[TOC]
<br/><br/><br/>
# <b style="color:#4F4F4F;">简介说明</b>
结构脑图:[地址](http://naotu.baidu.com/file/f2bb85c58468f9bcbca228ecb8ff004b?token=1fa1b5a27a1e902e)
原文链接:
- [Microsoft 技术文档](https://docs.microsoft.com/zh-cn/)
- [.NET 中的程序集](https://docs.microsoft.com/zh-cn/dotnet/standard/assembly/)
- [建议用于 C# 文档注释的 XML 标记](https://docs.microsoft.com/zh-cn/dotnet/csharp/codedoc)
- [.NET 微服务](https://learn.microsoft.com/zh-cn/dotnet/architecture/microservices/)
```
版本:C#
作用:简单的、现代的、通用的、面向对象的编程语言
```
<br/>
# <b style="color:#4F4F4F;">基本语法</b>
<br/>
# <span style="color:#619BE4">public</span>
*****
声明公共的内容
<br/>
# <span style="color:#619BE4">private</span>
*****
声明私有的内容
<br/>
# <span style="color:#619BE4">partial</span>
*****
声明部分的内容
<br/>
# <span style="color:#619BE4">internal</span>
*****
用于类型或成员,使用该修饰符声明的类型或成员只能在同一程集内访问
<br/>
# <span style="color:#619BE4">throw</span>
*****
抛出一个异常
<br/>
# <span style="color:#619BE4">using static</span>
*****
导入一个静态对象
<br/>
# <span style="color:#619BE4">using</span>
*****
导入一个命名空间
<br/>
### 参数说明
<b style="color:#808080;">static:</b>
* 类型:字符串
* 默认值:无
* 描述:导入一个静态的方法
* 可选值:[ ]
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
// 自动调用Dispose()方法
using (var i = new MyDispose())
{
i.Print();
};
```
<br/>
# <span style="color:#619BE4">switch</span>
*****
分支语句
<br/>
### 示例内容
<span style="color:red;">1. 类判断</span>
```
public static decimal ComputeSalesTax(Address location, decimal salePrice) {
return location switch
{
{ State: "WA" } => salePrice * 0.06M,
{ State: "MN" } => salePrice * 0.075M,
{ State: "MI" } => salePrice * 0.05M,
_ => 0M
};
}
```
<span style="color:red;">2. 位置模式</span>
```
static Quadrant GetQuadrant(Point point) => point switch
{
(0, 0) => Quadrant.Origin,
var (x, y) when x > 0 && y > 0 => Quadrant.One,
var (x, y) when x < 0 && y > 0 => Quadrant.Two,
var (x, y) when x < 0 && y < 0 => Quadrant.Three,
var (x, y) when x > 0 && y < 0 => Quadrant.Four,
var (_, _) => Quadrant.OnBorder,
_ => Quadrant.Unknown
};
```
<br/>
# <span style="color:#619BE4">try catch when</span>
*****
当when满足时捕获异常
<br/>
# <span style="color:#619BE4">$" "</span>
*****
使{ }里面的内容可以被替换
<br/>
# <span style="color:#619BE4">@" "</span>
*****
字符串中需要转义内容可以直接写入
<br/>
# <span style="color:#619BE4">type?</span>
*****
定义对象时预先声明对象可为空值
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
int?[] arr=new int?[7];
```
<br/>
# <span style="color:#619BE4">_</span>
*****
弃元,形参命名忽略占位符
<br/>
# <span style="color:#619BE4">::</span>
*****
命名冲突解决方案
<br/>
# <span style="color:#619BE4">?:</span>
*****
三元运算符
<br/>
# <span style="color:#619BE4">??</span>
*****
a??b 当a为null时则返回b,a不为null时则返回a本身
<br/>
# <span style="color:#619BE4">??=</span>
*****
仅当左操作数计算为 null 时,才能使用运算符 ??= 将其右操作数的值分配给左操作数
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
List<int> numbers = null;
int? i = null;
numbers ??= new List<int>();
numbers.Add(i ??= 17);
numbers.Add(i ??= 20);
Console.WriteLine(string.Join(" ", numbers)); // output: 17 17
Console.WriteLine(i); // output: 17
```
<br/>
# <span style="color:#619BE4">?.</span>
*****
如果对象为NULL,则不进行后面的获取成员的运算,直接返回NULL
<br/>
# <span style="color:#619BE4">?[]</span>
*****
不存在索引不继续调用,直接返回null
<br/>
# <span style="color:#619BE4">..</span>
*****
范围运算符
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
可以使用 ^1 索引检索最后一个词
