## :-: [Vue CLI3.0 中使用jQuery 和 Bootstrap - 简书](https://www.jianshu.com/p/0d0c1eaeb877)
## :-: vue - router Demo
:-: 
:-: main.js
```
import Vue from 'vue'
import App from './App.vue';
import router from './router.js';
import './plugins/axios';
// vue add vuex -- 数据仓库
//在这里引入 bootstrap。默认只引入 bootstrap 中的 js,css 需要另外引入,我的 bootstrap.ss 在APP.vue中引入的
import "bootstrap";
//也可以在这里引入 bootstrap.css ;
import "bootstrap/dist/css/bootstrap.min.css";
import x2js from 'x2js' //xml数据处理插件
import store from './store'
Vue.prototype.$x2js = new x2js() //创建x2js对象,挂到vue原型上
Vue.config.productionTip = false;
// 全局守卫 (main.js)
// 进页面前触发
router.beforeEach((to, from, next) => {
// if (['student', 'academic'].includes(to.name)) return;
// some every
let needLogin = to.matched.some(ele => ele.meta && ele.meta.login === true);
if (needLogin) {
// 判断是否需要登陆
let isLogin = document.cookie.includes('login=true');
if (isLogin) {
next();
} else {
isLogin = window.confirm('该页面需要登陆后才能访问 ~');
if (isLogin) {
next('/login');
}
// 需要登陆
}
} else {
next();
}
});
// // 解析完毕执行
// router.beforeResolve((to, from, next) => {
// // to and from are both route objects. must call `next`.
// // next();
// })
new Vue({
router,
store,
render: h => h(App)
}).$mount('#app');
```
:-: router.js
```
import Vue from 'vue';
import Router from 'vue-router';
import Home from './views/Home';
// Vue.use(Router); -- 使路由插件生效
// $router -- 路由方法 $route -- 路由属性
Vue.use(Router);
export default new Router({
// mode -- 切换路由的模式(hash、history)
// mode: 'hash',
mode: 'history',
// linkExactActiveClass -- 修改路由按钮被选中所添加的class值、
// linkExactActiveClass: 'abc',
routes: [
// {
// path: '/',
// // redirect -- 重定向
// redirect: '/home',
// },
{
// path: '*' -- 解释:当URL路径没有被匹配上的时候才会执行该方法、
path: '*',
// redirect -- 重定向(string,object)
redirect(to) {
// 路径优化
// console.log(to);
if (to.path === '/') {
return '/home';
} else {
return '/error';
}
// 跳转到指定路径 push、replace
// this.$router.push('/home'); // 添加 [a, b, c] => [a, b, c, d]
// this.$router.replace('/home'); // 完全替换 [a, b, c] => [a, b, d]
// go -- 刷新(0)、前进(1)、后退(-1)
// this.$router.go( -2 ); // 后退2步
}
}, {
path: '/home',
name: 'home',
component: Home,
// 路由独享守卫
// beforeEnter(to, from, next) {
// // next();
// }
},
{
path: '/community',
name: 'community',
component: () =>
import ('./views/Community'),
// redirect -- 重定向
redirect: '/community/academic',
// 路由元信息
meta: {
login: true
},
// 二级路由
children: [{
// path: '/community/academic' 可以省略成 path: 'academic'
path: 'academic',
name: 'academic',
component: () =>
import ('./views/Academic'),
}, {
path: 'download',
name: 'download',
component: () =>
import ('./views/Download'),
}, {
path: 'personal',
name: 'personal',
component: () =>
import ('./views/Personal'),
}]
}, {
path: '/learn',
name: 'learn',
// 分页懒加载
component: () =>
import ('./views/Learn'),
},
{
path: '/student',
name: 'student',
// 路由元信息
meta: {
login: true
},
component: () =>
import ('./views/Student')
},
{
path: '/about',
name: 'about',
component: () =>
import ('./views/About')
}, {
path: '/error',
name: 'error',
component: () =>
import ('./views/Error')
}, {
// 动态路由
path: '/question/:id',
name: 'question',
component: () =>
import ('./views/Question')
}, {
path: '/login',
name: 'login',
component: () =>
import ('./views/Login')
}
]
});
```
:-: App.vue
```
<template>
<div id="app" class="container">
<nav class="navbar navbar-inverse">
<div class="container-fluid">
<!-- Brand and toggle get grouped for better mobile display -->
