# OPENAPI
## 介绍
`OpenAPI`是一个与语言无关的`RESTful API`定义说明,`Nest`提供了一个专有的模块来利用装饰器生成类似声明。
### 安装
要开始使用,首先安装依赖、
```bash
$ npm install --save @nestjs/swagger swagger-ui-express
```
如果使用fastify,安装`fastify-swagger`而不是`swagger-ui-express`:
```bash
$ npm install --save @nestjs/swagger fastify-swagger
```
### 引导
安装完成后,在`main.ts`文件中定义并初始化`SwaggerModule`类:
```TypeScript
import { NestFactory } from '@nestjs/core';
import { SwaggerModule, DocumentBuilder } from '@nestjs/swagger';
import { AppModule } from './app.module';
async function bootstrap() {
const app = await NestFactory.create(AppModule);
const config = new DocumentBuilder()
.setTitle('Cats example')
.setDescription('The cats API description')
.setVersion('1.0')
.addTag('cats')
.build();
const document = SwaggerModule.createDocument(app, config);
SwaggerModule.setup('api', app, document);
await app.listen(3000);
}
bootstrap();
```
> 文档(通过`SwaggerModule#createDocument()`方法返回)是一个遵循[OpenAPI文档](https://swagger.io/specification/#openapi-document)的序列化对象。除了HTTP,你也可以以JSON/YAML文件格式保存和使用它。
`DocumentBuilder`建立一个遵循OpenAPI 标准的基础文档。它提供了不同的方法来配置类似标题、描述、版本等信息属性。要创建一个完整的文档(使用HTTP定义),我们使用`SwaggerModule`类的`createDocument()`方法。这个方法有两个参数,一个应用实例和一个Swagger选项对象。我们也可以提供第三个`SwaggerDocumentOptions`类型可选对象,见[文档选项](#文档选项)。
创建文档后,调用`setup()`方法,它接受:
1. 挂载Swagger界面的路径。
2. 应用实例。
3. 上述实例化的文档对象。
运行以下命令启动HTTP服务器。
```TypeScript
$ npm run start
```
浏览`http://localhost:3000/api`可以看到Swagger界面。
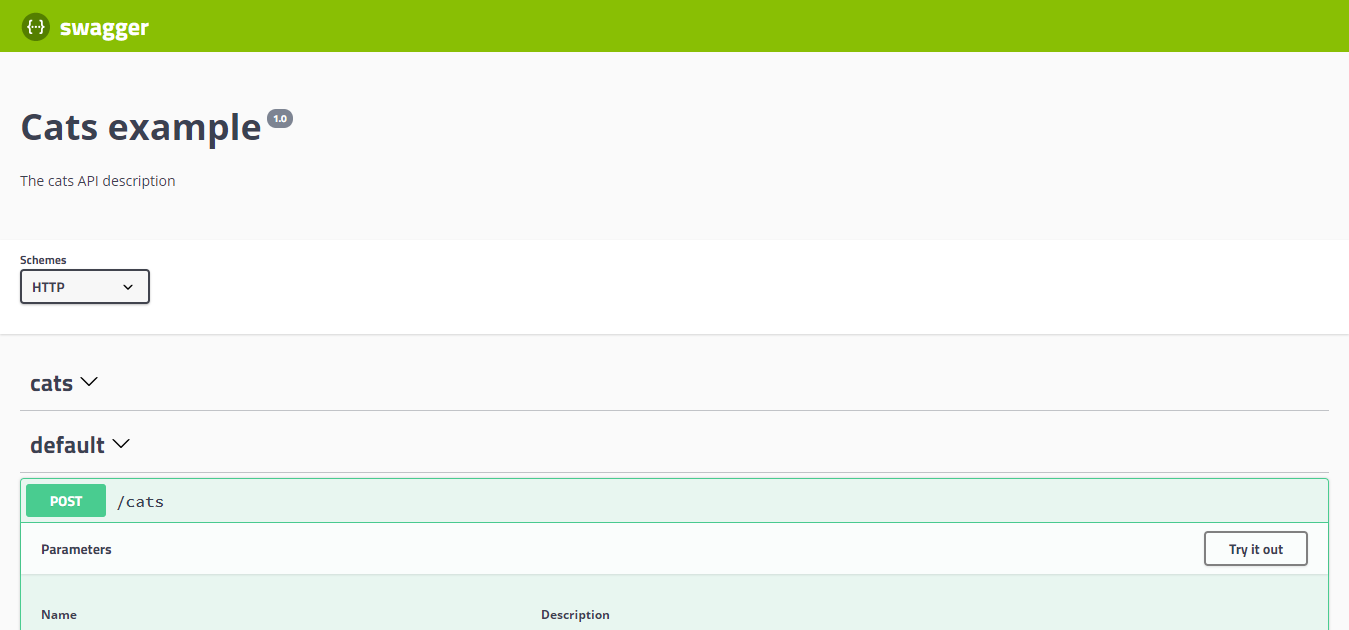
Swagger模块自动反射你所有的终端。注意Swagger界面根据平台不同,由`swagger-ui-express`或`fastify-swagger`生成。
> 要生成和下载一个Swagger JSON文件,导航到`http://localhost:3000/api-json` (`swagger-ui-express`) 或`http://localhost:3000/api/json` (`fastify-swagger`) (假设API文档在 http://localhost:3000/api路径)。
> 在使用`fastify-swagger`和`helmet`时可能有CSP问题,要处理这个冲突,参考如下配置CSP。
```TypeScript
app.register(helmet, {
contentSecurityPolicy: {
directives: {
defaultSrc: [`'self'`],
styleSrc: [`'self'`, `'unsafe-inline'`],
imgSrc: [`'self'`, 'data:', 'validator.swagger.io'],
scriptSrc: [`'self'`, `https: 'unsafe-inline'`],
},
},
});
// If you are not going to use CSP at all, you can use this:
app.register(helmet, {
contentSecurityPolicy: false,
});
```
### 文档选项
创建文档时,可以提供一些额外选项来配合库特性。这些选项应该是`SwaggerDocumentOptions`类型:
```TypeScript
export interface SwaggerDocumentOptions {
/**
* List of modules to include in the specification
*/
include?: Function[];
/**
* Additional, extra models that should be inspected and included in the specification
*/
extraModels?: Function[];
/**
* If `true`, swagger will ignore the global prefix set through `setGlobalPrefix()` method
*/
ignoreGlobalPrefix?: boolean;
/**
* If `true`, swagger will also load routes from the modules imported by `include` modules
