[toc]
# 基础部分
## 编译/执行
main.c
```c
#include <stdio.h>
int main() {
printf("我的第一个程序,你好,%s \n", "打工人");
return 0;
}
```
编译并运行 `gcc main.c && ./a.out`
gcc main.c 编译
./a.out 运行编译结果
## 文件操作
```c
char fname[] = "./lzsb.txt";
```
### 写入文件
```c
char str[] = "你好,打工人"; // 欲写入内容
FILE* f = fopen(fname, "w"); // 打开文件
fwrite(str, sizeof(str), 1, f); // 写入
fclose(f); // 关闭文件占用
```
### 读取文件
```
FILE *f = fopen(fname, "r");
/* 取文件长度 START (适用2GB内文件) */
fseek(f, 0, SEEK_END);
int size = ftell(f);
fseek(f, 0, SEEK_SET);
/* 取文件长度 END */
char buffer[size]; // 设置缓存区
fseek(f, 0, SEEK_SET);
fread(buffer, size, 1, f); // 缓存变量, 读取长度, 元素个数, 文件流
fclose(f);
printf("%s", buffer);
```
## bit和byte
> bit 比特位, 8bit = 1byte
计算机只能认识二进制,每个bit只能存放0或1, 所以`bit`是计算机能读懂的最小单位
```
11111111 一个字节,同时是8个bit(比特位)
01011011
```
```
比特位,bit, b
字节,Byte, B
```
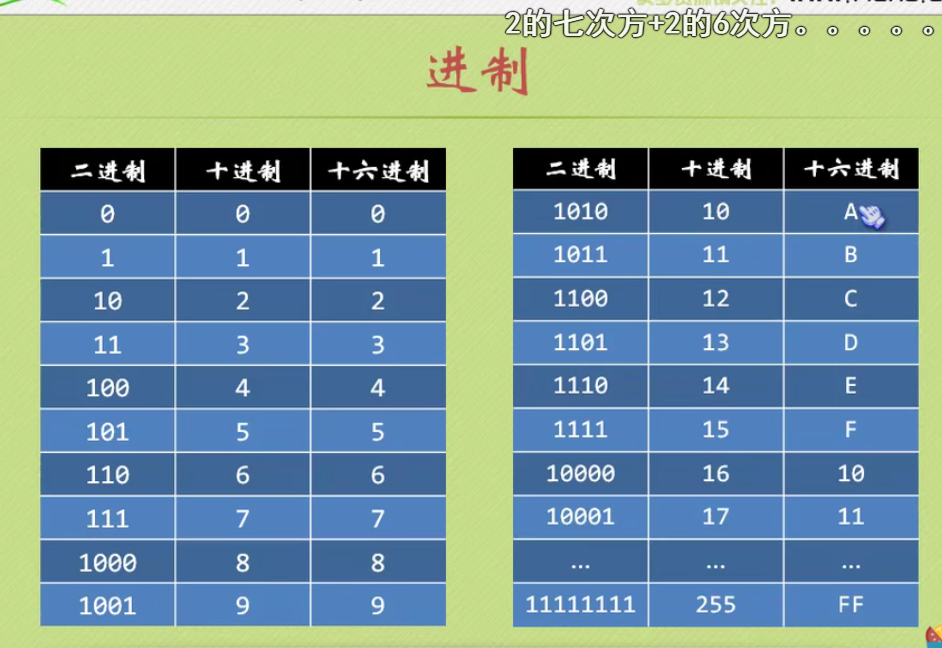
## 取值范围
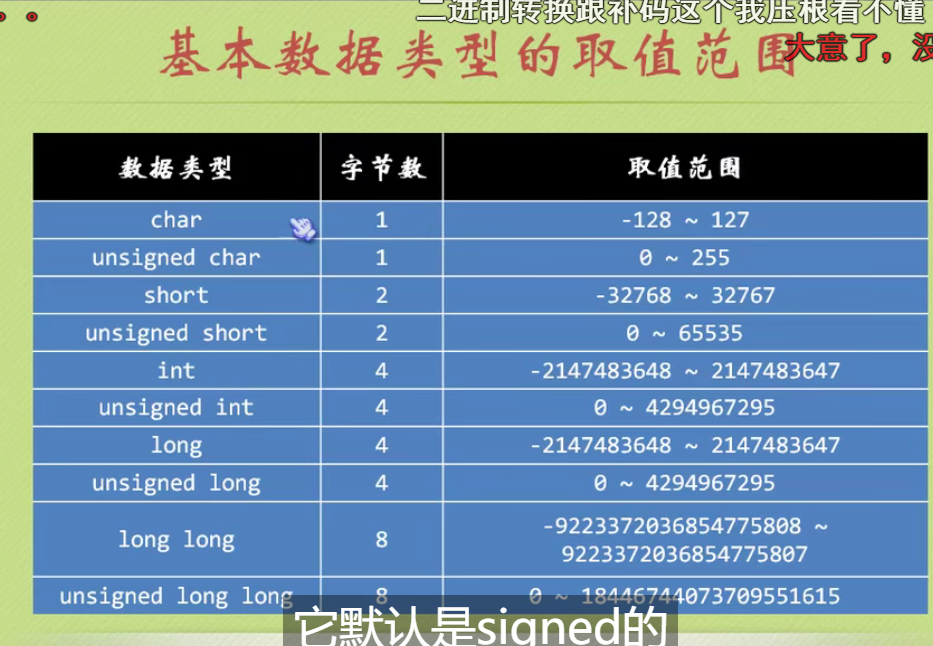
参考:[【C语言】《带你学C带你飞》\_哔哩哔哩\_bilibili](https://www.bilibili.com/video/BV17s411N78s?p=7)
## 基本数据类型
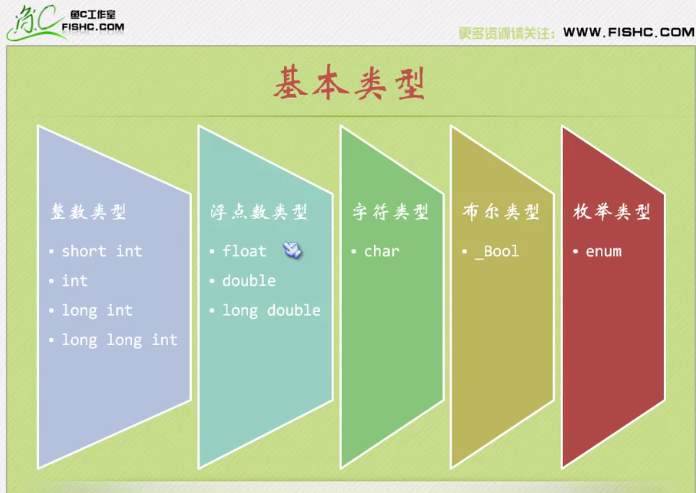
**数值类/字符数据类型长度**
|类型 | 长度 |
| ---|---|
|int |4|
|short int |2|
|long long int |8|
|char |1|
|_Bool |1|
|float |4|
|double |8|
|long double |16|
**signed 和 unsigned(无符号)**
- `signed` 带符号数字,可以存负数
- `unsigned` 不带符号数字,不允许负数;只能存在`0`和非负数,多出来的空间可以存更大的数, 比`signed`翻一倍(似乎?)
> 最佳实践:默认情况所有整形都是`signed`,另外`printf`时无符号数字需要选对格式字符
```
[signed] int age = -100; // 可以是负数
unsigned int age = 100; // 不带符号, 不能是负数,最小为0
```
### 数据类型强制转换
与php一样
```
int i = 1 + (int)1.8; // 强制把1.8转换整数,舍弃小数点
```
## 常用函数
### printf
格式化文本输出,全称`print format`
```
printf("%s出生于%d年%d三月", "余小波", 1997, 3);
> 余小波出生于1997年3月
```
**格式字符**
- `%s` 用于替代字符串
- `%c` 用于替代一个字符
