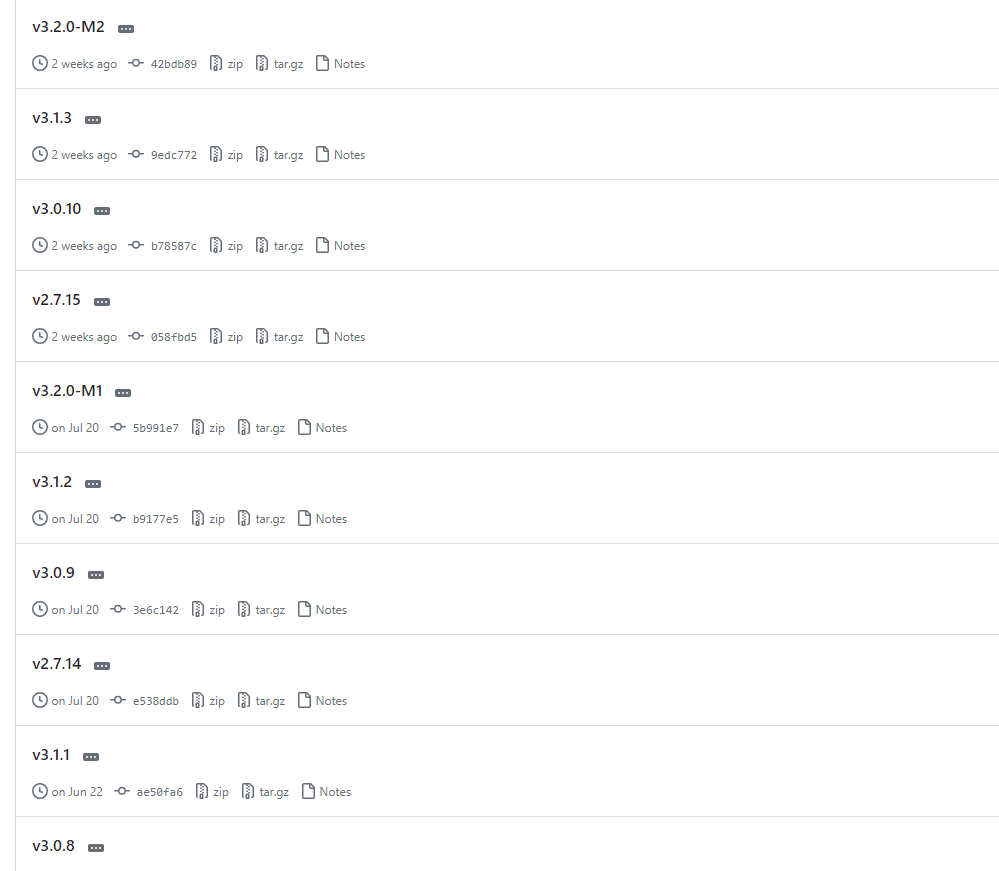
* 项目源码 https://github.com/spring-projects/spring-boot
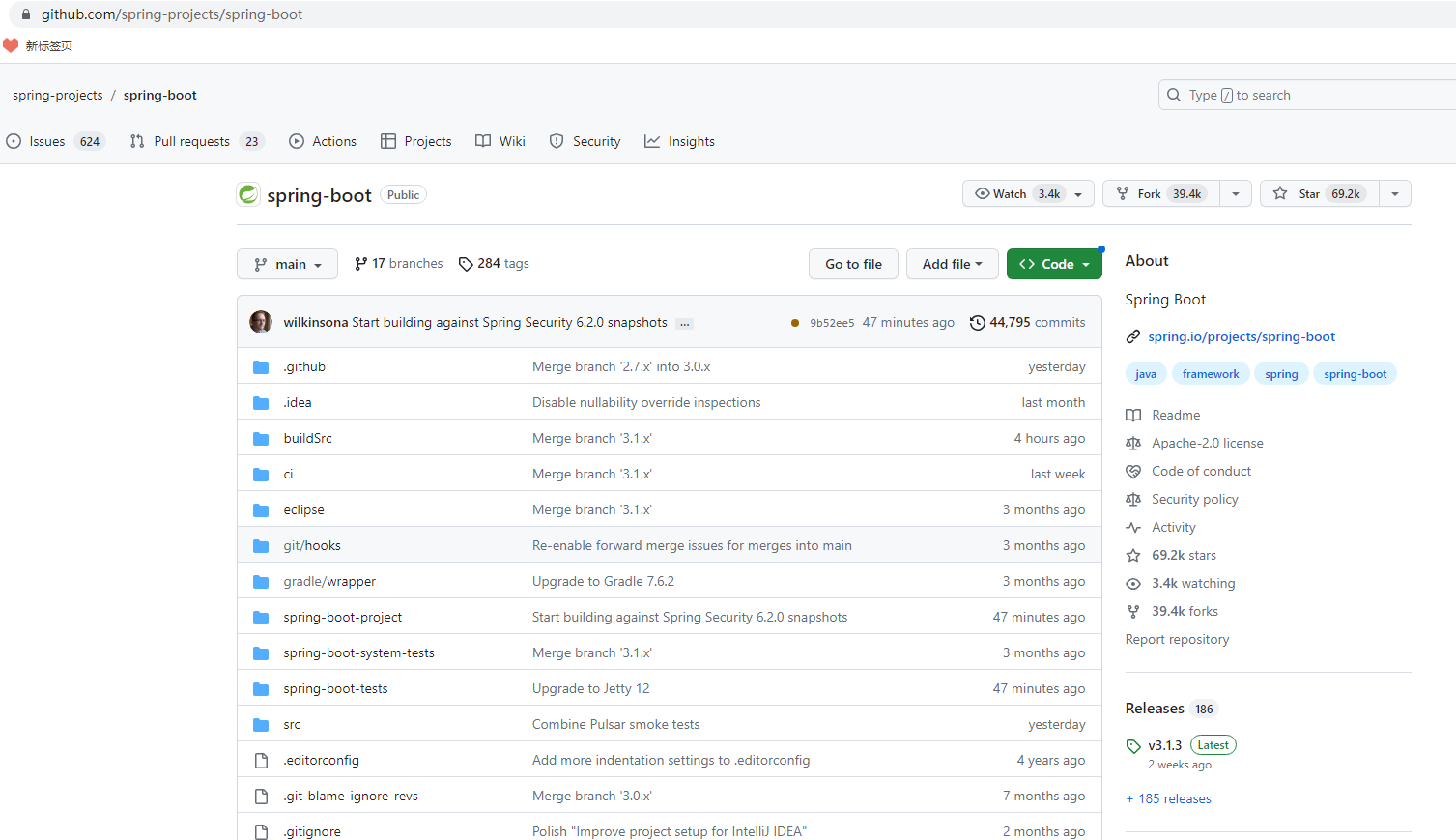
* 示例 https://github.com/netgloo/spring-boot-samples
## Spring Boot的特性:
Spring Boot的特性包括:
* 快速创建独立的Spring应用
* 内嵌了Tomcat , Jetty 等应用服务器, Web类型的项目不需要单独的部署就可以运行
* 提供了默认的初始化依赖
* 根据需要自动配置第三方依赖库
* 提供了现成可用的应用健康检查,测量以及外部配置
* 完全不需要代码生成以及XML配置。
* 自动配置(Auto Configuration): 简化配置, 比如在classpath 发现了Spring Security 的包, 则自动创建相关的Bean
* 启动器(Starters)
* CLI Comand-line interface 支持groovy 开发。
* Actuator : 查看运行参数、线程、GC等
## Spring Boot 项目创建与运行
Spring 官方提供了在线的项目生成器,可以很便捷的生成项目, 生成器的地址是https://start.spring.io/,除此之外, 也可以直接在IDE中创建Maven等类型的项目。 关于项目的创建和运行,可以参考:
[如何创建Spring Boot项目](https://blog.csdn.net/oscar999/article/details/101008543)
## Spring Boot 之约定优于配置
在Spring Boot中, 约定优于配置是一个入门需要了解的概念, 简单点理解就是默认配置。 大家遵循一定的契约。
### 约定优于配置之前
在Spring Boot之前, Spring + Spring MVC 开发Web需要配置很多的配置, 包括:
* 导入Web开发的很多jar (虽然可以依赖导入, 但还是要导入很多个)
* 在application-web.xml 配置视图解析器,类似
```
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/views/"/>
<property name="suffix" value=".jsp"/>
</bean>
```
* 在application.xml 中配置数据源, 事务等。
### Spring Boot 的配置
在Spring Boot中只需要导入 `spring-boot-starter-web`, 一切都默认配置好, 而且还内置一个Tomcat服务,是不是方便很多。
PS. 如果需要,可以对默认配置进行修改。
*****
*****
## 学习思维
* REST
* YAML 和Java Config
- 前言
- 1. 基础篇
- Spring Boot 介绍
- 如何创建Spring Boot项目
- Spring Boot基本使用
- 1. 系统构建
- 2. 代码结构和开发
- 项目配置
- 配置文件
- pom.xml 配置说明
- 测试
- 测试介绍
- 测试注解
- 常见问题及解决
- Spring Boot测试示例
- Spring Boot Test 进行JPA 测试保存数据到数据库
- 常见开发类
- Web
- HttpEntity
- CommandLineRunner
- 开发IDE
- Eclipse开发设置
- 开发知识点
- 项目相关路径与资源获取
- 2. 数据篇
- Spring操作数据库的方式汇总
- Spring Data JPA
- Spring Boot操作数据库的方式
- Spring Boot+JPA CriteriaBuilder查询
- Spring Boot+JPA 有查询条件的查询
- CriteriaBuilder转sql语句
- DAO
- Spring Boot+JPA 查询数据方式与代码演示
