## 一、概述
Netty 是一个利用 Java 基于NIO(Nonblocking I/O,非阻塞IO,相对的,阻塞IO即BIO)的高级网络的能力,隐藏其背后的复杂性而提供一个易于使用的 API 的客户端/服务器框架;
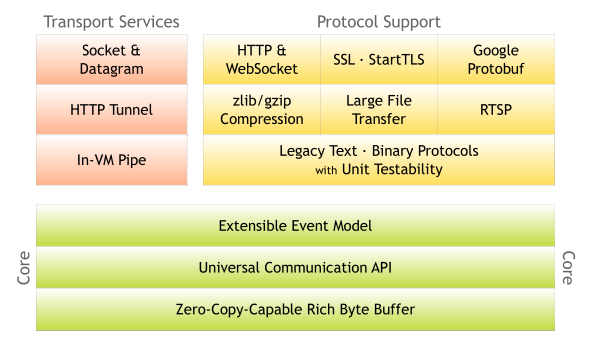
比起直接用NIO编程,用Netty要简化很多;
1. **并发高**
2. **传输快**
3. **封装好**
## 二、核心概念
### **Channel**
数据传输流,与channel相关的概念有以下四个,上一张图让你了解netty里面的Channel;
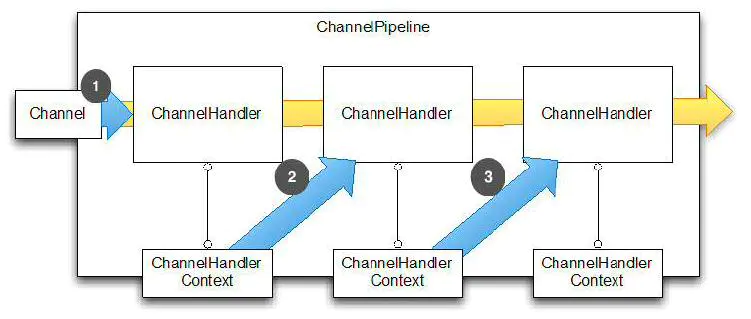
* **Channel**,表示一个连接,可以理解为每一个请求,就是一个Channel;
* **ChannelHandler**,核心处理业务就在这里,用于处理业务请求,分为ChannelInboundHandler输入数据处理器和ChannelOutboundHandler输出业务处理器;
* **ChannelHandlerContext**,用于传输业务数据;
* **ChannelPipeline**,用于保存处理过程需要用到的ChannelHandler和ChannelHandlerContext;
>[danger] 交互流程;
> 1. 事件传递给 ChannelPipeline 的第一个 ChannelHandler
> 2. ChannelHandler 通过关联的 ChannelHandlerContext 传递事件给 ChannelPipeline 中的 下一个;
> 3. ChannelHandler 通过关联的 ChannelHandlerContext 传递事件给 ChannelPipeline 中的 下一个,一直到最后一个处理完;
### **ByteBuf**
ByteBuf是一个存储字节的容器,最大特点就是**使用方便**,它既有自己的读索引和写索引,方便你对整段字节缓存进行读写,也支持get/set,方便你对其中每一个字节进行读写;
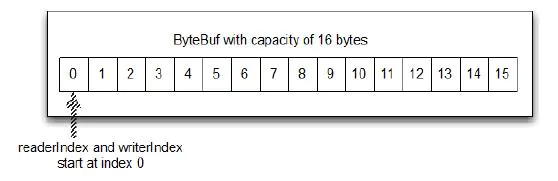
### **Codec**
包括Decoder和Encoder;
Netty中的编码/解码器,通过他你能完成字节与pojo、pojo与pojo的相互转换,从而达到自定义协议的目的,在Netty里面最有名的就是HttpRequestDecoder和HttpResponseEncoder了;
它们,实际上就是对应这**ChannelInboundHandler和ChannelOutboundHandler**,别用于在数据流进来的时候将字节码转换为消息对象和数据流出去的时候将消息对象转换为字节码;
## 三、快速入门
用一个简单的HttpServer实现例子作为示范;
只需要两个类,其中启动类负责启动(BootStrap)和main方法,另外是ChannelHandler,负责具体的业务逻辑;
```
public class NettySampleServer
{
private static Logger logger = LoggerFactory.getLogger(NettySampleServer.class);
public static void main(String[] args) throws Exception
{
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try
{
ServerBootstrap bootstrap = new ServerBootstrap();
bootstrap.group(bossGroup, workerGroup).channel(NioServerSocketChannel.class).option(ChannelOption.SO_BACKLOG, 128).childOption(ChannelOption.SO_KEEPALIVE, true).childHandler(new ChannelInitializer<SocketChannel>()
{
@Override
protected void initChannel(SocketChannel socketChannel) throws Exception
{
socketChannel.pipeline().addLast(new NettySampleServerHandler());
}
});
logger.info("server is ready......");
ChannelFuture channelFuture = bootstrap.bind(6666).sync();
channelFuture.channel().closeFuture().sync();
}
finally
{
