### 快代理2
gocolly包github官网:https://github.com/gocolly/colly
gocolly官网:http://go-colly.org/
快代理:"https://www.kuaidaili.com/free
首先安装goclly这个包,老方法,去github复制它示例代理,然后去项目文件打开cmd,使用指令go get获取
示例代码:
```
package main
import (
"fmt"
"github.com/gocolly/colly"
)
func main() {
c := colly.NewCollector()
// Find and visit all links
c.OnHTML("a[href]", func(e *colly.HTMLElement) {
e.Request.Visit(e.Attr("href"))
})
c.OnRequest(func(r *colly.Request) {
fmt.Println("Visiting", r.URL)
})
c.Visit("http://go-colly.org/")
}
```
这次爬取多页
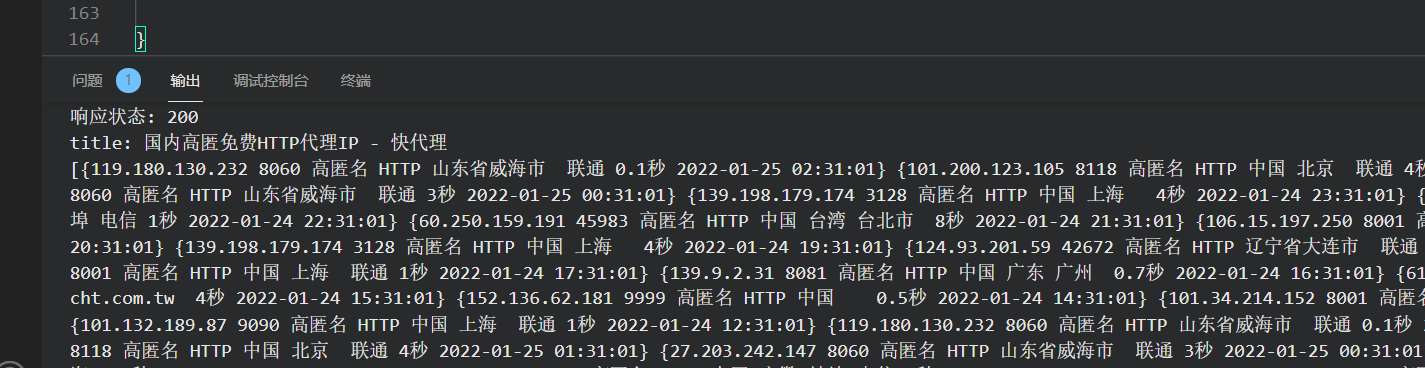
```
package main
import (
"fmt"
"strconv"
"time"
"github.com/gocolly/colly"
)
// 创建一个结构体 用于json数据的接收
type Datalist struct {
Ip string `json:"ip"` // ip
}
// 创建一个结构体 用于json数据的接收
type Datalist2 struct {
Port string `json:"port"` // port 端口
}
// 创建一个结构体 用于json数据的接收
type Datalist3 struct {
Anonymous string `json:"anonymous"` // 匿名度
}
// 创建一个结构体 用于json数据的接收
type Datalist4 struct {
Agreement string `json:"agreement"` //类型
}
// 创建一个结构体 用于json数据的接收
type Datalist5 struct {
Region string `json:"region"` //地区
}
// 创建一个结构体 用于json数据的接收
type Datalist6 struct {
Speed string `json:"speed"` //速度
}
// 创建一个结构体 用于json数据的接收
type Datalist7 struct {
Timeout string `json:"timeout"` //最后验证时间
}
// 再创一个结构体 汇总一下
type Presult struct {
Ips string `json:"ips"` // ip
Ports string `json:"ports"` // port 端口
Anonymouss string `json:"anonymouss"` // 匿名度
Agreements string `json:"agreements"` //类型
Regions string `json:"regions"` //地区
Speeds string `json:"speeds"` //速度
Timeouts string `json:"timeouts"` //最后验证时间
}
func Collection() {
// 使用结构题传入
proxyList := []Datalist{}
proxyList2 := []Datalist2{}
proxyList3 := []Datalist3{}
proxyList4 := []Datalist4{}
proxyList5 := []Datalist5{}
proxyList6 := []Datalist6{}
proxyList7 := []Datalist7{}
Prelist := []Presult{}
//初始化 设置一个请求头 浏览器标识
c := colly.NewCollector(
colly.UserAgent("Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/86.0.4240.198 Safari/537.36"))
// 发出请求时附的回调
c.OnRequest(func(r *colly.Request) {
// request头部设置
r.Headers.Set("Content-Type", "application/")
})
// 请求打印状态码
c.OnResponse(func(r *colly.Response) {
fmt.Println("响应状态:", r.StatusCode)
})
// 对响应的html元素处理
c.OnHTML("title", func(e *colly.HTMLElement) {
fmt.Println("title:", e.Text)
})
// 对响应的html元素处理
c.OnHTML("table tbody", func(e *colly.HTMLElement) {
// 爬取第一个 td标签的内容
e.ForEach("td:nth-of-type(1)", func(i int, element *colly.HTMLElement) {
