# 实操案例:烤土豆
为了更好的理解面向对象编程,下面以“烤土豆”为案例,进行分析
:-: 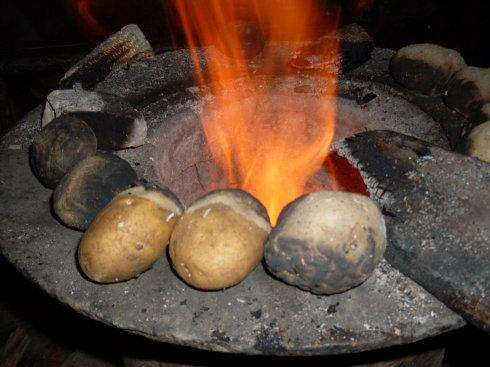
## 1.分析“烤土豆”的属性和方法
### 示例属性如下:
* cookedLevel : 这是数字;0~3表示还是生的,超过3表示半生不熟,超过5表示已经烤好了,超过8表示已经烤成木炭了!我们的土豆开始时时生的
* cookedString : 这是字符串;描述土豆的生熟程度
* condiments : 这是土豆的配料列表,比如番茄酱、芥末酱等
### 示例方法如下:
* `cook()`: 把土豆烤一段时间
* `addCondiments()`: 给土豆添加配料
* `__init__()`: 设置默认的属性
* `__str__()`: 让print的结果看起来更好一些
## 2\. 定义类,并且定义`__init__()`方法
~~~
# 定义土豆类
class Potato:
"""这是土豆的类"""
#定义初始化方法
def __init__(self):
self.cookedLevel = 0
self.cookedString = "生的"
self.condiments = []
~~~
## 3\. 添加"烤土豆"方法
~~~
#烤地瓜方法
def cook(self, time):
self.cookedLevel += time
if self.cookedLevel > 8:
self.cookedString = "烤成灰了"
elif self.cookedLevel > 5:
self.cookedString = "烤好了"
elif self.cookedLevel > 3:
self.cookedString = "半生不熟"
else:
self.cookedString = "生的"
~~~
## 4\. 基本的功能已经有了一部分,赶紧测试一下
把上面2块代码合并为一个程序后,在代码的下面添加以下代码进行测试
~~~
myPotato = Potato()
print(myPotato.cookedLevel)
print(myPotato.cookedString)
print(myPotato.condiments)
~~~
完整的代码为:
~~~
class Potato:
"""这是烤地瓜的类"""
# 定义初始化方法
def __init__(self):
self.cookedLevel = 0
self.cookedString = "生的"
self.condiments = []
# 烤土豆方法
def cook(self, time):
self.cookedLevel += time
if self.cookedLevel > 8:
self.cookedString = "烤成灰了"
elif self.cookedLevel > 5:
self.cookedString = "烤好了"
elif self.cookedLevel > 3:
self.cookedString = "半生不熟"
else:
self.cookedString = "生的"
# 用来进行测试
myPotato = Potato()
print(myPotato .cookedLevel)
print(myPotato .cookedString)
print(myPotato .condiments)
~~~
:-: 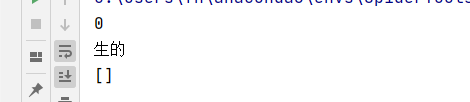
## 5\. 测试cook方法是否好用
在上面的代码最后面添加如下代码:
~~~
print("------接下来要进行烤地瓜了-----")
myPotato.cook(4) #烤4分钟
print(myPotato.cookedLevel)
print(myPotato.cookedString)
~~~
:-: 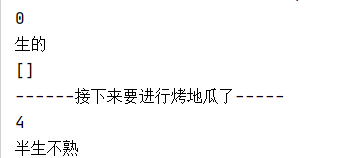
## 6\. 定义`addCondiments()`方法和`__str__()`方法
~~~
def __str__(self):
msg = self.cookedString + " 土豆"
if len(self.condiments) > 0:
msg = msg + "("
for temp in self.condiments:
msg = msg + temp + ", "
msg = msg.strip(", ")
msg = msg + ")"
return msg
def addCondiments(self, condiments):
self.condiments.append(condiments)
~~~
## 7\. 再次测试
完整的代码如下:
~~~
class Potato:
"""这是烤土豆的类"""
# 定义初始化方法
def __init__(self):
self.cookedLevel = 0
self.cookedString = "生的"
self.condiments = []
# 定制print时的显示内容
def __str__(self):
msg = self.cookedString + " 土豆"
if len(self.condiments) > 0:
msg = msg + "("
for temp in self.condiments:
msg = msg + temp + ", "
msg = msg.strip(", ")
msg = msg + ")"
return msg
# 烤地瓜方法
def cook(self, time):
self.cookedLevel += time