- Spring JPA 接口和类
- CrudRepository
- JPA Native Query(原生查询)的缓存问题
- Eclipse下安装EclipseLink进行JPA开发
- Eclipse Link or Hibernate
- 配置参考
- Spring Boot多数据源配置
- 错误解决
- ORA-00932: inconsistent datatypes: expected - got CLOB
- hibernate in 的list 大小不能超过1000
- java.lang.IllegalArgumentException: Cannot create TypedQuery for query with more than one return using requested result type
- ava.lang.IllegalArgumentException: org.hibernate.hql.internal.ast.QuerySyntaxException: Unable to locate class [XXX]
- JPA 基础
- Spring Jdbc 实现 Clob/Blob 类型字段的插入
- JPA Native Query(本地查询)及查询结果转换
- JPA如何查询部分字段
- JPA 主键策略
- JPA+EclipseLink+Oralce 通过GenerationType.AUTO 实现自增长字段
- JPA的persist() 和 merge() 方法的区别
- 关联
- 批量更新
- JPA重要知识点
- JPA中实体的状态
- 数据库操作技术
- JPA二级缓存
- JPA3
- 分页查询
- Spring Boot+JPA 事务
- Spring Boot 数据源
- Spring Boot 3 整合 H2 快速示例
- Spring Boot+JPA+Oralce 配置
- Spring Boot+JPA不同类型数据库的多数据源配置
- Spring Data JPA + Oracle
- Spring Boot + Oracle 处理Blob/Clob类型字段实例
- Spring Boot+JPA+Oracle Clob 类型字段查询处理
- Spring Boot + JPA + Oracle 自增长字段实现示例
- 内存数据库H2+Spring Boot
- 3. 实战篇
- 项目创建和管理
- 基于Maven创建多模块的Spring Boot项目
- 最佳实践
- REST —Java对象的字符串属性值转换为JSON对象的布尔类型值
- Spring Boot 项目中Java对象的字符串类型属性值转换为JSON对象的布尔类型键值的解决方法及过程
- [在线API]Spring Boot+springfox-swagger2 之RESTful API自动生成和测试
- Spring Boot与JWT整合实现前后端分离的用户认证之一
- 前后端分离的用户验证原理及Spring Boot + JWT的框架搭建(附完整的框架代码)之一
- 前后端分离的用户验证原理及Spring Boot + JWT的框架搭建(附完整的框架代码)之二
- SpringBoot应用中JSP的角色及整合
- Spring Boot项目中使用JSP实例
- Spring Boot 文件上传功能实现与简单示例
- Spring Boot+CKEditor 实现编辑器中的图片上传
- 开发注意事项
- 部署与维护
- SpringBoot项目在Gitee的控管步骤
- JSP如何获取Spring Boot的配置属性
- 错误页面处理
- JPA相关
- Spring Boot + JPA 表和类的对应处理
- Spring Boot + JPA +MySQL 数据操作及示例环境搭建(手动建表)
- Spring Boot + JPA +MySQL 数据操作及示例环境搭建(自动建表)
- 基于Spring Boot + JPA +MySQL的JPA关联查询ManyToOne示例
