网站一般都有banner轮播图,我们该怎么建表呢
一个banner_item就代表banner中的其中一个图,也就是banner_item
**banner**
| 字段 | 说明 |
| --- | --- |
| id | |
| name | |
| description | |
| del_time | |
| update_time | |

**banner_item**
| 字段 | 说明 |
| --- | --- |
| id | |
| img_id | |
| key_word | |
| del_time | |
| banner_id | |
| update_time | |
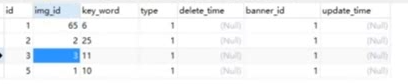
**image**
| 字段 | 说明 |
| --- | --- |
| id | |
| url| |
| from| |
| del_time | |
| update_time | |
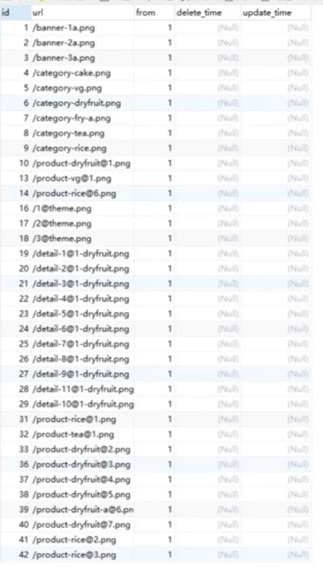
我们在banner控制器查询的时候使用with会将关联数据一起查询出来
直接查询:$banner = BannerModel::find($id)
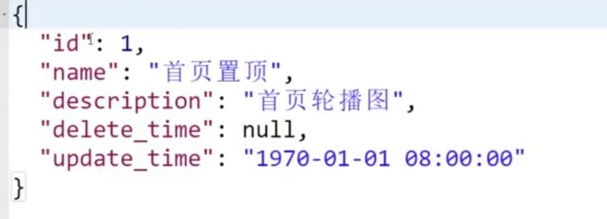
关联查询: $banner = BannerModel::with('items')->find($id)
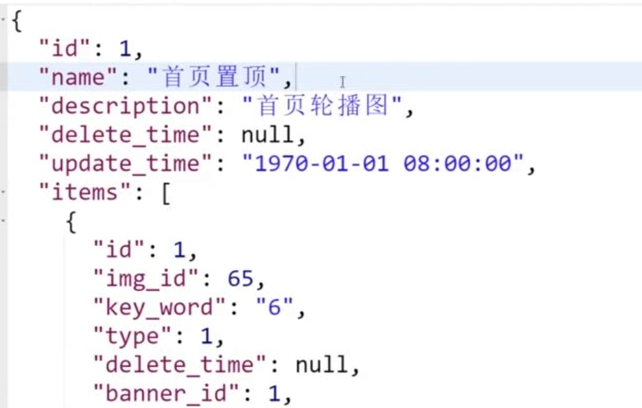
嵌套关联查询: $banner = BannerModel::with('items','items.img')->find($id)
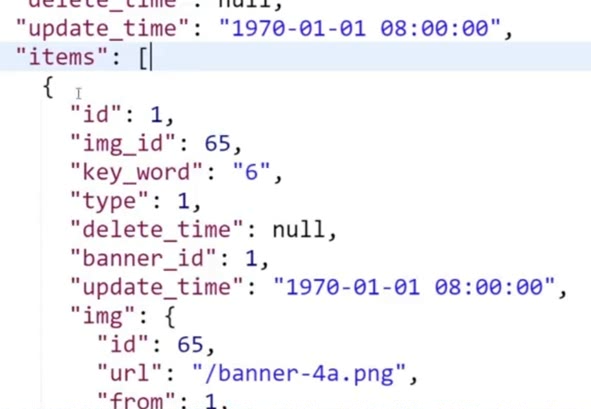
所以我们进一步优化封装代码
banner控制器
```
namespace app\api\controller\v1;
use app\api\controller\BaseController;
use app\api\validate\IDMustBePositiveInt;
use app\api\model\Banner as BannerModel;
use app\lib\exception\MissException;
/**
* Banner资源
*/
class Banner extends BaseController
{
// protected $beforeActionList = [
// 'checkPrimaryScope' => ['only' => 'getBanner']
// ];
/**
* 获取Banner信息
* @url /banner/:id
* @http get
* @param int $id banner id
* @return array of banner item , code 200
* @throws MissException
*/
public function getBanner($id)
{
$validate = new IDMustBePositiveInt();
$validate->goCheck();
$banner = BannerModel::getBannerById($id);
if (!$banner ) {
throw new MissException([
'msg' => '请求banner不存在',
'errorCode' => 40000
]);
}
return $banner;
}
}
```
Banner模型
```
namespace app\api\model;
use think\Model;
class Banner extends BaseModel
{
//方法名可以随意,但是with参数必须和此方法名一致,并且,此方法名也是返回数据的数组的键名
public function items()
{
return $this->hasMany('BannerItem', 'banner_id', 'id');
}
//
/**
* @param $id int banner所在位置
* @return Banner
*/
public static function getBannerById($id)
{
$banner = self::with(['items','items.img'])
->find($id);
// $banner = BannerModel::relation('items,items.img')
// ->find($id);
return $banner;
}
}
```
BannerItem模型
```
namespace app\api\model;
use think\Model;
class BannerItem extends BaseModel
{
protected $hidden = ['id', 'img_id', 'banner_id', 'delete_time'];
public function img()
{
return $this->belongsTo('Image', 'img_id', 'id');
}
//
}
```
**Image **
```
namespace app\api\model;
use think\Model;
class Image extends BaseModel
{
protected $hidden = ['delete_time', 'id', 'from'];
public function getUrlAttr($value, $data)
{
return $this->prefixImgUrl($value, $data);
}
}
```
**BaseModel**
```
