[TOC]
## **Command**
### **属性**
| 属性名 | 描述 |参数类型|默认值|权限|
| --- | --- | --- | --- | --- |
| $input | | Input | void | protected |
| $output | | Output | void | protected |
### **方法**
| 方法名 | 描述 |参数说明|返回值|权限|
| --- | --- | --- | --- | --- |
| __construct($name = null) | 构造方法 | string\|null $name 命令名称,如果没有设置则比如在 configure() 里设置 |void| public |
| ignoreValidationErrors() | 忽略验证错误 |void| void | public |
| setConsole(Console $console = null) | 设置控制台 |Console| void | public |
| getConsole() | 获取控制台 | void | Console |public|
| isEnabled() | 是否有效 | void |直接返回true| public |
| **configure**() | 配置指令,留给子类进行自定义命令参数配置 | void | void | protected |
| **execute**(Input $input, Output $output) | 执行指令,留给子类进行自定义命令执行程序的接口 | Input,Output | null\|int | protected |
| run(Input $input, Output $output) | 执行 | Input,Output | int | public |
| setCode(callable $code) | 设置执行代码 | callable(InputInterface $input, OutputInterface $output) | Command | public |
| mergeConsoleDefinition($mergeArgs = true) | 合并参数定义 | 合并的参数 | void | public |
| setDefinition($definition) | 设置参数定义 | array\|Definition $definition | Command |public |
| getDefinition() | 获取参数定义 | void | Definition | public |
| getNativeDefinition() | 获取当前指令的参数定义 | void | Definition | public |
| **addArgument**($name, $mode = null, $description = '', $default = null) | 添加参数 | string $name 名称<br>int $mode 类型<br>string $description 描述<br>mixed $default 默认值 | Command | public |
| **addOption**($name, $shortcut = null, $mode = null, $description = '', $default = null) | 添加选项 添加后 使用时跟在参数后面,前面加-- | string $name 选项名称<br>string $shortcut 别名<br>int $mode 类型<br>string $description 描述<br>mixed $default 默认值 | Command | public |
| **setName**($name) | 设置指令名称 | string $name | Command | public |
| getName() | 获取指令名称 | void | String 指令名称 |public |
| **setDescription**($description) | 设置描述 | string $description | Command |public |
| getDescription() | 获取描述 | void | string 描述 |public |
| setHelp($help) | 设置帮助信息 | string $help | Command |public |
| getHelp() | 获取帮助信息 | void | string 设置的帮助信息 |public |
| getProcessedHelp() | 获取描述信息 | void | string 描述信息 |public |
| setAliases($aliases) | 设置别名 | string[] $aliases | Command |public |
| getAliases() | 获取别名 | void | array |public |
| getSynopsis($short = false) | 获取简介 | bool $short 是否简单的 | string 简介 |public |
| addUsage($usage) | 添加用法介绍 | string $usage | Command |public |
| getUsages() | 获取用法介绍 | void | array |public |
### **示例:**
~~~php
<?php
namespace app\common\command;
use think\Console;
use think\console\{Command,Output,Input};
use think\console\Input\{Argument,Option};
/*
* 带参数脚本兼容linux定时和手动执行
*/
class Test extends Command
{
//定义任务名和描述
protected function configure(){
//设置参数
$this->addArgument('id',Argument::REQUIRED,'ID必填'); //必传参数
$this->addArgument('name', Argument::OPTIONAL,'NAME选填');//可选参数
//选项定义
$this->addOption('type', 't', Option::VALUE_REQUIRED,'TYPE必填',0); //选项值必填
$this->addOption('status', 's', Option::VALUE_OPTIONAL,'STATUS选填',1); //选项值选填
