[TOC]
### 一、Vue3.x中的路由
路由可以让应用程序根据用户输入的不同地址动态挂载不同的组件。
[https://next.router.vuejs.org/](https://next.router.vuejs.org/)
~~~
npm install vue-router@next --save
~~~
### **二、Vue3.x路由的基本配置**
#### **1、安装路由模块**
~~~
npm install vue-router@next --save 或者 cnpm install vue-router@next --save
~~~
#### **2、新建组件**
**components/Home.vue**
~~~
<template>
<div>
Home组件
</div>
</template>
<script lang="ts">
import {
defineComponent,
} from 'vue';
export default defineComponent({
name: 'Home',
});
</script>
<style>
</style>
~~~
**components/News.vue**
~~~
<template>
<div>
News组件
</div>
</template>
<script lang="ts">
import {
defineComponent,
} from 'vue';
export default defineComponent({
name: 'News',
});
</script>
<style>
</style>
~~~
#### **3、配置路由**
新建src/routes.ts 配置路由
~~~
import {createRouter,createWebHashHistory} from 'vue-router'
import Home from "./components/Home.vue"
import News from "./components/News.vue"
const router = createRouter({
// 4. Provide the history implementation to use. We are using the hash history for simplicity here.
history: createWebHashHistory(),
routes: [
{ path: '/', component: Home },
{ path: '/news', component: News }
],
})
export default router
~~~
#### **4、挂载路由**
在main.ts中挂载路由
~~~
import { createApp } from 'vue'
import App from './App.vue'
import router from './routes'
// createApp(App).mount('#app')
const app = createApp(App)
//挂载路由
app.use(router)
app.mount('#app')
~~~
#### **5、渲染组件**
App.vue中通过router-view渲染组件
~~~
<template>
<ul>
<li>
<router-link to="/">首页</router-link>
</li>
<li>
<router-link to="/news">新闻</router-link>
</li>
</ul>
<router-view></router-view>
</template>
<script lang="ts">
import {
defineComponent
} from 'vue';
export default defineComponent({
name: 'App',
});
</script>
<style>
</style>
~~~
### 三、Vue3.x动态路由
**1、配置动态路由**
~~~
const router = createRouter({
// 4. Provide the history implementation to use. We are using the hash history for simplicity here.
history: createWebHashHistory(),
routes: [
{ path: '/', component: Home },
{ path: '/news', component: News },
{ path: '/newsContent/:id', component: NewsContent },
],
})
~~~
**2、路由跳转**
~~~
<li v-for="(item,index) in list" :key="index">
<router-link :to="`/newsContent/${index}`">{{item}}</router-link>
</li>
~~~
**3、获取路由**
~~~
this.$route.params
~~~
### 四、Vue3.x Get传值
~~~
<router-link to="/newsContent?id=2">Get传值</router-link>
~~~
~~~
this.$route.query
~~~
### 五、Vue3.x路由编程式导航(Js跳转路由)
~~~
this.$router.push({ path: 'news' })
~~~
~~~
this.$router.push({
path: '/newsContent/495'
});
~~~
~~~
this.$router.push({ path: '/newscontent', query:{aid:14} }
~~~
~~~
this.$router.push({ path: '/newscontent/123'})
~~~
### 六、Vue3.x路由HTML5 History 模式和 hash 模式
#### 6.1、 hash 模式
~~~
import { createRouter, createWebHashHistory } from 'vue-router'
const router = createRouter({
history: createWebHashHistory(),
routes: [
//...
],
})
~~~
~~~
http://localhost:8080/#/user
http://localhost:8080/#/news
~~~
**如果想把上面的路由改变成下面方式:**
~~~
http://localhost:8080/news
http://localhost:8080/user
~~~
我们就可以使用HTML5 History 模式
#### 6.2、 HTML5 History 模式
~~~
import { createRouter, createWebHistory } from 'vue-router'
const router = createRouter({
history: createWebHistory(),
routes: [
//...
