[TOC]
## 线性渐变
语法:
```js
let gnt = cxt.createLinearGradient(x1, y1, x2, y2)
gnt.addColorStop(value1, color1)
gnt.addColorStop(value2, color2)
cxt.fillStyle = gnt
cxt.fill()
```
说明:在 Canvas 中,实现线性渐变有以下三个步骤
(1) 调用 createLinearGradient() 方法创建一个 linearGradient 对象,并赋值给变量 gnt
(2) 调用 linearGradient 对象的 addColorStop() 方法 N 次,第 1 次表示渐变开始的颜色,第 2 次表示渐变结束时的颜色。然后第 3 次则以第 2 次渐变颜色作为开始颜色,进行渐变,依此类推。
(3) 把 linearGradient 对象赋值给 fillStyle 属性,并调用 fill() 方法来绘制有渐变色的图形。
```
var gnt = cxt.createLinearGradient(x1, y1, x2, y2)
```
x1、y1 表示渐变色开始点的坐标,x2、y2 表示渐变色结束点的坐标,其有以下关系:
- 如果 y1 与 y2 相同,表示沿水平方向从左到右渐变
- 如果 x1 与 x2 相同,表示沿着垂直方向从左到右渐变
- 如果 x1 与 x2 不相同,且 y1 与 y2 也不相同,则表示渐变色沿着矩形对角线方向渐变
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>渐变</title>
</head>
<body>
<canvas id="canvas" style="margin:0 auto; border: 1px solid gray"></canvas>
<script>
window.onload = function () {
let canvas = document.getElementById('canvas')
canvas.width = 600
canvas.height = 600
let context = canvas.getContext('2d')
let linearGrad = context.createLinearGradient(0,0,400,400) // 改变坐标来改变渐变方向
linearGrad.addColorStop(0,'white') // ColorStop 可以添加无数个,相当于一个中间状态
linearGrad.addColorStop(0.25,'yellow') // 只能用小数不能用百分号...
linearGrad.addColorStop(0.5,'green')
linearGrad.addColorStop(0.75,'blue')
linearGrad.addColorStop(1,'black')
context.fillStyle = linearGrad
context.fillRect(0,0,800,800)
}
</script>
</body>
</html>
<!--
createLinearGradient(xstart,ystart,xend,yend) 渐变开始及结束的 x y 坐标 创建线性渐变
addColorStop(stop,color) 规定渐变对象中的颜色和停止位置
-->
```
下图分别是横向,纵向,对角的线型渐变的效果
  
## 阴影
| 属性 | 说明 |
| --- | --- |
| shadowOffsetX | 阴影与图形的水平距离,默认值为 0。大于 0 时向右偏移,小于 0 时向左偏移 |
| shadowOffsetY | 阴影与图形的垂直距离,默认值为 0。大于 0 时向下偏移,小于 0 时向上偏移 |
| shadowColor | 阴影的颜色,默认为黑色 |
| shadowBlur | 阴影的模糊值,默认为 0。该值越大,模糊度越强 |
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>canvas</title>
</head>
<body>
<canvas id="canvas" width="1024" height="700" style="border: 1px solid gray; display: block; margin: 0 auto"></canvas>
<script>
window.onload = function(){
