首先我们移除 eslint 的校验
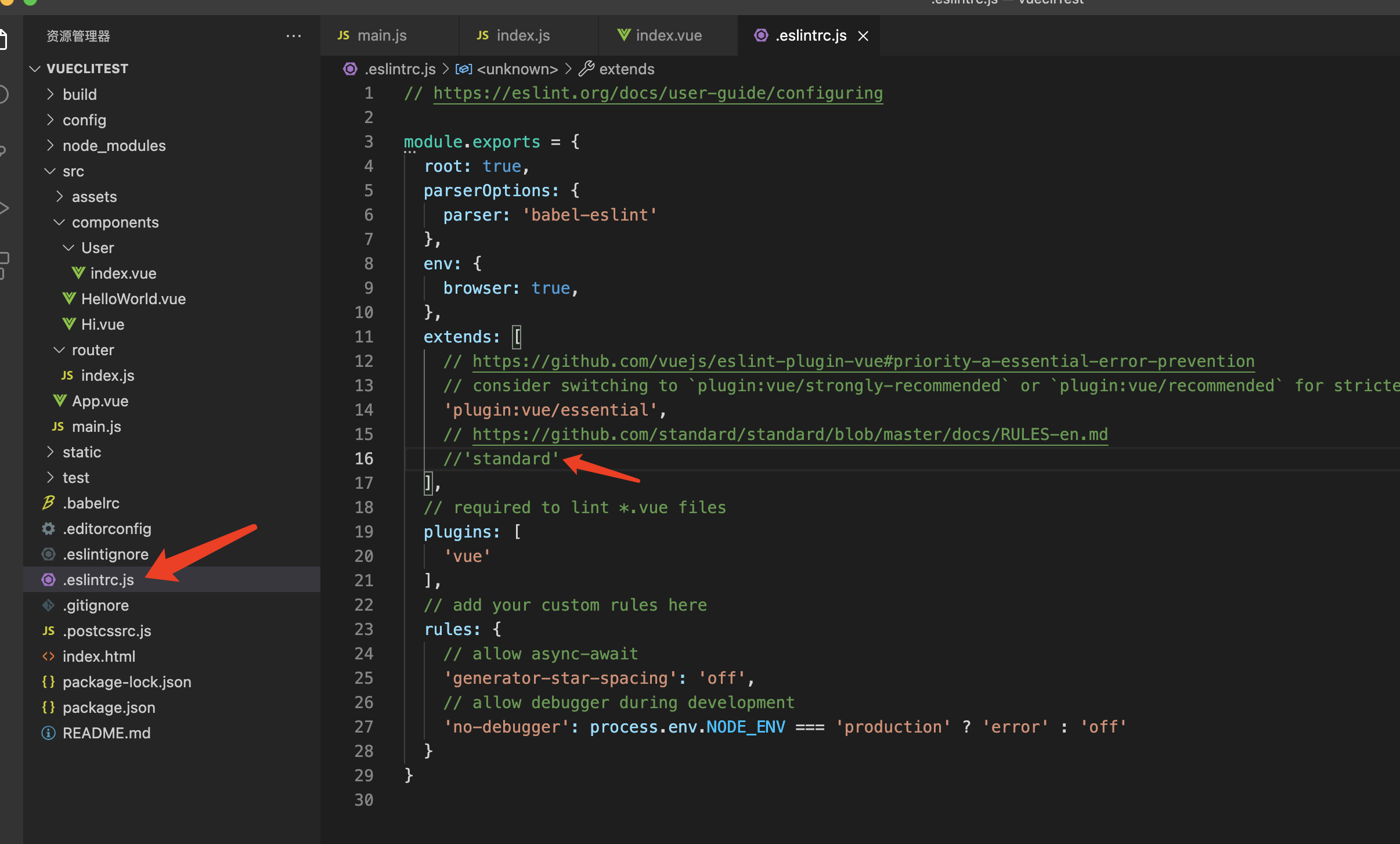
接下来我们正式进入`增删改查` 的正式操作。 查询
## 1、在 `router/index.js` 下添加如下的路由
```
import User from '@/components/User'
```
```
{
path: '/user',
name: 'User',
component: User
}
```
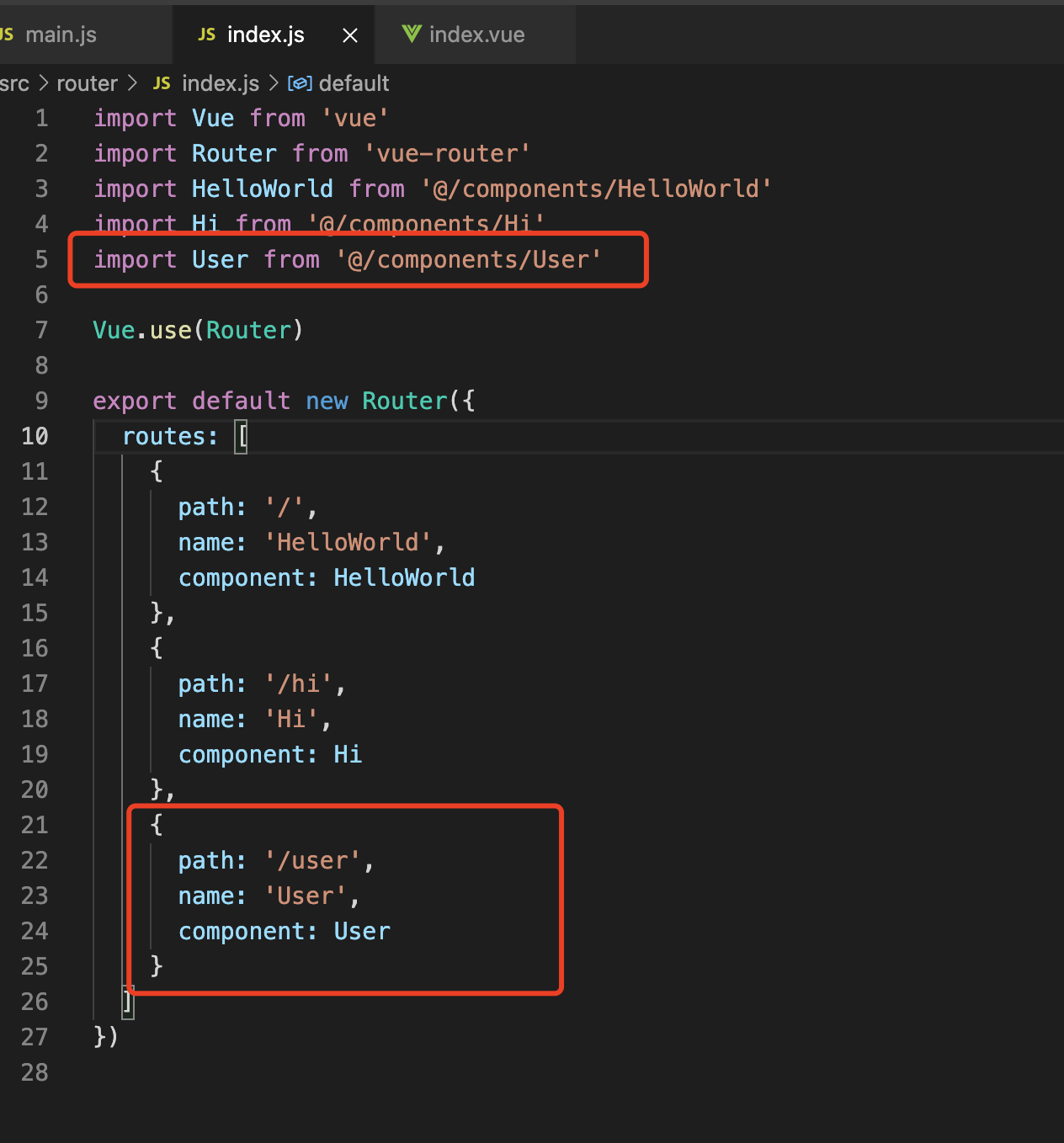
同时 在 components 下新建 User 文件夹,并在 User 文件夹下新建 index.vue 文件
