
## 依赖注入之-容器
设计架构图
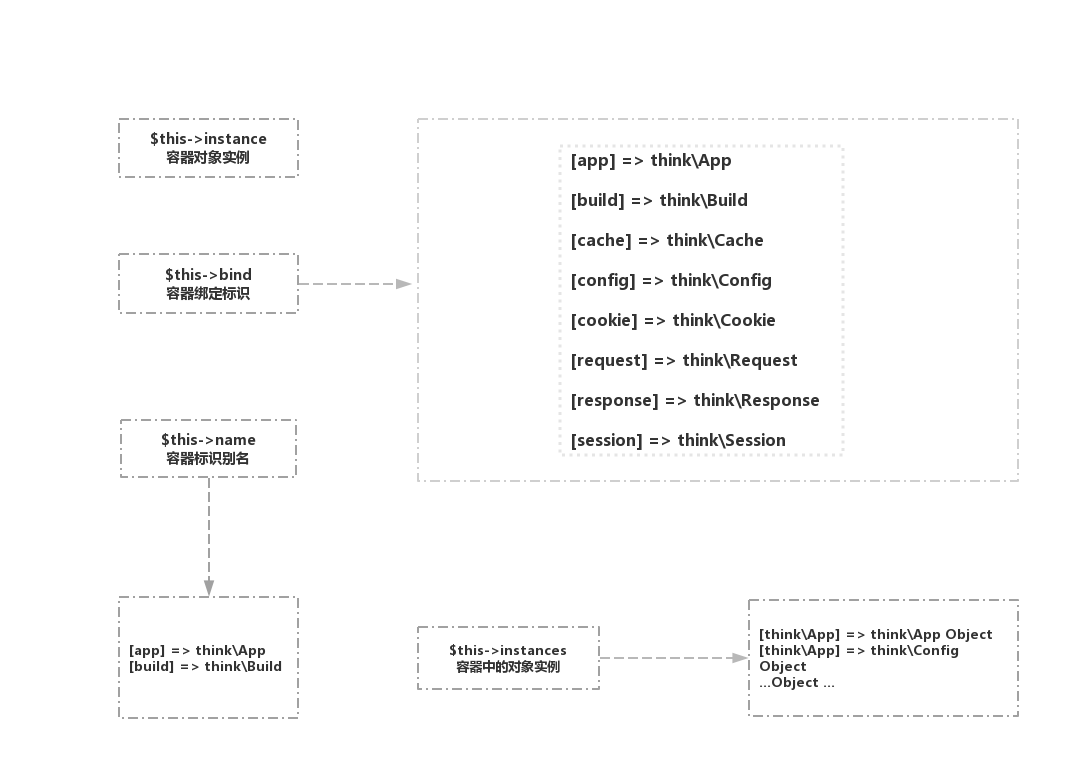
依赖注入(Dependency Injection,DI)容器就是一个对象,它知道怎样初始化并配置对象及其依赖的所有对象。
注册会用到一个依赖关系名称和一个依赖关系的定义。 依赖关系名称可以是一个类名,一个接口名或一个别名。
依赖关系的定义可以是一个类名,一个配置数组,或者一个 PHP 回调。
### 一、常见注入方式
#### 1、构造方法注入(Constructor Injection)
在参数类型提示的帮助下,DI 容器实现了构造方法注入。当容器被用于创建一个新对象时, 类型提示会告诉它要依赖什么类或接口。 容器会尝试获取它所依赖的类或接口的实例, 然后通过构造器将其注入新的对象。例如:
```
class Foo
{
public function __construct(Bar $bar)
{
}
}
$foo = $container->get('Foo');
// 上面的代码等价于:
$bar = new Bar;
$foo = new Foo($bar);
```
#### 2、方法注入(Method Injection)
通常,类的依赖关系传递给构造函数,并且在整个生命周期中都可以在类内部使用。 通过方法注入,可以提供仅由类的单个方法需要的依赖关系, 并将其传递给构造函数可能不可行,或者可能会在大多数用例中导致太多开销。
```php
class MyClass extends \yii\base\Component
{
public function __construct(/*Some lightweight dependencies here*/, $config = [])
{
// ...