$this->setName('test')->setDescription("php think test 测试"); //选项定义
}
//调用该类时,会自动运行execute方法
protected function execute(Input $input, Output $output){
set_time_limit(0);
@ob_end_clean();
@ob_implicit_flush(1);
ini_set('memory_limit','100M');
# echo substr_replace('18858281234','****',3,4);
header("Content-type: text/html; charset=utf-8");
date_default_timezone_set('Asia/Shanghai');
$excel_id = Cache::get('excel_id');
if(!empty($excel_id)){ //如果缓存有值,用定时脚本执行
$id = $excel_id;
}else{//如果使用命令行手动传参执行
$args = $input->getArguments();//获取传参数组
print_r($args);
$options = $input->getOptions(); //获取选项数组
print_r($options);
$output->writeln("参数接收为:".$args['id'].'--'.$args['name']);
//接收用全称name,不能简写shortcut
$output->writeln("选项接收为:".$options['type'].'--'.$options['status']);
}
$page = 0;
while(true){
$page++;
$res = $model->where(['id'=>$id])->paginate(100, false, [ 'page' => $page, 'query' => $query ]); //获取订单数据
$res = json_decode($res,true);
if( empty($res['data']['body'])){//分页获取数据为空,则说明执行完毕
exit("已同步完成\n\r");//退出
}
//数据不为空,循环遍历插入本地数据库
foreach($res['data']['body'] as $k=>$v){
$data['product_id'] = $v['product_id'];
$data['order_status'] = $status;
Db::table(Config('database.prefix').'table')->insert($data);
}
echo "同步第{$page}页完成\n\r"; //输出
}
}
}
~~~
### **调用:**
#### 控制台调用
~~~dart
# 四种调用结果相同
[root@root root]# php think test 1 admin --type "2" --status "3"
[root@root root]# php think test --type "2" --status "3" 1 admin
[root@root root]# php think test 1 admin -t"2" -s"3"
[root@root root]# php think test -t"2" -s"3" 1 admin
Array
(
[command] => test
[id] => 1
[name] => admin
)
Array
(
[type] => 2
[status] => 3
[help] =>
[version] =>
[quiet] =>
[verbose] =>
[ansi] =>
[no-ansi] =>
[no-interaction] =>
)
参数接收为:1--admin
选项接收为:2--3
[root@root root]#
~~~
#### 代码调用
~~~php
namespace app\index\controller;
use think\Console;//引入Console
class Index{
$output = Console::call('command');#无参数调用
$output = Console::call('command',[args1,args2,argsN...]);#带参数调用命令
$output = Console::call('test',['1','admin','-t2','-s3']);#带参数调用(options和arguments不区分顺序)
return $output->fetch(); #获取输出信息
}
~~~
## **Console**
### **方法**
| 属性名 | 描述 |参数说明|返回值|权限|
| --- | --- | --- | --- | --- |
| __construct($name = 'UNKNOWN', $version = 'UNKNOWN', $user = null) | | | | |
| setUser($user) | 设置执行用户 | $user | void | public |
| init($run = true) | 初始化 Console | bool $run 是否运行 Console | int|Console |public、static|
| call($command, array $parameters = [], $driver = 'buffer') | 调用命令 | string $command<br>array $parameters<br>string $driver | Output |public、static|
| run() | 执行当前的指令 | void | int |public|
| doRun(Input $input, Output $output) | 执行指令 | Input $input 输入<br>Output $output 输出 | int |public|
| setDefinition(InputDefinition $definition) | 设置输入参数定义 | InputDefinition $definition 输入定义 | Console |public|
| getDefinition() | 获取输入参数定义 | void | InputDefinition |public|
| getHelp() | 获取帮助信息 | void | string |public|
| setCatchExceptions($boolean) | 设置是否捕获异常 | bool $boolean 是否捕获 |Console|public|