Console.WriteLine($"The last word is {words[^1]}");
它包括 words[1] 到 words[3]。 元素 words[4] 不在该范围内
var quickBrownFox = words[1..4];
```
<br/>
# <span style="color:#619BE4">-\></span>
*****
结构体指针语法糖
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
public struct Coords
{
public int X;
public int Y;
public override string ToString() => $"({X}, {Y})";
}
public class PointerMemberAccessExample
{
public static unsafe void Main()
{
Coords coords;
Coords* p = &coords;
p->X = 3;
p->Y = 4;
Console.WriteLine(p->ToString()); // output: (3, 4)
}
}
```
<br/>
# <span style="color:#619BE4">record</span>
*****
定义整个对象都是不可变的,且行为像一个值
<br/>
# <span style="color:#619BE4">with</span>
*****
修改init属性值,生成副本
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
var person = new Person { FirstName = "Mads", LastName = "Nielsen" };
var otherPerson = person with { LastName = "Torgersen" };
```
<span style="color:red;">2. 定义解构方法</span>
```
using System;
namespace ConsoleApp2
{
public record Person
{
public string FirstName { get; init; }
public string LastName { get; init; }
public Person(string firstName, string lastName)
=> (FirstName, LastName) = (firstName, lastName);
public void Deconstruct(out string firstName, out string lastName) {
(firstName, lastName) = (FirstName, LastName);
}
}
class Program
{
static void Main(string[] args) {
var person = new Person("a", "b");
var (a, b) = person;
Console.WriteLine(a);
Console.WriteLine(b);
}
}
}
```
<br/>
# <span style="color:#619BE4">var</span>
*****
让编译器编译时类型推断
<br/>
# <span style="color:#619BE4">item</span>
*****
类定义item属性
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
int this[int index] { get; }
```
<br/>
# <span style="color:#619BE4">implicit</span>
*****
确保转换过程不会造成数据丢失,则可使用该关键字在用户定义类型和其他类型之间进行隐式转换
<br/>
# <span style="color:#619BE4">operator</span>
*****
操作符重载
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
class Trial1
{
public static Trial1 operator +(Trial1 t1)
{
// 运算符重载重载
return new Trial1();
}
public static implicit operator Trial2(Trial1 op1)
{
// 隐式转换重载
return new Trial2();
}
}
class Trial2
{
public static explicit operator Trial1(Trial2 op2)
{
// 显式转换重载
return new Trial1();
}
}
```
<br/>
# <span style="color:#619BE4">override</span>
*****
重写父类方法
<br/>
# <span style="color:#619BE4">new</span>
*****
隐藏父类方法
<br/>
# <span style="color:#619BE4">dynamic</span>
*****
声明动态类型,编译时不进行类型检测,对象方法属性都是可变的
<br/>
# <span style="color:#619BE4">struct</span>
*****
定义一个结构变量
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
class Program
{
struct Light
{
public Color color;
public int size;
}
private static void Main(string[] args)
{
if (args is null)
{
throw new ArgumentNullException(nameof(args));
}
Light myLight;
myLight.size = 21;
myLight.color = Color.RED;
string testStr = JsonConvert.SerializeObject(myLight);
Console.WriteLine(testStr);
Console.ReadKey();
}
}
```
<br/>
# <span style="color:#619BE4">enum</span>
*****
定义枚举类型
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
using System;
namespace ConsoleApp
{
enum Orientation : int
{
RED = 1,
BLUE = 2,
YELLOW = 3
}
class Program
{
private static void Main(string[] args)
{
if (args is null)
{
throw new ArgumentNullException(nameof(args));
}
Console.WriteLine($"{Orientation.BLUE.ToString()}");
Console.WriteLine($"{(int)Orientation.BLUE}");
