[TOC]
# Zepto 框架
**Zepto** 是一个**轻量级**的针对现代高级浏览器的 **JavaScript 库**, 它**与jquery 有着类似的api**。 如果你会用 jquery,那么你也会用 zepto。
**Zepto**的**设计目的是提供 jQuery 的类似的API**,但**并不是100%覆盖 jQuery** 。Zepto设计的目的是有一个**5-10k**的通用库、下载并快速执行、有一个熟悉通用的API,所以你能把你主要的精力放到应用开发上。
## **jQuery和Zepto.js的区别**
1. **jQuery**更多是在**PC**端被应用,因此,考虑了很多**低级浏览器的的兼容性**问题;而**Zepto**.js则是直接**抛弃了低级浏览器的适配**问题,显得很轻盈;
2. **Zepto**.js在**移动端**被运用的更加广泛;
3. **jQuery**的底层是通过**DOM**来实现效果的, **zepto**.js 是用**css3** 来实现的;
4. **Zepto**.js可以说是**阉割**版本的**jQuery**。
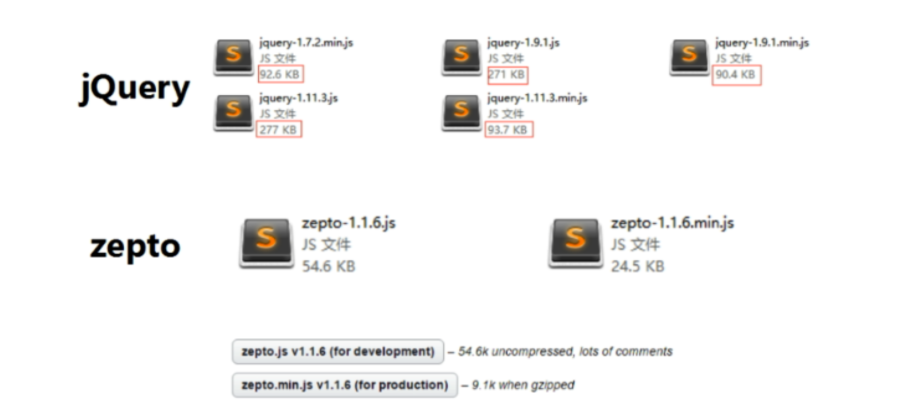
5. **zepto**与**jquery**主要的区别是在**模块**上的区别:[http://www.css88.com//doc//zeptojs\_api//](http://www.css88.com//doc//zeptojs_api//)
* zepto默认只有**5个基本的模块**,其他功能模块需要**单独引用** 。
* 引用的模块,必须放在zepto的后面,`fx.js 和fx_methods.js`他们之间必须是fx\_methods.js在fx.js的后面;其他的包之间顺序无所谓;
* jQuery默认是一个文件中,**包含所有zepto中的功模块**;
* zepto的底层是通过**css3** 实现的,jQuery是操作的DOM,**所以**有些css3的效果,是jquery做不到的;
* zepto比jQuery多了更多的**移动端**的 事件的支持,比如说**tap, swipe**……
* zepto的兼容性比jQuery差,因为zepto更多的是**注重移动端和效率**,jQuery注重的是**兼容性**。
> 注意:zepto上面的动画,不要加太多, 因为动画很耗性能,加多了会很卡,特别是一些webview开发;
英文版:[http://zeptojs.com//](http://zeptojs.com//)