}
public function doSomething($param1, \my\heavy\Dependency $something)
{
// do something with $something
}
}
```
#### 3、Setter 和属性注入(Setter and Property Injection)
Setter 和属性注入是通过[配置](https://www.yiichina.com/doc/guide/2.0/concept-configurations)提供支持的。 当注册一个依赖或创建一个新对象时,你可以提供一个配置, 该配置会提供给容器用于通过相应的 Setter 或属性注入依赖。 例如:
```
use yii\base\BaseObject;
class Foo extends BaseObject
{
public $bar;
private $_qux;
public function getQux()
{
return $this->_qux;
}
public function setQux(Qux $qux)
{
$this->_qux = $qux;
}
}
$container->get('Foo', [], [
'bar' => $container->get('Bar'),
'qux' => $container->get('Qux'),
]);
```
### 二、直接注入类到容器中
TP5.1 `绑定一个类到容器中(第一个参数直接传入类名)`
**1、使用容器**
```
// 绑定类库标识
Container::getInstance()->bindTo(GameService::class);
// 自动实例化
$obj = Container::get(GameService::class);
// 快速调用
halt($obj->test());
```
**2、使用助手函数**
```
// 绑定类库标识
bind(GameService::class);
// 快速调用(自动实例化)
$obj = app(GameService::class);
halt($obj);
```
Yii2.0 `注册一个同类名一样的依赖关系`
```
$container = new \yii\di\Container;
// 注册一个同类名一样的依赖关系,这个可以省略。
$container->set(GameService::class);
```
### 三、绑定类标识
TP5.1 `可以对已有的类库绑定一个标识(唯一),便于快速调用`
**1、使用容器**
```
// 绑定类库标识
Container::getInstance()->bindTo('game_service2',GameService::class);
// 快速调用(自动实例化)
$obj = Container::get('game_service2');
halt($obj);
```
**2、使用助手函数**
```
// 绑定类库标识
bind('game_service',GameService::class);
// 快速调用(自动实例化)
$obj = app('game_service');
// 带参数实例化调用
$obj = app('game_service',['file']);
halt($obj->test());
```
Yii2.0 `注册一个别名`
```
$container = new \yii\di\Container;
$container->set('foo', 'yii\db\Connection');
// 创建一个 Connection 实例
$obj = $container->get('foo');
```
### 四、绑定闭包
TP5.1 使用
```
// 使用助手函数
bind('sayHello', function ($name) {
return 'hello,' . $name;
});
echo app('sayHello',['thinkphp']);
// 使用容器
Container::getInstance()->bindTo('sayTinywan',function ($age){
return 'Tinywan is '.$age;
});
echo Container::get('sayTinywan',[24]);
```
Yii2.0 `注册一个 PHP 回调`
```
// 每次调用 $container->get('db') 时,回调函数都会被执行。
$container->set('db', function ($container, $params, $config) {
return new \yii\db\Connection($config);
});
```
### 四、类绑定到接口
```
// 绑定think\LoggerInterface接口实现到think\Log
bind('think\LoggerInterface','think\Log');
```
使用接口作为依赖注入的类型
```
<?php
namespace app\index\controller;
use think\LoggerInterface;
class Index
{
public function hello(LoggerInterface $log)
{
$log->record('hello,world!');
}
}
```
或者使用容器
```
// 绑定 app\driver\DriverInterface接口实现到 app\driver\YoungDriver
$driver = Container::getInstance()->bindTo(DriverInterface::class,YoungDriver::class);
$car = Container::get(Car::class, [$driver]);
var_dump($car->run());
```
## 依赖注入之-服务定位器
服务定位器是一个了解如何提供各种应用所需的服务(或组件)的对象。在服务定位器中, 每个组件都只有一个单独的实例,并通过ID 唯一地标识。 用这个 ID 就能从服务定位器中得到这个组件。