]
})
~~~
\*\*注意:\*\*开启Html5 History模式后,发布到服务器需要配置伪静态:
[https://router.vuejs.org/zh/guide/essentials/history-mode.html](https://router.vuejs.org/zh/guide/essentials/history-mode.html)
### 七、Vue3.x命名路由
有时候,通过一个名称来标识一个路由显得更方便一些,特别是在链接一个路由,或者是执行一些跳转的时候。你可以在创建 Router 实例的时候,在 `routes` 配置中给某个路由设置名称。
~~~
const router = new VueRouter({
routes: [
{
path: '/user/:userId',
name: 'user',
component: User
}
]
})
~~~
要链接到一个命名路由,可以给 `router-link` 的 `to` 属性传一个对象:
~~~
<router-link :to="{ name: 'user', params: { userId: 123 }}">User</router-link>
~~~
这跟代码调用 `router.push()` 是一回事:
~~~
this.$router.push({ name: 'user', params: { userId: 123 }})
~~~
这两种方式都会把路由导航到 `/user/123` 路径。
~~~
this.$router.push({name:'content',query:{aid:222}})
~~~
### 八、路由重定向
重定向也在`routes`配置中完成。要从重定向`/a`到`/b`:
~~~
const routes = [{ path: '/home', redirect: '/' }]
~~~
重定向也可以针对命名路由:
~~~
const routes = [{ path: '/home', redirect: { name: 'homepage' } }]
~~~
甚至使用函数进行动态重定向:
~~~
const routes = [
{
// /search/screens -> /search?q=screens
path: '/search/:searchText',
redirect: to => {
// the function receives the target route as the argument
// we return a redirect path/location here.
return { path: '/search', query: { q: to.params.searchText } }
},
},
{
path: '/search',
// ...
},
]
~~~
#### 相对重定向
也可以重定向到相对位置:
~~~
const routes = [
{
path: '/users/:id/posts',
redirect: to => {
// the function receives the target route as the argument
// return redirect path/location here.
},
},
]
~~~
### 九、路由别名
重定向是指用户访问时`/home`,URL将被替换`/`,然后与匹配`/`。但是什么是别名?
**别名`/`as`/home`表示用户访问时`/home`,URL保持不变`/home`,但将被匹配,就像用户正在访问时一样`/`。**
以上内容可以在路由配置中表示为:
~~~
const routes = [{ path: '/', component: Homepage, alias: '/home' }]
~~~
别名使您可以自由地将UI结构映射到任意URL,而不受配置的嵌套结构的约束。使别名以a开头,`/`以使路径在嵌套路由中是绝对的。您甚至可以将两者结合起来,并为数组提供多个别名:
~~~
const routes = [
{
path: '/users',
component: UsersLayout,
children: [
// this will render the UserList for these 3 URLs
// - /users
// - /users/list
// - /people