Console.ReadKey();
}
}
}
```
<br/>
# <span style="color:#619BE4">lambada</span>
*****
匿名函数
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
static int Add(int x, int y) => x + y;
```
<br/>
# <span style="color:#619BE4">event</span>
*****
声明事件委托变量
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
using System;
namespace Sample001
{
public delegate void InformHandle(object sender);
public class JIA
{
public event InformHandle EatOver;
public void Eat()
{
Console.WriteLine("吃饭中......");
System.Threading.Thread.Sleep(2000); //吃饭事件两秒
OnEating(); //这个相当于是一个信号,当运行这个函数的时候会发出一个信号。
}
public virtual void OnEating()
{
EatOver?.Invoke(this);
}
}
public class YI
{
public string name = "yi";
public void TakeJiaToWangBa(object sender)
{
Console.WriteLine(name + "带" + sender.ToString() + "去网吧!");
}
}
public class BING
{
public string name = "bing";
public void TakeJiaToWangBa(object sender)
{
Console.WriteLine(name + "带" + sender.ToString() + "去网吧!");
}
}
class Program
{
static void Main(string[] args)
{
JIA jia1 = new JIA();
YI yi1 = new YI();
BING bing1 = new BING();
jia1.EatOver += new InformHandle(yi1.TakeJiaToWangBa);
jia1.EatOver += new InformHandle(bing1.TakeJiaToWangBa);
Console.WriteLine("空闲中");
Console.WriteLine("现在甲不知道在干什么");
jia1.Eat();
Console.WriteLine("去了网吧通宵一个晚上到了第二天中午");
jia1.Eat();
Console.ReadKey();
}
}
}
```
<br/>
# <span style="color:#619BE4">delegate</span>
*****
委托函数
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
class Program
{
delegate int DelegateProcess(int x, int y);
static int Add(int x, int y) => x + y;
static int Subtraction(int x, int y) => x - y;
private static void Main(string[] args)
{
if (args is null)
{
throw new ArgumentNullException(nameof(args));
}
DelegateProcess process;
string directive = Console.ReadLine();
if (directive.ToLower() == "m")
{
process = new DelegateProcess(Add);
}
else
{
process = new DelegateProcess(Subtraction);
}
Console.WriteLine($"{process(15, 23)}");
Console.ReadKey();
}
}
```
<span style="color:red;">2. 接收委托</span>
```
namespace ConsoleApp
{
public delegate string MyCalculator();
class Trial
{
public void Add(MyCalculator culator)
{
Console.WriteLine(culator());
}
}
class Program
{
public static string Print()
{
return "hello";
}
static void Main(string[] args)
{
Trial t1 = new Trial();
t1.Add(delegate ()
{
return "abc";
});
MyCalculator c = new MyCalculator(Print);
Trial t2 = new Trial();
t2.Add(c);
Console.ReadKey();
}
}
}
```
<br/>
# <span style="color:#619BE4">params</span>
*****
定义参数数组,可以传入任意数量参数
<br/>
# <span style="color:#619BE4">ref</span>
*****
定义引用类型变量,无须返回,直接影响作用域变量值
<br/>
# <span style="color:#619BE4">out</span>
*****
定义输出类型变量,和ref用法一样,但是能接收未初始化变量,直接影响作用域变量值
<br/>
# <span style="color:#619BE4">base</span>
*****
调用继承后父类内容
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
using System;
namespace ConsoleApp
{
class Parent
{
public Parent()
{
Console.WriteLine("hello parent");
}
public void Print()
{
Console.WriteLine("print");
}
}
class Child : Parent
{
public Child() : base()
{
Console.WriteLine("hello child");
}
public new void Print() // new 关键字代表覆盖掉父类方法
{
base.Print();
Console.WriteLine("abc");
}
}
class Program
{
private static void Main(string[] args)
{
if (args is null)
{
throw new ArgumentNullException(nameof(args));
}