中文版:[http://www.css88.com//doc//zeptojs\_api//](http://www.css88.com//doc//zeptojs_api//)
github : [https://github.com/madrobby/zepto](https://github.com/madrobby/zepto)
## zepto兼容的浏览器
> Safari 6+
> Chrome 30+
> Firefox 24+
> iOS 5+ Safari
> Android 2.3+ Browser
> Internet Explorer 10+
## Zepto初体验
```
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
div{
width: 200px;
height: 200px;
background-color: red;
}
</style>
</head>
<body>
<button id="btn">点击改颜色</button>
<div class="box"></div>
<!--引入jq-->
<!--<script src="js/jquery-3.2.0.js"></script>-->
<!--引入zepto-->
<script src="js/zepto.min.js"></script>
<script>
$(function () {
$('#btn').on('click',function () {
$('.box').css({
backgroundColor:'green',
});
});
})
</script>
</body>
</html>
```
## zepto与jquery使用上的区别
注意:**zepto**与**jquery**主要的区别是在**模块**上的区别:[http://www.css88.com//doc//zeptojs\_api//](http://www.css88.com//doc//zeptojs_api//)
### 1.选择器-模块
选择器$( ' div:eq(1) ' ) : [http://www.w3school.com.cn/jquery/jquery\_ref\_selectors.asp](http://www.w3school.com.cn/jquery/jquery_ref_selectors.asp)