<div class="navbar-header">
<button
type="button"
class="navbar-toggle collapsed"
data-toggle="collapse"
data-target="#bs-example-navbar-collapse-1"
aria-expanded="false"
>
<span class="sr-only">Toggle navigation</span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
<router-link class="navbar-brand" to="/home">Brand</router-link>
</div>
<!-- Collect the nav links, forms, and other content for toggling -->
<div class="collapse navbar-collapse" id="bs-example-navbar-collapse-1">
<ul class="nav navbar-nav navbar-right">
<li>
<router-link to="/learn">课程学习</router-link>
</li>
<li>
<router-link to="/student">学员展示</router-link>
</li>
<li>
<router-link to="/about">关于</router-link>
</li>
<li>
<router-link to="/community">社区</router-link>
</li>
</ul>
</div>
<!-- /.navbar-collapse -->
</div>
<!-- /.container-fluid -->
</nav>
<!-- <router-view /> -- 切换路径时所展示的组件 -->
<router-view :jsonArr="jsonArr" />
</div>
</template>
<script>
export default {
data() {
return { jsonArr: [] };
},
mounted() {
//在页面加载完毕后初始化 tooltip, 相当于$(function(){ $('[data-toggle="tooltip"]').tooltip(); }
$('[data-toggle="tooltip"]').tooltip();
this.$axios
.get("https://api.bootcdn.cn/libraries.min.json")
.then(({ data }) => {
this.jsonArr = data || [];
});
}
};
</script>
<style lang="less">
#app .router-link-active {
color: #fff;
}
</style>
```
:-: views (页面组件列表)
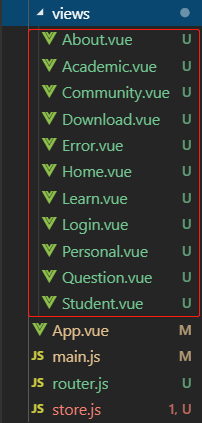
:-: Community.vue (二级路由)
```
<template>
<div class="community">
<!-- html -->
<ul id="nav">
<router-link tag="li" to="/community/academic">学术讨论</router-link>
<router-link tag="li" :to="{path:'/community/download'}">资源下载</router-link>
<router-link tag="li" :to="{name:'personal'}">个人中心</router-link>
</ul>
<router-view :jsonArr="jsonArr"></router-view>
</div>
</template>
<script>
export default {
props: ["jsonArr"]
};
</script>
<style lang="less">
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
#nav {
margin: 0;
cursor: default;
li {
background: #55a0c3;
display: inline-block;
line-height: 35px;
padding: 0 20px;
color: #fff;
cursor: pointer;
}
.router-link-active {
background: #6ba7c3;
}
}
</style>
```
- 前端工具库
- HTML
- CSS
- 实用样式
- JavaScript
- 模拟运动
- 深入数组扩展
- JavaScript_补充
- jQuery
- 自定义插件
- 网络 · 后端请求
- css3.0 - 2019-2-28
- 选择器
- 边界样式
- text 字体系列
- 盒子模型
- 动图效果
- 其他
- less - 用法
- scss - 用法 2019-9-26
- HTML5 - 2019-3-21
- canvas - 画布
- SVG - 矢量图
- 多媒体类
- H5 - 其他
- webpack - 自动化构建
- webpack - 起步
- webpack -- 环境配置
- gulp
- ES6 - 2019-4-21
- HTML5补充 - 2019-6-30
- 微信小程序 2019-7-8
- 全局配置
- 页面配置
- 组件生命周期
- 自定义组件 - 2019-7-14
- Git 基本操作 - 2019-7-16
- vue框架 - 2019-7-17
- 基本使用 - 2019-7-18
- 自定义功能 - 2019-7-20
- 自定义组件 - 2019-7-22
- 脚手架的使用 - 2019-7-25
- vue - 终端常用命令
- Vue Router - 路由 (基础)
- Vue Router - 路由 (高级)
- 路由插件配置 - 2019-7-29
- 路由 - 一个实例
- VUEX_数据仓库 - 2019-8-2
- Vue CLI 项目配置 - 2019-8-5
- 单元测试 - 2019-8-6
- 挂载全局组件 - 2019-11-14
- React框架
- React基本使用
- React - 组件化 2019-8-25
- React - 组件间交互 2019-8-26
- React - setState 2019-11-19
- React - slot 2019-11-19
- React - 生命周期 2019-8-26
- props属性校验 2019-11-26
- React - 路由 2019-8-28
- React - ref 2019-11-26
- React - Context 2019-11-27
- PureComponent - 性能优化 2019-11-27
- Render Props VS HOC 2019-11-27
- Portals - 插槽 2019-11-28
- React - Event 2019-11-29
- React - 渲染原理 2019-11-29
- Node.js
- 模块收纳
- dome
- nodejs - tsconfig.json
- TypeScript - 2020-3-5
- TypeScript - 基础 2020-3-6
- TypeScript - 进阶 2020-3-9
- Ordinary小助手
- uni-app
- 高德地图api
- mysql
- EVENTS
- 笔记
- 关于小程序工具方法封装
- Tool/basics
- Tool/web
- parsedUrl
- request