let cnv = document.getElementById('canvas')
let cxt = cnv.getContext('2d')
// 定义文字
const text = 'Canvas 阴影'
cxt.font = "bold 60px 微软雅黑"
// 定义阴影
cxt.shadowOffsetX = 5
cxt.shadowOffsetY = 5
cxt.shadowColor = 'LightSkyBlue'
cxt.shadowBlur = 10
// 填充文字
cxt.fillStyle = 'HotPink'
cxt.fillText(text, 10, 90)
// 图片阴影
let image = new Image()
image.src = './image.jpg'
image.onload = function () {
cxt.shadowOffsetX = 0
cxt.shadowOffsetY = 0
cxt.shadowColor = '#FF6666'
cxt.shadowBlur = 10
cxt.fillRect(10, 150, 500, 281)
cxt.drawImage(image, 10, 150)
}
}
</script>
</body>
</html>
```
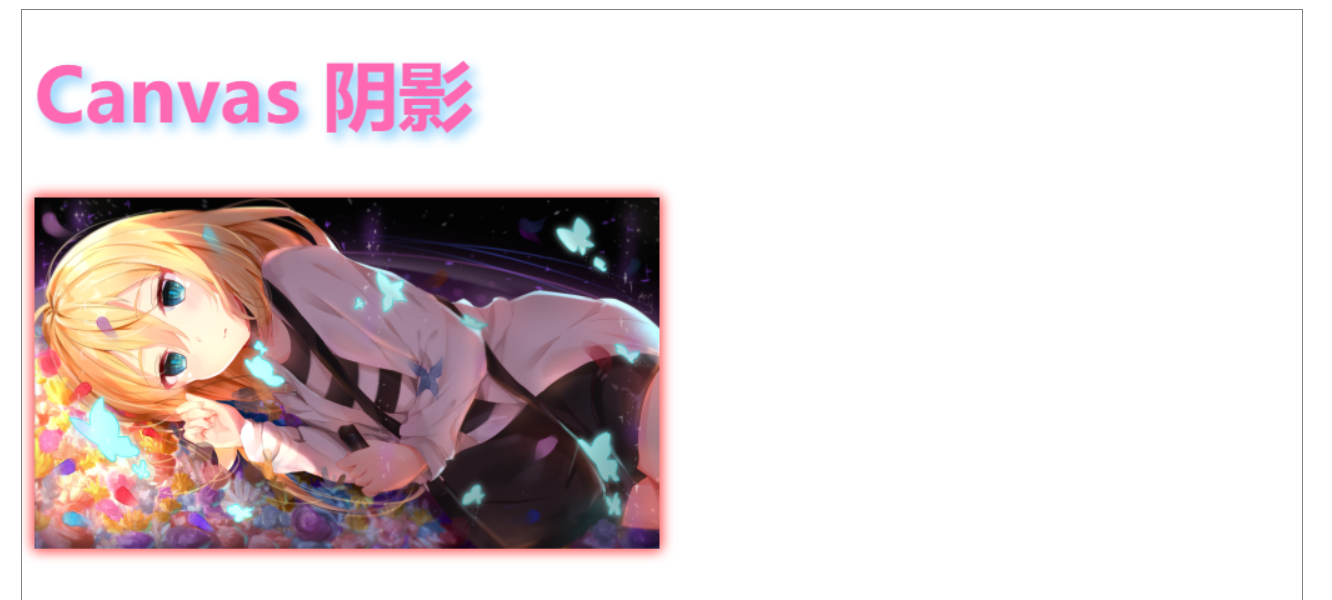
- 序言 & 更新日志
- H5
- Canvas
- 序言
- Part1-直线、矩形、多边形
- Part2-曲线图形
- Part3-线条操作
- Part4-文本操作
- Part5-图像操作
- Part6-变形操作
- Part7-像素操作
- Part8-渐变与阴影
- Part9-路径与状态
- Part10-物理动画
- Part11-边界检测
- Part12-碰撞检测
- Part13-用户交互
- Part14-高级动画
- CSS
- SCSS
- codePen
- 速查表
- 面试题
- 《CSS Secrets》
- SVG
- 移动端适配
- 滤镜(filter)的使用
- JS
- 基础概念
- 作用域、作用域链、闭包
- this
- 原型与继承
- 数组、字符串、Map、Set方法整理
- 垃圾回收机制
- DOM
- BOM
- 事件循环
- 严格模式
- 正则表达式
- ES6部分
- 设计模式
- AJAX
- 模块化
- 读冴羽博客笔记
- 第一部分总结-深入JS系列
- 第二部分总结-专题系列
- 第三部分总结-ES6系列
- 网络请求中的数据类型
- 事件
- 表单
- 函数式编程
- Tips
- JS-Coding
- Framework
- Vue
- 书写规范
- 基础
- vue-router & vuex
- 深入浅出 Vue
- 响应式原理及其他
- new Vue 发生了什么
- 组件化
- 编译流程
- Vue Router
- Vuex
- 前端路由的简单实现
- React
- 基础
- 书写规范
- Redux & react-router
- immutable.js
- CSS 管理
- React 16新特性-Fiber 与 Hook
- 《深入浅出React和Redux》笔记
- 前半部分
- 后半部分
- react-transition-group
- Vue 与 React 的对比
- 工程化与架构
- Hybird
- React Native
- 新手上路
- 内置组件
- 常用插件
- 问题记录
- Echarts
- 基础
- Electron
- 序言
- 配置 Electron 开发环境 & 基础概念
- React + TypeScript 仿 Antd
- TypeScript 基础
- 样式设计
- 组件测试
- 图标解决方案
- Algorithm
- 排序算法及常见问题
- 剑指 offer
- 动态规划
- DataStruct
- 概述
- 树
- 链表
- Network
- Performance
- Webpack
- PWA
- Browser
- Safety
- 微信小程序
- mpvue 课程实战记录
- 服务器
- 操作系统基础知识
- Linux
- Nginx
- redis
- node.js
- 基础及原生模块
- express框架
- node.js操作数据库
- 《深入浅出 node.js》笔记
- 前半部分
- 后半部分
- 数据库
- SQL
- 面试题收集
- 智力题
- 面试题精选1
- 面试题精选2
- 问答篇
- Other
- markdown 书写
- Git
- LaTex 常用命令