`sudo cnpm i typescript -g` 安装
`tsc --version` 查看版本
`tsc --init `创建一个tsconfig文件
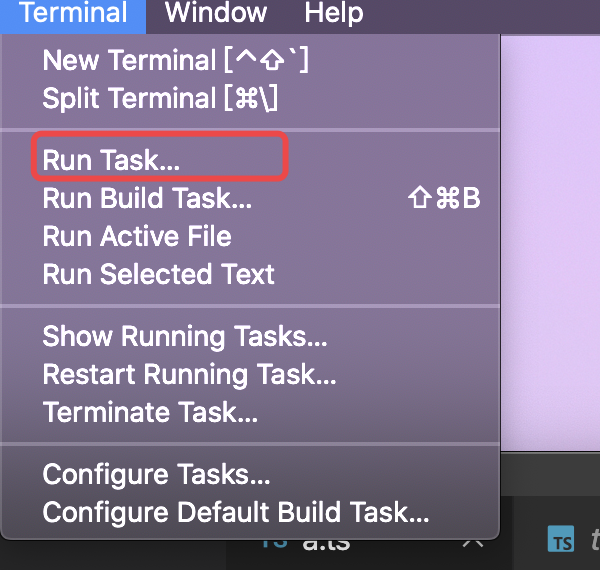
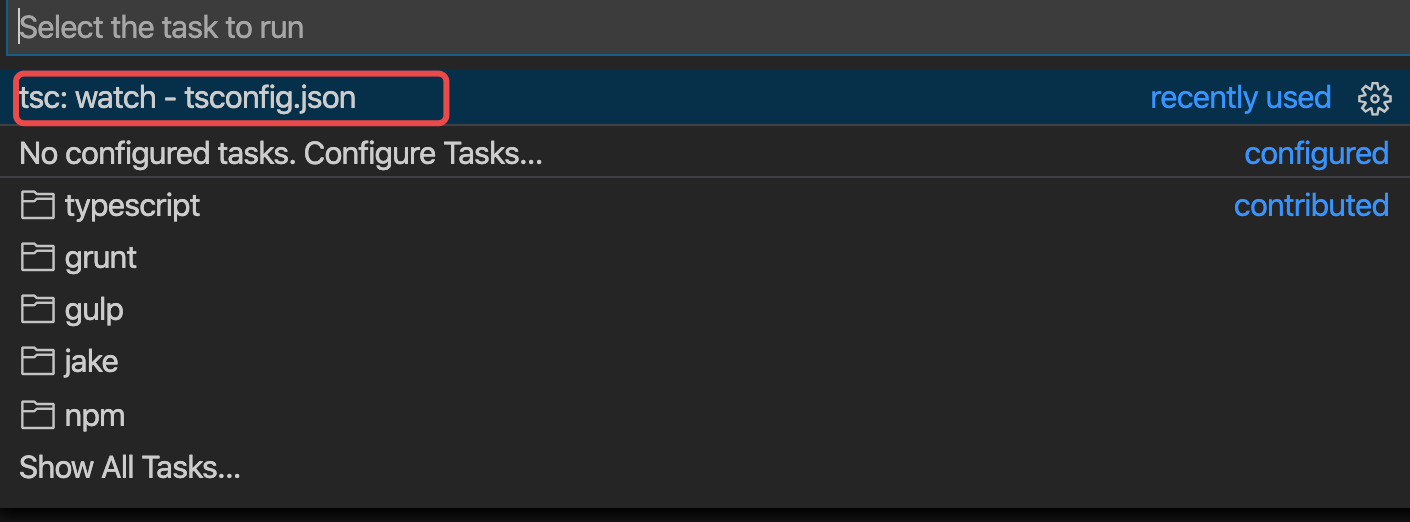
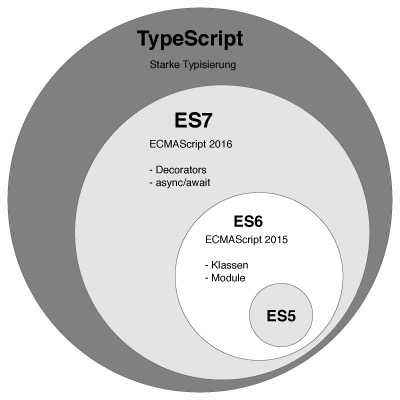
~~~
//Cannot redeclare block-scoped variable 'name'.