new Child().Print();
Console.ReadKey();
}
}
}
```
<br/>
# <span style="color:#619BE4">T</span>
*****
定义泛类型
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
public class MyClass<T>
{
readonly T innerT;
public MyClass(T val)
{
innerT = val;
Console.WriteLine(default(T));
}
public void Print()
{
Console.WriteLine(innerT);
}
}
class Program
{
private static void Main(string[] args)
{
new MyClass<int>(233).Print();
Console.ReadKey();
}
}
```
<span style="color:red;">2. 泛型约束</span>
```
public class MyClass5<T,K,V,W,X,Y,Z>
where T:struct //约束T必须是值类型
where K:class //约束K必须是引用类型
where V:IComparable //约束V必须实现IComparable,int就符合,原因是int类型实现了IComparable接口
where W:K //要求W必须是K类型或者K类型的子类
where X:class,new() //多个约束,其中new()表示无参数的构造函数,表示约束是引用类型并且有无参数的构造函数
{
}
```
<br/>
# <span style="color:#619BE4">contravariance</span>
*****
协变,关键字out,隐式改变T的类型
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
namespace ConsoleApp
{
public class RectangleBase
{
public int ID { get; set; }
}
public class Rectangle : RectangleBase
{
// 继承RectangleBase
public string Name { get; set; }
}
public interface IIndex<out T>
{
T this[int index] { get; }
int Count { get; }
}
public class RectangleCollection : IIndex<Rectangle>
{
List<Rectangle> list = new List<Rectangle>();
public Rectangle this[int index]
{
get
{
if (index < 0 || index > Count)
throw new ArgumentOutOfRangeException("index");
return list[index];
}
}
public int Count
{
get { return list.Count; }
}
public void Add(Rectangle value)
{
list.Add(value);
}
}
class Program
{
static void Main(string[] args)
{
RectangleCollection list = new RectangleCollection();
list.Add(new Rectangle { ID = 1, Name = "111" });
list.Add(new Rectangle { ID = 2, Name = "222" });
list.Add(new Rectangle { ID = 3, Name = "33" });
// RectangleCollection 同时也是 IIndex<Rectangle>
// 如果接口去掉out,无法隐式改变成IIndex<RectangleBase>
// 加上out关键字,代表可以隐式发生协变
IIndex<RectangleBase> Bas = list;
for (int i = 0; i < Bas.Count; i++)
{
Console.WriteLine(Bas[i].ID);
}
Console.ReadKey();
}
}
}
```
<br/>
# <span style="color:#619BE4">convariance</span>
*****
抗变,关键字in,抗变让 T 只能用作方法参数,不能用作返回内容
<br/>
# <span style="color:#619BE4">new{}</span>
*****
快捷生成字典
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
var c = new { Name = 123 };
```
<br/>
# <span style="color:#619BE4">new[]</span>
*****
快捷生成列表
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
var c = new[] {
1,2,3,4,5,6
};
```
<br/>
# <span style="color:#619BE4">sealed</span>
*****
定义类无法被继承,无法继承的类
<br/>
# <span style="color:#619BE4">kwargs</span>
*****
命名参数
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
class Trial
{
public void Add([Optional] int age, string name = "zyp")
{
Console.WriteLine(name);
Console.WriteLine(age);
}
}
class Program
{
static void Main(string[] args)
{
new Trial().Add(name: "kkp");
Console.ReadKey();
}
}
```
<br/>
# <span style="color:#619BE4">Extensions</span>
*****
静态方法拓展
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
public static class MyConfigurationBuilderExtensions
{
public static IConfigurationBuilder AddMyConfiguration(this IConfigurationBuilder builder) {
builder.Add(new MyConfigurationSource());
return builder;
}
}
```
<br/>
# <span style="color:#619BE4">is</span>
*****
检查类型是否兼容
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