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
}
```
```
public class NettySampleServerHandler extends ChannelInboundHandlerAdapter
{
private static Logger logger = LoggerFactory.getLogger(NettySampleServerHandler.class);
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception
{
ByteBuf byteBuf = (ByteBuf) msg;
logger.info("收到[{}]消息[{}]", ctx.channel().remoteAddress(), byteBuf.toString(CharsetUtil.UTF_8));
}
@Override
public void channelReadComplete(ChannelHandlerContext ctx) throws Exception
{
ctx.writeAndFlush(Unpooled.copiedBuffer("已收到", CharsetUtil.UTF_8));
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception
{
ctx.close();
}
}
```
- 引言
- 01、开发工具
- Maven
- 术语
- 仓库
- Archetype
- 安装配置
- 典型配置
- 内置变量
- eclipse插件
- 本地包安装
- 依赖库更新
- 依赖库排错
- 常见问题
- Gradle
- build.gradle
- gradle插件
- eclipse插件
- Eclipse
- json生成bean
- 常见问题
- IDEA Community
- 工程管理
- maven操作
- 格式化
- 常见问题
- Git
- GitHub
- 快速开始
- 既有工程
- 新建工程
- 日常提交
- PR操作
- 多人协作
- 常用命令
- 常见问题
- 同步代码
- 发布库包
- CodeGenerator
- VSCode
- 安装
- 配置
- 快速开始
- 与GitHub整合
- 断点调试
- 便捷开发
- 扩展
- prettier+
- Vetur
- 前端调试
- F12调试工具
- Vue前端调试
- 测试工具
- 压力测试
- 接口测试
- 抓包工具
- 导入证书
- SecureCRT
- 02、前端技术
- 前端设计
- javascript
- 基本语法
- 数据类型
- 类型转换
- 错误处理
- console对象
- 标准库
- 异步操作
- ES6及后续增强
- 模块化
- 扩展运算符
- 解构变量
- 箭头函数
- 混入模式
- web标准
- css
- html
- HistoryApi
- dom
- 如何理解
- 虚拟dom
- JSON
- svg
- WebAssembly
- web components
- HtmlComponents
- Custom Elements
- 标准扩展
- javascript
- Babel
- TypeScript
- JavaScript
- ECMAScript
- 模块化
- CommonJS
- require
- exports与module.exports
- ES6模块
- export
- import
- AMD
- define
- require
- CMD
- define
- require
- Web Storage
- JSX
- ES6语法
- 语法糖
- ==和===
- let与const
- call&apply
- 内置对象
- Object
- Class
- Promise对象
- then
- catch
- finally
- resolve
- reject
- Module
- Generator函数
- arguments
- 函数扩展
- 数组
- 对象
- Set和Map
- Proxy对象
- css
- sass
- less
- postcss
- CSS Modules
- Node.js
- 安装
- npm
- ls
- init
- install
- run
- uninstall
- update
- version
- npm生态
- yarn
- package.json
- node_modules
- 常用技术
- 应用实例
- Web框架
- Express
- Egg.js
- Mock
- Mock.js
- 语法规范
- 非核心api
- 核心api
- easymock
- 开发测试
- ESLint
- jest
- Travis
- Prettier
- stylelint
- 构建工具
- gulp
- Browserify
- webpack
