### 运行环境
* PHP 7.0+ (v2.8.0 开始 >= 7.1.3)
* composer
> php5 请使用 v1.x 版本[https://github.com/yansongda/pay/tree/v1.x](https://github.com/yansongda/pay/tree/v1.x)
详情 请查看 https://github.com/yansongda/pay/tree/v2
### 1、支付宝
* 电脑支付
* 手机网站支付
* APP 支付
* 刷卡支付
* 扫码支付
* 账户转账
* 小程序支付
| method | 描述 |
| :-: | :-: |
| web | 电脑支付 |
| wap | 手机网站支付 |
| app | APP 支付 |
| pos | 刷卡支付 |
| scan | 扫码支付 |
| transfer | 帐户转账 |
| mini | 小程序支付 |
### 2、微信
* 公众号支付
* 小程序支付
* H5 支付
* 扫码支付
* 刷卡支付
* APP 支付
* 企业付款
* 普通红包
* 分裂红包
| method | 描述 |
| :-: | :-: |
| mp | 公众号支付 |
| miniapp | 小程序支付 |
| wap | H5 支付 |
| scan | 扫码支付 |
| pos | 刷卡支付 |
| app | APP 支付 |
| transfer | 企业付款 |
| redpack | 普通红包 |
| groupRedpack |
## 安装
**安装 方式1**
~~~shell
composer require yansongda/pay -vvv
~~~
**安装 方式2**
执行上面命令或者执行下面
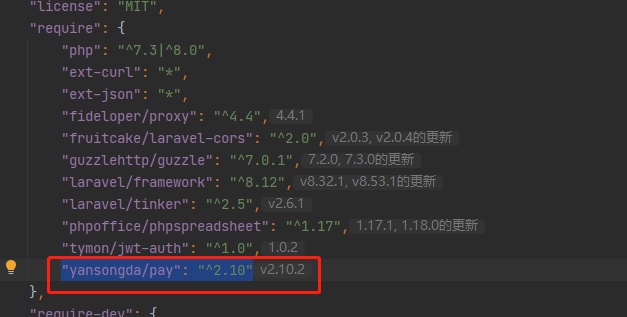
~~~
"yansongda/pay": "^2.10"
~~~
`composer install`
composer install 报错版本不匹配 或者如下图
执行 `composer install --ignore-platform-reqs` 忽略版本号
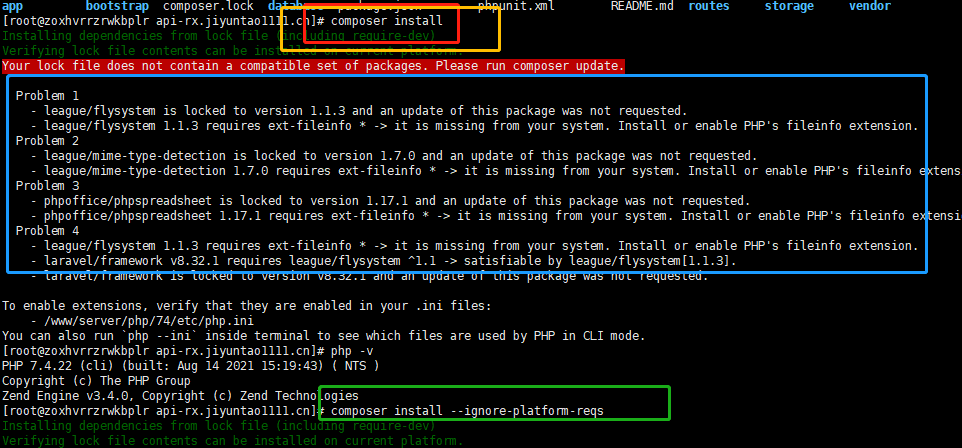
*****
**使用**
下面例子是 laravel8.0 且仅仅用了 h5 和web
app 支付测试 总是报错 最后使用支付宝 官方提供
~~~
//支付入口
public function alipayIndex(Request $request)
{
$money = $request->money ?? 39.9; //支付金额
$user_id = $request->id ?? null; //用户ID
$pay_type = $request->pay_type ?? 'h5'; // 支付方式
$out_trade_no = date("YmdHis").rand(10,99).rand(100,999);//订单号
$alipay_order = [
'out_trade_no' => $out_trade_no,
'total_amount' => $money,
'subject' => 'fenqixin',
];
$config = Config::get("alipay.h5_pay");
$alipay = Pay::alipay($config);
$result = Order::insertOrder($user_id,$money,$out_trade_no,2);
if(!$result) return false;
if($pay_type == 'h5'){
return $alipay->wap($alipay_order);
}
return $alipay->web($alipay_order);
}
~~~
~~~
//支付宝验签 h5
public function alipayNoticeApp()
{
$config = Config::get("alipay.h5_pay");
$data = Pay::alipay($config)->verify();
$order_id = $data->out_trade_no;
$money = $data->total_amount;
$where = [
['order_id','=',$order_id],
['money','=',$money]
];
if($data->trade_status == "TRADE_SUCCESS" || $data->trade_status == "TRADE_FINISHED"){
$order_data = Order::query()->where($where)->first();
if($order_data->status == 's') return true;//该订单 已经处理过 无需更新 订单 用户 状态
Order::query()->where($where)->update(['status' => 's']);//处理订单成功修改
User::updateUserVipStatus($order_data->user_id);//修改用户VIP状态 和 VIP时间
