# 捕获线程运行期间的异常
上一篇文章我们学习了在我们使用application的时候在他出现问题或者人为终止的时候,我们怎么有机会捕获异常或者人为的做一些操作,比如写一些日志,或者发送一个RESTful或者关闭释放一些资源,那么线程运行期间的异常该如何捕获呢?我们知道线程的运行逻辑单元在run方法里,run方法的签名是不允许抛出异常的,今天我们就来学习一下线程的Exception。
先来一段抛出异常的代码:
```java
public class ThreadException {
private final static int A = 10;
private static final int B = 0;
public static void main(String[] args) {
/// 捕获线程运行期间的异常
Thread t = new Thread(() -> {
try {
Thread.sleep(1_000L);
int result = A / B;
System.out.println("result = " + result);
} catch (InterruptedException e) {
e.printStackTrace();
}
});
t.start();
}
}
```
运行效果如下:
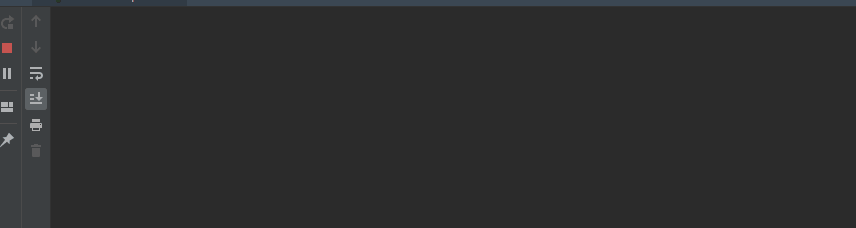
我们改造一下我们的代码,Thread对象提供了`setUncaughtExceptionHandler`方法用来获取线程中产生的异常。而且建议使用该方法为线程设置异常捕获方法:
```java
t.setUncaughtExceptionHandler((thread, e) -> {
System.out.println(e);
System.out.println(thread.getName());
System.out.println(thread.getThreadGroup().getName());
System.out.println(thread.getThreadGroup().getParent().getName());
});
```
运行效果如下:
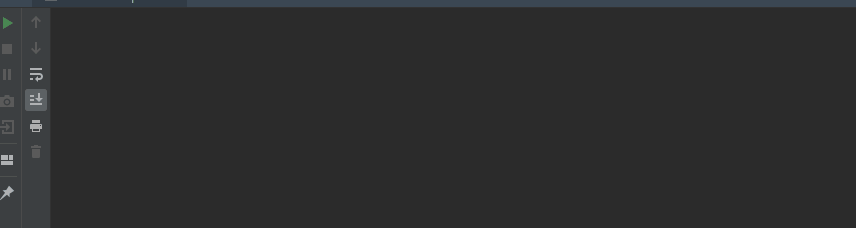
可以看到异常的详细堆栈信息已经打印出来了,下面做个实验来输出一下,先建几个类分别进行调用,如下:
```java
public class ThreadException {
public static void main(String[] args) {
// stack trace
new Test1().test();
}
}
```
```java
public class Test1 {
private Test2 test2 = new Test2();
public void test() {
test2.test();
}
}
```
```java
public class Test2 {
public void test() {
StackTraceElement[] stackTrace = Thread.currentThread().getStackTrace();
Stream.of(stackTrace)
.filter(e -> !e.isNativeMethod())
.forEach(e ->
Optional.of(e.getClassName() + "." + e.getMethodName() + ":" + e.getLineNumber())
.ifPresent(System.out::println)
);
}
}
```
运行效果如下:
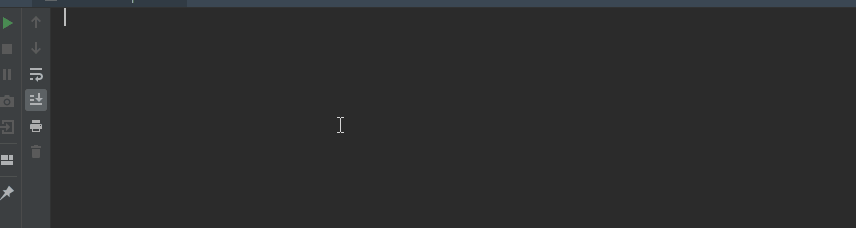
这个在后续使用中有补充再继续补充,后面开始学习ThreadGroupApI的学习,然后实现一个线程池,java并发编程的基础就码一遍了。
- 微服务
- 服务器相关
- 操作系统
- 极客时间操作系统实战笔记
- 01 程序的运行过程:从代码到机器运行
- 02 几行汇编几行C:实现一个最简单的内核
- 03 黑盒之中有什么:内核结构与设计
- Rust