{ path: '', component: UserList, alias: ['/people', 'list'] },
],
},
]
~~~
如果您的路线包含参数,请确保将其包含在任何绝对别名中:
~~~
const routes = [
{
path: '/users/:id',
component: UsersByIdLayout,
children: [
// this will render the UserDetails for these 3 URLs
// - /users/24
// - /users/24/profile
// - /24
{ path: 'profile', component: UserDetails, alias: ['/:id', ''] },
],
},
]
~~~
### 十、嵌套路由
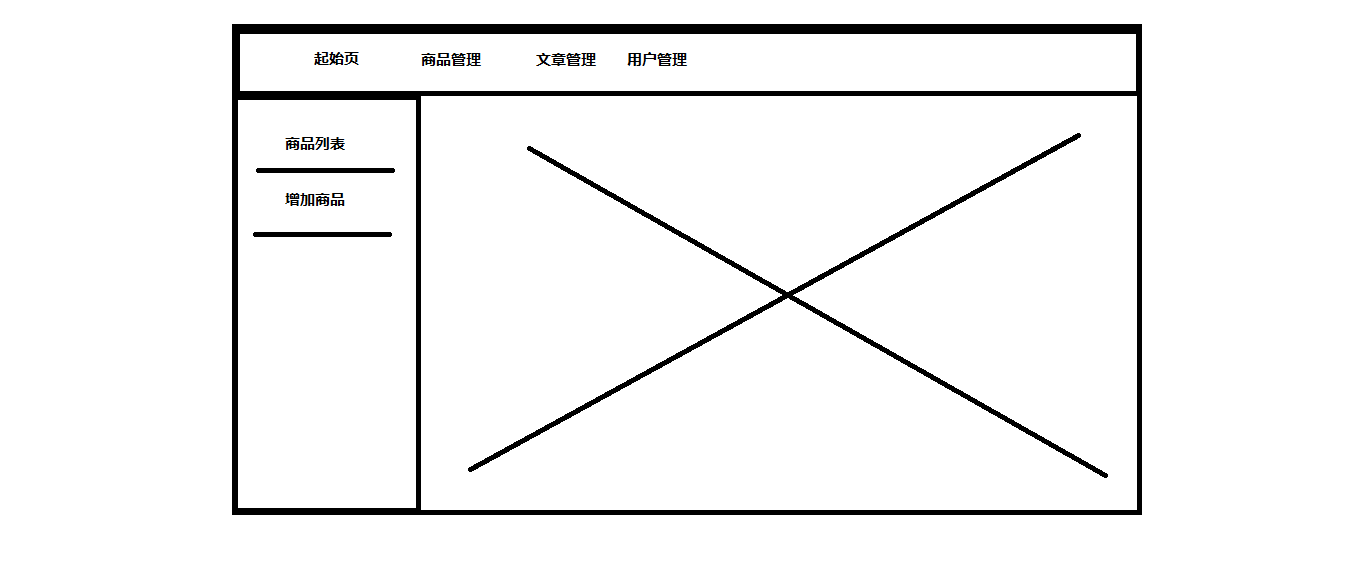
配置News组件的子组件
#### **1、新建news/Add.vue**
~~~
<template>
<div>
增加新闻
</div>
</template>
<script lang="ts">
import { defineComponent } from 'vue';
export default defineComponent({
data(){
return{}
},methods:{
}
})
</script>
~~~
#### **2、新建news/Edit.vue**
~~~
<template>
<div>
修改新闻
</div>
</template>
<script lang="ts">
import { defineComponent } from 'vue';
export default defineComponent({
data(){
return{
}
},methods:{
}
})
</script>
~~~
#### 3、配置嵌套路由
~~~
import { createRouter, createWebHistory } from 'vue-router'
//引入组件
import Home from "./components/Home.vue"
import News from "./components/News.vue"
import NewsAdd from "./components/News/Add.vue"
import NewsEdit from "./components/News/Edit.vue"
import User from "./components/User.vue"
//配置路由
const router = createRouter({
history: createWebHistory(),
routes: [
{ path: '/', component: Home, alias: '/home' },
{
path: '/news', component: News,
children: [ //子路由
{ path: '', redirect:"/news/add"},
{ path: 'add', component: NewsAdd },
{ path: 'edit', component: NewsEdit },
]
},
{ path: '/user', component: User },
],
})
export default router
~~~
#### 4、News.vue中挂载路由
~~~
<template>
<div class="content">
<div class="left">
<ul>
<li><router-link to="/news/add">增加新闻</router-link></li>
<li><router-link to="/news/edit">修改新闻</router-link></li>
</ul>
</div>
<div class="right">
<router-view></router-view>
</div>
</div>
</template>
<script lang="ts">
import { defineComponent } from "vue";
export default defineComponent({
data() {
return {};
},
});
</script>
<style lang="scss">