闭包的好处:[http://blog.csdn.net/vuturn/article/details/43055279](http://blog.csdn.net/vuturn/article/details/43055279)
例子:点击改第二个颜色
```
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
div{
width: 200px;
height: 200px;
background-color: red;
margin: 10px;
}
</style>
</head>
<body>
<button id="btn">点击改第二个颜色</button>
<div >1</div>
<div >2</div>
<!--引入jq-->
<!--<script src="js/jquery-3.2.0.js"></script>-->
<!--引入zepto-->
<script src="js/zepto.min.js"></script>
<!--selector要放在zepto后面-->
<script src="js/selector.js"></script>
<script>
$(function () {
/*点击改第二个颜色*/
$('#btn').on('click',function () {
$('div:eq(1)').css({
backgroundColor:'green',
});
});
});
</script>
</body>
</html>
```
### 2.动画-模块-animate
例子:点击改变宽度
```
~~~
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>03-zepto动画</title>
<style>
div {
width: 200px;
height: 200px;
background: skyblue;
}
</style>
<!--<script src="js/jquery-1.7.2.min.js"></script>-->
<script src="js/zepto.js"></script>
<script src="js/fx.js"></script>
</head>
<body>
<button>d点击</button>
<div></div>
<script>
$(function () {
$('button').click(function () {
$('div').animate({width: 400, background: 'gold'}, 5000);