System.Boolean b1 = (o is System.Object);//b1 为true
System.Boolean b2 = (o is Employee);//b2为false
```
<br/>
# <span style="color:#619BE4">field</span>
*****
使用它时会自动为属性创建字段定义
<br/>
# <span style="color:#619BE4">global</span>
*****
定义一系列的类型别名在整个项目内使用
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
```
global using System.Linq;
global using static System.Math;
global using i32 = System.Int32;
global using i64 = System.Int64;
```
<br/>
# <span style="color:#619BE4">extern</span>
*****
声明在外部实现的方法
<br/>
# <span style="color:#619BE4">nameof()</span>
*****
可生成变量、类型或成员的名称作为字符串常量
<br/>
# <span style="color:#619BE4">typeof()</span>
*****
获取对象类型
<br/>
### 返回类型
```
System.Type
```
<br/>
# <span style="color:#619BE4">sizeof()</span>
*****
获取变量大小
<br/>
# <span style="color:#619BE4">default()</span>
*****
获取该类型的默认值,同时把关键字赋值给变量同样可以得到默认值
<br/>
# <span style="color:#619BE4">checked()</span>
*****
检查是否强制转换会产生数据溢出,溢出报异常
<br/>
# <span style="color:#619BE4">unchecked()</span>
*****
不检查强制转换会产生数据溢出,直接自动舍去多余数据
<br/>
# <b style="color:#4F4F4F;">特性内容</b>
<br/>
# <span style="color:#619BE4">编译过程</span>
*****
编译过程及原理图
<br/>
### 示例内容
<span style="color:red;">1. 举例说明</span>
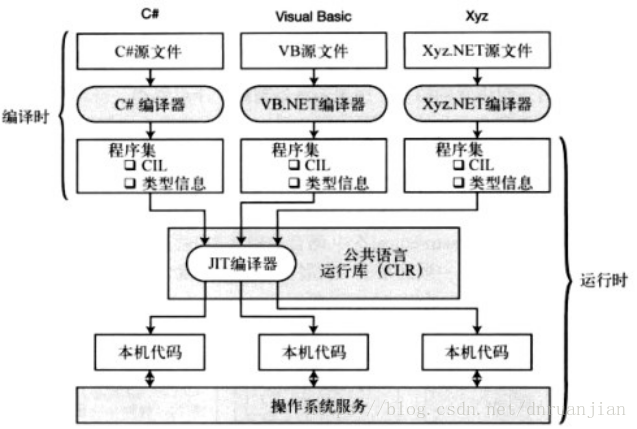
<br/>
- Blade
- Design
- Adobe Photoshop
- 指令指南
- issue
- Adobe illustrator
- 指令指南
- issue
- Adobe Audition
- 指令指南
- issue
- Adobe Premiere
- 指令指南
- issue
- Adobe After Effects
- 指令指南
- issue
- CorelDraw
- 指令指南
- issue
- Sketch
- 指令指南
- Pixso
- 指令指南
- Blender
- 指令指南
- issue
- Program
- Assembly
- 标准库
- builtins
- 三方库
- C
- 标准库
- sys
- stat.h
- timeb.h
- time.h
- assert.h
- conio.h
- ctype.h
- errno.h
- float.h
- iconv.h
- io.h
- limits.h
- locale.h
- math.h
- regex.h
- setjmp.h
- signal.h
- stdarg.h
- stdatomic.h
- stdbool.h
- stddef.h
- stdint.h
- stdio.h
- stdlib.h
- string.h
- time.h
- unistd.h
- wchar.h
- windows.h
- consoleapi2.h
- synchapi.h
- 三方库
- curl
- glib
- gio
- gapplication.h
- gioenums.h
- glib
- gmessages.h
- gobject
- gobject.h
- gsignal.h
- gclosure.h
- glib.h
- gtk
- gtk.h
- gtksettings.h
- gtkmain.h
- gtkbuilder.h
- gtkapplication.h
- gtkfixed.h
- gtkbox.h
- gtkgrid.h
- gtktextbuffer.h
- gtkwidget.h
- gtkcontainer.h
- gtkwindow.h
- gmodule-export
- pango
- pango.h
- python
- Python.h
- import.h
- modsupport.h
- object.h
- pylifecycle.h
- pythonrun.h
- tcl/tk
- tcl.h
- tk.h
- tinycthread
- zlib
- zlib.h
- C++
- 标准库
- cstdlib
- iostream
- string
- 三方库
- CSharp
- 标准库
- Microsoft.AspNetCore.Builder
- Microsoft.AspNetCore.Hosting
- Microsoft.AspNetCore.Http
- Microsoft.AspNetCore.Mvc
- Microsoft.AspNetCore.Mvc.Filters
- Microsoft.AspNetCore.Mvc.Razor
- Microsoft.AspNetCore.Razor.TagHelpers
- Microsoft.AspNetCore.Mvc.RazorPages
- Microsoft.AspNetCore.Mvc.Rendering
- Microsoft.AspNetCore.Rewrite
- Microsoft.AspNetCore.Routing
- Microsoft.AspNetCore.WebUtilities
- Microsoft.Extensions.Configuration
- Microsoft.Extensions.DependencyInjection
- Microsoft.AspNetCore.Authorization
- Microsoft.Extensions.FileProviders
- Microsoft.Extensions.Hosting
- Microsoft.Extensions.Logging
- Microsoft.Extensions.Options
- Microsoft.Extensions.Primitives
- Microsoft.OpenApi.Models
- Microsoft.Net.Http.Headers
- Microsoft.VisualBasic.CompilerServices
- System
- ICloneable
- IDisposable
- IEnumerable
- IEnumerator
- Activator
- AppDomain
- AppDomainSetup
- Array