.content {
display: flex;
padding: 20px;
.left {
width: 200px;
border-right: 1px solid #ddd;
min-height: 400px;
}
.right {
flex: 1;
}
}
</style>
~~~
- vuecli3
- vue cli3 安装
- Vue 引入css 方法
- vue 创建
- vue 组件模板引入
- vue 绑定参数
- vue 方法动态class
- Vue3.x中的事件方法详解、事件监听、方法传值、事件对象、多事件处理程序、事件修饰符、按键修饰符
- Vue3.x中Dom操作$refs 以及表单( input、checkbox、radio、select、 textarea )
- Vue3.x中使用JavaScript表达式 、条件判断、 计算属性和watch侦听
- Vue3.x中的单文件组件 定义组件 注册组件 以及组件的使用
- Vue3.x父组件给子组件传值、Props、Props验证、单向数据流
- 父组件主动获取子组件的数据和执行子组件方法 、子组件主动获取父组件的数据和执行父组件方法
- 组件自定义事件 以及mitt 实现非父子组件传值
- 自定义组件的双休数据绑定。
- 多个input数据进行绑定
- 案例
- Vue3.x中组件的生命周期函数(lifecycle)、 this.$nextTick、动态组件 keep-alive、Vue实现Tab切换
- 全局绑定属性、使用Axios和fetchJsonp请求真实api接口数据、函数防抖实现百度搜索
- Vue3.x中的Mixin实现组件功能的复用 、全局配置Mixin
- Teleport、使用Teleport自定义一个模态对话框的组件
- Vue3.x Composition API 详解
- setup--ref reactive使用案例
- toRefs - 解构响应式对象数据
- computed 计算属性使用
- watchEffect 监听属性
- Provider Injectj介绍
- vue路由重点
- Vuex 中的 State Mutation Getters mapGetters Actions Modules
- Antd 框架操作案例
- 输入框、单选框、多炫酷、时间选择案例
- upload 结合图片上传功能
- Vue结合HIghtchaers 使用
- vue 结合axios 异步通过php获取数据
- vue3结合异步 登录提交数据
- 封装本地存储
- 路由守卫
- vue 结合异步添加数据 拉取数据
- vue 结合异步 编辑 删除数据
- vue 结合挂载先拉取数据,保存数据,判断token 是否过期
- vue 结合moment 转换时间戳为年月日
- vue 结合时间搜索案例
- vue 结合异步 获取antd 饼状图 柱状图数据
- Vue 街composition Api 异步获取数据案例
- vue3 安装typescript
- 组件基本介绍
- swoole 手册
- PCNTL
- Server
- set 设置进程
- Server 属性
- On 回调函数四种用法
- 回到函数属性
- 异步任务投递
- 心跳检测
- 定时器
- Thinkphp6个人使用案例
- Tp6 视图下载
- Log 日志
- ThinkPhp - 中间件
- Thinkphp - 中间件全局上
- Thinkphp - 中间件路由/控制器 下
- Tp6.0 操作redis
- 分析日志/qps
- Tp验证码 自刷新功能
- TP6创建多应用模式
- thinkphp6.0路由
- 自定义验证规则
- Tp6 数据表隐藏字段
- with 关联 表,进行倒叙排列
- 采坑案例
- 使用postman 不带htpp请求都是get请求
- 购物车批量传入多个数据,如何进行验证
- 查看自己thinkphp版本
- 查看自己安装swoole 版本
- 查看Tp 执行sql语句
- thinkphp - 在线更新自己版本
- Thinkphp - 连接多个数据库
- Thinkphp - 数据库的时间查询
- Tphinkphp - 聚合.原生.子查询
- Thinkphp - limit/page/order/group/having
- Thinkphp - 数据集
- Thinkphp- 验证器
- 验证器定义
- 验证规则和错误信息
- 验证场景和路由验证
- 验证内置规则