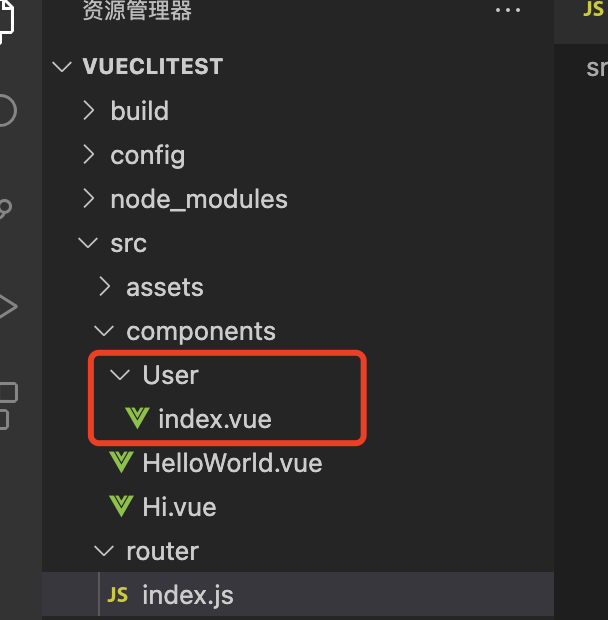
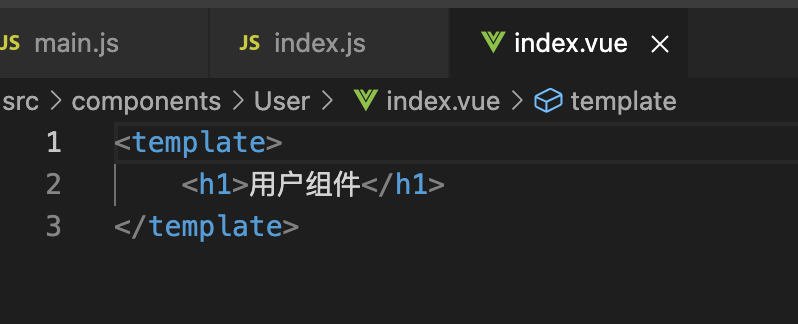
## 2、启动 npm 调试
```
npm run dev
```
访问 http://localhost:8080/#/user 看到如下页面
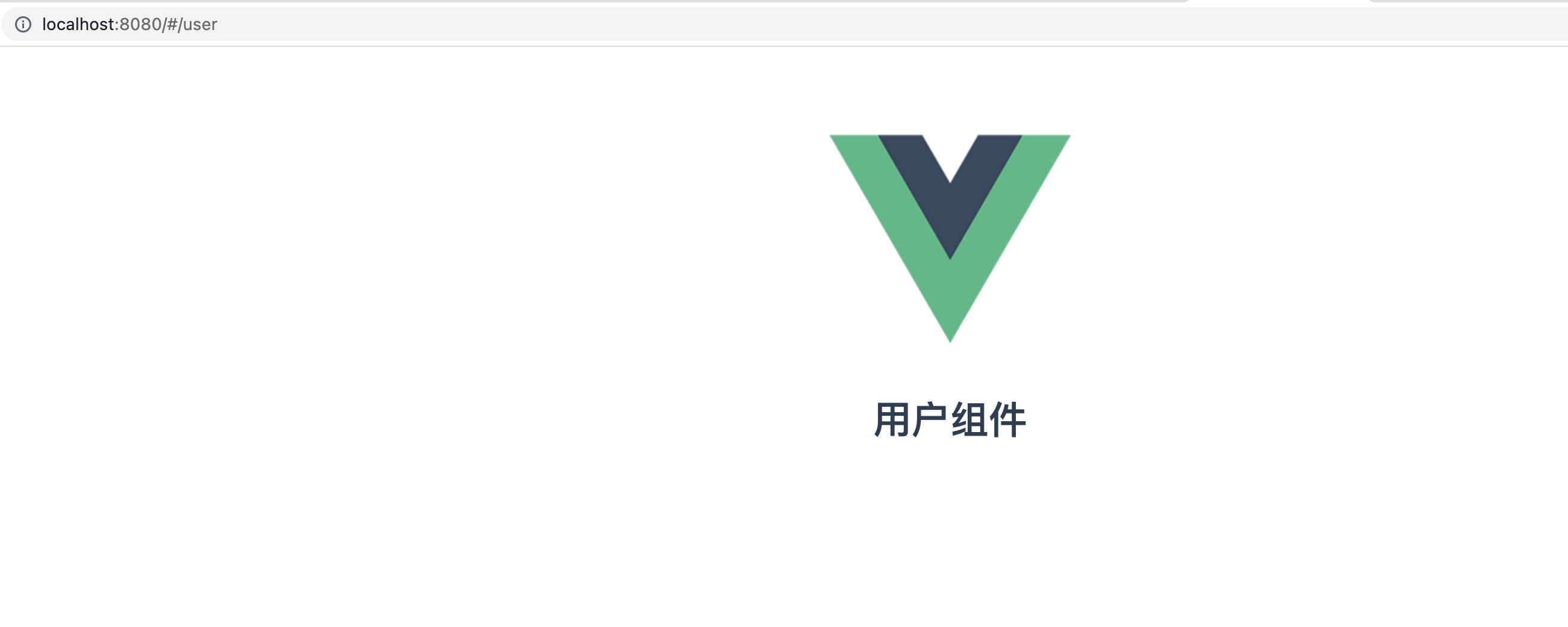
## 3、去 elementui 网站去找 table组件
https://element.eleme.cn/#/zh-CN/component/table