//如果代码里有export import 之类的代码,那么这个文件变成一个模块
//export { }
//在一个作用域 内部,相同的变量名只能声明一次
export { }
let name: string = 'zhufeng';
let age: number = 10;
let married: boolean = true;
let hobbies: string[] = ['1', '2', '3'];
let interests: Array<string> = ['4', '5', '6'];
//元组 类似一个数组 它是一个长度和类型都固定的数组
//1 长度固定 2 类型可以不一样
let point: [number, number] = [100, 100];
point[0], point[1];
let person: [string, number] = ['zhufeng', 10];
enum Gender {
BOY,
GIRL
}
console.log(`李雷是${Gender.BOY}`);
console.log(`MM是${Gender.GIRL}`);
/* var Gender2;
(function (Gender) {
Gender2[Gender2["BOY"] = 0] = "BOY";
Gender2[Gender2["GIRL"] = 1] = "GIRL";
})(Gender2 || (Gender2 = {}));
console.log("\u674E\u96F7\u662F" + Gender2.BOY);
console.log("MM\u662F" + Gender2.GIRL); */
let Gender2 = {
0: "BOY",
1: "GIRL",
"BOY": 0,
"GIRL": 1
}
let s = Symbol.for('xx');
//数字 字符串 它的值可能会写
let person2 = {
gender: Gender.BOY
}
enum Week {
MONDAY = 1,
TUESDAY = 'y'
}
console.log(Week.TUESDAY);
//常数枚举
const enum Colors {
Red,
Yellow,
Blue
}
console.log(Colors.Red, Colors.Yellow, Colors.Blue);
//任意类型 anyscript
// 第三库没有类型定义 类型转换的时候 数据结构太复杂太灵活 any
//ts 为 dom提供了一整套的类型声明
/* let root: HTMLElement | null = document.getElementById('root');
root!.style.color = 'red';//!断言不为空
*/
//null undefined
// null 空 undefined 未定义
//它们都其它类型的子类型 你可以把它们赋给其它类型的变量
let myname1: string | null = null;
let myname2: string | undefined = undefined;
let x: number | null | undefined;
x = 1;
x = undefined;
x = null;
// void类型 空的 没有
function greeting(name: string): null | void {
console.log(`hello ${name}`);
return null;
}
greeting('zhufeng');
//never 永远不
//never是其它类型的子类型,代表不会出现的值
//A function returning 'never' cannot have a reachable end point
//在函数内部永远会抛出错误,导致函数无法正常结束
function createError(message: string): never {
console.log(1);
throw new Error('error');
console.log('end point');
}
function sum(): never {
while (true) {
console.log('hello');
}
console.log('end point');
}
//| 和 || ,& 和 && 的区别
let num1 = 3 | 5;
console.log(num1);
let num2 = 3 || 5;
console.log(num2);
let num3 = 3 & 5;
console.log(num3);//1
let num4 = 3 && 5;
console.log(num4);//5
let num: string | number
//推论 猜
let name2 = 2;
name2 = 3;
name2 = 4;
let name3;
name3 = 4;
name3 = 'zhufeng';
//包装对象 java装箱和拆箱 c#
//自动在基本类型的对象类型之间切换
//1.基本类型上没有方法
//2.在内部迅速的完成一个装箱的操作,把基本类型迅速包装成对象类型,然后用对象来调用方法
let name4: string = 'zhufeng';