// $('div').animate({background:'gold'},5000);
});
})
</script>
</body>
</html>
~~~
```
### 3.隐藏toggle-模块
```
~~~
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<title>Title</title>
<style>
div {
width: 200px;
height: 200px;
background-color: red;
}
</style>
</head>
<body>
<button id="btn">来回切换隐藏</button>
<div class="box"></div>
<!--<script src="js/jquery-3.2.0.js"></script>-->
<script src="js/zepto.min.js"></script>
<script src="js/fx.js"></script>
<script src="js/fx_methods.js"></script>
<script>
$(function () {
$('#btn').on('click', function () {
$('.box').toggle(1000);
})
})
</script>
</body>
</html>
~~~
```
### 4.Tap事件-模块
**tap** `只作用在移动端,PC端是无效的;`
**click** 在pc端和移动端都是ok的;
但是我们在移动端要用tap,因为 tap 比 click 快200-300ms。
例子,点击改变颜色:
```
~~~
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<title>Title</title>
<style>
div {
width: 200px;
height: 200px;
background-color: red;
}
</style>
</head>
<body>
<button id="btn">点击修改颜色</button>
<div class="box"></div>
<!--<script src="js/jquery-3.2.0.js"></script>-->
<script src="js/zepto.min.js"></script>
<script src="js/touch.js"></script>
<script>
$(function () {