*/
deepScanRoutes?: boolean;
/**
* Custom operationIdFactory that will be used to generate the `operationId`
* based on the `controllerKey` and `methodKey`
* @default () => controllerKey_methodKey
*/
operationIdFactory?: (controllerKey: string, methodKey: string) => string;
}
```
例如,如果你要确保库像`createUser`而不是`UserController_createUser`一样生成操作名称,可以做如下配置:
```TypeScript
const options: SwaggerDocumentOptions = {
operationIdFactory: (
controllerKey: string,
methodKey: string
) => methodKey
});
const document = SwaggerModule.createDocument(app, config, options);
```
### 设置选项[#](#setup-options)
您可以通过传递满足`ExpressSwaggerCustomOptions`(如果使用express)接口的选项对象作为`SwaggerModule#setup`方法的第四个参数来配置Swagger UI。
~~~typescript
export interface ExpressSwaggerCustomOptions {
explorer?: boolean;
swaggerOptions?: Record<string, any>;
customCss?: string;
customCssUrl?: string;
customJs?: string;
customfavIcon?: string;
swaggerUrl?: string;
customSiteTitle?: string;
validatorUrl?: string;
url?: string;
urls?: Record<'url' | 'name', string>[];
}
~~~
如果你使用 fastify,你可以通过传递`FastifySwaggerCustomOptions`对象来配置用户界面。
~~~typescript
export interface FastifySwaggerCustomOptions {
uiConfig?: Record<string, any>;
}
~~~
例如,如果您想确保身份验证令牌在刷新页面后仍然存在,或者更改页面标题(显示在浏览器中),您可以使用以下设置:
~~~typescript
const customOptions: SwaggerCustomOptions = {
swaggerOptions: {
persistAuthorization: true,
},
customSiteTitle: 'My API Docs',
};
SwaggerModule.setup('docs', app, document, customOptions);
~~~
### 示例
一个例子见[这里](https://github.com/nestjs/nest/tree/master/sample/11-swagger)。
- 介绍
- 概述
- 第一步
- 控制器
- 提供者
- 模块
- 中间件
- 异常过滤器
- 管道
- 守卫
- 拦截器
- 自定义装饰器
- 基础知识
- 自定义提供者
- 异步提供者
- 动态模块
- 注入作用域
- 循环依赖
- 模块参考
- 懒加载模块
- 应用上下文
- 生命周期事件
- 跨平台
- 测试
- 技术
- 数据库
- Mongo
- 配置
- 验证
- 缓存
- 序列化
- 版本控制
- 定时任务
- 队列
- 日志
- Cookies
- 事件
- 压缩
- 文件上传
- 流式处理文件
- HTTP模块
- Session(会话)
- MVC
- 性能(Fastify)
- 服务器端事件发送
- 安全
- 认证(Authentication)
- 授权(Authorization)
- 加密和散列
- Helmet
- CORS(跨域请求)
- CSRF保护
- 限速
- GraphQL
- 快速开始
- 解析器(resolvers)
- 变更(Mutations)
- 订阅(Subscriptions)
- 标量(Scalars)
- 指令(directives)
- 接口(Interfaces)
- 联合类型
- 枚举(Enums)
- 字段中间件
- 映射类型
- 插件
- 复杂性
- 扩展
- CLI插件
- 生成SDL
- 其他功能
- 联合服务
- 迁移指南
- Websocket
- 网关
- 异常过滤器
- 管道
- 守卫
- 拦截器
- 适配器
- 微服务
- 概述
- Redis
- MQTT
- NATS
- RabbitMQ
- Kafka
- gRPC
- 自定义传输器
- 异常过滤器
- 管道
- 守卫
- 拦截器
- 独立应用
- Cli
- 概述
- 工作空间
- 库
- 用法
- 脚本
- Openapi
- 介绍
- 类型和参数
- 操作
- 安全
- 映射类型
- 装饰器
- CLI插件
- 其他特性
- 迁移指南
- 秘籍
- CRUD 生成器
- 热重载
- MikroORM
- TypeORM
- Mongoose
- 序列化
- 路由模块
- Swagger
- 健康检查
- CQRS
- 文档
- Prisma
- 静态服务
- Nest Commander
- 问答
- Serverless
- HTTP 适配器
- 全局路由前缀
- 混合应用
- HTTPS 和多服务器
- 请求生命周期
- 常见错误
- 实例
- 迁移指南
- 发现
- 谁在使用Nest?