继续以文章分类为例,我们在/application/cms/controller/admin/目录下创建Cate.php文件,注意这里我们就会用到很重要的一个特性:动态页面,我们不需要去写.vue,只要在这里编写PHP代码即可自动解析生页面,内容如下:
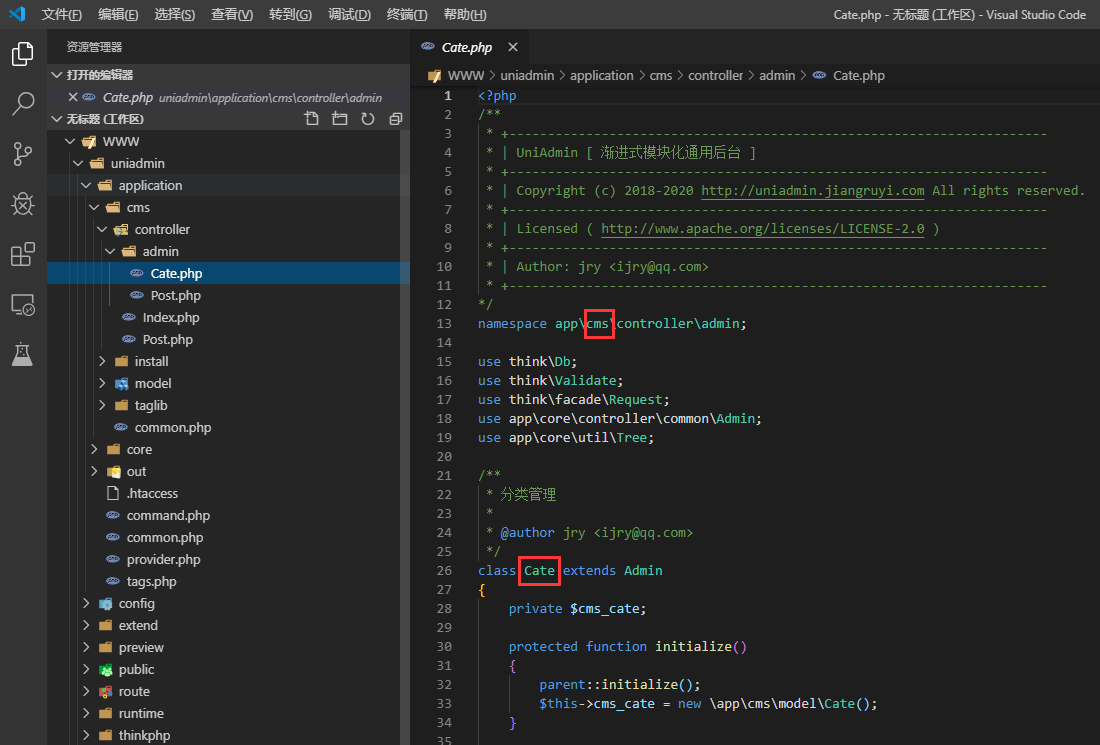
```
<?php
/**
* +----------------------------------------------------------------------
* | UniAdmin [ 渐进式模块化通用后台 ]
* +----------------------------------------------------------------------
* | Copyright (c) 2018-2020 http://uniadmin.jiangruyi.com All rights reserved.
* +----------------------------------------------------------------------
* | Licensed ( http://www.apache.org/licenses/LICENSE-2.0 )
* +----------------------------------------------------------------------
* | Author: jry <ijry@qq.com>
* +----------------------------------------------------------------------
*/
namespace app\cms\controller\admin;
use think\Db;
use think\Validate;
use think\facade\Request;
use app\core\controller\common\Admin;
use app\core\util\Tree;
/**
* 文章分类
*
* @author jry <ijry@qq.com>
*/
class Cate extends Admin
{
private $example_cate;
protected function initialize()
{
parent::initialize();
$this->cms_cate = new \app\cms\model\Cate();
}
/**
* 分类列表
*
* @return \think\Response
* @author jry <ijry@qq.com>
*/
public function lists()
{
//分类列表
$data_list = $this->example_cate->select()->toArray();
$tree = new Tree();
$data_list = $tree->list2tree($data_list);
//构造动态页面数据
$ibuilder_list = new \app\core\util\xybuilder\XyBuilderList();
$list_data = $ibuilder_list->init()
->addTopButton('add', '添加分类', ['api' => '/v1/admin/example/cate/add'])
->addRightButton('edit', '修改', ['api' => '/v1/admin//example/cate/edit', 'title' => '修改分类信息'])
->addRightButton('delete', '删除', [
'api' => '/v1/admin//example/cate/delete',
'title' => '确认要删除该分类吗?',
'modal_type' => 'confirm',
'width' => '600',
'okText' => '确认删除',
'cancelText' => '取消操作',
'content' => '<p>删除后前台将无法访问</p>',
])
->addColumn('id' , 'ID', ['width' => '50px'])
->addColumn('nickname', '昵称', ['width' => '120px'])
->addColumn('username', '用户名', ['width' => '120px'])
->addColumn('mobile', '手机号', ['width' => '120px'])
->addColumn('email', '邮箱', ['width' => '120px'])
->addColumn('sortnum', '排序', ['width' => '50px'])
->addColumn('rightButtonList', '操作', [
'minWidth' => '50px',
'type' => 'template',
'template' => 'right_button_list'
])
->getData();
//返回数据
return json([
'code' => 200, 'msg' => '成功', 'data' => [
'dataList' => $data_list,
'listData' => $list_data
]
]);
}
/**
* 添加
*
* @return \think\Response
* @author jry <ijry@qq.com>
*/
public function add()
{
if (request()->isPost()) {
//数据验证
$validate = Validate::make([
'title' => 'require'
],
[
'title.require' => '分类标题必须'
]);
$data = input('post.');
if (!$validate->check($data)) {
return json(['code' => 200, 'msg' => $validate->getError(), 'data' => []]);
}
//数据构造
$data_db = $data;
if (count($data_db) <= 0 ) {
return json(['code' => 0, 'msg' => '无数据提交', 'data' => []]);
