[TOC]
<!--
The Toolbar module allow users to easily format Quill's contents.
-->
工具栏模块允许用户容易的设置Quill的内容格式。
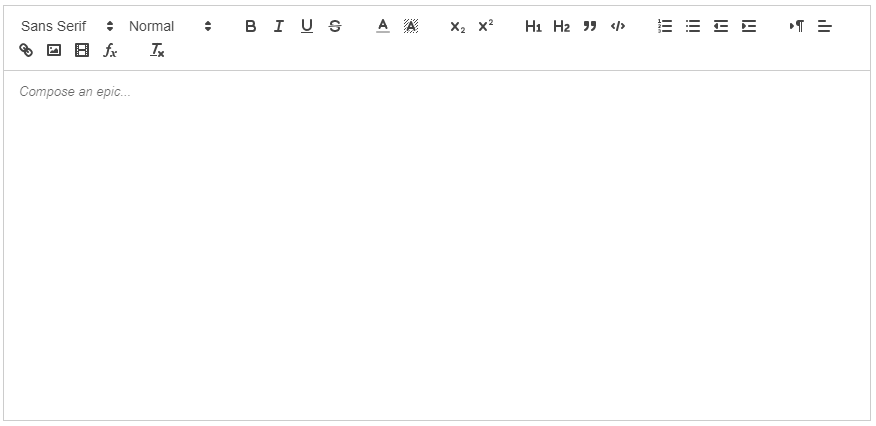
<!--
It can be configured with a custom container and handlers.
-->
可以用自定义的容器和处理程序定义。
```javascript
var quill = new Quill('#editor', {
modules: {
toolbar: {
container: '#toolbar', // Selector for toolbar container
handlers: {
'formula': customFormulaHandler
}
}
}
});
```
<!--
Because the `container` option is so common, a top level shorthand is also allowed.
-->
因为`container`选项是非常通用的,允许在上一级简写。
```javascript
var quill = new Quill('#editor', {
modules: {
// Equivalent to { toolbar: { container: '#toolbar' }}
toolbar: '#toolbar'
}
});
```
## 容器
<!--
Toolbar controls can either be specified by a simple array of format names or a custom HTML container.
-->
工具栏控件即可以用一个格式名称数组定义,也可以用一般的HTML容器定义。
To begin with the simpler array option:
```javascript
var toolbarOptions = ['bold', 'italic', 'underline', 'strike'];
var quill = new Quill('#editor', {
modules: {
toolbar: toolbarOptions
}
});
```
<!--
Controls can also be grouped by one level of nesting an array. This will wrap controls in a `<span>` with class name `ql-formats`, providing structure for themes to utilize. For example [Snow](/docs/themes/#snow/) adds extra spacing between control groups.
-->
控件也可以按嵌套数组的同一级别分组。将会用带有类名`ql-formats`的`<span>`包裹控件,提供给主题使用。例如,主题[Snow](../主题themes.md)在控件组之间添加了额外的间距。
```javascript
var toolbarOptions = [['bold', 'italic'], ['link', 'image']];
```
<!--
Buttons with custom values can be specified with an Object with the name of the format as its only key.
-->
自定义的按钮可以用带有作为它唯一键值的格式名称组成的对象来定义。
```javascript
var toolbarOptions = [{ 'header': '3' }];
```
<!--
Dropdowns are similarly specified by an Object, but with an array of possible values. CSS is used to control the visual labels for dropdown options.
-->
类似的,下拉菜单也是用对象定义,但是一用可能值组成的数组来定义。CSS被用作控制下拉菜单的可视标签。
```javascript
// Note false, not 'normal', is the correct value
// quill.format('size', false) removes the format,
// allowing default styling to work
var toolbarOptions = [
{ size: [ 'small', false, 'large', 'huge' ]}
];
```
<!--
Note [Themes](/docs/themes/) may also specify default values for dropdowns. For example, [Snow](/docs/themes/#snow/) provides a default list of 35 colors for the `color` and `background` formats, if set to an empty array.
-->
注意,[主题](../主题themes.md)也可以定义下拉菜单的默认值。例如,如果下拉菜单被设置成空数组,[Snow](../主题themes.md) 主题默认提供 `color` 和 `background` 格式的35种颜色列表供选择。
```javascript
var toolbarOptions = [
['bold', 'italic', 'underline', 'strike'], // toggled buttons
['blockquote', 'code-block'],
[{ 'header': 1 }, { 'header': 2 }], // custom button values
[{ 'list': 'ordered'}, { 'list': 'bullet' }],
[{ 'script': 'sub'}, { 'script': 'super' }], // superscript/subscript
[{ 'indent': '-1'}, { 'indent': '+1' }], // outdent/indent
[{ 'direction': 'rtl' }], // text direction
[{ 'size': ['small', false, 'large', 'huge'] }], // custom dropdown
[{ 'header': [1, 2, 3, 4, 5, 6, false] }],
[{ 'color': [] }, { 'background': [] }], // dropdown with defaults from theme
[{ 'font': [] }],
[{ 'align': [] }],
['clean'] // remove formatting button
];
var quill = new Quill('#editor', {
modules: {
toolbar: toolbarOptions
},
theme: 'snow'
});
```
<!--
For use cases requiring even more customization, you can manually create a toolbar in HTML, and pass the DOM element or selector into Quill. The `ql-toolbar` class will be added to the toolbar container and Quill attach appropriate handlers to `<button>` and `<select>` elements with a class name in the form `ql-${format}`. Buttons element may optionally have a custom `value` attribute.