/*$('#btn').on('click', function () {
$('.box').css({
backgroundColor: 'green',
});
});*/
$('#btn').tap(function () {
$('.box').css({
backgroundColor:'green',
})
});
});
</script>
</body>
</html>
~~~
```
### 5.swipe滑动-模块-animate
注意: 如果想在移动设备上使用swipe事件,先要**清除系统默认的手势事件:touch-action: none**,如果没有清楚系统默认的事件可能swiper事件不会回调和tap事件触发多次。
```
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<title>Title</title>
<style>
div{
width: 100px;
height: 100px;
background-color: red;
position: absolute;
left: 30px;
top: 30px;
}
/*清楚系统默认的事件*/
*{
touch-action: none;
}
</style>
</head>
<body>
<div class="box"></div>
<script src="js/zepto.min.js"></script>
<script src="js/touch.js"></script>
<script src="js/fx.js"></script>
<script>
$(function () {
/**
*
$('.box').swipe(function () {
console.log('滑动了')
});
$('.box').swipeLeft(function () {
console.log('向左滑动了')
});
$('.box').swipeRight(function () {
console.log('向右滑动了')
});
$('.box').swipeUp(function () {
console.log('向上滑动了')
});
$('.box').swipeDown(function () {
console.log('向下滑动了')
});
*/
$('.box').swipeLeft(function () {
$(this).animate({
left:0,
})
});
$('.box').swipeRight(function () {