- 单个验证和注解验证
- Thinkphp - phpspreadsheet 导出excel
- ThinkPhp - 图像处理功能
- ThinkPhp- 异常处理
- Thinkphp - 门面介绍
- Thinkphp - 事件
- Thinkphp - 全局异常类封装
- 全局验证代码
- Thinkphp - 控制器创建规则
- Thinkphp-6.0 符合查询(重要)
- Thinkphp - 在自己模型类处理数据
- Tp6 结合swool使用/演示案例(重点观看)
- swoole 配置文件
- swoole websocket 发送信息
- Task 异步任务投递
- 连接池
- Table 高性能共享内存
- 原生态操作
- Tp6 操作
- RPC操作教程
- 协程
- Swool结合tp6 演示操作(重点)
- swoole 安装
- 基本介绍
- swoole 在linux 查看版本
- 进程跟协程区别
- swoole基础-TCP服务
- swoole基础-UDP服务
- swoole基础-HTTP服务
- swoole基础-WebSocket服务
- swoole基础-TASK异步任务
- swoole基础-一键协程
- swoole基础-协程 MySQL 客户端
- swoole基础-协程 Redis 客户端
- swoole基础-毫秒定时器
- swoole基础-高性能内存操作table
- think-swoole应用-HTTP请求和热更新
- think-swoole应用-进程设置
- think-swoole应用-启用数据库连接池
- think-swoole应用-异步TASK发送短信任务
- think-swoole应用-集成think-queue消息队列,优化异步发短信任务,支持任务重试机制
- think-swoole应用-毫秒定时器取消超时订单
- think-swoole应用-高性能共享内存table应用
- think-swoole应用-微服务之RPC远程调用通信实战
- think-swoole应用-websocket消息、群发广播
- Nginx负载均衡部署-转发swoole服务
- Typescript
- Typescript类型介绍
- Linxu从入门到精通
- 基本命令
- shell 命令
- Linux 目录查看
- 文件基本操作
- 目录操作
- 打包解压
- 用户管理 和 用户权限管理 chmod、ACL、 visudo
- Yum 安装
- 进程查看跟关闭
- Linux 内存、cpu、进程、端口、硬盘管理
- systemctl 管理服务、防火墙 firewalld 以及 SELinux 配置
- Linux - ps命令
- ps -ef【查看所有进程】
- ps -ef | grep ssh 【查找某一进程】
- kill 2868 【杀掉2868编号的进程】
- kill -9 2868 【强制杀死进程】
- Linux - 管道
- Linux - 主机名配置
- Linux - ip地址配置
- Linux - 防火墙
- Nginx 配置文件详细说明
- Linux 信号
- Linux系统目录
- 微信小程序从入门到精通
- 微信小程序页面文件组成
- 自定义组件
- 创建组件
- 父子组件传值
- Solt
- 父组件调用子组件方法/子组件数据
- 子组件调用父组件方法
- 给自定义组件加一个属性
- 外部样式类设计
- 创建一个自定义组件,外部引用
- wxs 使用
- 模块化把公共的功能封装成
- 解析html 代码
- 异步请求数据
- 页面之间传值
- 页面跳转
- 全局 app.json 介绍
- 微信小程序数据绑定
- 基本数据绑定
- 对象数据绑定
- 控制属性绑定
- 数组循环
- 数组对象循环
- 多层数组嵌套
- 微信小程序事件、方法、事件对象、方法传值、获取data数据、修改data数据、冒泡非冒泡事件
- 微信小程序自定义方法
- 事件对象
- 获取data数据
- 修改data数据
- 冒泡事件
- 方法传值
- 上拉加载数据
- from 提交数据
- picker 左右联动多列选择器
- 微信小程序拍照上传
- 微信小程序存储本地数据
- 获取位置/查看位置/选择位置/系统信息
- 扫一扫/拔打电话/用户截屏事件/振动/手机联系人
- 微信小程序生成二维码
- 小程序导入数据 导出数据
- 定义全局变量
- 下拉刷新
- 微信小程序结合php后台接口
- openid跟unid 区别