- JPA的单向一对多关联(oneToMany)实现示例(基于Spring Boot + JPA +MySQL,表自动维护)
- JPA的双向一对多关联(oneToMany)实现示例(基于Spring Boot + JPA +MySQL,表自动维护)
- JPA的双向一对一关联实现示例(基于Spring Boot + JPA +MySQL,表自动维护)
- 一次使用JUnit + JPA 实现数据Migrate的实例介绍
- Spring Boot整合H2内存数据库配置及常见问题处理
- Spring Boot + Spring Data JPA 实现数据库操作的标准示例
- REST
- Spring Boot 访问HTTPS的url
- Spring Boot集成Log4j2
- Spring Boot + Ext JS 安全
- Spring Boot Web
- 内置服务器
- Spring Boot 中Filter的定义方式
- 开发工具
- Spring 开发利器之——Spring Tools 4
- Spring OAuth2+Basic
- Spring 缓存
- 快速实例
- Spring Security实战
- Spring Boot OAuth2 快速入门示例
- Spring Boot 项目使用Spring Security防护CSRF攻击实战
- Spring Boot OAuth2 认证服务器搭建及授权码认证演示
- 基于Spring OAuth2 之客户端凭证模式演示
- 基于Spring OAuth2 之简化模式演示
- 密码模式
- 客户端凭证
- 设置JWT Claim
- Client 数据库配置
- Spring Boot+Security 实现Basic认证
- Spring Boot + WebLogic 12c 之Basic认证
- spring-security-oauth2-authorization-server
- 4. 专题篇
- 项目与属性配置
- 外部化程序的配置
- 服务配置
- 不使用spring-boot-starter-parent作为Parent
- 数据库配置
- Spring Boot 2.7.14+Oracle 11+WebLogic
- Spring Boot+Vaadin
- Spring Boot+Vaadin 14项目初始化
- Vaadin 导入JS和添加JS代码段
- 常见及疑难问题解决
- Could not find artifact org.vaadin.artur:a-vaadin-helper:jar:1.6.2
- Spring Boot+Activiti
- Spring Boot + Activiti 工作流框架搭建
- Activiti 知识
- Spring Boot+Activiti开发注意点
- Activiti 代码
- Spring Boot缓存
- Spring Boot Cache
- Spring Boot整合Redis快速入门实例
- 配置 JSON 序列器
- 错误解决篇
- Unable to connect to Redis; nested exception is io.lettuce.core.RedisConnectionException: Unable to connect to localhost:6379
- Spring Root+Cache+Redis 实战
- Spring Boot+Redis 实现分布式缓存
- Spring Cloud + Redis 配置
- 主要类和方法
- 使用方式
- 缓存配置
- Redis Cache
- 缓存注解
- 类配置
- Spring Cloud
- 微服务架构
- 快速了解Spring Cloud
- Spring Cloud Eureka 最简入门示例
- Spring Cloud Ribbon 负载均衡客户端调用示例
- Spring Cloud Eureka注册服务器集群配置
- Spring Cloud常见问题及解决
- Spring Cloud Eureka Server的部署与启动