}else{
Order::query()->where($where)->update(['status' => 'f']);
}
return true;
}
~~~
支付宝官方支付
1 下载官方包 放在laravel框架 vendor目录下
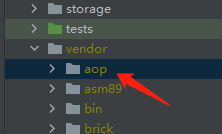
~~~
public function _aliPay($alipay_order,$config)
{
$dir = __DIR__.'/../../../vendor/';//根据自己控制器路径调整对应位置
require_once $dir.'aop/AopClient.php';
require_once $dir.'aop/request/AlipayTradeAppPayRequest.php';
$aop = new \AopClient;
$aop->gatewayUrl = "https://openapi.alipay.com/gateway.do";
$aop->appId = $config['app_id'];
$aop->rsaPrivateKey = $config['private_key'];
$aop->format = "json";
$aop->charset = "UTF-8";
$aop->signType = "RSA2";
$aop->alipayrsaPublicKey = $config['ali_public_key'];
//实例化具体API对应的request类,类名称和接口名称对应,当前调用接口名称:alipay.trade.app.pay
$req = new \AlipayTradeAppPayRequest();
$notify_url = "https://api-fqx.fenqiqian.com.cn/app/alipay-notice-app-app";
$subject = $alipay_order['subject'];
$body = '订单详情';
$out_trade_no = $alipay_order['out_trade_no'];
$money = $alipay_order['total_amount'];
$bizcontent = "{\"body\":\"".$body."\","
. "\"subject\": \"".$subject."\","
. "\"out_trade_no\": \"".$out_trade_no."\","
. "\"timeout_express\": \"30m\","
. "\"total_amount\": \"".$money."\","
. "\"product_code\":\"QUICK_MSECURITY_PAY\""
. "}";
$req->setNotifyUrl($notify_url);
$req->setBizContent($bizcontent);
return $aop->sdkExecute($req);
}
~~~
~~~
//支付宝验签 app
public function alipayNoticeAppAPP()
{
$config = Config::get("alipay.app_pay");
$data = Pay::alipay($config)->verify();
$order_id = $data->out_trade_no;
$money = $data->total_amount;
$where = [
['order_id','=',$order_id],
['money','=',$money]
];
DB::table("alipay")->insert(['status' => $order_id]);
DB::table("alipay")->insert(['status' => $money]);
if($data->trade_status == "TRADE_SUCCESS" || $data->trade_status == "TRADE_FINISHED"){
return true;
}
}
~~~
*****
config 配置
~~~
'app_pay' => [
// APPID
'app_id' => '2021002156678492',
// 支付宝 支付成功后 主动通知商户服务器地址 注意 是post请求
'notify_url' => 'https://api-xxxx.cn/app/alipay-notice-app-app',
// 支付宝 支付成功后 回调页面 get
// 'return_url' => 'https://xxx/pages/gather/initialSuccess',
// 公钥(注意是支付宝的公钥,不是商家应用公钥)
'ali_public_key' => '',
// 加密方式: **RSA2** 私钥 商家应用私钥
'private_key' => "",
// 'http' => [
// 'timeout' => 5.0,
// 'connect_timeout' => 5.0,