namespace app\api\model;
use think\Model;
use traits\model\SoftDelete;
class BaseModel extends Model
{
// 软删除,设置后在查询时要特别注意whereOr
// 使用whereOr会将设置了软删除的记录也查询出来
// 可以对比下SQL语句,看看whereOr的SQL
use SoftDelete;
protected $hidden = ['delete_time'];
protected function prefixImgUrl($value, $data){
$finalUrl = $value;
if($data['from'] == 1){
$finalUrl = config('setting.img_prefix').$value;
}
return $finalUrl;
}
}
```
- 空白目录
- php语法结构
- 安装与更新
- 开启调试模式及代码跟踪器
- 架构
- 源码分析
- 应用初始化
- 请求流程
- 中间件源码分析
- 请求处理源码分析
- Request源码分析
- 模板编译流程
- 路由与请求流程
- 容器
- 获取目录位置
- 入口文件
- 多应用模式及URL访问
- 依赖注入与容器
- 容器属性及方法
- Container
- App
- facade
- 中间件(middleware)
- 系统服务
- extend 扩展类库
- 笔记
- 配置
- env配置定义及获取
- 配置文件的配置获取
- 单应用模式-(配置)文件目录结构(默认)
- 多应用模式(配置)文件目录结构(配置文件)
- 配置文件
- 应用配置:app.php
- 缓存配置: cache.php
- 数据库配置:database.php
- 路由和URL配置:route.php
- Cookie配置:cookie.php
- Session配置:session.php
- 命令行配置:console.php
- 多语言配置:lang.php
- 日志配置:log.php
- 页面Trace配置:trace.php
- 磁盘配置: filesystem.php
- 中间件配置:middleware.php
- 视图配置:view.php
- 改成用yaconf配置
- 事件
- 例子:省略事件类的demo
- 例子2:完整事件类
- 例子3:事件订阅,监听多个事件
- 解析
- 路由
- 路由定义
- 路由地址
- 变量规则
- MISS路由
- URL生成
- 闭包支持
- 路由参数
- 路由中间件
- 路由分组
- 资源路由
- 注解路由
- 路由绑定
- 域名路由
- 路由缓存
- 跨域路由
- 控制器
- 控制器定义
- 空控制器、空操作
- 空模块处理
- RESTFul资源控制器
- 控制器中间件
- 请求对象Request(url参数)
- 请求信息
- 获取输入变量($_POST、$_GET等)
- 请求类型的获取与伪装
- HTTP头信息
- 伪静态
- 参数绑定
- 请求缓存
- 响应对象Response
- 响应输出
- 响应参数
- 重定向
- 文件下载
- 错误页面的处理办法
- 应用公共文件common.php
- 模型
- 模型定义及常规属性
- 模型数据获取与模型赋值
- 查询
- 数据集
- 增加
- 修改
- 删除
- 条件
- 查询范围scope
- 获取器
- 修改器
- 搜索器
- 软删除
- 模型事件
- 关联预载入
- 模型关联
- 一对一关联
- 一对多关联
- 多对多关联
- 自动时间戳
- 事务
- 数据库
- 查询构造器
- 查询合集
- 子查询
- 聚合查询
- 时间查询
- 视图查询(比join简单)
- 获取查询参数
- 快捷方法
- 动态查询
- 条件查询
- 打印sql语句
- 增
- 删
- 改
- 查
- 链式操作
- 查询表达式
- 分页查询
- 原生查询
- JSON字段
- 链接数据库配置
- 分布式数据库
- 查询事件
- Db获取器
- 事务操作
- 存储过程
- Db数据集
- 数据库驱动
- 视图
- 模板
- 模板配置
- 模板位置
- 模板渲染
- 模板变量与赋值(assign)
- 模板输出替换
- url生成
- 模板详解
- 内置标签
- 三元运算
- 变量输出
- 函数输出
- Request请求参数
- 模板注释及原样输出
- 模板继承
- 模板布局
- 原生PHP
- 模板引擎
- 视图过滤
- 视图驱动
- 验证
- 验证进阶之最终版
- 错误和日志
- 异常处理
- 日志处理
- 调试
- 调试模式
- Trace调试
- SQL调试
- 变量调试
- 远程调试
- 杂项
- 缓存
- Session
- Cookie
- 多语言
- 上传
- 扩展说明
- N+1查询
- TP类库
- 扩展类库
- 数据库迁移工具
- Workerman
- think助手工具库
- 验证码
- Swoole
- request
- app
- Response
- View
- Validate
- Config
- 命令行
- 助手函数
- 升级指导(功能的添加与删除说明)
- siyucms
- 开始
- 添加页面流程
- 列表页加载流程
- 弹出框
- 基础控制器
- 基础模型
- 快速构建
- 表单form构建
- 表格table构建
- MakeBuilder
- 前端组件
- 日期组件
- layer 弹层组件
- Moment.js 日期处理插件
- siyucms模板布局
- 函数即其变量
- 前端页面
- $.operate.方法
- $.modal.方法:弹出层
- $.common.方法:通用方法
- 被cms重写的表格options
- 自定义模板
- 搜索框
- 自定义form表单
- 获取表单搜索参数并组装为url字符串