## 1. 获取内置浏览器标识
```javascript
/**
* 微信
*
* @return {Boolean}
*/
export function isWeixin() {
let ua = window.navigator.userAgent.toLowerCase()
if(ua.match(/MicroMessenger/i) == 'micromessenger') {
return true
}
return false
}
```
```javascript
/**
* 支付宝
*
* @return {Boolean}
*/
export function isAlipay() {
let ua = window.navigator.userAgent.toLowerCase()
if(ua.match(/Alipay/i) == "alipay") {
return true
}
return false
}
```
## 2. methods(数据处理)
**couponOrder接口返回** (**调用支付前** 接口返回所需的支付参数信息)
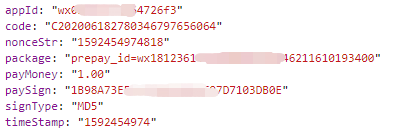
## 3.支付
### 微信支付(两种方法):
1. JSAPI支付:在微信支付菜单栏中,有一个使用教程。里面有一个使用JS API发起支付请求的小菜单
> WeixinJSBridge.invoke()
>
> * WeixinJSBridge 内置对象在其他浏览器中无效。
>
> * getBrandWCPayRequest 参数以及返回值定义
```javascript
WeixinJSBridge.invoke('getBrandWCPayRequest', {
'appId': params.appId, // 公众号id
'timeStamp': params.timeStamp, // 当前的时间戳
'nonceStr': params.nonceStr, // 支付签名随机字符串
'package': params.package, // 订单详情扩展字符串,prepay_id=****
'signType': params.signType, // 签名类型,默认为MD5
'paySign': params.paySign // 支付签名
}, (res) => {
if (res.err_msg === 'get_brand_wcpay_request:ok') {
this.toSuccess(code, payMoney, payTool)
} else if (res.err_msg === 'get_brand_wcpay_request:fail') {
MessageBox('错误提示', "支付失败")
}else if (res.err_msg === 'get_brand_wcpay_request:cancel') {
MessageBox('错误提示', "支付失败")
}
}
)
```
参考文档:[https://pay.weixin.qq.com/wiki/doc/api/jsapi.php?chapter=7\_7&index=6](https://pay.weixin.qq.com/wiki/doc/api/jsapi.php?chapter=7_7&index=6)
2. 微信JS-SDK说明文档发起微信支付的请求API
> wx.chooseWXPay() 需签名后调用
```javascript
wx.chooseWXPay({
timestamp: 0, // 支付签名时间戳,
nonceStr: '', // 支付签名随机字符串,不长于 32 位
package: '', // 订单详情扩展字符串
signType: '', // 签名方式,默认为'SHA1',使用新版支付需传入'MD5'
paySign: '', // 支付签名
success: (res) => {
// 支付成功后的回调函数
}
})
```
### 支付宝支付 AlipayJSBridge.call
```javascript
AlipayJSBridge.call('tradePay', {
orderStr: response.ali
}, (result) => {
let resultCode = result.resultCode
if (resultCode == '9000') {
this.toSuccess(code, payMoney, payTool)
} else if (resultCode == '4000') {
MessageBox('错误提示', '支付失败')
} else if (resultCode == '6001') {
MessageBox('错误提示', '支付取消')
}
})
```
参考文档: [http://myjsapi.alipay.com/jsapi/native/trade-pay.html](http://myjsapi.alipay.com/jsapi/native/trade-pay.html)
```javascript
toSuccess(code, payMoney, payTool) {
this.$router.push({
path: '/coupon_pay_success?code=' + code
+ "&sellerName=" + encodeURIComponent(this.sellerName)
+ "&name=" +encodeURIComponent(this.name)
+ "&payMoney=" + payMoney
+ "&payTool=" + payTool + "&count=" + this.num
+ "&sellerId=" + this.sellerId
+ "&memberId=" + this.memberId
})
}
```
原文链接:https://blog.csdn.net/weixin_43924228/article/details/100580439