$(this).animate({
left:'200px',
})
});
$('.box').swipeUp(function () {
$(this).animate({
top:0,
})
});
$('.box').swipeDown(function () {
$(this).animate({
top:'200px',
})
});
})
</script>
</body>
</html>
```
# Zepto实现tab效果am
## 1.新建一个项目
~~~
<!--引入zepto-->
<script src="js/zepto.min.js"></script>
<!--引入touch-->
<script src="js/touch.js"></script>
~~~
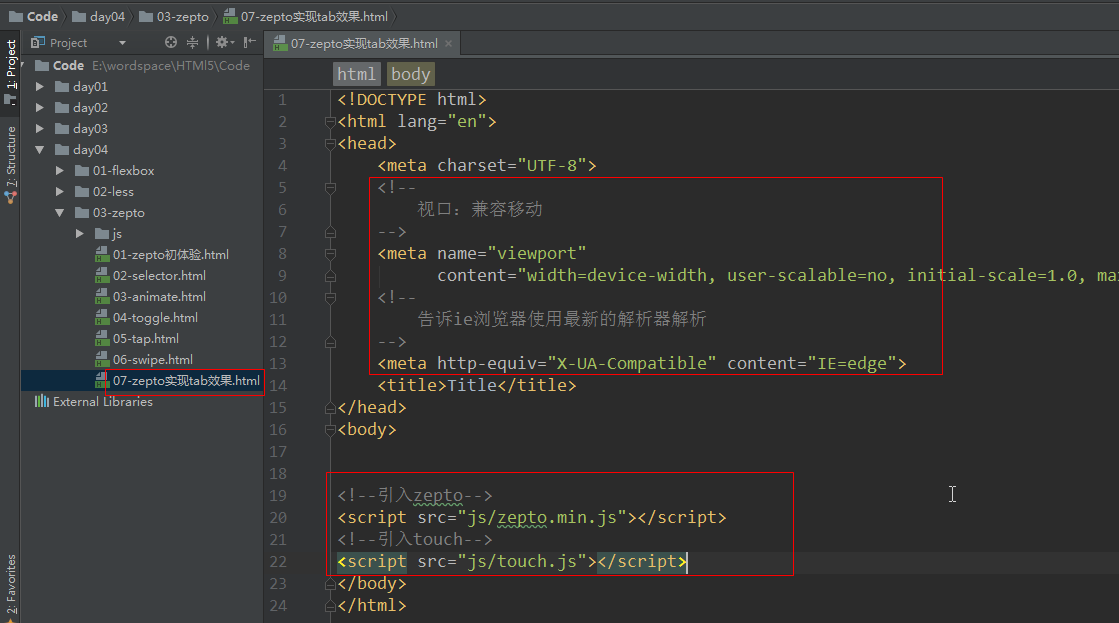
## 2.搭建布局
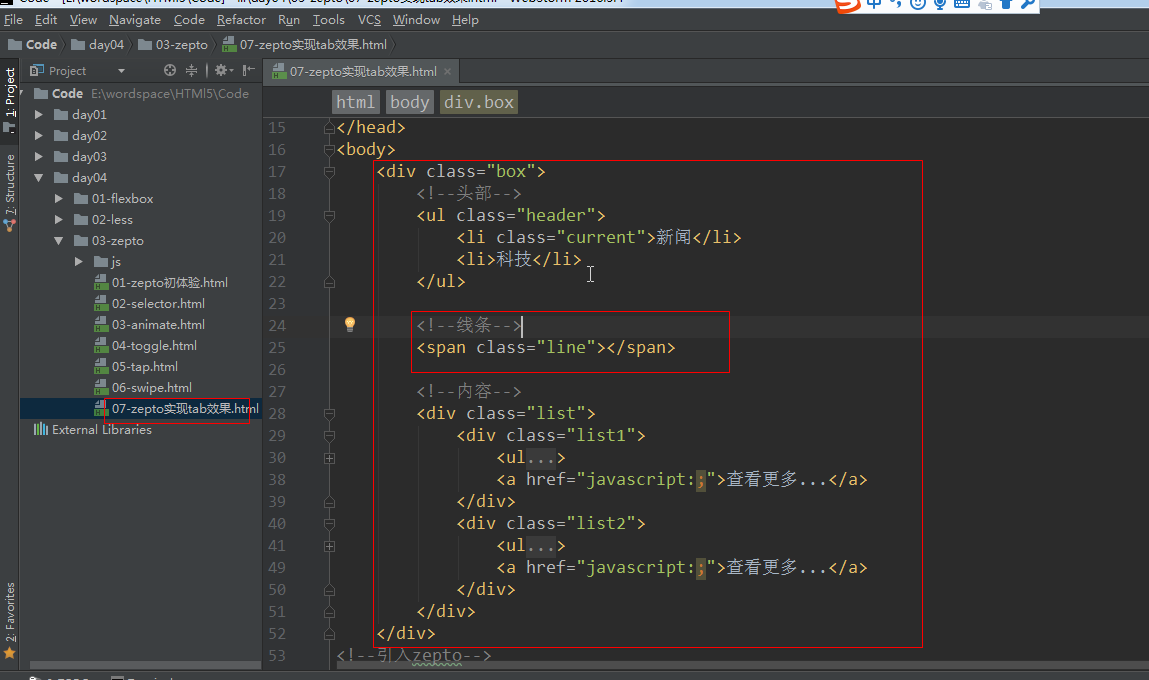
~~~
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<!--
视口:兼容移动
-->
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<!--
告诉ie浏览器使用最新的解析器解析
-->
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>Title</title>
</head>
<body>
<div class="box">
<!--头部-->
<ul class="header">
<li class="current">新闻</li>
<li>科技</li>
</ul>
<!--线条-->
<span class="line"></span>
<!--内容-->
<div class="list">
<div class="list1">
<ul>
<li>新闻内容新闻内容</li>
<li>新闻内容新闻内容</li>
<li>新闻内容新闻内容</li>
<li>新闻内容新闻内容</li>
<li>新闻内容新闻内容</li>
<li>新闻内容新闻内容</li>
</ul>
<a href="javascript:;">查看更多...</a>
</div>
<div class="list2">
<ul>
<li>科技内容新闻内容</li>
<li>科技内容新闻内容</li>
<li>科技内容新闻内容</li>
<li>科技内容新闻内容</li>