从业务需求来看,我们需要在列表上展示 `id`,`name`, `age`,`create_time` 同时还得有个操作的按钮,这几个内容,因此我们需要编辑表格的 表头
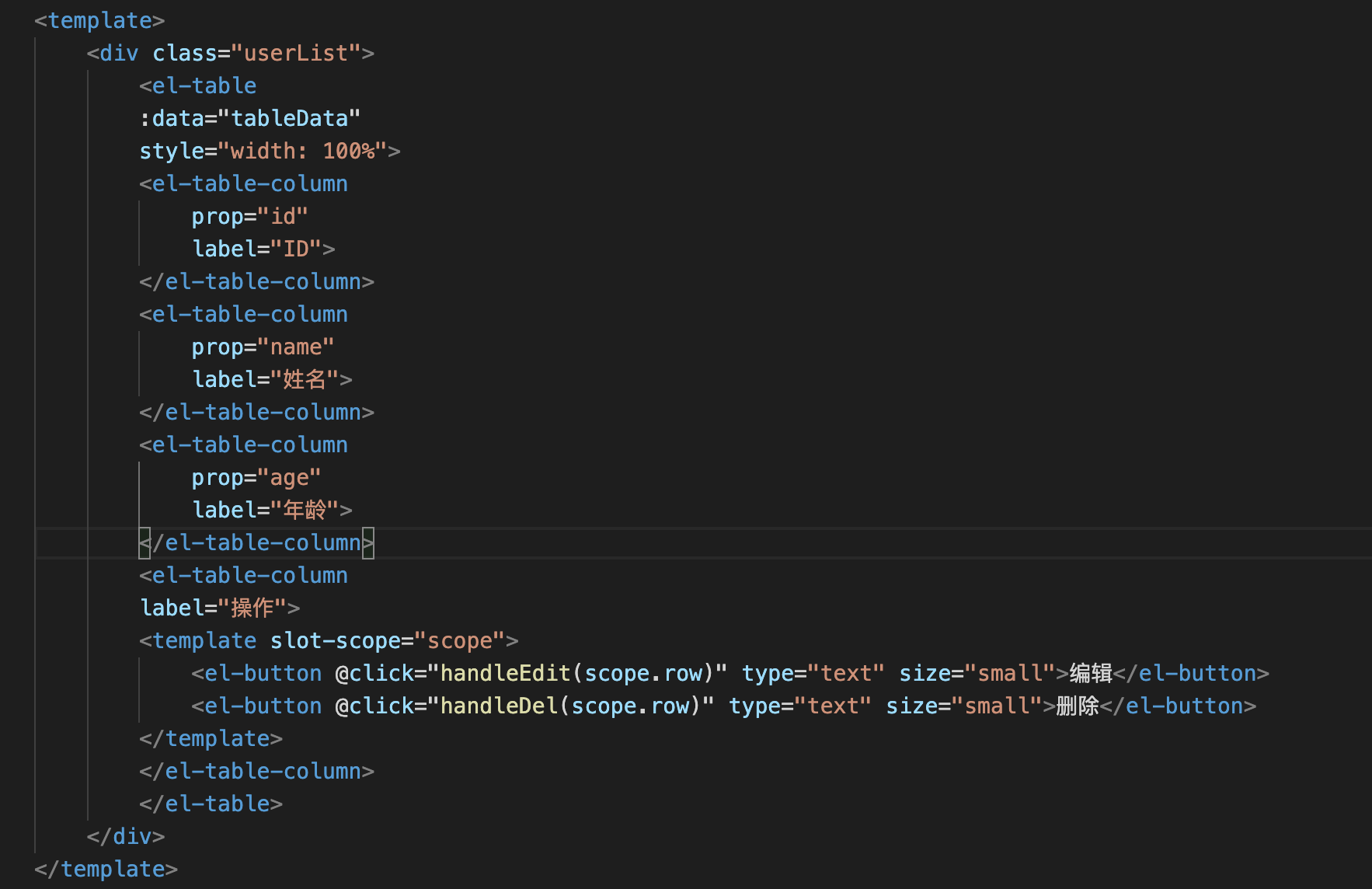
另外,我们操作的地方放了 两个点击的操作,因此我们要在 methods 中定义这两个操作
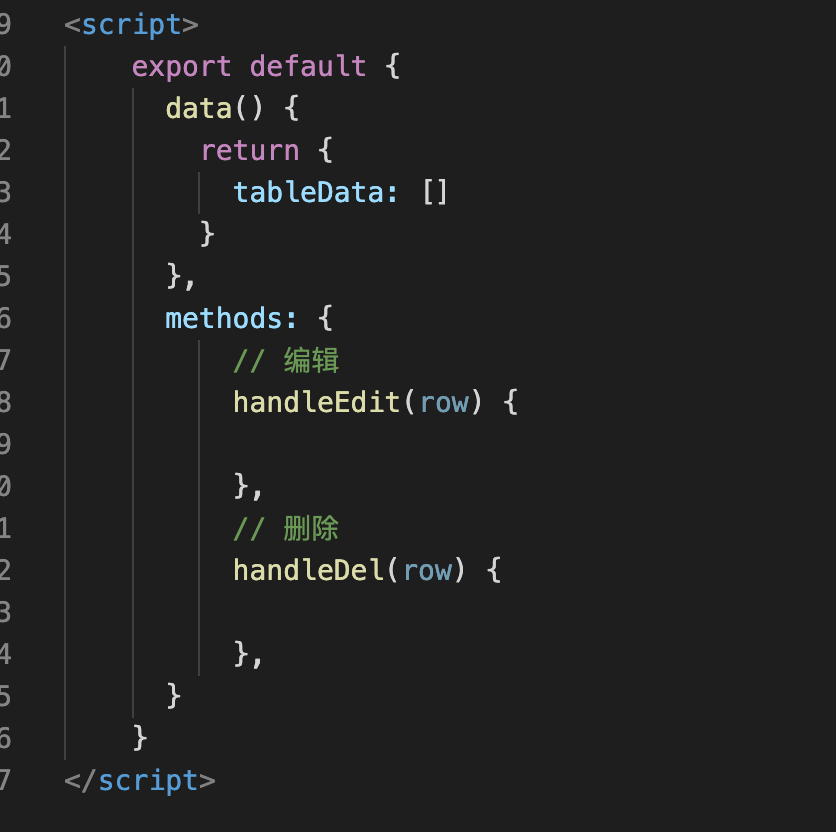
```
<template>
<div class="userList">
<el-table
:data="tableData"
style="width: 100%">
<el-table-column
prop="id"
label="ID">
</el-table-column>