//name4.toLocaleLowerCase();
let name44 = new String(name4);
name44.toLocaleLowerCase();
//let isOk1: boolean = true;
//let isOk2: boolean = Boolean('xx');
//let isOk3: boolean = new Boolean(1);
let name5: string | number;
name5 = 'zhufeng';
name5.toLowerCase();
name5 = 5;
name5.toFixed(3);
/* let name7: string | number = 1;
let name6: string | number = name7;
(name6 as string).toLowerCase();
(name6 as number).toFixed(2);
*/
//字面量类型
let Gender4: 'Boy' | 'GIRL';
Gender4 = 'Boy';
Gender4 = 'GIRL';
~~~
## 3\. 数据类型
### 3.1 布尔类型(boolean)
~~~
let married: boolean=false;
~~~
### 3.2 数字类型(number)
~~~
let age: number=10;
~~~
### 3.3 字符串类型(string)
~~~
let firstname: string='zfpx';
~~~
### 3.4 数组类型(array)
~~~
let arr2: number[]=[4,5,6];
let arr3: Array<number>=[7,8,9];
~~~
### 3.5 元组类型(tuple)
* 在 TypeScript 的基础类型中,元组( Tuple )表示一个已知`数量`和`类型`的数组
~~~
let zhufeng:[string,number] = ['zhufeng',5];
zhufeng[0].length;
zhufeng[1].toFixed(2);
~~~
| 元组 | 数组 |
| --- | --- |
| 每一项可以是不同的类型 | 每一项都是同一种类型 |
| 有预定义的长度 | 没有长度限制 |
| 用于表示一个结构 | 用于表示一个列表 |
~~~
const animal:[string,number,boolean] = ['zhufeng',10,true];
~~~
### 3.6 枚举类型(enum)
* 事先考虑某一个变量的所有的可能的值,尽量用自然语言中的单词表示它的每一个值
* 比如性别、月份、星期、颜色、单位、学历
#### 3.6.1 普通枚举
~~~
enum Gender{
GIRL,
BOY
}
console.log(`李雷是${Gender.BOY}`);
console.log(`韩梅梅是${Gender.GIRL}`);
enum Week{
MONDAY=1,
TUESDAY=2
}
console.log(`今天是星期${Week.MONDAY}`);
~~~
#### 3.6.2 常数枚举
* 常数枚举与普通枚举的区别是,它会在编译阶段被删除,并且不能包含计算成员。
* 假如包含了计算成员,则会在编译阶段报错
~~~
const enum Colors {
Red,
Yellow,
Blue
}
let myColors = [Colors.Red, Colors.Yellow, Colors.Blue];
~~~
~~~
const enum Color {Red, Yellow, Blue = "blue".length};
~~~
### 3.7 任意类型(any)
* `any`就是可以赋值给任意类型
* 第三方库没有提供类型文件时可以使用`any`
* 类型转换遇到困难时
* 数据结构太复杂难以定义
~~~
let root:any=document.getElementById('root');
root.style.color='red';
~~~
### 3.8 null 和 undefined
* null 和 undefined 是其它类型的子类型,可以赋值给其它类型,如数字类型,此时,赋值后的类型会变成 null 或 undefined
* strictNullChecks
~~~
let x: number;
x = 1;
x = undefined;
x = null;
let y: number | null | undefined;
y = 1;
y = undefined;
y = null;
~~~
### 3.9 void 类型
* void 表示没有任何类型
* 当一个函数没有返回值时,TS 会认为它的返回值是 void 类型。
* 当我们声明一个变量类型是 void 的时候,它的非严格模式下仅可以被赋值为 null 和 undefined;
~~~
function greeting(name:string):void {
console.log('hello',name);
}
greeting('zfpx');
~~~
### 3.10 never类型
never是其它类型(null undefined)的子类型,代表不会出现的值