<li>科技内容新闻内容</li>
<li>科技内容新闻内容</li>
</ul>
<a href="javascript:;">查看更多...</a>
</div>
</div>
</div>
<!--引入zepto-->
<script src="js/zepto.min.js"></script>
<!--引入touch-->
<script src="js/touch.js"></script>
</body>
</html>
~~~
执行的效果:
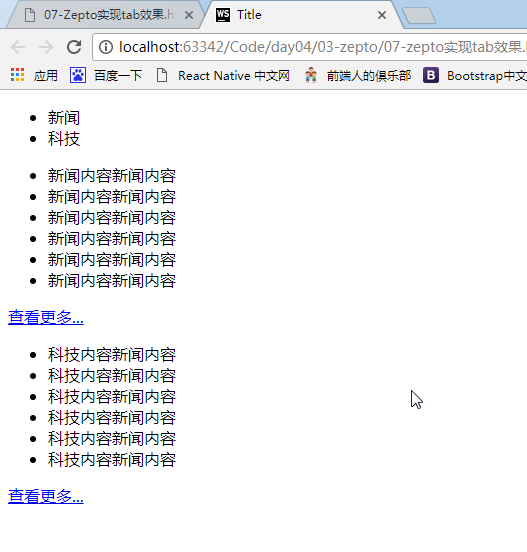
## 3.添加默认的样式
~~~
*{
.....
/*清除系统的触摸事件*/
touch-action: none;
/*盒子大小的计算方式*/
/*box-sizing: border-box;*/
}
/*box里面的盒子要移动,移动要用到定位*/
.box{
position: relative;
}
~~~
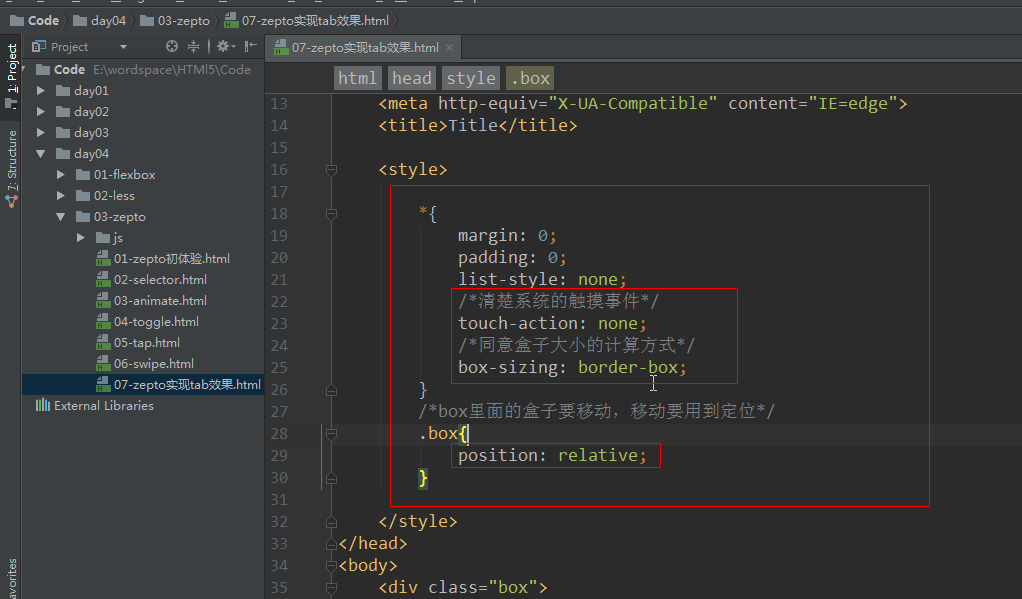
## 4.头部的样式-40-16
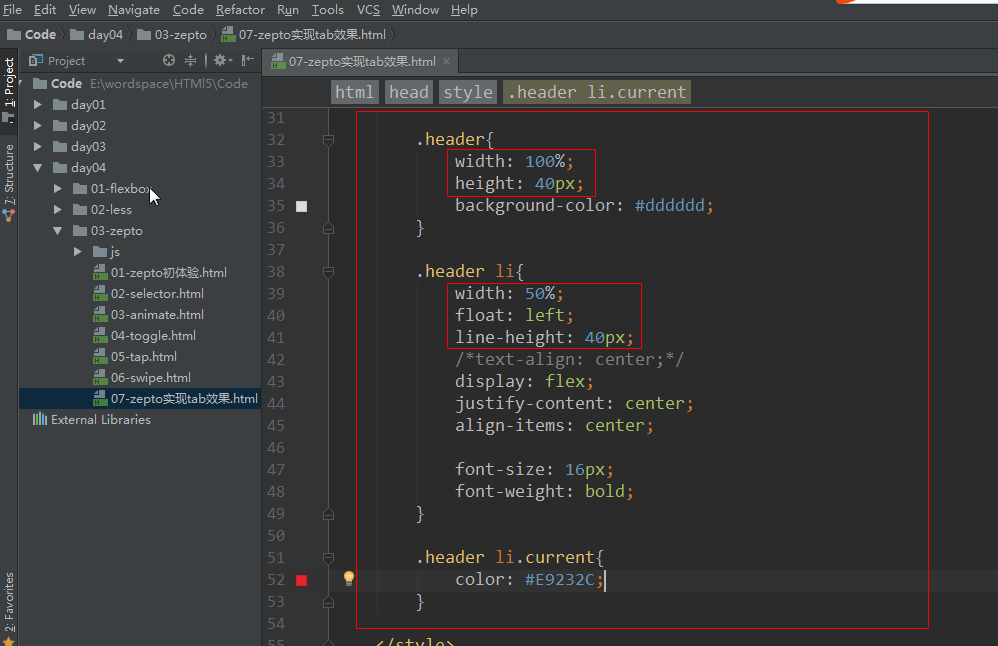
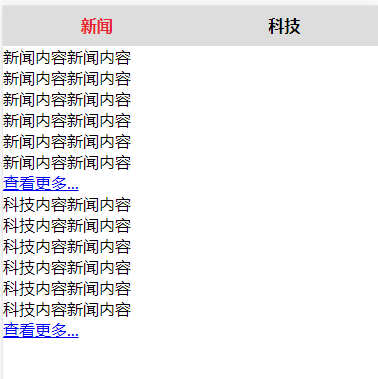
## 5.线条样式-37-3
> 线条span通过定位来改变标签类型,不然宽高不起作用
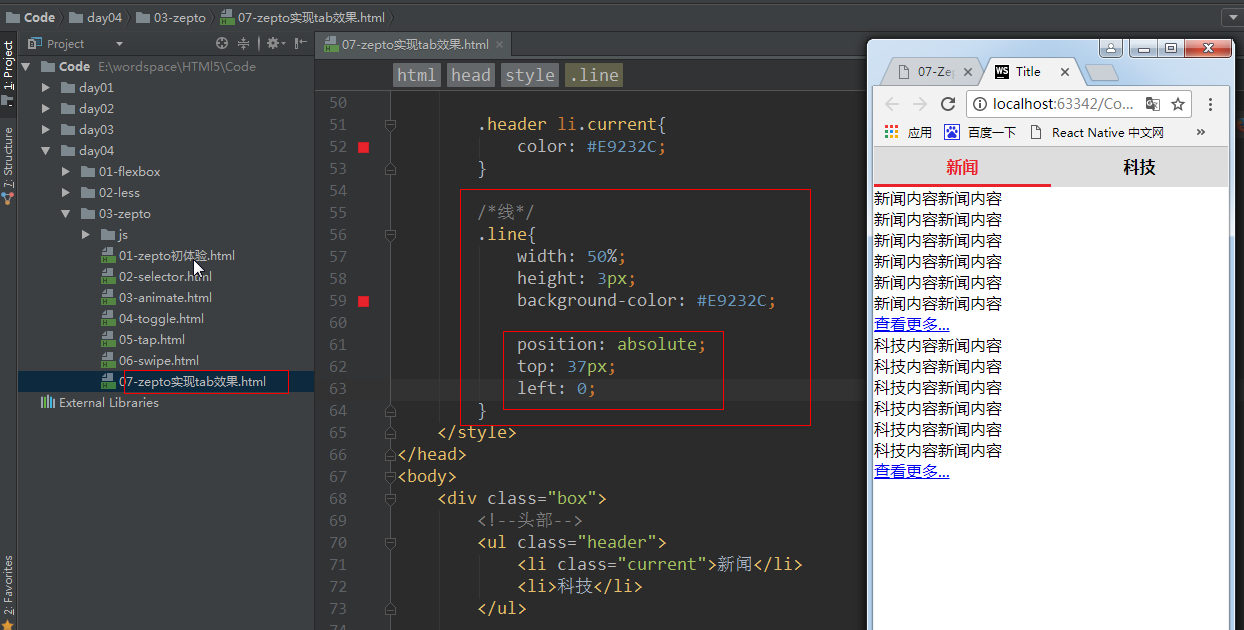
## 6.内容样式
1.list 整个列表的样式
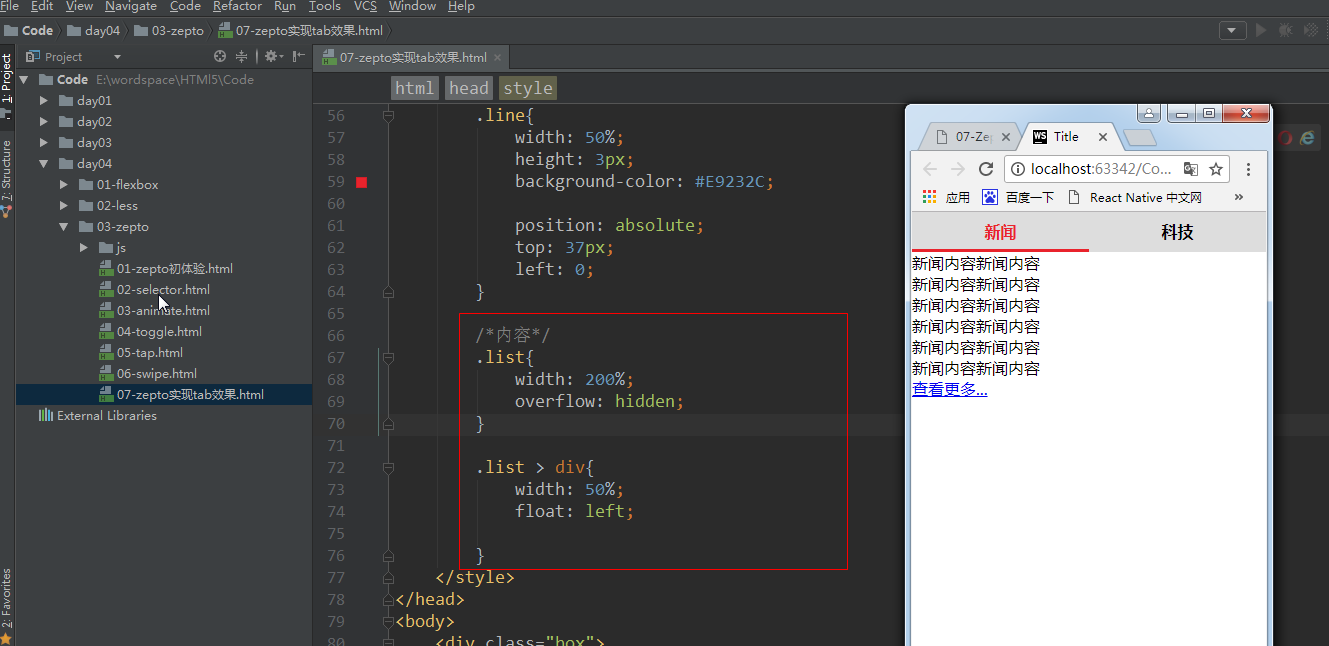
2.列表中ul 和 li的样式
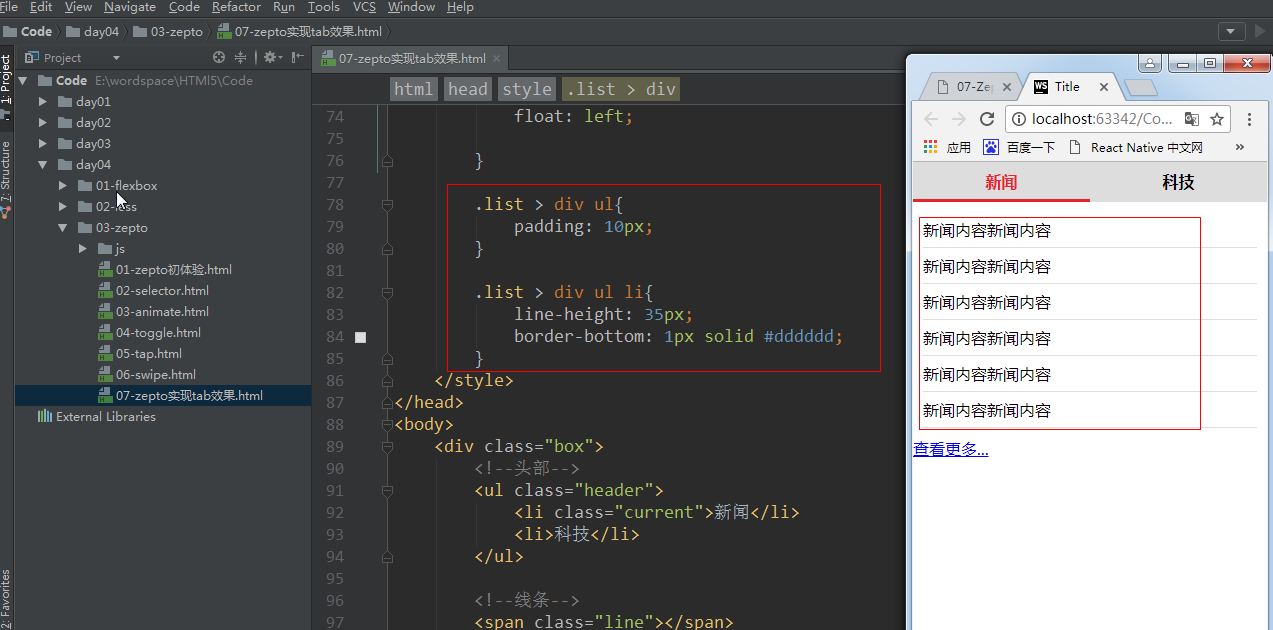
3.查看更多a标签样式
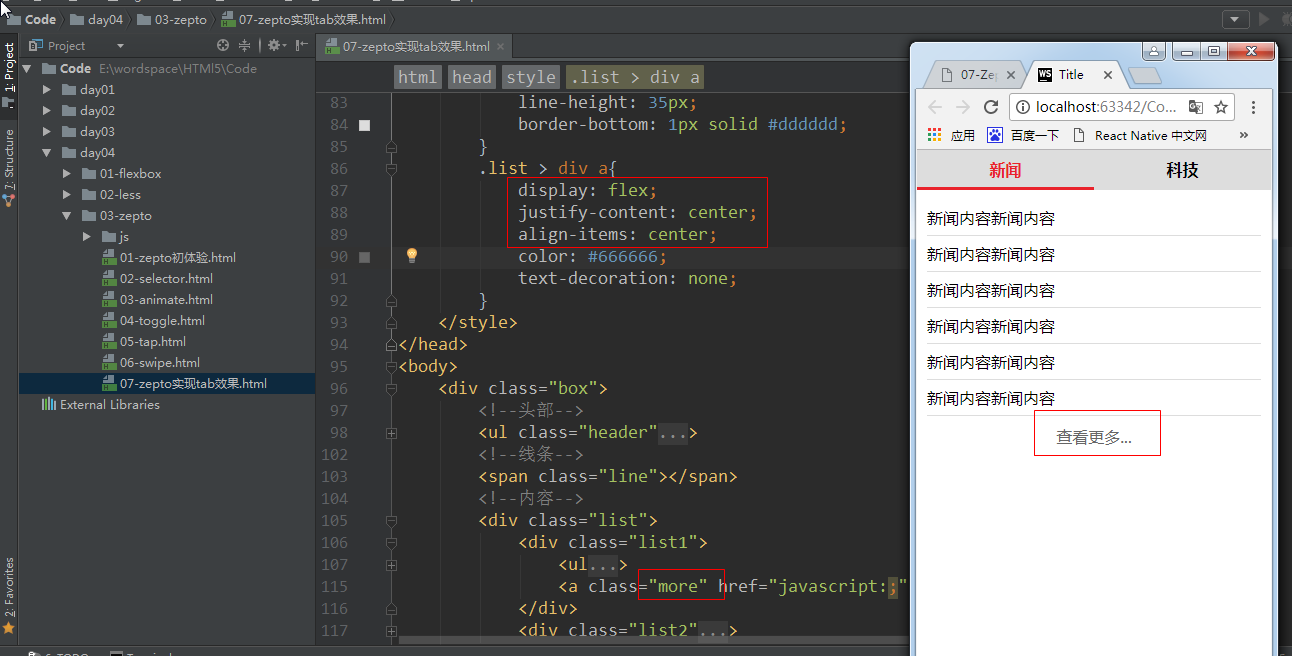
## 7.点击实现切换-tap
### 1.监听头部的点击事件
~~~
$(function () {
//1.头部的点击事件
$('.header li').tap(function () {
//2.拿到点击的索引
var index=$(this).index();
alert(index);
});
})
~~~
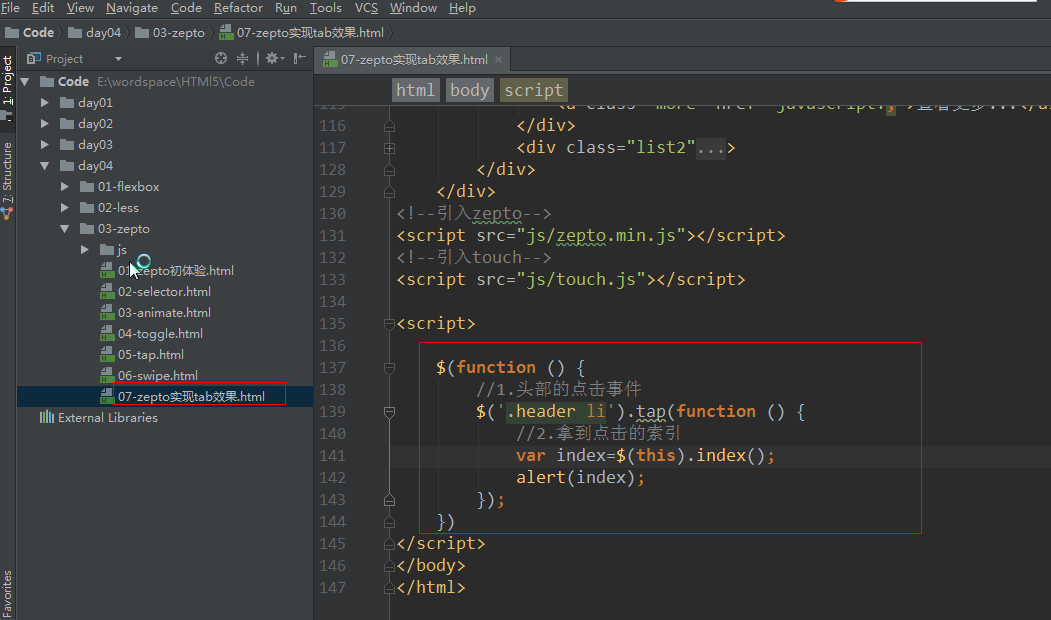
### 2.点击实现切换-css
#### 1.文字的切换
~~~
$(function () {
//1.头部的点击事件
$('.header li').tap(function () {
