ThinkPHP可以将更新和插入操作合并,并且支持直接读取JSON格式的请求数据。
实现代码:
~~~
public function saveBookInfo()
{
if (Request::isPost()) {
//如果包含ID则更新,否则新增
$data = $this->request->post();
$result = Db::name('book')->save($data);
$response = [
'errno' => 0,
'errmsg' => '数据更新成功',
'data' => $result
];
//返回新增或者更新成功的记录数量
return json($response);
} else {
$response = [
'errno' => 1000,
'errmsg' => '错误的请求',
'data' => null
];
}
}
~~~
代码解读:
1. 首先控制器`Request::isPost()`判断前端提交的请求类型是否是Post类型
2. 通过`$this->request->post()`获取提交的数据。
3. 通过` Db::name('book')->save($data)`直接更新数据库表,如果$data包含主键id字段,则更新,否则插入记录
完整的代码清单:
~~~
<?php
namespace app\controller;
use app\BaseController;
use think\facade\Db;
use think\facade\Request;
class Book extends BaseController
{
/**
* 获取图书的分页数据
*/
public function getBookList($page = 1, $pageSize = 10)
{
// 获取数据集
$books = Db::name('book')->order('id', 'desc')->paginate(['page' => $page, 'list_rows' => $pageSize]);
$books = $books->toArray();
//转换分页器满足约定的接口规范
$data = array(
'count' => $books['total'],
'currentPage' => $books['current_page'],
"pageSize" => $books['per_page'],
"totalPages" => $books['last_page'],
"data" => $books['data']
);
$response = [
'errno' => 0,
'errmsg' => '',
'data' => $data
];
return json($response);
}
/**
* 获取所有的图书列表
*/
public function getAllBook()
{
// 获取数据集
$books = Db::name('book')->select();
$response = [
'errno' => 0,
'errmsg' => '',
'data' => $books
];
return json($response);
}
/**
* 获取图书数据
*/
public function getBookInfo($id = 0)
{
$book = Db::name('book')->where(['id' => $id])->find();
if ($book) {
$response = [
'errno' => 0,
'errmsg' => '',
'data' => $book
];
} else {
$response = [
'errno' => 1000,
'errmsg' => '没有满足条件的数据',
'data' => null
];
}
return json($response);
}
/**
* 删除图书数据
*/
public function deleteBookInfo($id)
{
$result = Db::name('book')->where(['id' => $id])->delete();
$response = null;
$response = [
'errno' => 0,
'errmsg' => '成功删除记录的数量',
'data' => $result
];
return json($response);
}
public function saveBookInfo()
{
if (Request::isPost()) {
//如果包含ID则更新,否则新增
$data = $this->request->post();
$result = Db::name('book')->save($data);
$response = [
'errno' => 0,
'errmsg' => '数据更新成功',
'data' => $result
];
//返回新增或者更新成功的记录数量
return json($response);
} else {
$response = [
'errno' => 1000,
'errmsg' => '错误的请求',
'data' => null
];
}
}
}
~~~
## 测试接口
设置请求的数据格式为`Content-Type = application/json`,然后在body部分输入要更新或者新增的记录的JSON格式的信息。如果包含id字段则执行更新操作,否则执行新增操作。
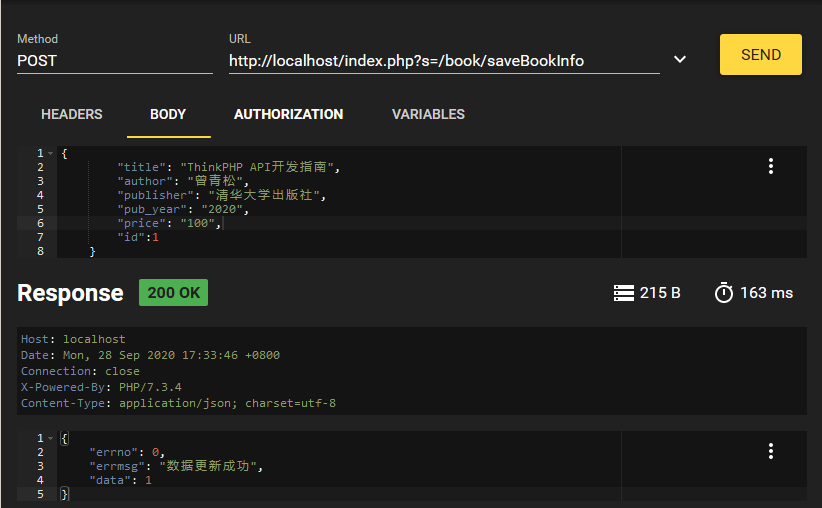