- 配置导航栏标题
- 15秒之后进行跳转
- Css介绍
- Flex 弹性布局
- 把盒子打成弹性盒模型
- 弹性盒模型排列方向
- 盒模型宽度不够溢出处理
- 排列方向跟溢出统一代码
- 控制主轴进行居中
- 控制交叉轴 就是数轴
- 对单个交叉轴控制
- 调节每一个盒子里面的大小布局;类似于栅格化
- 主轴缩小比例
- 放大 缩小 定义基准尺寸
- 弹性盒子排序
- 图片文字左右居中
- 出现省略号功能
- 文字溢出代码
- Rest
- 什么是Rest
- RestFul api 最佳实践
- Js个人案例
- JSON.stringify()与JSON.parse()的区别
- Jquery 个人案例
- jquery操作表单清空
- jquery 五秒后执行一段代码
- jquery ajax 提交表单携带file
- Jquery strr方法
- Html 块元素 内联元素
- Jquery 全选 全不选功能
- Jquer Htm 表单新语法
- ajax 异步支付3秒钟检测支付情况
- 对象转字符串
- Mysql
- mysql 基本语法
- 连接数据库
- 展示数据库
- 创建数据库
- 选择数据库
- 删除数据库
- 判断数据库是否被删除
- 创建数据库表
- 展示表结构
- 删除数据表
- mysql 中级操作
- 主键自增
- 创建一个用户表
- 创建一个完整数据库数据
- 复制表结构
- 复制表数据
- 复制表名字跟表数据
- 修改数据库内容
- 起别名
- where 条件 升序 降序
- in(),在...里面
- like 模糊匹配 %
- 删除数据库里面内容
- 修改数据库表表名
- 修改数据库表表字段名字
- 修改数据库表类型
- 清空所有数据,让id重新从1开始
- 添加字段
- 删除地段
- mysql - 慢查询
- php7/函数方法案例
- json 转换
- php传址
- 对象
- Static 静态
- 对象三种权限
- 类常量
- 魔术常量
- 自动加载
- 抽象类 抽象方法
- 检测变量是否存在
- 删除变量
- php 类型
- php 转义符
- Php 定界符
- 获取字符串长度
- 清楚字符串左右空白
- 加密 explode 跟 implode区别
- 中文截取
- Php 常量
- php 三元表达式
- php ?? 表达式
- swith 循环
- while 循环
- do while循环
- 接受参数不定的数据
- 函数强制转换
- 数组
- 数组合并
- 数组组建转换大小写
- 数组序列化
- 计算指定日期差多少天
- 计算增加多少天
- 正则匹配
- 正则匹配好字符
- 正则拆分
- 正则网站匹配
- UniApp
- 引入全局样式
- 接口问题去
- MongnDb
- MonDb -介绍
- MongoDb 基本操作
- 创建
- 创建单个文档
- 创建多个文档
- 读取操作
- 读取
- 逻辑操作符
- 数组操作符
- 运算操作符
- 游标
- 投射
- 更新操作
- db.collection.update()
- $set操作
- $unset 操作
- 数组更新操作
- $addToset
- $pull
- $pullAll
- $Push
- $pop
- 删除操作
- PHP操作MongoDB(增删改查)
- MongoDb = 聚合操作
- $project
- $match
- $limit 和 $skip
- $unwind
- $sort
- MongonDb - 索引
- 创建索引
- 创建一个复合索引
- Laravel 操作 MongoDb
- php操作
- 聚合查询
- Docker
- 前端常用12种正则表达式
- Nginx
- Nginx 负载均衡s
- Nginx - 概念
- Nginx - 应用场景
- Nginx - 配置文件
- Nginx - 基本命令
- Nginx - 域名绑定
- Nginx - 反向代理
- 反向代理 - 简介
- 反向代理 - 配置
- Nginx - 负载均衡
- 负载均衡 - 准备工作
- 负载均衡 - 开始配置
- 消息队列系统
- WebSocket
- 官方网站
- 安装说明
- 基础案例
- 框架图片
- Worker介绍
- Worker
- 类的属性
- 回调属性
- worker代码分析
- Connection
- Connection 类
- 介绍
- 文件目录
- Tp框架配置
- Tp 使用