}
$data_db['status'] = 1;
$data_db['create_time'] = time();
//存储数据
$ret = $this->example_cate->save($data_db);
if ($ret) {
return json(['code' => 200, 'msg' => '添加成功', 'data' => []]);
} else {
return json(['code' => 0, 'msg' => '添加失败:' . $this->core_user->getError(), 'data' => []]);
}
} else {
//构造动态页面数据
$ibuilder_form = new \app\core\util\xybuilder\XybuilderForm();
$form_data = $ibuilder_form->init()
->setFormMethod('post')
->addFormItem('title', '标题', 'text', '', [
'placeholder' => '请输入标题',
'tip' => '分类标题'
])
->addFormRule('title', [
['required' => true, 'message' => '请填写标题', 'trigger' => 'change'],
])
->setFormValues()
->getData();
//返回数据
return json([
'code' => 200,
'msg' => '成功',
'data' => [
'formData' => $form_data
]
]);
}
}
/**
* 修改
*
* @return \think\Response
* @author jry <ijry@qq.com>
*/
public function edit($id)
{
if (request()->isPut()) {
//数据验证
$validate = Validate::make([
'title' => 'require'
],
[
'title.require' => '标题必须'
]);
$data = input('post.');
if (!$validate->check($data)) {
return json(['code' => 200, 'msg' => $validate->getError(), 'data' => []]);
}
//数据构造
$data_db = $data;
if (count($data_db) <= 0 ) {
return json(['code' => 0, 'msg' => '无数据提交', 'data' => []]);
}
//存储数据
$ret = $this->example_cate->update($data_db, ['id' => $id]);
if ($ret) {
return json(['code' => 200, 'msg' => '修改信息成功', 'data' => []]);
} else {
return json(['code' => 0, 'msg' => '修改信息失败:' . $this->core_user->getError(), 'data' => []]);
}
} else {
//用户信息
$info = $this->core_user
->where('id', $id)
->find();
//构造动态页面数据
$ibuilder_form = new \app\core\util\xybuilder\XyBuilderForm();
$form_data = $ibuilder_form->init()
->setFormMethod('put')
->addFormItem('title', '标题', 'text', $info['title'], [
'placeholder' => '请输入标题',
'tip' => '分类标题'
])
->addFormRule('title', [
['required' => true, 'message' => '请填写标题', 'trigger' => 'change'],
])
->setFormValues()
->getData();
//返回数据
return json([
'code' => 200,
'msg' => '成功',
'data' => [
'formData' => $form_data
]
]);
}
}
/**
* 删除
*
* @return \think\Response
* @author jry <ijry@qq.com>
*/
public function delete($id)
{
//删除
$ret = $this->example_cate
->where(['id' => $id])
->find()
->delete();
if ($ret) {
return json(['code' => 200, 'msg' => '删除成功', 'data' => []]);
} else {
return json(['code' => 0, 'msg' => '删除错误:' . $this->core_user->getError(), 'data' => []]);
}
}
}
```
- 说明
- 简介
- 系统安装
- 后端注意
- 目录结构
- 数据表
- 用户注册
- 前端注意
- 后端接口开发
- 新建模块
- 创建数据表
- 创建模型
- 创建后台控制器
- 添加后台接口
- 创建前台控制器
- 添加前台接口
- 常用接口
- 检查用户登录
- 内置接口
- Builder动态页面
- Builder列表
- addTopButton
- addRightButton
- addColumn
- setDataList
- setDataPage
- getData
- Builder表单
- setFormMethod
- addFormItem
- 单图image
- 多图images
- addFormRule
- setFormValues
- getData
- 自定义组件
- 自定义页面组件
- 自定义Form组件
- 加载第三方js插件
- 常见问题
- 模块开发者
- 升级指南
- 图标
- 扩展
- Composer
- ThinkPHP5.1
- GuzzleHttp
- phpspreadsheet
- QueryList
- phpseclib
- 云后台接口