- `%d` 用于替代整数
- `%f` 用于替代小数
[更多参考](https://www.runoob.com/cprogramming/c-function-printf.html)
### sizeof
用于计算一个变量/常量/数据类型的占用长度
```shell
int age = 24;
sizeof(age)
> 4
```
- 前言
- Android
- 签名证书生成
- JavaScript
- WebSocket 协议心跳
- 选择预览本地图片(不上传)
- Ajax 上传文件
- 代码片
- js 播放声音
- Verify 验证
- 验证金额 / 限制保留两位小数
- 原型链&继承
- 经典算法
- 冒泡排序
- 递归算法
- 二分查找
- 帮助函数
- 取URL查询参数为对象
- Date 日期时间
- 浅拷贝与深拷贝
- 进制转换
- Canvas
- 画圆形头像
- 计算字符串宽度
- 常用第三方包
- XLSX
- Layui
- layer.open select 遮挡问题
- 模板中使用 JS 表达式
- Form 表单
- on 监听事件
- select 下拉选择框
- 取表单数据 / 置表单数据
- form.verify 表单验证
- address 三级联动
- Table 表格
- 动态添加表格行
- 设置行高
- 单元格显示图片
- 开启编辑-阻止用户修改
- 主动触发事件
- 文件上传
- 单图上传
- Vue
- Vue.js 代码片
- 模态框/弹出层
- 单页面电影院选座
- vuex
- WebSocket聊天模板
- 解决办法
- vue-router多级路由中无父级组件设置方法
- Mui
- vue2+mui实现上拉刷新下拉加载
- JQuery
- 序列号表单为 JSON 对象
- Ajax 网络请求
- 选择器
- 插件
- selectpage
- HTML+CSS 布局
- Nodejs
- flex 布局
- 水平对齐方式
- 垂直对齐方式
- 九宫格/列表
- PHP
- gd 图像处理
- 图片写字
- 图片合并
- 图像压缩
- 图片写字居中
- 超全局变量
- helper 帮助函数
- ThinkPHP
- 生成用户分享二维码
- tp5.0 模型关联查询field无效
- Composer
- phpmailer
- 第三方接口
- 快递 100 物流记录
- 动态加载
- pdf解决方案
- 图片转换pdf
- 阿里云
- OSS 对象储存
- 阿里短信
- 辅助函数
- 取两个数组差异部分
- 从完整地址中取出省市区
- 钉钉
- 进制转换 & pack/unpack
- Yii2
- ActiveForm 常见问题
- FastAdmin
- layer的妙用
- \fast\Auth 权限
- 前端的各种常见问题
- 点击图片放大预览
- js
- ajax请求
- 前端
- Table
- selectPage
- 后端
- 腾讯cos插件后台上传文件工具类
- XDebug
- php.ini
- Linux
- RabbitMQ
- 防火墙
- iptables
- firewalld
- 环境变量
- shell
- bash
- crontab 定时任务
- 数据归档与解压缩
- tar
- 图像处理
- imagemagick
- 文档表格处理
- 转换word、pdf等
- 常用命令
- 文件资源管理
- xxd 十六进制编辑与查看
- 疑难杂症
- 开启swap虚拟文件系统缓解内存紧张卡顿
- wine
- deepin-wine(qq/微信综合)
- Python
- 代码片
- bs4 解析 html 插入到数据库
- webdriver 操作浏览器
- requests 请求库
- easyxlsx 导出 excel
- opencv