- 订阅类代码
- PHP - 附加
- cgi、php-cgi 、fastcgi 、php-fpm
- PHP- 面试案例
- Redis 常用
- Php 个人案例代码
- 去除二维数组重复数据
- php 获取本月最后一天
- FTP服务器架设
- Crontab任务计划
- Php8新特性
- 第1章:LNP Web环境搭建
- 1-1 Nginx1.19源码编译安装
- 1-2 Nginx1.19环境配置
- 1-3 Nginx1.19性能优化与测试
- 1-4 PHP8.0源码编译安装
- 1-5 PHP8.0环境配置
- 1-6 PHP8.0性能优化与测试
- 第2章:JIT即时编译
- 2-1 JIT编译原理
- 2-2 Tracing JIT和Function JIT编译引擎
- 2-3 Opcodes编译原理
- 2-4 Opcache和JIT功能开启
- 2-5 JIT高性能测试
- 第3章:PHP8的主要新特性
- 3-1 php8的命名参数
- 3-2 Reflection反射
- 3-3 注解
- 3-4 构造器属性提升
- 3-5 联合类型
- 3-6 Nullsafe空安全运算符
- 3-7 Match表达式
- 第4章:PHP8的新功能和类
- 4-1 PhpToken类
- 4-2 Stringable接口
- 4-3 WeakMap类
- 4-4 Str_contains函数
- 4-5 Str_starts_with和Str_ends_with函数
- 4-6 Fdiv函数
- 4-7 Get_resource_id函数
- 4-8 Get_debug_type函数
- 第5章:类型系统改进
- 5-1 新的Mixed伪类型
- 5-2 Static类方法的返回类型
- 第6章:错误处理方面的改进
- 6-1 系统函数引发TypeError和ValueError异常
- 6-2 Throw表达式抛出异常
- 6-3 无变量捕获的Catch
- 6-4 默认错误报告设置为E_ALL
- 6-5 默认情况下显示PHP启动错误
- 6-6 Assert断言默认情况下引发异常
- 6-7 操作符@不再抑制Fatal错误
- 6-8 PDO默认错误模式为ERRMODE_EXCEPTION
- 第7章:资源到对象的迁移
- 7-1 GdImage类对象替换了GD映像资源
- 7-2 CurlHandle类对象替换Curl处理程序
- 7-3 套接字扩展资源Socket是类对象
- 7-4 XMLWriter对象替换xmlwriter资源
- 第8章:PHP面向对象的编程更改
- 8-1 不兼容的方法签名的致命错误
- 8-2 严格执行类魔术方法签名
- 8-3 静态调用非静态类方法会导致致命错误
- 8-4 继承规则不适用于Private类方法
- 8-5 对象支持Class魔术常量
- 第9章:与字符串相关的更改
- 9-1 Substr和Iconv_substr偏移越境返回空字符串
- 9-2 加减运算符优先级高于点连接符
- 第10章:其他功能与特性
- 10-1 Printf采用新精度和宽度修饰符
- 10-2 内置Web服务器支持动态端口选择
- 10-3 参数列表和闭包Use列表中允许结尾逗号
- 10-4 隐式负数组键增量不会跳过负数
- 10-5 Crypt函数Salt为必选参数
- 10-6 调用禁用函数或类为未定义状态
- 10-7 可选参数之后禁止出现必选参数
- 第11章:弃用的函数与方法
- 11-1 ReflectionFunction::isDisabled弃用
- 11-2 ReflectionParameter::getClass弃用
- 11-3 ReflectionParameter::isArray弃用
- 11-4 ReflectionParameter::isCallable弃用
- 11-5 ReflectionClass::export弃用
- 11-6 ReflectionFunction::export弃用
- 11-7 Get_defined_functions改进禁用函数
- 11-8 24个PostgreSQL的别名函数弃用
- swooletw/laravel-swoole
- Git 常用命令
- 常用
- 别名 alia
- 创建版本库
- 修改和提交
- 查看历史
- 撤销
- 分支与标签
- 合并与衍合
- 远程操作
- 打包
- 全局和局部配置
- 远程与本地合并
- laravel 使用 Memcached