ip := element.Text
proxyList = append(proxyList, Datalist{
Ip: ip,
})
})
e.ForEach("td:nth-of-type(2)", func(i int, element *colly.HTMLElement) {
port := element.Text
proxyList2 = append(proxyList2, Datalist2{
Port: port,
})
})
e.ForEach("td:nth-of-type(3)", func(i int, element *colly.HTMLElement) {
anonymous := element.Text
proxyList3 = append(proxyList3, Datalist3{
Anonymous: anonymous,
})
})
e.ForEach("td:nth-of-type(4)", func(i int, element *colly.HTMLElement) {
agreement := element.Text
proxyList4 = append(proxyList4, Datalist4{
Agreement: agreement,
})
})
e.ForEach("td:nth-of-type(5)", func(i int, element *colly.HTMLElement) {
region := element.Text
proxyList5 = append(proxyList5, Datalist5{
Region: region,
})
})
e.ForEach("td:nth-of-type(6)", func(i int, element *colly.HTMLElement) {
speed := element.Text
proxyList6 = append(proxyList6, Datalist6{
Speed: speed,
})
})
e.ForEach("td:nth-of-type(7)", func(i int, element *colly.HTMLElement) {
timeout := element.Text
proxyList7 = append(proxyList7, Datalist7{
Timeout: timeout,
})
})
for i, _ := range proxyList {
Prelist = append(Prelist, Presult{
Ips: proxyList[i].Ip, //ip
Ports: proxyList2[i].Port, // port 端口
Anonymouss: proxyList3[i].Anonymous, // 匿名度
Agreements: proxyList4[i].Agreement, //类型
Regions: proxyList5[i].Region, //地区
Speeds: proxyList6[i].Speed, //速度
Timeouts: proxyList7[i].Timeout, //最后验证时间
})
}
fmt.Println(Prelist)
})
// 请求出错时
c.OnError(func(r *colly.Response, e error) {
fmt.Println(e.Error())
})
// 设置线程数
c.Limit(&colly.LimitRule{
Parallelism: 2,
})
// 爬取前3页 每页停留5秒
for i := 1; i <= 3; i++ {
c.Visit("https://www.kuaidaili.com/free/inha/" + strconv.Itoa(i) + "/")
time.Sleep(time.Second * 5)
}
}
func main() {
Collection()
}
```
- 安装开发环境
- 安装开发环境
- 安装详细教程
- 引入包
- Go语言基础
- 基本变量与数据类型
- 变量
- 数据类型
- 指针
- 字符串
- 代码总结
- 常量与运算符
- 常量
- 运算符
- 流程控制
- if判断
- for循环
- switch分支
- goto跳转
- 斐波那契数列
- Go语言内置容器
- 数组
- 切片
- 映射
- 函数
- 函数(上)
- 函数(中)
- 函数(下)
- 小节
- 包管理
- 结构体
- 结构体(上)
- 结构体(中)
- 结构体(下)
- 小节
- 错误处理
- 错误处理
- 宕机
- 错误应用
- 小节
- 文件操作
- 获取目录
- 创建和删除目录
- 文件基本操作(上)
- 文件基本操作(中)
- 文件基本操作(下)
- 处理JSON文件
- 接口与类型
- 接口的创建与实现
- 接口赋值
- 接口嵌入
- 空接口
- 类型断言(1)
- 类型断言(2)
- 小节
- 并发与通道
- goroutine协程
- runtime包
- 通道channel
- 单向通道channel
- select
- 线程同步
- 多线程的深入学习
- http编程
- http简介
- Client和Request
- get请求
- post请求
- 模块函数方法
- 模块
- fmt库,模块
- 项目练习
- 爬虫:高三网
- 爬虫:快代理
- 爬虫:快代理2
- 多线程:通道思路
- 多线程爬虫:快代理