//2.拿到点击的索引
var index=$(this).index();
// alert(index);
$(this).addClass('current')
/*同级节点,清除样式*/
.siblings().removeClass('current');
});
})
~~~
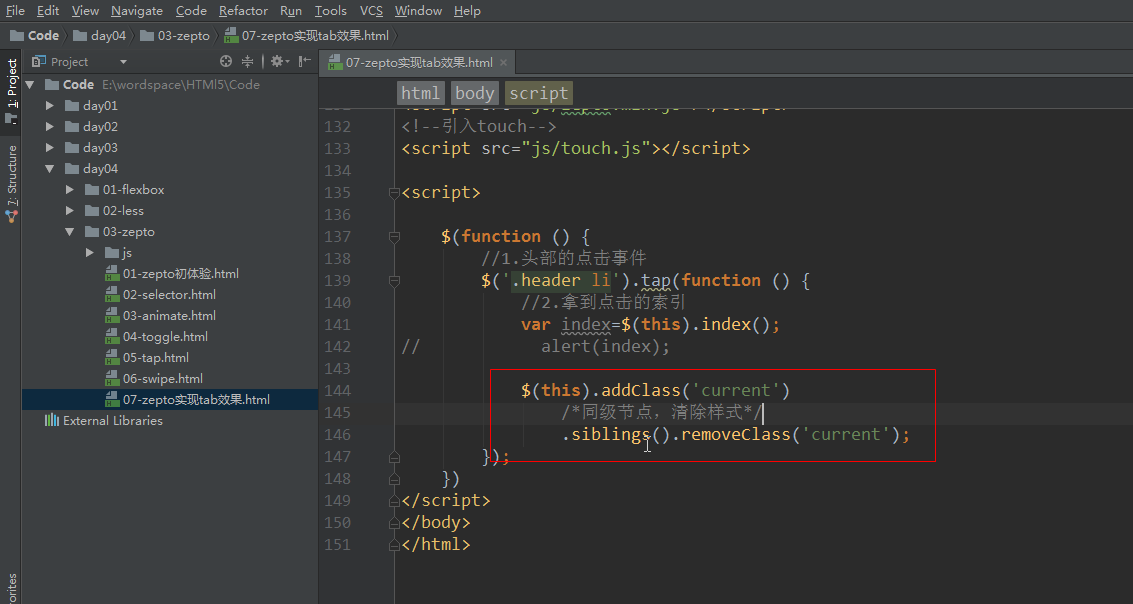
#### 2.线条的移动
~~~
$('.line').css({
transform:'translateX('+ index * 100 +'%)', // 相对自身移动100%
transition:'all 0.35s linear',
})
~~~
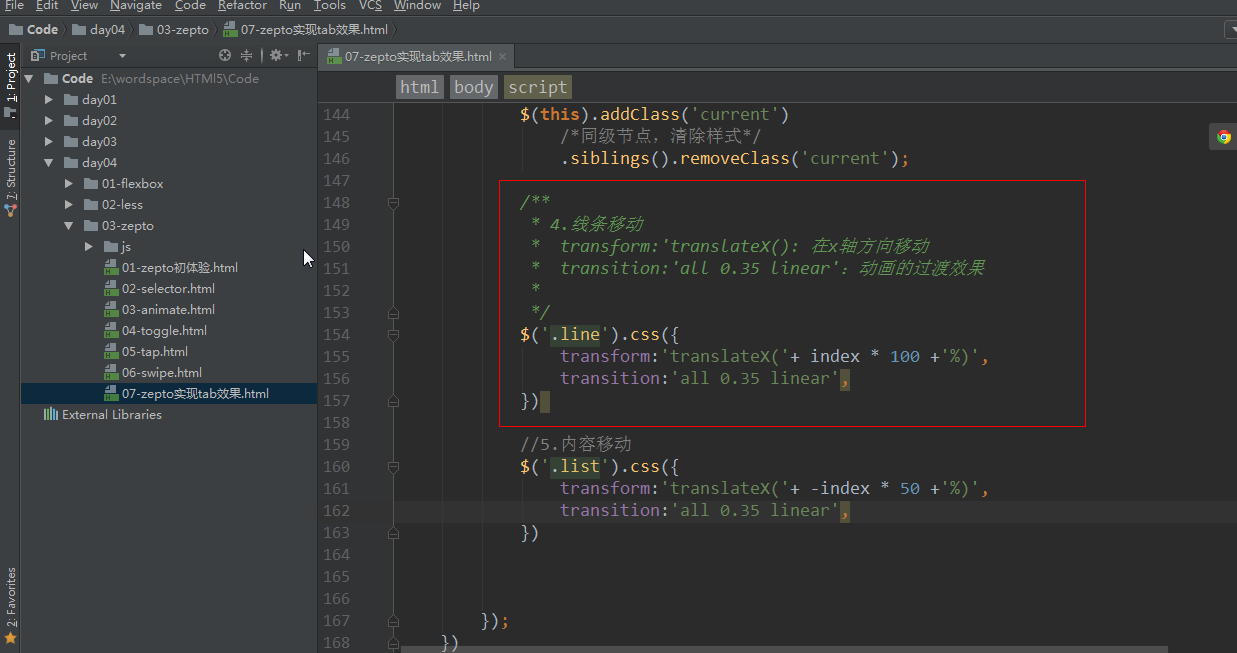
3.内容的移动
~~~
$('.list').css({
transform:'translateX('+ -index * 50 +'%)', // 相对自身移动50%
transition:'all 0.35s linear',
})
~~~
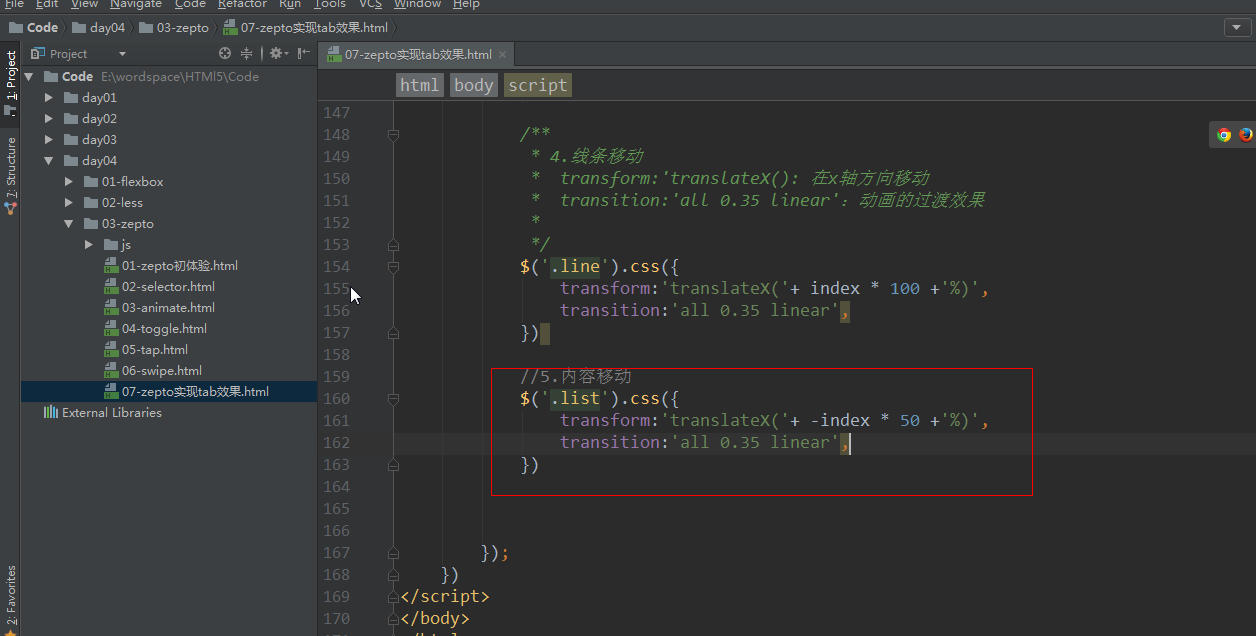
## 8.滑动实现切换
### 1.监听左滑动和右滑
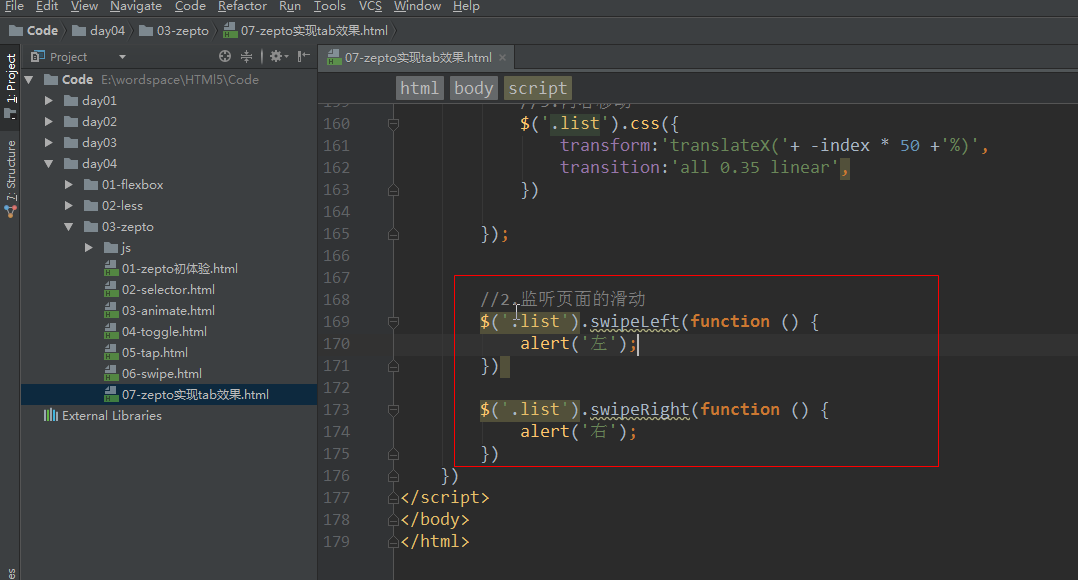
### 2.处理左滑-css
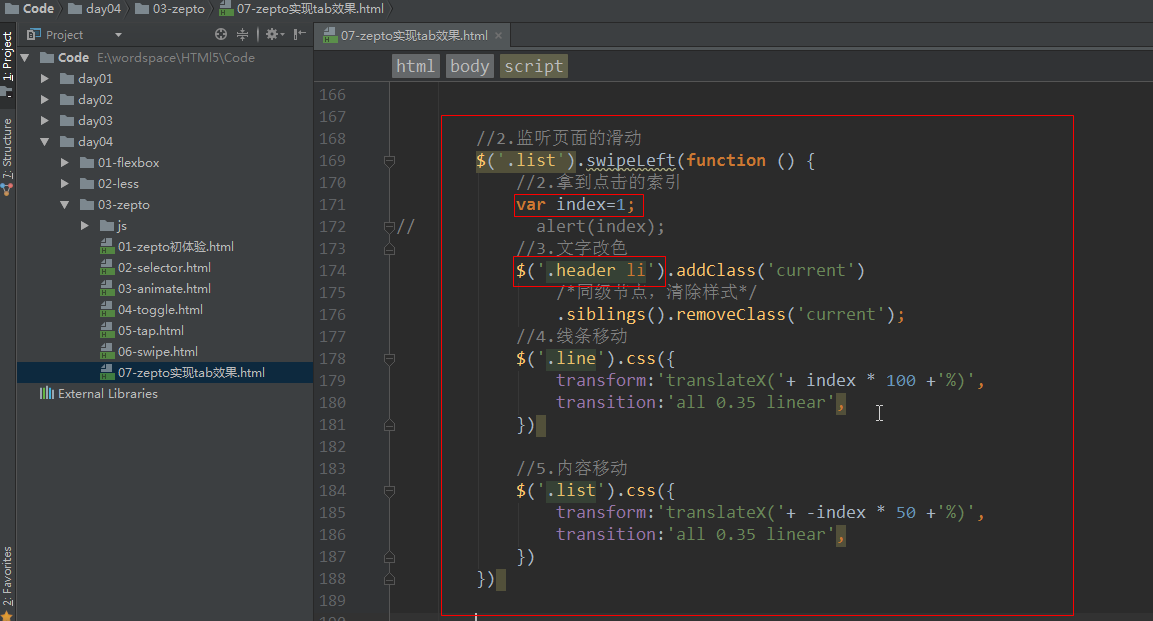
~~~
//2.监听页面的滑动
$('.list').swipeLeft(function () {
//2.拿到点击的索引
var index=1;
// alert(index);
//3.文字改色
$('.header li').eq(index).addClass('current')
/*同级节点,清除样式*/
.siblings().removeClass('current');
//4.线条移动
$('.line').css({
transform:'translateX('+ index * 100 +'%)',
transition:'all 0.35 linear',
})
//5.内容移动
$('.list').css({
transform:'translateX('+ -index * 50 +'%)',
transition:'all 0.35 linear',
})
})
~~~
### 5.处理右滑-css
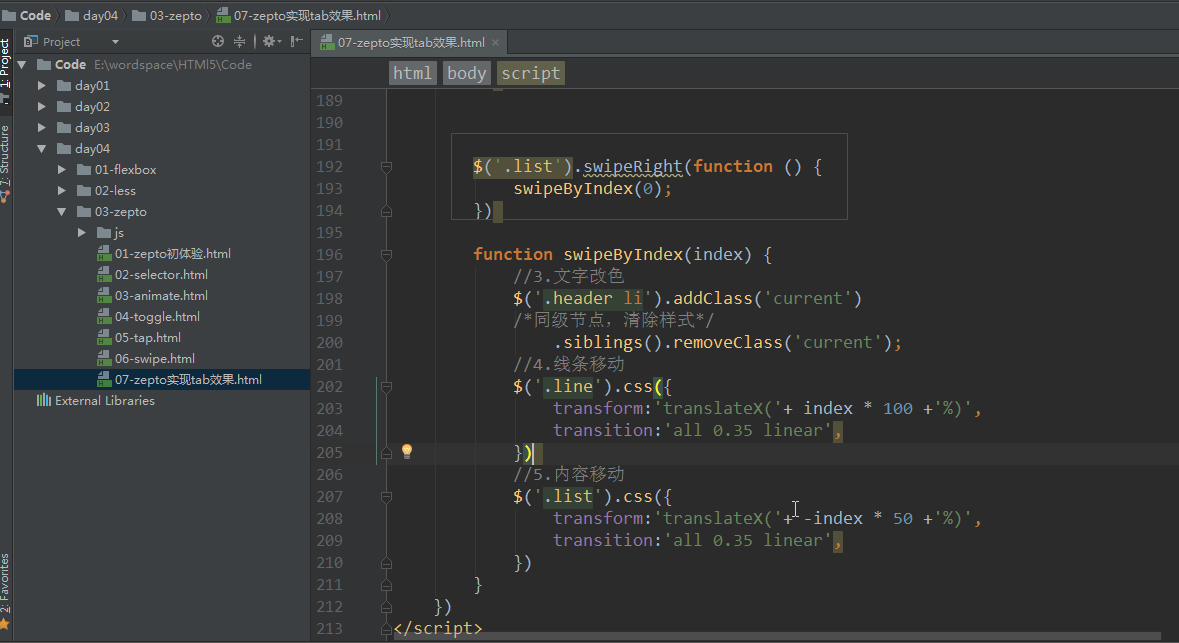