-->
如果用户案例需要更多的定制,你可以用HTML手动创建一个工具栏,然后传入DOM元素或选择器到Quill。工具栏容器将添加`ql-toolbar`类,Quill附加合适的处理程序给带有`ql-${format}`格式类名的 `<button>` 和`<select>` 元素。 按钮元素可选设置一个自定义 `value`属性。
```html
<!-- Create toolbar container -->
<div id="toolbar">
<!-- Add font size dropdown -->
<select class="ql-size">
<option value="small"></option>
<!-- Note a missing, thus falsy value, is used to reset to default -->
<option selected></option>
<option value="large"></option>
<option value="huge"></option>
</select>
<!-- Add a bold button -->
<button class="ql-bold"></button>
<!-- Add subscript and superscript buttons -->
<button class="ql-script" value="sub"></button>
<button class="ql-script" value="super"></button>
</div>
<div id="editor"></div>
<!-- Initialize editor with toolbar -->
<script>
var quill = new Quill('#editor', {
modules: {
toolbar: '#toolbar'
}
});
</script>
```
<!--
Note by supplying your own HTML element, Quill searches for particular input elements, but your own inputs that have nothing to do with Quill can still be added and styled and coexist.
-->
注意,如果你提供自己的HTML元素,Quill会搜索特定输入的元素,与Quill无关的,你自己输入的元素仍然可以添加、设置样式、共存。
```html
<div id="toolbar">
<!-- Add buttons as you would before -->
<button class="ql-bold"></button>
<button class="ql-italic"></button>
<!-- But you can also add your own -->
<button id="custom-button"></button>
</div>
<div id="editor"></div>
<script>
var quill = new Quill('#editor', {
modules: {
toolbar: '#toolbar'
}
});
var customButton = document.querySelector('#custom-button');
customButton.addEventListener('click', function() {
console.log('Clicked!');
});
</script>
```
## Handlers
<!--
The toolbar controls by default applies and removes formatting, but you can also overwrite this with custom handlers, for example in order to show external UI.
Handler functions will be bound to the toolbar (so using `this` will refer to the toolbar instance) and passed the `value` attribute of the input if the corresponding format is inactive, and `false` otherwise. Adding a custom handler will overwrite the default toolbar and theme behavior.
-->
工具栏空间默认定义应用和删除格式,但是你也可以用自定义处理程序重写,例如为了显示外部UI。
处理程序函数将始终绑定到工具栏(所以使用`this`指的是工具栏实例),并在对应格式没有激活下传入`value`值,否则传入`false`。添加自定义处理程序将覆盖默认的工具栏和主题行为。
```javascript
var toolbarOptions = {
handlers: {
// handlers object will be merged with default handlers object
'link': function(value) {
if (value) {
var href = prompt('Enter the URL');
this.quill.format('link', href);
} else {
this.quill.format('link', false);
}
}
}
}
var quill = new Quill('#editor', {
modules: {
toolbar: toolbarOptions
}
});
// Handlers can also be added post initialization
var toolbar = quill.getModule('toolbar');
toolbar.addHandler('image', showImageUI);
```
<!-- script -->
<script src="{{site.cdn}}1.3.6/{{site.quill}}"></script>
<script>
var quill = new Quill('#toolbar-editor', {
modules: {
toolbar: { container: '#toolbar-toolbar' }
},
theme: 'snow'
});
</script>
<!-- script -->
- 前言
- 快速开始(quick_start)
- 下载(download)
- 配置(configuration)
- 格式(formats)
- API
- 内容(contents)
- 格式化(formatting)
- 选区(selection)
- 编辑器(editor)
- 事件(events)
- 模型(model)
- 扩展(extension)
- 增量(Delta)
- 模块(modules)
- 工具栏(toolbar)
- 键盘(keyboard)
- 历史记录(history)
- 粘贴板(clipboard)
- 语法高亮(syntax)
- 主题(themes)
- 更多教程
- 为什么选择Quill?
- 如何定制Quill?
- 设计Delta格式(未翻译)
- 构建一个自定义模块
- 将Quill加入你的编译管线(未翻译)
- Cloning Medium with Parchment
- 和其它富文本编辑器的对比(未翻译)
- Designing the Delta Format
- 扩展模块
- vue-quill-editor
- quill-image-extend-module
- quill-image-resize-module
- quill-image-drop-module
- quill-better-table
- quilljs-table
- 更多模块