- Attribute
- Console
- ConsoleColor
- Convert
- Enum
- Environment
- EventHandler
- int
- Object
- Random
- string
- Type
- Uri
- Tuple
- ValueTuple
- System.Collections
- System.Collections.Generic
- System.ComponentModel
- System.ComponentModel.DataAnnotations
- System.ComponentModel.DataAnnotations.Schema
- System.Data
- System.Diagnostics
- System.Drawing
- System.Dynamic
- System.IO
- System.Linq
- Enumerable
- System.Net
- WebRequest
- HttpWebRequest
- HttpWebResponse
- WebClient
- System.Net.Http
- System.Net.Http.Json
- System.Net.Mime
- System.Net.Sockets
- System.Reflection
- System.Runtime.InteropServices
- System.Runtime.Serialization
- System.Text
- System.Text.Json
- System.Text.Json.Serialization
- System.Text.Encodings.Web
- System.Text.RegularExpressions
- System.Threading
- System.Threading.Tasks
- System.Timers
- System.Web
- System.Windows
- System.Windows.Forms
- Form
- FlowLayoutPanel
- TableLayoutPanel
- MessageBox
- Button
- Label
- LinkLabel
- TextBox
- 三方库
- Autofac
- Autofac.Configuration
- Autofac.Extensions.DependencyInjection
- Autofac.Extras.DynamicProxy
- AutoMapper
- castle.core
- Glob
- IronPython
- Hosting
- Microsoft.Extensions.Logging.Log4Net.AspNetCore
- Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation
- Microsoft.AspNetCore.Mvc.Versioning
- Microsoft.AspNetCore.SpaServices.Extensions
- Microsoft.AspNetCore.SpaServices
- ReactDevelopmentServer
- StaticFiles
- Microsoft.EntityFrameworkCore
- Infrastructure
- Metadata
- Builders
- Microsoft.EntityFrameworkCore.SqlServer
- Microsoft.Web.WebView2
- Core
- WinForms
- MySql.Data
- MySqlClient
- Newtonsoft.Json
- Converters
- Linq
- Serialization
- QRCoder
- RazorEngine.NetCore
- RazorLight
- Serilog.AspNetCore
- SixLabors.ImageSharp
- Swashbuckle.AspNetCore
- System.Data.SqlClient
- System.Drawing.Common
- Java
- 标准库
- java.applet
- java.awt
- image
- BufferedImage
- java.beans
- java.io
- Serializable
- OutputStream
- InputStream
- Writer
- Reader
- File
- FileOutputStream
- RandomAccessFile
- OutputStreamWriter
- InputStreamReader
- FileWriter
- PipedOutputStream
- PrintStream
- BufferedReader
- SequenceInputStream
- PrintWriter
- DataOutputStream
- DataInputStream
- ByteArrayOutputStream
- java.lang
- annotation
- ref
- reflect
- AutoCloseable
- Boolean
- Class
- ClassLoader
- Comparable
- CharSequence
- Error
- Exception
- Integer
- Math
- Object
- Runtime
- StringBuilder
- StringBuffer
- String
- System
- Thread
- Throwable
- java.math
- BigInteger
- BigDecimal
- java.net
- ServerSocket
- Socket
- URL
- URLConnection
- HttpURLConnection
- URLEncoder
- java.nio
- charset
- StandardCharsets
- channels
- file
- FileSystems
- FileSystem
- Paths
- Path
- Files
- java.rmi
- java.security
- java.sql
- DriverManager
- java.text
- MessageFormat
- DateFormat
- SimpleDateFormat
- NumberFormat
- java.time
- format
- DateTimeFormatter
- Clock
- Instant
- LocalDateTime
- ZonedDateTime
- ZoneId
- Period
- Duration
- java.util
- regex
- Pattern
- Matcher
- function
- Consumer
- Supplier
- Function
- BinaryOperator
- Predicate
- stream
- IntStream
- Stream
- concurrent
- Callable
- Optional
- Scanner
- EnumSet
- Collections
- Collection
- Iterator
- Arrays
- Vector
- ArrayList
- LinkedList
- List
- Set
- HashSet
- Map
- HashMap
- Hashtable
