[TOC]
*****
# 4.1 遍历整个列表
**遍历列表**
:之后可以包含缩进的多行代码,代码块
```
magicians = ['alice', 'david', 'carolina']
for magician in magicians:
print(magician.title() + ", that was a great trick!")
print("I can't wait to see your next trick, " + magician.title() + ".\\n")
```
# 4.2 避免缩进错误
Python根据缩进来判断代码行与前一个代码行的关系。在前面的示例中,向各位魔术师显示消息的代码行是for循环的一部分,因为它们缩进了
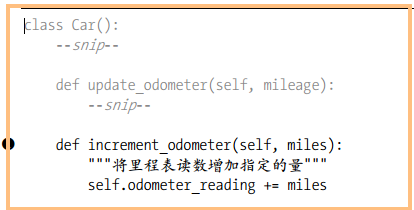
**注意**
for语句末尾不要漏掉冒号
# 4.3 创建数值列表
## 4.3.1 使用函数 range()
range()生成一系列的数字
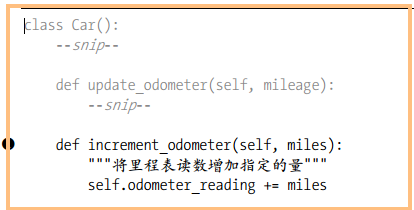
## 4.3.2 使用 range()创建数字列表
用函数list()将range()的结果转换为列表
生成1到5的数字列表
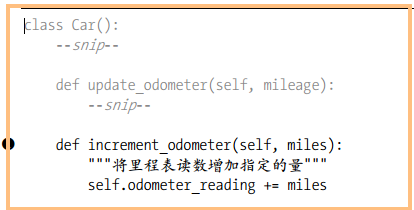
**指定生成数字列表的步长**
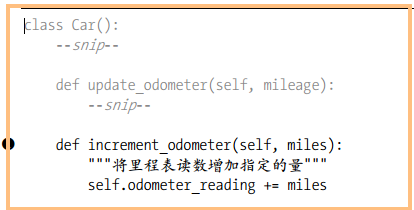
**两个星号(**)表示乘方运算**
```
int a = 5;
b = a**2;
# b =25
```
## 4.3.3 对数字列表执行简单的统计计算
```
digits = [1, 2, 3, 4, 5, 6, 7, 8, 9, 0]
min(digits)
max(digits)
sum(digits)
```
## 4.3.4 列表解析
创建数字列表
```
squares = [value**2 for value in range(1,11)]
#结果
#[1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
```
# 4.4 使用列表的一部分
切片: 列表的部分元素
## 4.4.1 切片
在到达你指定的第二个索引前面的元素停止,要输出列表中的前三个元素,需要指定索引0~3
```
players = ['charles', 'martina', 'michael', 'florence', 'eli']
print(players[0:3])
#结果
# ['charles', 'martina', 'michael']
```
没有指定第一个索引, Python将自动从列表开头开始:
```
players = ['charles', 'martina', 'michael', 'florence', 'eli']
print(players[:4])
#结果
#['charles', 'martina', 'michael', 'florence']
```
让切片终止于列表末尾 例如: 从第3个元素到列表末尾的所有元素
```
players = ['charles', 'martina', 'michael', 'florence', 'eli']
print(players[2:])
#结果
#['michael', 'florence', 'eli']
```
负数索引返回离列表末尾相应距离的元素
例如: 返回从倒数第三个元素到列表末尾的所有元素
使用切片players[-3:]
## 4.4.2 用for循环遍历切片
```
players = ['charles', 'martina', 'michael', 'florence', 'eli']
for player in players[:3]:
print(player.title())
#Here are the first three players on my team:
#Charles
#Martina
#Michael
```
## 4.4.3 使用切片复制列表
```
my_foods = ['pizza', 'falafel', 'carrot cake']
friend_foods = my_foods[:]
```
friend_foods 是 my_foods 列表的复制
# 4.5 元组
不可变的列表被称为元组
## 4.5.1 定义元组
```
dimensions = (200, 50)
print(dimensions[0])
#结果
# 200
```
如果试图修改元组中的元素,将返回错误消息
dimensions[0] = 250
```
Traceback (most recent call last):
File "dimensions.py", line 3, in
dimensions\[0\] = 250
TypeError: 'tuple' object does not support item assignment
```
## 4.5.2 for循环遍历元组中的所有值
```
dimensions = (200, 50)
for dimension in dimensions:
print(dimension)
```
200
50
## 4.5.3 修改元组变量
```
dimensions = (200, 50)
dimensions = (400, 100)
```
# 4.6 设置代码格式
PEP 8:请访问 https://python.org/dev/peps/pep-0008/