- 配置中心
- Spring Boot+HATEOAS
- HATEOAS概念
- Spring Boot+HATEOAS快速介绍与示例
- 开发说明
- Spring 安全
- Spring 框架RCE 安全漏洞
- Spring RCE 漏洞 CVE-2022-22965 的终极解决方案
- Spring Boot 跨域访问实现方式
- 轻量级网关-反向代理
- Spring Security
- 基本使用
- 主要问题
- securityChain
- UserDetailsService
- 忽略请求URL
- 安全
- Spring Boot项目CSRF (跨站请求伪造)攻击演示与防御
- Spring Boot CSRF 防御+Coverity
- 认证管理器-AuthenticationManager
- Spring Security开发介绍
- Spring Security 快速入门
- Spring Security 依赖导入
- Spring Security 用户认证
- Spring CSRF 实现机制
- 实战示例
- 入门示例
- Spring Security 常见问题
- Caused by: java.lang.IllegalArgumentException: realmName must be specified
- 总是报403错误
- Spring访问http/https
- Spring RestTemplate 访问https站点
- Spring Boot RestTemplate 忽略证书访问https
- Spring Boot OAuth2 Client -客户端模式 client credentials 获取token
- OAuth2RestTemplate
- Spring Security OAuth 访问OAuth2.0 的https站点
- Spring RestTemplate 忽略证书访问https的OAuth2 认证站点
- Spring OAuth 2.0
- 授权服务器和资源服务器
- Spring 对 OAuth2.0的解决方案
- OAuth2 认证服务器
- jjwt+oauth
- Spring OAuth2 的认证
- Spring Boot实现访问接口的Basic认证
- CORS
- Access-Control-Allow-Credentials
- Java JWT
- JWT
- 声明-subject
- 安全基本知识
- BCrypt
- Spring LDAP
- Spring Boot LDAP 认证
- LDAP问题与解决
- javax.naming.PartialResultException: Unprocessed Continuation Reference(s);
- Spring IO
- 接口和类
- Resource
- 性能
- Web缓存
- Spring Web
- 如何添加Filter
- OncePerRequestFilter
- 得到请求地址和参数
- 应用上下文路径
- 5. 开发参考
- 注解
- WebMvcAutoConfiguration
- JPA
- 如何加载 jar 中的注解类
- 1
- @EnableAsync
- 6. 常见问题
- bean named 'entityManagerFactory' that could not be found错误及解决
- invalid LOC header错误及解决
- No identifier specified for entity:
- Optional int parameter 'id' is present but cannot be translated into a null value due to being declared as a primitive type. Consider declaring it as object wrapper for the corresponding primitive type.