- TreeMap
- Properties
- Queue
- Deque
- Locale
- ResourceBundle
- Date
- Calendar
- TimeZone
- Random
- Comparator
- Observer
- Observable
- TimerTask
- Timer
- ServiceLoader
- javax.accessibility
- javax.activation
- javax.activity
- javax.annotation
- javax.crypto
- javax.imageio
- ImageIO
- javax.jws
- javax.lang
- javax.management
- javax.naming
- javax.net
- javax.print
- javax.rmi
- javax.script
- javax.security
- javax.sound
- javax.sql
- javax.swing
- javax.tools
- javax.transaction
- javax.xml
- org.ietf
- org.omg
- org.w3c
- org.xml
- 三方库
- joda-time
- joda-time
- mysql
- mysql-connector-java
- redis.clients
- jedis
- Jedis
- JedisPool
- javax.servlet
- javax.servlet-api
- http
- HttpServlet
- HttpServletRequest
- ServletContext
- jstl
- javax.servlet.jsp
- jsp-api
- PageContext
- junit
- junit
- runner
- commons-beanutils
- commons-beanutils
- commons-dbutils
- commons-dbutils
- DbUtils
- commons-codec
- commons-codec
- commons-configuration
- commons-configuration
- commons-fileupload
- commons-fileupload
- commons-io
- commons-io
- commons-httpclient
- commons-httpclient
- io.jsonwebtoken
- jjwt
- ch.qos.logback
- logback-core
- logback-classic
- logback-access
- cn.hutool
- hutool-all
- com.alibaba
- fastjson
- druid
- pool
- DruidDataSourceFactory
- dubbo
- config
- annotation
- com.alibaba.spring.boot
- dubbo-spring-boot-starter
- com.baomidou
- mybatis-plus-boot-starter
- com.fasterxml.jackson.core
- jackson-core
- TypeReference
- jackson-annotations
- jackson-databind
- ObjectMapper
- SimpleModule
- StdDeserializer
- StdSerializer
- com.github.axet
- kaptcha
- com.github.pagehelper
- pagehelper
- PageHelper
- pagehelper-spring-boot-starter
- com.google.code.gson
- gson
- com.google.zxing
- core
- javase
- com.101tec
- zkclient
- com.zaxxer
- HikariCP
- org.apache.httpcomponents
- httpclient
- org.apache.commons
- commons-lang3
- commons-jexl
- org.apache.curator
- curator-framework
- CuratorFrameworkFactory
- CuratorFramework
- ExponentialBackoffRetry
- curator-recipes
- NodeCache
- PathChildrenCache
- TreeCache
- org.apache.poi
- poi
- org.apache.logging.log4j
- log4j-core
- org.apache.shiro
- shiro-core
- org.apache.struts
- struts2-core
- xwork2
- config
- entities
- ActionConfig
- ConfigurationManager
- Configuration
- RuntimeConfiguration
- util
- ValueStack
- inject
- Container
- ActionContext
- ActionProxy
- ActionSupport
- dispatcher
- mapper
- ActionMapper
- Dispatcher
- impl
- StrutsActionProxy
- factory
- StrutsActionProxyFactory
- ServletActionContext
- org.apache.tomcat.embed
- tomcat-embed-core
- tomcat-embed-jasper
- org.apache.zookeeper
- zookeeper
- org.hashids
- hashids
- org.hibernate
- hibernate-core
- cfg
- Configuration
- org.slf4j
- slf4j-api
- slf4j-log4j12
- org.aspectj
- aspectjweaver
- org.projectlombok
- lombok
- org.junit.jupiter
- junit-jupiter-api
- runner
- JUnitCore
- org.hamcrest
- hamcrest-all
- org.mybatis
- mybatis
- annotations
- session
- SqlSession
- SqlSessionFactory
- SqlSessionFactoryBuilder
- io
- Resources
- mybatis-spring
- org.mybatis.spring.boot
- mybatis-spring-boot-starter
- annotation
- org.springframework
- spring-core
- core
- type
- classreading
- CachingMetadataReaderFactory
- io
- support
- PathMatchingResourcePatternResolver
- DefaultResourceLoader