| setAutoExit($boolean) | 设置是否自动退出 | bool $boolean 是否自动退出 | Console |public|
| getName() | 获取名称 | void | string |public|
| setName($name) | 设置名称 | string $name 名称 |Console|public|
| getVersion() | 获取版本 | void | string |public|
| setVersion($version) | 设置版本 | string $version 版本信息 |Console |public|
| getLongVersion() | 获取完整的版本号 | void | string |public|
| register($name) | 注册一个指令 | string $name 指令名称 | Command |public|
| addCommands(array $commands) | 批量添加指令 | Command[] $commands 指令实例 |Console|public|
| add(Command $command) | 添加一个指令 | Command $command 命令实例 | Command\|bool |public|
| get($name) | 获取指令 | string $name 指令名称 | Command |public|
| has($name) | 某个指令是否存在 | string $name 指令名称 | bool |public|
| getNamespaces() | 获取所有的命名空间 | void | array |public|
| findNamespace($namespace) | 查找注册命名空间中的名称或缩写 | string $namespace | string |public|
| find($name) | 查找指令 | string $name 名称或者别名 | Command |public|
| all($namespace = null) | 获取所有的指令 | string $namespace 命名空间 | Command[] |public|
| getAbbreviations($names) | 获取可能的指令名 | array $names 指令名 | array |public|
| configureIO(Input $input, Output $output) | 配置基于用户的参数和选项的输入和输出实例 | Input $input 输入实例<br>Output $output 输出实例 | void |public,protected|
| doRunCommand(Command $command, Input $input, Output $output) | 执行指令 | Command $command 指令实例<br>Input $input 输入实例<br>Output $output 输出实例| int |public,protected|
| getCommandName(Input $input) | 获取指令的名称 | Input $input 输入实例 | string |public,protected|
| etDefaultInputDefinition() | 获取默认输入定义 | void | InputDefinition |public,protected|
| getDefaultCommands() | 获取默认命令 | void | Command[] |public,protected|
| addDefaultCommands(array $classes) | 添加默认指令 | array $classes 指令 | void |public,static|
| extractNamespace($name, $limit = null) | 返回指令的命名空间部分 | string $name 指令名称<br>string $limit 部分的命名空间的最大数量 | string |public|
| setDefaultCommand($commandName) | 设置默认的指令 | string $commandName 指令名称 | Console |public|
## **Output**
### **常量**
| 常量名 | 描述 |参数类型|默认值|
| --- | --- | --- | --- |
| VERBOSITY_QUIET | | | 0 |
| VERBOSITY_NORMAL | | | 1 |
| VERBOSITY_VERBOSE | | | 2 |
| VERBOSITY_VERY_VERBOSE | | | 3 |
| VERBOSITY_DEBUG | | | 4 |
| OUTPUT_NORMAL | | | 0 |
| OUTPUT_RAW | | | 1 |
| OUTPUT_PLAIN | | | 2 |
### **方法**
实例化时传入的think\console\output\driver\里的类(默认Console) 存入handle属性,这里的方法差不多都是操作传入的类的方法
| 属性名 | 描述 |参数说明|返回值|权限|
| --- | --- | --- | --- | --- |
| __construct($driver = 'console') | | | |public|
| ask(Input $input, $question, $default = null, $validator = null) | | | |public|
| askHidden(Input $input, $question, $validator = null) | | | |public|
| confirm(Input $input, $question, $default = true) | | | |public|
| choice(Input $input, $question, array $choices, $default = null) | 窗口输出时需要接受手动输入值,如:$res=$output->choice($input,"请选择正确答案",[1,2,3,4]); if($res==2){$output->write("恭喜你,回答正确!!!");}<br>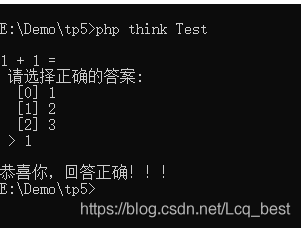 | | |public|
| askQuestion(Input $input, Question $question) | | | |public,protected|
| block($style, $message) | 调用的是进一步封装的newLine方法 | | |public,protected|