- 提取印章
- orc图片文字识别
- tesseract
- 进制转换
- WebSocket
- ws 服务示例
- 进程守护
- PM2
- Golang
- net/http
- Server
- 基本
- array 数组
- 循环
- os 文件目录基本操作
- map
- json处理
- Qrcode 二维码
- go-sqlite3
- go-redis
- mysql
- go-sql-driver
- 正则表达式
- beego
- fmt
- io
- ioutil
- net
- http
- Docker
- docker run
- Nginx
- CORS 允许跨域配置
- 负载均衡&反向代理
- rewaite 重写
- nginx配置
- 易语言
- 汇编 call 执行子程序
- HpSocketTcp 大文件传输
- 类指针
- Vim
- UniApp
- request.js
- 微信小程序登录处理(oauth2)
- vuex
- WebSocket聊天
- 腾讯云-人脸核身
- Java
- Spring Boot
- 请求与响应
- nginx部署
- 配置文件
- 创建项目
- 控制器
- 一个控制器示例
- 数据库
- MySQL
- 集成 SLF4J
- 集成 PageHelper 分页
- maven
- Markdown
- mermaid
- ffmpeg
- 基本使用
- 介绍/安装
- 简单示例
- php
- python
- office
- excel
- 单元格选择数据区域
- 截取字符串
- vlookup 选择值
- iferror 错误默认值
- search 搜索
- weekday 取星期几
- left 取左边n个字符
- right 取右边n个字符
- sum 合计
- sumif 条件合计
- sumifs 多条件合计
- datedif 取时间间隔
- roundup 向上舍入数字
- rounddown 向下舍入数字
- large 取第n大值
- mod 求余函数
- word
- 邮件合并-保留两位小数
- MySql
- 安装
- 基础操作
- 备份还原数据库
- 创建、删除、修改数据表
- 表结构查询
- Docker方案
- 慢查询
- 全文搜索
- 备份与还原
- 备份数据表
- 支付
- 支付宝
- ssh证书生成
- 支付申请
- 成考
- 英语
- 音标-掐头法
- 音标-去尾法
- 音标-元音
- 对比法学习辅音发音
- 短元音-长元音
- 双元音
- 数学
- 1.实数系
- 2.乘方
- 3.代数式
- 4.单项式多项式
- 5.整式的概念与方程综合
- 6.合并同类项和移项
- 7.二次根式
- 8.方程
- Git
- WebHook
- 宝塔
- webhook工具实现
- commit 规范
- 安装
- 创建
- 发布、提交
- 查看
- 更新
- 分支
- 找回文件、目录
- Svn
- 设置默认编辑器
- ignore 忽略提交目录
- revert 恢复撤销
- C语言
- 环境安装
- 基础部分
- 单片机
- ESP8266
- ESP8266开发环境安装
- 基础知识
- sim800
- Windows
- Win7
- Win10
- wsl (Linux子系统)
- ArtTemplate
- 正则表达式
- 常用正则
- VsCode
- easy less
- 腾讯云
- 数据万象
- 任务接口
- 音视频转码任务
- 算法
- 宽高按比例缩放大小尺寸
- IOS
- 开发者账号
- uniapp-ios打包上架的坑
- 协议模板
- 隐私协议
- 酸酸乳
- bower
- SQL Server
- 多行数据连接字符串
- GROUP BY选取其他字段
- 支付宝
- IoT小程序
- 支付
- RSA秘钥生成