#### 3.10.1
* 作为不会返回( return )的函数的返回值类型
~~~
// 返回never的函数 必须存在 无法达到( unreachable ) 的终点
function error(message: string): never {
throw new Error(message);
}
// 由类型推论得到返回值为 never
function fail() {
return error("Something failed");
}
// 返回never的函数 必须存在 无法达到( unreachable ) 的终点
function infiniteLoop(): never {
while (true) {}
}
~~~
#### 3.10.2 strictNullChecks
* 在 TS 中, null 和 undefined 是任何类型的有效值,所以无法正确地检测它们是否被错误地使用。于是 TS 引入了 --strictNullChecks 这一种检查模式
* 由于引入了 --strictNullChecks ,在这一模式下,null 和 undefined 能被检测到。所以 TS 需要一种新的底部类型( bottom type )。所以就引入了 never。
~~~
// Compiled with --strictNullChecks
function fn(x: number | string) {
if (typeof x === 'number') {
// x: number 类型
} else if (typeof x === 'string') {
// x: string 类型
} else {
// x: never 类型
// --strictNullChecks 模式下,这里的代码将不会被执行,x 无法被观察
}
}
~~~
#### 3.10.3 never 和 void 的区别
* void 可以被赋值为 null 和 undefined的类型。 never 则是一个不包含值的类型。
* 拥有 void 返回值类型的函数能正常运行。拥有 never 返回值类型的函数无法正常返回,无法终止,或会抛出异常。
### 3.11 类型推论
* 是指编程语言中能够自动推导出值的类型的能力,它是一些强静态类型语言中出现的特性
* 定义时未赋值就会推论成any类型
* 如果定义的时候就赋值就能利用到类型推论
~~~
let username2;
username2 = 10;
username2 = 'zhufeng';
username2 = null;
~~~
### 3.12 包装对象(Wrapper Object)
* JavaScript 的类型分为两种:原始数据类型(Primitive data types)和对象类型(Object types)。
* 所有的原始数据类型都没有属性(property)
* 原始数据类型
* 布尔值
* 数值
* 字符串
* null
* undefined
* Symbol
~~~
let name = 'zhufeng';
console.log(name.toUpperCase());
console.log((new String('zhufeng')).toUpperCase());
~~~
* 当调用基本数据类型方法的时候,JavaScript 会在原始数据类型和对象类型之间做一个迅速的强制性切换
~~~
let isOK: boolean = true; // 编译通过
let isOK: boolean = Boolean(1) // 编译通过
let isOK: boolean = new Boolean(1); // 编译失败 期望的 isOK 是一个原始数据类型
~~~
### 3.13 联合类型
* 联合类型上只能访问两个类型共有的属性和方法
~~~
let name4: string | number;
name4 = 3;
name4 = 'zhufeng';
console.log(name4.toUpperCase());
~~~
### 3.14 类型断言
* 类型断言可以将一个联合类型的变量,指定为一个更加具体的类型
* 不能将联合类型断言为不存在的类型
~~~
let name5: string | number;
(name5 as number).toFixed(3);
(name5 as string).length;
(name5 as boolean);
~~~
### 3.15 字符串、数字、布尔值字面量
~~~
type Lucky = 1 | 'One'|true;
let foo:Lucky = 'One';
~~~
### 3.16 字符串字面量 vs 联合类型
* 字符串字面量类型用来约束取值只能是某`几个字符串`中的一个, 联合类型(Union Types)表示取值可以为`多种类型`中的一种
* 字符串字面量 限定了使用该字面量的地方仅接受特定的值,联合类型 对于值并没有限定,仅仅限定值的类型需要保持一致
- 文档简介
- 基础面试题【珠峰2019.8】
- P01_call,aplly区别
- P02_综合面试题讲解2-2
- P03_箭头函数和普通函数区别-综合面试题讲解2-3
- P05_实现indexOf
- P06_综合面试题讲解2-6
- P07_URL解析题
- P08_原型题
- P09_图片延时加载
- P10_正则-包含数字字母下划线