- 安装配置
- 入口起点entry
- 输出output
- 装载器loader
- 插件plugins
- webpack-cli
- public目录
- 技术概念
- CSR与SSR
- polyfill
- axios
- 请求对象
- 响应对象
- 自定义实例
- 拦截器
- 跨域访问
- 03、前端框架
- mvvm
- vue.js
- 简明指南
- vue文件结构
- 组件指南
- 组件命名
- 应用流程
- 单文件组件
- 组件导入导出
- 生命周期
- Prop
- 复用方法
- 懒加载
- 全局环境
- 全局配置
- 全局API
- 选项对象
- 混入选项
- vue实例$
- vue指令
- v-bind(:)
- v-on(@)
- v-model
- 特殊属性
- 内置组件
- 自定义机制
- 组件
- 指令
- 过滤器
- 混入
- slot插槽
- 渲染函数
- 注意事项
- 总结
- vueCli
- 安装
- 组成部分
- vue.config.js
- vue核心文件
- 状态管理
- 简单状态
- Vuex
- 构造器选项
- 实例属性
- 实例方法
- 绑定辅助函数
- 模块化
- 总结
- 路由管理
- 简单路由
- Vue Router
- 路由模式
- route
- router
- <router-link>与编程式
- <router-view>
- 嵌套路由
- 导航守卫
- 总结
- vue插件
- Vue Loader
- 实战举例
- vue快速入门
- vue与后台联动
- vue完整实例
- vue组件库
- vue-ls
- Enquire.js
- lodash
- md5.js
- moment
- nprogress
- viser-vue
- vue-clipboard2
- vue-cropper
- vue-quill-editor
- wangeditor
- vue-svg-icon-loader
- 实战参考
- Vue Antd Admin
- ant-design-vue
- 快速开始
- 要点解析
- vuepress
- vant
- 04、后端框架
- SprigBoot
- 快速入门
- 完整示例
- 完整进阶
- 核心技术
- 核心标记
- 页面技术
- Thymeleaf
- 数据访问
- 基本用法
- 事务控制
- 事务规则
- 注意事项
- 实体状态
- 数据查询
- 普通查询
- 分页查询
- 统计查询
- 命名访问
- 公用共享
- 缓存机制
- 服务层
- 控制器
- AOP
- 定时任务
- 异步任务
- 静态注入
- WebClient
- 启动机制
- 应用监控
- 线程安全
- 调试测试
- 打包部署
- 打jar包
- 常见问题
- 配置问题
- 开发问题
- 文档生成
- 相关技术
- springfox
- knife4j
- actuator
- kaptcha
- YAML
- API Blueprint
- 启用https
- SpringSecurity
- 快速入门
- 核心元素
- jwt
- 与springsecurity集成
- 05、运行容器
- artemis
- 协议支持
- mqtt
- 安装运行
- 管理配置
- 日志配置
- 业务配置
- 安全配置
- 数据存储
- SSL支持
- 运行维护
- mosquitto
- 安装运行
- 管理配置
- SSL支持
- rocketmq
- 安装运行
- 控制台
- 代码实例
- kafka
- ZooKeeper
- 安装运行
- 代码实例
- zookeeper
- 安装运行
- 应用实例
- dubbo
- 代码实例
- hadoop
- 安装配置
- 快速运行
- netty
- 06、相关技术
- Serverless
- Protobuf
- SSL
- 证书
- 认证类型
- 硬件技术
- 基础知识
- 开发技术
- 消息协议
- 07、项目实战
- 前端开发
- 从零开始开发
- 开发环境搭建
- 原生技术开发
- 路由守卫
- 动态路由菜单
- 全局API
- 登录认证
- 与后端交互
- 代码开发调试
- 快速打包发布
- 常见问题收集
- 后端开发
- 从零开始开发
- 开发环境搭建
- 常用注解说明
- 常用基础设施
- 核心业务约定
- 平台配置文件
- 业务配置清单
- 关键配置参数
- 项目必配参数
- 项目调优参数
- 返回结果处理
- 字段翻译机制
- 列表字段翻译
- 实体字段翻译
- 组合字段翻译
- 列表数据增强
- 列表数据简化
- 返回字段过滤
- 返回字段改名
- 定制返回结果
- 原生技术开发
- 动态级联字典
- 简单数据查询
- 短信验证业务
- 测试数据模拟
- 开放平台登陆
- 微信开放平台
- 抖音开放平台
- 文件处理方案
- 文件字段存储
- 文件字段解析
- 图像数据存取
- 文件资源方案
- 服务集成开发
- redis服务集成
- mqtt服务集成
- kafka集成
