## 创建core/Database.php
```
<?php
namespace core;
use core\database\connection\MysqlConnection;
class Database
{
protected $connections = []; // all connection
// 获取默认链接
protected function getDefaultConnection()
{
return \App::getContainer()->get('config')->get('database.default');
}
// 设置默认链接
public function setDefaultConnection($name)
{
\App::getContainer()->get('config')->set('database.default', $name);
}
// 根据配置信息的name来创建链接
public function connection($name = null)
{
if (isset($this->connections[$name])) // 如果存在就直接返回
return $this->connections[$name];
if ($name == null) // 选择默认链接
$name = $this->getDefaultConnection();
$config = \App::getContainer()->get('config')->get('database.connections.' . $name); // 获取链接的配置
$connectionClass = null; // 链接处理的类
switch ($config['driver']) {
case 'mysql':
$connectionClass = MysqlConnection::class;
break;
// 如果有其他类型的数据库 那就继续完善
}
$dsn = sprintf('%s:host=%s;dbname=%s', $config['driver'], $config['host'], $config['dbname']);
try {
$pdo = new \PDO($dsn, $config['username'], $config['password'], $config['options']);
} catch (\PDOException $e) {
die($e->getMessage());
}
return $this->connections[$name] = new $connectionClass($pdo, $config);
}
// 代理模式
public function __call($method, $parameters)
{
return $this->connection()->$method(...$parameters);
}
}
```
## 创建core/database/connection/Connection.php
```
<?php
namespace core\database\connection;
// 链接的基础类
class Connection
{
protected $pdo;
protected $tablePrefix;
protected $config;
public function __construct($pdo, $config)
{
$this->pdo = $pdo;
$this->tablePrefix = $config['prefix'];
$this->config = $config;
}
}
```
## 创建core/database/connection/MysqlConnection.php
```
<?php
namespace core\database\connection;
// 继承基础类
class MysqlConnection extends Connection
{
protected static $connection;
public function getConnection()
{
return self::$connection;
}
// 执行sql
public function select($sql, $bindings = [], $useReadPdo = true)
{
$statement = $this->pdo;
$sth = $statement->prepare($sql);
try {
$sth->execute( $bindings);
return $sth->fetchAll();
} catch (\PDOException $exception){
echo ($exception->getMessage());
}
}
}
```
## 绑定db
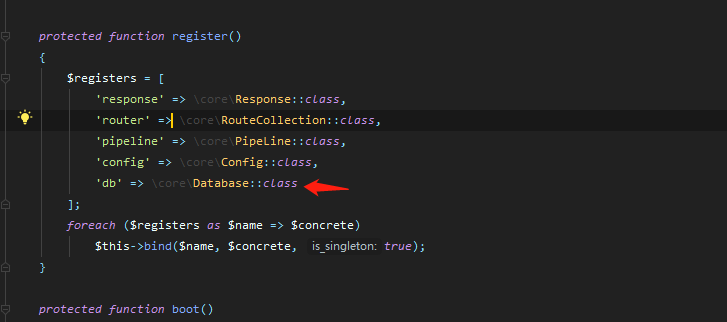
## 运行
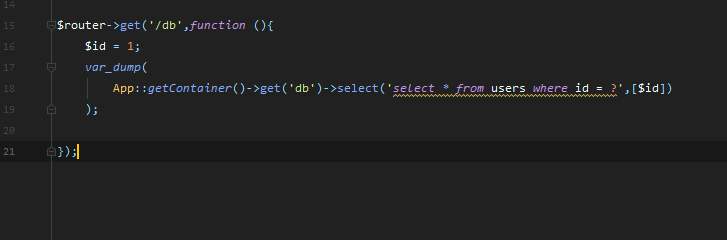
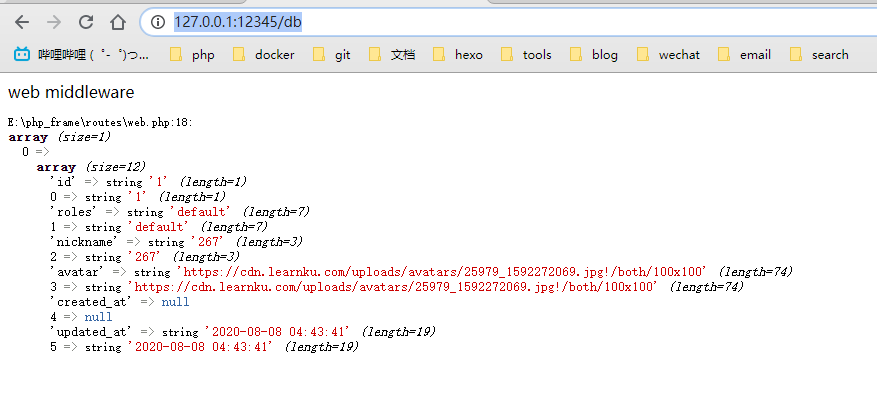
### 关于数据库配置 config/database.php
```
<?php
return [
'default' => 'mysql_one',
'connections' => [
'mysql_one' => [
'driver' => 'mysql',
'host' => '134.175.80.215', // 这是我的一个远程服务器 没用的 但是也别乱搞
'username' => 'php_frame',
'dbname' => 'php_frame',
'password' => '12345678',
'prefix' => '',
'options' => [
]
],
]
];
```
- 前言
- 基础篇
- 1. 第一步 创建框架目录结构
- 2. 引入composer自动加载
- 3. php自动加载 (解释篇)
- 4. 创建容器 注册树模式
- 5. 关于psr规范解释
- 6. 关于"容器" "契约" "依赖注入" (解释篇)
- 7. 添加函数文件helpers.php
- 8. 初始化请求(Request)
- 9. 响应 (Response)
- 10. 路由一 (路由组实现)
- 11. 路由二 (加入中间件)
- 12. 配置信息 (类似laravel)
- 13. 数据库连接 (多例模式)
- 14. 查询构造器 (query builder)
- MVC实现
- M 模型实现 (数据映射 + 原型 模式)
- C 控制器实现 + 控制器中间件
- V 视图实现 (Laravel Blade 引擎)
- V 视图切换成 ThinkPhp 模板 引擎)
- 其他轮子
- 日志
- 自定义异常 (异常托管)
- 单元测试 (phpunit)
- 替换成swoole的http服务器
- 协程上下文解决request问题
- qps测试
- 发布到packagist.org