### TP5.1 使用
**1、配置文件`provider.php`**
系统会自动批量绑定类库到容器中
```
// 应用容器绑定定义
return [
'game_player' => \app\common\repositories\player\Game::class,
'random' => \app\common\repositories\game\RandomGroup::class
];
```
**2、那类玩家在玩那个游戏**
```
// 声明那类玩家
Container::getInstance()->bindTo(PlayerInterface::class,TeacherPlayer::class);
// 要玩那个游戏
$game = app('random');
// 获取玩家实例
$player = app('game_player');
// 玩家开始玩什么游戏
halt($player->play($game));
```
**3、携带参数**
```
// 声明那类玩家
Container::getInstance()->bindTo(PlayerInterface::class, StudentPlayer::class);
// 要玩那个游戏
$game = app('random');
// 获取玩家实例
$parms = ['caode' => 200, 'name' => 'wangwang'];
$player = app('game_player',['ddd']);
// 玩家开始玩什么游戏
halt($player->play($game,$parms));
```
- 序言
- 专题一 PHP基础教程
- 1、empty、isset、is_null的用法
- 2、线程安全与非线程
- 3、大文件上传需要修改的配置
- 10、魔术方法
- 4、编译安装PHP7
- 5、编译安装PHP7.4
- 6、PECL 安装 PHP 扩展库
- 专题二 PHP高级教程
- 1、类和对象
- 2、继承
- 3、魔术方法
- 4、抽象类
- 5、接口
- 6、反射机制实现自动依赖注入
- 7、服务容器与依赖注入的思想
- 8、并发解决方案之opcache
- 9、Composer自动加载原理
- 1、安装与使用
- 10、抽象类和接口的区别
- 11、self和static的区别
- 12、PHP7 变量
- 13、PHP8.3 错误 Error 和 异常 Exception 树列表
- 专题三 ThinkPHP6专题
- 1、DI容器
- 2、AUTH权限认证
- 3、Nginx URI重写方式
- 4、并发锁问题
- 5、自定义全局异常
- 6、CLI 模式跨模块查询数据库
- 7、数据库优化方案
- 附录一 常见错误
- 附录二 自定义分页类
- 附录三 cropper.js图片上传和裁剪
- 附录四 数据库和模型源码解读
- 1、Db类
- 2、查询构造器
- 3、模型
- 附录五 权限认证Auth类
- 附录六 ThinkPHP5.1 源码分析
- (一)类的自动加载
- (二)配置文件Config类
- (三)容器Container类
- (四)门面Facade
- (五)框架执行流程
- (六)路由解析
- 附录七 官方扩展
- 1、think-queue 队列
- 附录八 易错整理
- 附录九 问题列表
- 附录十 任务队列异步通过视图导出PDF
- 专题四 Docker教程
- 1、Docker安装
- 2、如何在本地构建镜像
- 3、镜像、容器以及命令操作
- 4、容器进入的4种方式
- 5、Dockerfile常用指令详解
- 6、发布自己的镜像
- 7、数据卷管理
- 8、docker-compose概念
- 9、docker-compose入门
- 10、如何构建docker-compose
- 11、Docker网络
- 12、搭建私有仓库
- 13、Docker部署方式
- 14、推送到Github仓库
- 附录一 PHPStrom 调试XDebug
- 附录二 安装RabbitMQ
- 附录 日常使用笔记
- 附录四 Docker 调试XDebug
- 专题五 Redis教程
- 1、编译安装
- 2、配置文件详解
- 3、Lua 脚本的应用和实践
- 4、Redis实现分布式锁(集群版)
- 5、Redis键空间通知
- 6、Redis5.0 搭建集群
- (1)、创建和使用Redis群集
- (2)、新增节点
- 7、限流器的实现
- 8、Redis5.0 新特性
- 8.1、注意要点
- 8.2 xreadgroup 命令
- 9、延迟任务队列
- 10 Stream 消息队列
- 11、基于 Redis 的 Stream 类型的完美消息队列解决方案
- 12、Streams 实现延迟消息队列
- 13、Stream流三种ACK机制
- 14、read error on connection排查
- 附录一 常见问题
- 附录二 Redis面试大全
- 附录三 有序集合使用场景
- 附录四 Lua脚本调试
- 附录五 高性能、高可扩展关键技术
- 专题六 MySQL教程
- 1-1、二进制安装
- 1-2、安装包安装(推荐)
- 2、索引、锁、事务
- 3、字符集
- 4、导出导入数据
- 5、5.7版本兼容性
- 6、数据库自动备份
- 7、如何重置MySQL 5.7 root密码
- 8、MySQL自动完成和语法突出
- 9、普通索引和唯一索引的区别
- 10、深入了解行锁、表锁、索引
- 11、索引数据结构
- 12、MySQL规范
- 13、开发高频面试题精选(重要)
- 14、锁专题
- 1、可重复读(REPEATABLE_READ)
- 2、事务隔离级别概述
- 15、MySQL外键约束
- 16、left join 查询
- 17、 MySQL设计三范式和反范式
- 18、性能分析-Profiling
- 19、查询好慢,除了索引,还能因为什么?(重要)
- 20、常用日期字段
- MYSQL 5.7 VARCHAR 类型详解
- 专题七 Nginx教程
- 1、什么是Nginx?