- P11_综合面试题讲解2-11
- P12_英文字母加空格
- P13_数组扁平化并去重
- P14_模拟实现new
- P15_合并数组
- P16_定时器,打印012345
- P17_匿名函数输出值问题
- P18_a在什么情况下打印输出+1+1+1
- P19_对数组的理解
- P20_冒泡排序
- P21_插入排序
- P22_快速排序
- P23_销售额存在对象中
- P24_求数组的交集
- P25_旋转数组
- P26_ [函数柯理化思想]
- P27_ [柯理化函数的递归]
- 网络协议【珠峰2019.6】
- TypeScript+Axios入门+实战【珠峰2019.11】
- 1.数据结构
- 2.函数和继承
- 3.装饰器
- 4.抽象类-接口-泛型
- 05-结构类型系统和类型保护
- 06-类型变换
- AST-抽象语法树
- React性能优化【珠峰2019.10】
- 1-react性能优化
- 2-react性能优化
- 3.react-immutable
- React Hooks【珠峰2019.12】
- 前端框架及项目面试
- 第07章 React 使用
- 7-1 React使用-考点串讲
- 7-2 JSX基本知识点串讲
- 7-3 JSX如何判断条件和渲染列表
- 7-4 React事件为何bind this
- 7-5 React事件和DOM事件的区别
- 7-6 React表单知识点串讲
- 7-7 React父子组件通讯
- 7-8 setState为何使用不可变值
- 7-9 setState是同步还是异步
- 7-10 setState合适会合并state
- 7-11 React组件生命周期
- 7-12 React基本使用-知识点总结和复习
- 7-13 React函数组件和class组件有何区别
- 7-14 什么是React非受控组件
- 7-15 什么场景需要用React Portals
- 7-16 是否用过React Context
- 7-17 React如何异步加载组件
- 7-18 React性能优化-SCU的核心问题在哪里
- 7-19 React性能优化-SCU默认返回什么
- 7-20 React性能优化-SCU一定要配合不可变值
- 7-21 React性能优化-PureComponent和memo
- 7-22 React性能优化-了解immutable.js
- 7-23 什么是React高阶组件
- 7-24 什么是React Render Props
- 7-25 React高级特性考点总结
- 7-26 Redux考点串讲
- 7-27 描述Redux单项数据流
- 7-28 串讲react-redux知识点
- 7-29 Redux action如何处理异步
- 7-30 简述Redux中间件原理
- 7-31 串讲react-router知识点
- 7-32 React使用-考点总结
- 第08章 React 原理
- 8-1 React原理-考点串讲
- 8-2 再次回顾不可变值
- 8-3 vdom和diff是实现React的核心技术
- 8-4 JSX本质是什么
- 8-5 说一下React的合成事件机制
- 8-6 说一下React的batchUpdate机制
- 8-7 简述React事务机制
- 8-8 说一下React组件渲染和更新的过程
- 8-9 React-fiber如何优化性能
- 第09章 React 面试真题演练
- 9-1 React真题演练-1-组件之间如何通讯
- 9-2 React真题演练-2-ajax应该放在哪个生命周期
- 9-3 React真题演练-3-组件公共逻辑如何抽离
- 9-4 React真题演练-4-React常见性能优化方式
- 9-5 React真题演练-5-React和Vue的区别
- 第10章 webpack 和 babel
- 10-1 webpack考点梳理
- 10-2 webpack基本配置串讲(上)
- 10-3 webpack基本配置串讲(下)
- 10-4 webpack如何配置多入口
- 10-5 webpack如何抽离压缩css文件
- 10-6 webpack如何抽离公共代码和第三方代码
- 10-7 webpack如何实现异步加载JS
- 10-8 module chunk bundle 的区别
- 10-9 webpack优化构建速度-知识点串讲
- 10-11 happyPack是什么
- 10-12 webpack如何配置热更新
- 10-13 何时使用DllPlugin
- 10-14 webpack优化构建速度-考点总结和复习
- 10-15 webpack优化产出代码-考点串讲
- 10-16 什么是Tree-Shaking
- 10-17 ES Module 和 Commonjs 的区别
- 10-18 什么是Scope Hostin
- 10-19 babel基本概念串讲
- 10-20 babel-polyfill是什么
- 10-21 babel-polyfill如何按需引入
- 10-22 babel-runtime是什么
- 10-23 webpack考点总结和复习
- 10-24 webpack面试真题-前端代码为何要打包
- 10-25 webpack面试真题-为何Proxy不能被Polyfill
- 10-26 webpack面试真题-常见性能优化方法