// // 更多配置项请参考 [Guzzle](https://guzzle-cn.readthedocs.io/zh_CN/latest/request-options.html)
// ],
]
~~~
- 文档说明
- 开始
- linux
- 常用命令
- ps -ef
- lsof
- netstat
- 解压缩
- 复制
- 权限
- 其他
- lnmp集成安装
- supervisor
- 安装
- supervisor进程管理
- nginx
- 域名映射
- 负载均衡配置
- lnmp集成环境安装
- nginx源码安装
- location匹配
- 限流配置
- 日志配置
- 重定向配置
- 压缩策略
- nginx 正/反向代理
- HTTPS配置
- mysql
- navicat创建索引
- 设置外网链接mysql
- navicat破解
- sql语句学习
- 新建mysql用户并赋予权限
- php
- opcache
- 设计模式
- 在CentOS下安装crontab服务
- composer
- 基础
- 常用的包
- guzzle
- 二维码
- 公共方法
- 敏感词过滤
- IP访问频次限制
- CURL
- 支付
- 常用递归
- 数据排序
- 图片相关操作
- 权重分配
- 毫秒时间戳
- base64<=>图片
- 身份证号分析
- 手机号相关操作
- 项目搭建 公共处理函数
- JWT
- 系统函数
- json_encode / json_decode 相关
- 数字计算
- 数组排序
- php8
- jit特性
- php8源码编译安装
- laravel框架
- 常用artisan命令
- 常用查询
- 模型关联
- 创建公共方法
- 图片上传
- 中间件
- 路由配置
- jwt
- 队列
- 定时任务
- 日志模块
- laravel+swoole基本使用
- 拓展库
- 请求接口log
- laravel_octane
- 微信开发
- token配置验证
- easywechart 获取用户信息
- 三方包
- webman
- win下热更新代码
- 使用laravel db listen 监听sql语句
- guzzle
- 使用workman的httpCLient
- 修改队列后代码不生效
- workman
- 安装与使用
- websocket
- eleticsearch
- php-es 安装配置
- hyperf
- 热更新
- 安装报错
- swoole
- 安装
- win安装swoole-cli
- google登录
- golang
- 文档地址
- 标准库
- time
- 数据类型
- 基本数据类型
- 复合数据类型
- 协程&管道
- 协程基本使用
- 读写锁 RWMutex
- 互斥锁Mutex
- 管道的基本使用
- 管道select多路复用
- 协程加管道
- beego
- gin
- 安装
- 热更新
- 路由
- 中间件
- 控制器
- 模型
- 配置文件/conf
- gorm
- 初始化
- 控制器 模型查询封装
- 添加
- 修改
- 删除
- 联表查询
- 环境搭建
- Windows
- linux
- 全局异常捕捉
- javascript
- 常用函数
- vue
- vue-cli
- 生产环境 开发环境配置
- 组件通信
- 组件之间通信
- 父传子
- 子传父
- provide->inject (非父子)
- 引用元素和组件
- vue-原始写法
- template基本用法
- vue3+ts项目搭建
- vue3引入element-plus
- axios 封装网络请求
- computed 计算属性
- watch 监听
- 使用@符 代替文件引入路径
- vue开发中常用的插件
- vue 富文本编辑
- nuxt
- 学习笔记
- 新建项目踩坑整理
- css
- flex布局
- flex PC端基本布局
- flex 移动端基本布局
- 常用css属性
- 盒子模型与定位
- 小说分屏显示
- git
- 基本命令
- fetch
- 常用命令
- 每次都需要验证
- git pull 有冲突时
- .gitignore 修改后不生效
- 原理解析
- tcp与udp详解
- TCP三次握手四次挥手
- 缓存雪崩 穿透 更新详解
- 内存泄漏-内存溢出
- php_fpm fast_cgi cig
- redis
- 相关三方文章
- API对外接口文档示范
- elaticsearch
- 全文检索
- 简介
- 安装
- kibana
- 核心概念 索引 映射 文档
- 高级查询 Query DSL
- 索引原理
- 分词器
- 过滤查询
- 聚合查询
- 整合应用
- 集群
- docker
- docker 简介
- docker 安装
- docker 常用命令
- image 镜像命令
- Contrainer 容器命令
- docker-compose
- redis 相关
- 客户端安装
- Linux 环境下安装
- uni
- http请求封装
- ios打包
- 视频纵向播放
- 日记
- 工作日记
- 情感日志
- 压测
- ab
- ui
- thorui
- 开发规范
- 前端
- 后端
- 状态码
- 开发小组未来规划