- Could not resolve view with name 'xxxx' in servlet with name 'dispatcherServlet'
- JSON parse error: Unrecognized field
- multipart/form-data;boundary=----WebKitFormBoundaryRAYPKeHKTYSNdzc1;charset=UTF-8' not supported
- 跨域 Access to XMLHttpRequest at ' xxxxx' from origin 'null' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: No 'Access-Control-Allow-Origin' header is present on the requested resource.
- maven 下载问题
- Maven 无法下载Oracle 驱动解决
- Spring Boot常见面试题
- Spring Boot 访问JSP主页面出现下载页面
- Spring Boot 面试题大全
- Spring Cloud相关问题
- java.lang.IllegalStateException: Request URI does not contain a valid hostname: http://eurekaclient_service/
- Spring JPA
- Access to DialectResolutionInfo cannot be null when 'hibernate.dialect' not set。
- 同时排序和分页查询的数据不对
- No EntityManager with actual transaction available for current thread
- should be mapped with insert="false" update="false"
- java.lang.IllegalArgumentException: Not a managed type:XX
- java.lang.IllegalArgumentException: org.hibernate.hql.internal.ast.QuerySyntaxException: XX is not mapped
- org.springframework.transaction.UnexpectedRollbackException: Transaction silently rolled back because it has been marked as rollback-only
- Transaction rolled back because it has been marked as rollback-only
- org.hibernate.LazyInitializationException: could not initialize proxy xxx - no Session
- [EclipseLink]The attribute [xxx] of class [xxx] is mapped to a primary key column in the database. Updates are not allowed.
- cannot simultaneously fetch multiple bags
- Spring Boot + Hibernate java.lang.IllegalArgumentException: Not an entity: class
- mvn spring-boot class file for javax.interceptor.InterceptorBinding not found
- sun.security.validator.ValidatorException: PKIX path building failed: sun.security.provider.certpath.SunCertPathBuilderException: unable to find valid certification path to requested target
- Spring Boot返回的数据格式是XML 而不是JSON之原因探求的和解决
- Failed to parse multipart servlet request; nested exception is javax.servlet.ServletException: The request content-type is not a multipart/form-data
- WebSecurityConfigurerAdapter 过时及替代写法
- consider increasing the maximum size of the cache
- java.lang.ClassCastException: org.springframework.context.annotation.AnnotationConfigApplicationContext cannot be cast to org.springframework.web.context.WebApplicationContext
- org.springframework.core.NestedIOException: ASM ClassReader failed to parse class file - probably due to a new Java class file version that isn't supported yet
- Caused by: org.springframework.core.NestedIOException: ASM ClassReader failed to parse class file - probably due to a new Java class file version that isn't supported yet
- Spring Boot测试无法运行问题解决(注入的组件为空)
- web.xml is missing and <failOnMissingWebXml> is set to true
- no main manifest attribute
- Refused to display 'http://localhost:8080/' in a frame because it set 'X-Frame-Options' to 'deny'.