<el-table-column
prop="name"
label="姓名">
</el-table-column>
<el-table-column
prop="age"
label="年龄">
</el-table-column>
<el-table-column
label="操作">
<template slot-scope="scope">
<el-button @click="handleEdit(scope.row)" type="text" size="small">编辑</el-button>
<el-button @click="handleDel(scope.row)" type="text" size="small">删除</el-button>
</template>
</el-table-column>
</el-table>
</div>
</template>
<script>
export default {
data() {
return {
tableData: []
}
},
methods: {
// 编辑
handleEdit(row) {
},
// 删除
handleDel(row) {
},
}
}
</script>
```
## 4、开始请求接口获取列表数据
我们定义一个方法 叫 getUserList 去调用接口获取数据
根据接口文档,
请求地址: http://api2.pfecms.com/user/index
参数:page limit
```
getUserList() {
this.$http({
method: 'get',
url: 'http://api2.pfecms.com/user/index',
data: {
limit: 15,
page: 1
},
headers:{
'Content-Type': 'application/x-www-form-urlencoded; charset=UTF-8'
}
}).then((res) => {
let result = res.data;
}).catch((error) => {
console.log(error);
});
}
```
并且在 组件挂载之后就调用,填入我们对应的 地址和参数,就可以调用接口了
在 then的回调中,res.data 就是服务器返回的数据
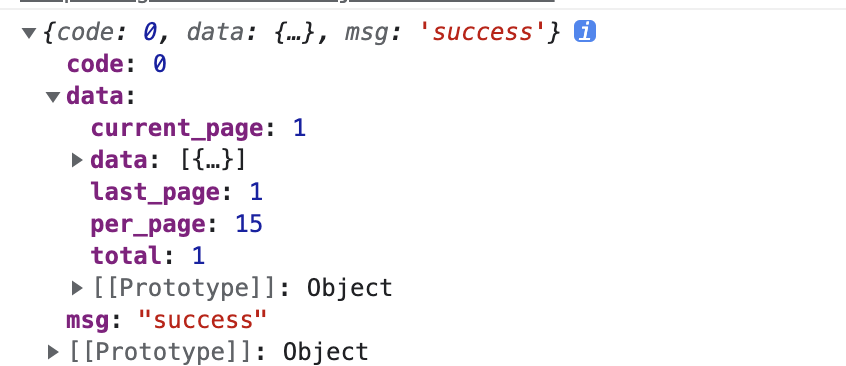
>[danger] 服务器返回的数据中data 中的 data 就是存放的用户列表数据
```
let result = res.data;
if (result.code == 0) {
this.tableData = result.data.data
}
```
因此,我们只需要将 tableData 赋值,即可让数据表格显示用户列表数据
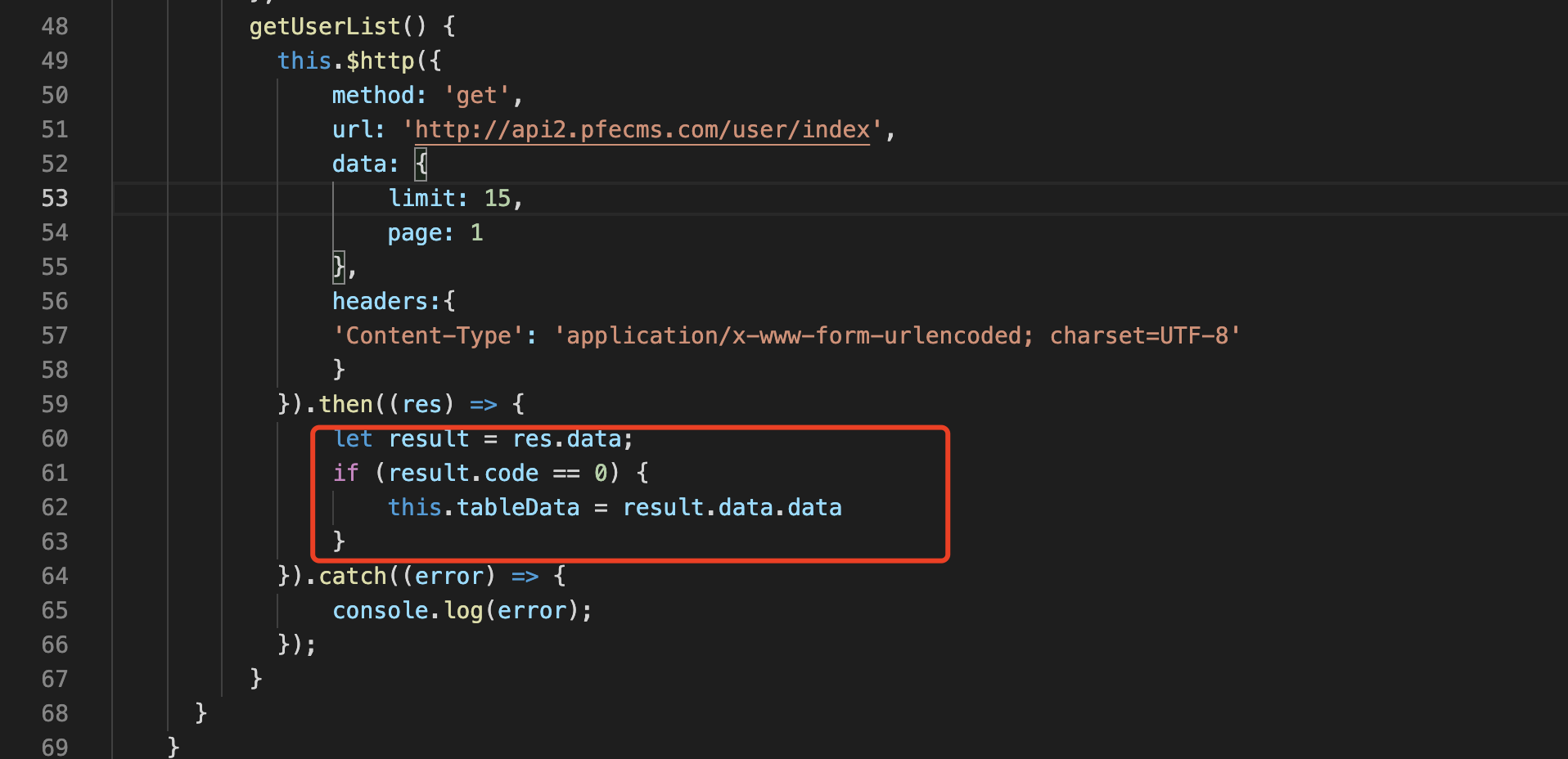