- rocketmq集成
- websocket集成
- elasticsearch集成
- netty集成
- 外部工具开发
- 发送短信服务
- 发送邮件服务
- 动态pdf生成
- 数据处理开发
- 同步导出数据
- 异步导出数据
- 同步导入数据
- 异步导入数据
- 多线程与并发
- 线程并发安全
- 操作间隔控制
- 异步待办机制
- 平台定时任务
- 平台异步任务
- 常见注意事项
- 安全相关开发
- 接口安全策略
- 接口限流策略
- 接口授权策略
- 权限相关开发
- 路由权限方案
- 组织权限方案
- 数据权限方案
- 字段权限方案
- 按钮权限方案
- 支付相关开发
- 微信原生支付
- 微信H5支付
- 微信JSAPI支付
- 微信批量转账
- 微信动态支付
- 支付宝移动网站支付
- 支付宝PC网站支付
- 平台缓存机制
- 内置进程内缓存
- 内置分布式缓存
- 平台自定义缓存
- 平台插件机制
- 账号的邀请码
- 账号的二维码
- 定制事件机制
- 约定实现机制
- 请求回调机制
- 启动自动加载
- 平台基础设施
- 动态参数加载
- 定制待定常量
- 定制单位组织
- 平台缓存机制
- 平台外访机制
- 静态资源获取
- 调试打印机制
- 数据源随时用
- 上下文随处拿
- 平台诊断机制
- 平台内置资源
- 强制间隔时间
- 账号扩展开发
- 账号变更事件
- 业务开发指南
- 字典数据获取
- 数据层持久化
- 基础服务调用
- 查询时间范围
- 代码开发调试
- 常见问题收集
- 从零开始
- PCV1运行
- PCV2运行
- H5端运行
- 开发进阶
- 最佳实践
- 开发方案
- 前后分离
- 跨域访问
- 库表设计
- 模型设计
- 容器部署
- 集群部署
- 日志收集
- 动态配置
- 开发管理
- 开发环境
- 代码控制
- 问题跟踪
- 进度跟踪
- 测试环境
- 调试辅助
- DevOps
- 代码风格
- 运行维护
- 基本监控知识
- 线程堆栈分析
- 内存堆栈分析
- 应用诊断工具
- 工程示范
- 后端开发
- 前端开发
- PC端
- 移动端
- 08、内置容器
- 调度服务
- 调度容器
- 快速开发
- 线程并发
- 多点部署
- 本地调试
- 常见问题
- 开放服务
- 快速接入
- 接口开发
- 09、开放平台
- 微信公号
- 环境准备
- 环境配置
- 技术方案
- 获取OpenId
- 常见问题
- 10、平台功能
- 系统管理
- 单位组织
- 角色管理
- 账号管理
- 子账号
- 财务账户
- 开放数据
- 绑定数据
- 套餐权益
- 会员定义
- 变更审核
- 注册审核
- 系统配置
- 路由配置
- 参数配置
- 属性配置
- 树形设置
- 服务接口
- 访问设置
- 系统监控
- 在线用户
- 内存数据
- 系统变量
- 外访数据
- 到访数据
- 操作记录
- 静态字典
- 日志管理
- 元数据
- 接入管理
- 微信公号
- 微信支付
- 开放服务
- 客户端
- 服务列表
- 请求历史
- 请求服务
- 调度服务
- 调度监控
- 11、补充语言
- php
- 生产环境
- 安装
- 初始配置
- nginx集成
- 配置文件
- 语法
- 变量和常量
- 数据类型
- 条件控制
- 运算符
- 数组
- 指针
- 循环控制
- 函数
- 语法糖
- 预定义变量
- session和cookie
- 命名空间
- 面向对象
- 数据库操作
- 表单
- 错误
- 异常
- 过滤器
- JSON
- XML
- AJAX
- Composer
- 开发环境
- 本地调试
- 远程调试
- .net
- 开发环境
- C#快速入门
- 12、依赖容器
- elasticsearch
- 运行配置
- 命令操作
- 中文分词
- Kibana
- Logstash
- 开发技术
- 搜索类型
- 代码示例
- 应用场景
- 常见问题
- nginx
- 下载安装
- 基本配置
- 服务启停
- 安全防护
- 常见问题
- linux
- 常用操作
- 常用命令
- 用户管理
- ftp服务
- 防火墙
- 运维
- 网络安全
- 内核参数
- 安装
- yum源问题
- mysql
- 安装配置
- 快速安装
- 正式安装
- 参数配置
- 性能优化
- 语句优化
- 配置优化
- 设计优化
- 运维常识
- 系统监控
- 连接数
- 超时
- cpu利用率
- 数据备份
- 导入复制
- 经验举例
- 故障处理
- 用户管理
- 系统日志
- 日志清理
- 安全经验
- 集群方案
- MySQL Replication
- MySQL Cluster
- 常见问题
- redis
- 安装配置
- 安装运行
- 参数配置
- 运维常识
- 技术要点
- pubSub
- 操作命令
- 持久化
- 常见问题
- docker
- 安装运行
- 镜像操作
- 容器操作
- 仓库操作
- 实战案例
- kubernetes
- 后记