- 2、编译安装
- 3、日志配置和模块讲解
- 4、静态资源和缓存服务
- 5、正向和反向服务
- 6、Rewrite规则
- 7、HTTP负载均衡(七层)
- 8、TCP负载均衡(四层)
- 9、如何配置HTTPS服务
- 10、Nginx的负载均衡算法
- 11、如何配置http和https同时访问
- 12、灰度发布
- 13、常见负载均衡算法
- 14、Openresty 专题
- 15、如何改进 NGINX 配置文件节省带宽?
- 16、谈谈基于 OpenResty 的接口网关设计
- 附录一 阿里云负载均衡配置
- 附录二、基础配置文件
- nginx.conf
- 附录三、Nginx+lua+Memcache 实现灰度发布
- 附录四 视频监控RTSP转HLS解决方案
- 附录五 Openresty 编译
- 附录六 Vod模块
- 1、本地模式
- 2、映射模式
- 专题八 Git版本管理
- 1、Git 基础知识
- 2、团队分支模型
- 3、储藏与清理
- 4、如何同步Fork
- 5、多Git账户id_rsa私钥
- 6、高效规范使用Git
- 7、远程分支的创建
- 8、GitFlow工作流
- 9、Git撤销&回滚操作(git reset 和 get revert)
- 10、合并时 --no-ff 的作用
- 11、 删除本地、远程、缓存分支
- 13、Git和Windows的大小写不敏感产生的问题
- 附录一 、一次记录
- 附录二、常用工作流程
- 附录三、每次更新代码都要输入用户名密码
- 附录四、OEM版本控制
- 附录五 常用记录
- 1、查看某一个文件修改的具体内容
- 2、强制推送到远程分支
- 3、生产环境代码回滚
- 附录六 三年 Git 使用心得 & 常见问题整理
- 附录七 Git 忽略文件,不提交文件 清空缓存
- 12/找回历史删除分支
- 专题九 WorkerMan服务
- 3、SocketIO消息推送
- 4、master和worker模型
- 5、GatewayWorker
- 6、使用systemd管理workerman
- 7、TCP长连接应用GatewayWorker心跳检测
- 附录一 运行问题
- 附录二 问题与解决方法
- 专题十 MQ消息中间件
- 1、为什么要使用消息队列
- 2、RabbitMQ
- 『1』AMQP核心概念
- 『2』交换机模式讲解
- 『3』RabbitMQ高级特性
- (1)hello
- 3、NSQ
- 4、RabbitMQ延迟队列
- 附件一 RabbitMQ 注意要点
- 5、RocketMQ PHP 生产端和消费端代码优雅实现
- 专题十一 PHP函数整理
- 1、系统函数
- 2、自定义函数
- 3、回调函数
- 4、匿名函数
- 5、递归函数
- 6、常用函数库
- 7、call_user_func函数
- 8、preg_replace_callback函数
- 专题十二 常用设计模式
- 1、创建型模式
- (1)单例模式
- (2)工厂模式
- (3)抽象工厂模式
- (4)建造者模式(Builder)
- (5)原型模式(Prototype)
- 2、结构型模式
- (1)适配器模式(Adapter)
- (2)桥接模式(Bridge)
- (3)合成模式(Composite)
- (4)装饰器模式(Decorator)
- (5)代理模式(Proxy)
- (6)享元模式(Flyweight)
- 3、行为型模式
- 2、策略模式( Strategy)
- 4、六大原则
- 1、依赖注入
- 5、其他
- 6、Presenter模式
- 4、Service 模式
- 5、Repository模式
- 外观设计模式示例
- 专题十三 实时通信
- 1、pusher 入门教程
- 2、pusher 演示与频道实时通信
- 3、pusher 如何使用私有频道
- 4、pusher 实时图表展示
- 11、webman插件push入门教程
- 12、webman插件push如何使用私有频道
- 13、webman插件push私有频道客户端推送
- 14、webman插件push的webhooks
- 15、webman插件push的实时动态图表
- 专题十四 PHP异常处理
- 1、Exception 类
- 2、如何自定义异常?
- 3、处理PHP重错误
- 4、自定义错误处理器
- 专题十五 Shell脚本案例
- 1、crontab任务脚本无法执行问题
- 专题十六 Jenkins自动化部署
- 1、Jenkins安装
- 2、Pipeline插件
- 3、BlueOcean
- 4、OPENSSH PRIVATE KEY转换为RSA PRIVATE KEY
- 专题十七 常用工具整理
- 1、证书在线打印
- 2、密码生成规则
- 3、vscode插件
- 专题十八 常用功能列表
- 1、frp 内网穿透工具
- (1)如何做成一个服务
- (2)代理Websocket服务
- (3)代理N个Web服务
- (4)设置为系统服务
- (5)Https 配置
- 附录一 常见问题排查
- 2、如何美化文档
- 3、如何提高访问github的速度?