- 入门:Rust开发一个简单的web服务器
- Rust的引用和租借
- 函数与函数指针
- Rust中如何面向对象编程
- 构建单线程web服务器
- 在服务器中增加线程池提高吞吐
- Java
- 并发编程
- 并发基础
- 1.创建并启动线程
- 2.java线程生命周期以及start源码剖析
- 3.采用多线程模拟银行排队叫号
- 4.Runnable接口存在的必要性
- 5.策略模式在Thread和Runnable中的应用分析
- 6.Daemon线程的创建以及使用场景分析
- 7.线程ID,优先级
- 8.Thread的join方法
- 9.Thread中断Interrupt方法学习&采用优雅的方式结束线程生命周期
- 10.编写ThreadService实现暴力结束线程
- 11.线程同步问题以及synchronized的引入
- 12.同步代码块以及同步方法之间的区别和关系
- 13.通过实验分析This锁和Class锁的存在
- 14.多线程死锁分析以及案例介绍
- 15.线程间通信快速入门,使用wait和notify进行线程间的数据通信
- 16.多Product多Consumer之间的通讯导致出现程序假死的原因分析
- 17.使用notifyAll完善多线程下的生产者消费者模型
- 18.wait和sleep的本质区别
- 19.完善数据采集程序
- 20.如何实现一个自己的显式锁Lock
- 21.addShutdownHook给你的程序注入钩子
- 22.如何捕获线程运行期间的异常
- 23.ThreadGroup API介绍
- 24.线程池原理与自定义线程池一
- 25.给线程池增加拒绝策略以及停止方法
- 26.给线程池增加自动扩充,闲时自动回收线程的功能
- JVM
- C&C++
- GDB调试工具笔记
- C&C++基础
- 一个例子理解C语言数据类型的本质
- 字节顺序-大小端模式
- Php
- Php源码阅读笔记
- Swoole相关
- Swoole基础
- php的五种运行模式
- FPM模式的生命周期
- OSI网络七层图片速查
- IP/TCP/UPD/HTTP
- swoole源代码编译安装
- 安全相关
- MySql
- Mysql基础
- 1.事务与锁
- 2.事务隔离级别与IO的关系
- 3.mysql锁机制与结构
- 4.mysql结构与sql执行
- 5.mysql物理文件
- 6.mysql性能问题
- Docker&K8s
- Docker安装java8
- Redis
- 分布式部署相关
- Redis的主从复制
- Redis的哨兵
- redis-Cluster分区方案&应用场景
- redis-Cluster哈希虚拟槽&简单搭建
- redis-Cluster redis-trib.rb 搭建&原理
- redis-Cluster集群的伸缩调优
- 源码阅读笔记
- Mq
- ELK
- ElasticSearch
- Logstash
- Kibana
- 一些好玩的东西
- 一次折腾了几天的大华摄像头调试经历
- 搬砖实用代码
- python读取excel拼接sql
- mysql大批量插入数据四种方法
- composer好用的镜像源
- ab
- 环境搭建与配置
- face_recognition本地调试笔记
- 虚拟机配置静态ip
- Centos7 Init Shell
- 发布自己的Composer包
- git推送一直失败怎么办
- Beyond Compare过期解决办法
- 我的Navicat for Mysql
- 小错误解决办法
- CLoin报错CreateProcess error=216
- mysql error You must reset your password using ALTER USER statement before executing this statement.
- VM无法连接到虚拟机
- Jetbrains相关
- IntelliJ IDEA 笔记
- CLoin的配置与使用
- PhpStormDocker环境下配置Xdebug
- PhpStorm advanced metadata
- PhpStorm PHP_CodeSniffer