- 问题模板
- Spring Security问题
- WebSecurityConfigurerAdapter过期了
- @EnableAuthorizationServer 已经废弃
- com.fasterxml.jackson.databind.exc.InvalidTypeIdException: Could not resolve subtype of [map type; class java.util.Map, [simple type, class java.lang.String] -> [simple type, class java.lang.Object]]: missing type id property '@class'
- Spring Web问题
- I/O error while reading input message; nested exception is java.io.IOException: Stream closed
- Filter读取JSON参数之后, Spring无法自动封装
- 部署到 WebLogic的问题
- 如何导入其他jar 的组件
- 类型转换
- JSON 格式转换
- Spring开发问题之org.springframework.beans.factory.BeanDefinitionStoreException: Failed to read candidate component class
- 7. 官方文档翻译篇
- Spring Framework参考文档-V6.0.8
- 总览
- 核心
- 测试-
- 数据访问
- Web Servlet
- Web Reactive
- 整合
- 1. REST 客户端
- 2.JMS(Java消息服务)
- 3. JMX
- 4. 邮件
- 5. 任务执行与排程
- 6. 缓存抽象
- 7. 监控支持
- 语言
- Spring Boot核心功能
- 1. SpringApplication 启动引导类
- 2. 属性配置
- 2.8 类型安全的属性配置
- 3. Profile
- 4. 日志
- 5. 国际化
- 6. JSON
- 7. 任务执行和计划
- 8. 测试
- 8.1 测试范围依赖
- 8.2 测试Spring应用
- 8.3 测试Spring Boot应用
- 8.3.1 侦测Web应用的类型
- 8.3.2 侦测测试配置
- 8.3.3 排除测试配置
- 8.3.4 使用应用的参数
- 8.3.5 使用模拟环境测试
- 8.3.6 使用服务器进行测试
- 8.3.7 自定义WebTestClient
- 8.3.8 使用JMX
- 8.3.9 使用Metrics
- 8.3.10 模拟和窥探Beans
- 8.3.11 自动配置的测试
- 8.3.12 自动配置的JSON测试
- 8.3.13 Spring MVC测试的自动配置
- 8.3.14 Spring WebFlux 测试的自动配置
- 8.3.15 Cassandra 测试的自动配置
- 8.3.16 数据 JPA 测试的自动配置
- 8.4 测试工具类
- 9. 定制属于你的自动配置
- 9.1 了解自动配置的Beans
- 9.2 定位自动配置的候选者
- 9.3 条件注释
- 10. Kotlin 支持
- Spring Boot 其他功能
- 7. Web 应用的开发
- Spring MVC 框架
- Spring WebFlux 框架
- JAX-RS 和Jersey
- 内置 Servlet 容器支持
- 内置响应式服务器支持
- 响应式服务器资源配置
- 8. 优雅关闭
- 9. RSocket
- 10. 安全
- 11. 使用SQL 数据库
- 11.2 JdbcTemplate 的使用
- 12. NoSQL技术
- 13. 缓存
- 14. 消息
- 15. 使用RestTemplate调用REST服务
- 16. 使用WebClient调用REST服务
- 17. 验证
- 18. 发送邮件
- 19. 使用JTA实现分布式事务
- 20. 分布式内存-Hazelcast
- 21. 定时器Quartz Scheduler
- 22. 任务执行和计划
- 23. Spring 整合
- 24. Spring 会话
- 25. 使用JMX 监控和管理
- 26. Testing 测试
- 27. WebSockets
- 28. Web Services
- 29. 创建自动配置
- 30. 对Kotlin 的支持
- 31. 容器
- Spring Boot开发指南 V3.1
- 入门
- Spring Boot应用升级
- 如何使用Spring Boot进行开发
- 核心功能
- Web-
- Data-数据
- IO
- 缓存
- 消息
- 容器
- 生产就绪功能
- 部署
- GraalVM Native Image Support
- Spring Boot命令行
- 构建工具插件
- 1. Spring Boot 应用
- 1.1 自定义错误分析器
- 1.2 自动配置的故障排除
- 1.3 启动之前自定义环境(Environment)和应用上下文(ApplicationContext)
- Spring Data Redis -Version 3.0.5
- 前言
- Spring Redis 开发参考文档
- 响应式 Redis 支持
- Redis集群
- Redis存储库
- Spring Security
- 参考
- Spring Authorization Server-0.4.2
- 概括
- 帮助
- Spring认证服务入门
- Spring Authorization Server-0.4.3
- 协议端点(Protocol Endpoints)
- OAuth2 授权端点
- 核心模型组件
- OAuth2TokenCustomizer
- 关联知识
- JWK Set
- 默认端点
- JWT JWK
- OpenID Connect 1.0
- OAuth2
- 问题
- [invalid_request] OAuth 2.0 Parameter: redirect_uri
- [invalid_request] OAuth 2.0 Parameter: state
- There is no PasswordEncoder mapped for the id "null"
- 实际开发
- 8. 补充篇
- Jackson
- 资源
- 配置大全
- Spring Boot 与 Java 版本对应
- Java Web
- 如何在Filter中读取JSON 类型的参数