- 4、Vultr搭建SS教程
- 5、PPH编译安装
- 6、Supervisor进程管理工具
- 7、Umeditor 上传文件阿里云和本地
- 8、scp 远程上传或下载 文件/文件夹
- 9、安装和使用守护进程Supervisor
- 10、人脸识别
- 专题十九 流媒体直播实战
- 1、什么是视频直播?
- 2、如何使用推流软件OBS?
- 3、基于Nginx 的RTMP模块搭建系统
- 4、直播流程
- 附录一 阿里云直播
- 5、典型业务场景
- 8、直播回调授权观看
- 9、视频直播源如何加密
- 10、如何实现视频在线云剪辑
- 11、视频点播以及加密技术实现
- 12、FFmpeg 入门教程
- 13、HLS 直播加密播放
- 14、nginx-vod-module 模块
- 15、车辆维修直播系统
- 『16』HLS-m3u8专题
- 附件一 FFmpeg 命令
- 附件二 阿里云点播
- 专题二十 微信
- 附录一 遇到的坑
- 专题二十一 支付专题
- 『1』支付宝支付
- 『2』微信支付
- 『3』支付宝直付通
- 「1」什么是直付通?
- 「2」二级商户进件
- 「3」统一交易收款
- 「4」资金结算
- 「5」分账
- 「6」支付接入流程
- 「?」问题列表
- 附录一 return_url和notify_url的区别
- 附录二 常见错误信息
- 附录三 电脑端支付案例
- 附录四 其他问题
- 1、购买了线上课程后不能退款 这样的现象合法吗?
- 专题二十二 Vue3笔记
- 1、开发环境搭建
- 专题二十三 开放API专题
- 1、错误码
- 2、OAuth2流的简单说明
- 『3』HTTP API 身份验证和授权
- 专题二十四 测试专题
- 1、并发测试
- 专题二十五 DevOps专题
- 『1』PHP代码质量实战
- 专题二十六 前后端分离
- 『1』前后端分离介绍
- 『2』控制权限管理
- 专题二十七 微服务专题
- 1、服务发现 Nacos
- 1.1 服务发现
- 专题二十八 Casbin权限专题
- 1、设置超级管理员的三种方法
- 2、多租户权限和基本设置
- 3、casbin简化策略数据
- 4、多个RBAC
- 5、身份验证和基于角色的RBAC授权
- 6、Casbin在RESTful及中间件使用
- 7、Casbin 中 ABAC 的使用方法
- 8、Model语法和策略存储
- 9、Casbin的Model和Policy
- 10、RBAC的RESTful完全匹配访问模型
- 11、自定义函数使用
- 12、webman中使用
- 13、分布式服务中如何使用Watcher
- 14、Casbin 项目实战ABAC模型策略
- 附录一 源码解读
- 附录二 常见问题
- 专题二十九 PHP 常见错误处理
- 专题三十 ELK日志系统
- 1、docker-elk
- 专题三十一 Swoole专题
- 1、ThinkPHP6中RPC服务
- 专题三十二 Webman框架
- 1、自定义进程执行异步任务
- 2、实现WebRTC信令服务器
- 3、实现一个RPC服务
- 4、对象和资源的持久化
- 5、ThinkORM持久化连接
- 6、ThinkORM悲观锁解决商品超卖问题的实现
- 7、monolog日志神器
- 附录一 为什么?
- 附录二 编码规范
- 附录一 游戏
- 1、初级程序员常犯的错误
- 附录三 设计模式
- 1、单例模式
- 2、工厂方法模式
- 3、抽象工厂模式
- 4、装饰器模式
- 附录四 Docker安装SqlServer
- 专题二十一 Layui
- 我的技术栈【重要】
- 附录四 Linux 日常运维
- 1、sudo 权限
- 2、用户和用户组管理
- 3、grep 多条件查询
- 其他系列
- 1、fnm:基于Rust开发的高效Node版本管理工
- 样式
- Mall商城
- 1、系统架构
- 1.1 mall整合ThinkPHP+ThinkORM搭建基本骨架
- 1.2 mall整合Elasticsearch实现商品搜索
- 1.3 mall整合OSS实现文件上传
- 2、业务篇