if self.cookedLevel > 8:
self.cookedString = "烤成灰了"
elif self.cookedLevel > 5:
self.cookedString = "烤好了"
elif self.cookedLevel > 3:
self.cookedString = "半生不熟"
else:
self.cookedString = "生的"
# 添加配料
def addCondiments(self, condiments):
self.condiments.append(condiments)
# 用来测试的代码
myPotato = Potato()
print("------有了一个土豆,还没有烤-----")
print(myPotato.cookedLevel)
print(myPotato.cookedString)
print(myPotato.condiments)
print("------接下来要进行烤土豆了-----")
print("------土豆经烤了4分钟-----")
myPotato.cook(4) #烤4分钟
print(myPotato)
print("------土豆又经烤了3分钟-----")
myPotato.cook(3) #又烤了3分钟
print(myPotato)
print("------接下来要添加配料-番茄酱------")
myPotato.addCondiments("番茄酱")
print(myPotato)
print("------土豆又经烤了5分钟-----")
myPotato.cook(5) #又烤了5分钟
print(myPotato)
print("------接下来要添加配料-芥末酱------")
myPotato.addCondiments("芥末酱")
print(myPotato)
~~~
:-: 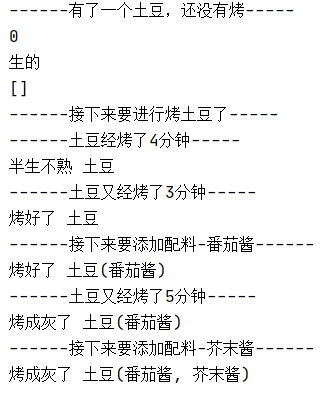
- 计算机组成原理和Python基础知识
- 计算机组成原理
- 编程语言和Python
- 开发第一个Python程序
- 注释
- 变量以及类型
- 标识符和关键字
- 输出
- 输入
- 运算符
- 数据类型转换
- 判断语句和循环语句
- 判断语句介绍
- if语句
- 比较、关系运算符
- if...else...语句格式
- if...elif...else语句格式
- if语句的嵌套
- if应用:猜拳游戏
- 循环语句介绍
- while循环
- while循环应用
- while循环的嵌套以及应用
- for循环
- break和continue
- 字符串、列表、元组、字典
- 字符串介绍
- 字符串输出
- 字符串输入
- 下标和切片
- 字符串常见操作
- 列表介绍
- 列表的循环遍历
- 列表的常见操作
- 列表的嵌套
- 元组
- 字典介绍
- 字典的常见操作1
- 字典的常见操作2
- 字典的遍历
- 集合(扩展)
- 公共方法
- 4.函数(一)
- 4.1.函数介绍
- 4.2.函数定义和调用
- 4.3.函数的文档说明
- 4.4.函数参数(一)
- 4.5.函数返回值(一)
- 4.6.函数的嵌套调用
- 4.7.函数应用:打印图形和数学计算
- 5.函数(二)
- 5.1.局部变量
- 5.2.全局变量
- 5.3.多函数程序的基本使用流程
- 5.4.函数返回值(二)
- 5.5.函数参数(二)
- 5.6.拆包、交换变量的值
- 5.7.引用(一)
- 5.8.可变、不可变类型
- 5.9.引用(二)
- 5.10.函数使用注意事项
- 6.强化练习
- 6.1.函数应用:学生管理系统
- 6.2.递归函数
- 6.3. 匿名函数
- 6.4.列表推导式
- 6.5.set、list、tuple
- 6.6.高阶函数: map reduce filter
- 7.文件操作,综合应用
- 7.1.文件操作介绍
- 7.2.文件的读写
- 7.3.应用1:制作文件的备份
- 7.4.文件的相关操作
- 7.5.应用:批量修改文件名
- 7.6.综合应用:学生管理系统(文件版)
- 8.面向对象(上)
- 8.1.认识面向对象编程
- 8.2.类和对象
- 8.3.定义类
- 8.4.创建对象
- 8.5.添加和获取对象的属性
- 8.6.在方法内通过self获取对象属性
- 8.7.魔法方法 - init()
- 8.8.魔法方法 - 有参数的__init__()方法
- 8.9.魔法方法 - str()方法
- 8.10.魔法方法 - del()方法
- 8.11.实操案例 - 烤土豆
- 9.面向对象(中)
- 9.1.实操案例 - 放家具
- 9.2.继承的概念
- 9.3.单继承
- 9.4.多继承
- 9.5.子类重写父类的同名属性和方法
- 9.6.子类调用父类同名属性和方法
- 9.7.多层继承
- 9.8.通过super()来调用父类的方法
- 10.面向对象(下)
- 10.1.私有属性和私有方法
- 10.2.修改私有属性的值
- 10.3.多态
- 10.4.类属性和实例属性
- 10.5.静态方法和类方法
- 11.异常处理与模块初识
- 11.1.异常
- 11.2.捕获异常
- 11.3.异常的传递
- 11.4.抛出自定义的异常
- 11.5.获取异常完整信息的正确姿势
- 11.6.认识模块
- 11.7.开发模块
- 11.8.模块中的__all__(未完成)
- 11.9.python中的包(未完成)
- 12.课后加餐
- 12.1.基础应用 - 进销存管理系统(未完成)
- 12.2.基础应用 - 员工信息管理系统(未完成)
- 12.1.编码规范 - PEP8编码规范(未完成)