| newLine($count = 1) | 输出空行,调用的是进一步封装的write方法 | int $count | |public|
| writeln($messages, $type = self::OUTPUT_NORMAL) | 输出信息并换行,调用的是进一步封装的write方法 | string $messages<br>int $type | |public|
| write($messages, $newline = false, $type = self::OUTPUT_NORMAL) | 输出信息 | string $messages<br>bool $newline<br>int $type | |public|
| renderException(\Exception $e) | | | |public|
| setVerbosity($level) | | | |public|
| getVerbosity() | | | |public|
| isQuiet() | | | |public|
| isVerbose() | | | |public|
| isVeryVerbose() | | | |public|
| isDebug() | | | |public|
| describe($object, array $options = []) | | | |public|
| __call($method, $args) | | | |public|
- 目录结构与基础
- 修改数据后页面无变化
- 防跨目录设置
- input
- 系统目录
- 自动生成的文件以及目录
- 类自动加载
- url生成
- 数据增删改查
- 增加数据
- 数据更新
- 数据删除
- 数据查询
- 架构
- 生命周期
- 入口文件
- URL访问规则
- 配置
- 默认惯例配置配置
- 初始应用配置
- 路由
- 域名路由
- URL生成
- 数据库操作
- 方法列表
- 连接数据库
- 分布式数据库
- 查询构造器
- 查询数据
- 添加数据
- 更新数据
- 删除数据
- 查询语法
- 聚合查询(统计)
- 时间查询
- 高级查询
- 视图查询
- 子查询
- 辅助查询之链式操作
- where
- table
- alias
- field
- order
- limit
- page
- group
- having
- join
- union
- distinct
- lock
- cache
- comment
- fetchSql
- force
- bind
- partition
- strict
- failException
- sequence(pgsql专用)
- 查询事件
- 事务操作
- 监听SQL
- 存储过程
- 数据集
- 控制器
- 跳转和重定向
- 空控制器和空操作
- 分层控制器
- Rest控制器
- 资源控制器
- 自动定位控制器
- tp3的增删改查
- 方法注入
- 模型
- 属性方法一览
- 类方法详解
- Model
- 调用model不存在的属性
- 调用model中不存在的方法
- 调用model中不存在的静态方法
- hasOne
- belongsTo
- hasMany {Relation}
- belongsToMany
- hasManyThrough
- morphMany
- morphOne
- morphTo
- ::hasWhere {Query}
- ::has
- relationCount
- data 【model】
- setInc {integer|true}
- setDec {integer|true}
- save {integer | false}
- saveAll {array}
- delete {integer}
- ::get 查询单条数据 {Model}
- ::all 查询多条数据{Model [ ]}
- ::create 新增单条数据 {Model}
- ::update 更新单条数据 {Model}
- ::destroy {integer}
- ::scope {Query}
- getAttr {mixed}
- xxx
- append
- appendRelationAttr
- hidden
- visible
- except
- readonly
- auto
- together
- allowField
- isUpdate
- validate
- toCollection
- toJson
- toArray
- 定义
- 新增
- 更新
- 查询
- 删除
- 聚合
- 获取器
- 修改器
- 时间戳
- 只读字段
- 软删除
- 类型转换
- 数据完成
- 查询范围
- 模型分层
- 数组访问和转换
- JSON序列化
- 事件
- 关联
- 一对一关联
- 主表一对一关联
- 从表一对一关联(相对关联)
- 一对多关联
- 主表定义一对多关联
- 从表定义一对多关联
- 远程一对多
- 多对多关联
- 多态关联
- 动态属性
- 关联预载入with()
- 关联统计
- N+1查询
- 聚合模型
- Model方法集合
- 表单验证
- 验证器
- 验证规则
- 错误信息
- 验证场景
- 控制器验证
- 模型验证
- 内置规则
- 静态调用
- 表单令牌
- Token身份令牌
- 视图
- 模版
- 变量输出
- 函数输出
- Request请求参数
- 模板注释及原样输出
- 三元运算
- 内置标签
- 模板继承
- 模板布局
- 日志
- 日志初始化
- 日志驱动
- 日志写入
- 独立日志
- 日志清空
- 写入授权
- 自定义日志
- 错误和调试
- 异常
- php系统异常及thinkphp5异常机制
- 异常处理
- 抛出异常
- 异常封装
- resful
- 404页面
- 调试模式
- Trace调试
- SQL调试
- 变量调试
- 性能调试
- 远程调试
- 安全
- 输入安全
- 数据库安全
- 上传安全
- 其它安全建议
- xss过滤
- 扩展
- 函数
- 类库
- 行为
- 驱动
- Composer包
- Time
- 数据库迁移工具
- Workerman
- MongoDb
- htmlpurifier XSS过滤
- 新浪SAE
- oauth2.0
- 命令行及生成文件
- 系统现成命令
- 创建类库文件
- 生成类库映射文件
- 生成路由缓存
- 清除缓存文件
- 生成配置缓存文件
- 生成数据表字段缓存
- 自定义命令行
- 开始
- 调用命令
- 杂项
- 助手函数
- URL重写
- 缓存
- 缓存总结
- Session
- Cookie
- 多语言
- 分页
- 上传
- 验证码
- 图像处理
- 文件处理
- 单元测试
- 自定义表单令牌