- spring-jcl
- spring-expression
- spring-beans
- factory
- config
- BeanFactoryPostProcessor
- BeanPostProcessor
- PropertyPlaceholderConfigurer
- support
- BeanDefinitionRegistryPostProcessor
- DefaultListableBeanFactory
- RootBeanDefinition
- xml
- XmlBeanDefinitionReader
- InitializingBean
- FactoryBean
- spring-aop
- spring-context
- cache
- annotation
- context
- annotation
- ClassPathScanningCandidateComponentProvider
- support
- ClassPathXmlApplicationContext
- FileSystemXmlApplicationContext
- AnnotationConfigApplicationContext
- ApplicationContext
- ApplicationContextAware
- ui
- Model
- spring-context-support
- spring-tx
- transaction
- support
- DefaultTransactionDefinition
- PlatformTransactionManager
- TransactionStatus
- spring-jdbc
- core
- JdbcTemplate
- RowMapper
- spring-web
- web
- bind
- annotation
- context
- request
- RequestContextHolder
- RequestAttributes
- ServletRequestAttributes
- support
- AnnotationConfigWebApplicationContext
- WebApplicationContextUtils
- XmlWebApplicationContext
- WebApplicationContext
- filter
- CharacterEncodingFilter
- HiddenHttpMethodFilter
- utils
- HtmlUtils
- spring-webmvc
- config
- annotation
- ModelAndView
- HandlerInterceptor
- spring-test
- test
- context
- junit4
- SpringJUnit4ClassRunner
- org.springframework.boot
- spring-boot
- web
- servlet
- support
- SpringBootServletInitializer
- FilterRegistrationBean
- context
- properties
- SpringApplication
- spring-boot-autoconfigure
- domain
- autoconfigure
- spring-boot-actuator-autoconfigure
- spring-boot-devtools
- spring-boot-starter-parent
- spring-boot-starter-web
- spring-boot-starter-freemarker
- spring-boot-starter-thymeleaf
- spring-boot-starter-security
- spring-boot-starter-validation
- spring-boot-starter-data-redis
- spring-boot-starter-actuator
- spring-boot-starter-test
- Groovy
- 标准库
- groovy.lang
- GroovyInterceptable
- GroovyObject
- GroovyShell
- Script
- Binding
- Closure
- groovy.transform
- stc
- groovy.xml
- MarkupBuilder
- org.codehaus.groovy
- runtime
- DefaultGroovyMethods
- InvokerHelper
- 三方库
- Scala
- 标准库
- Kotlin
- 标准库
- 三方库
- Android
- AndroidXML
- anim
- color
- drawable
- layout
- ConstraintLayout
- LinearLayout
- RelativeLayout
- FrameLayout
- TableLayout
- View
- RecyclerView
- TextView
- ScrollView
- ImageView
- ProgressBar
- EditText
- Button
- ToggleButton
- Switch
- RadioGroup
- RadioButton
- CheckBox
- Spinner
- menu
- raw
- values
- xml
- AndroidManifest
- 标准库
- android
- Manifest
- android.annotation
- android.content
- res
- pm
- android.intent
- action
- android.app
- Activity
- ProgressDialog
- android.os
- AsyncTask
- CountDownTimer
- Handler
- Process
- android.view
- View
- LayoutInflater
- MotionEvent
- androidx.recyclerview
- widget
- android.widget
- Toast
- TextView
- ScrollView
- ProgressBar
- android.webkit
- android.text
- android.database
- android.opengl
- android.graphics
- android.util
- android.media
- SoundPool
- AudioAttributes
- AudioManager
- android.bluetooth
- android.support.v4
- app
- FragmentPagerAdapter
- content
- 三方库
- QMUI
- XUI
- androidx.appcompat
- app
- androidx.constraintlayout
- com.bumptech.glide
- com.google.android.material
- issue
- issue
- Go
- 标准库
- fmt
- 三方库
- Erlang
- 标准库
- builtins
- io
- lists
- 三方库
- Lua
- 标准库
- Perl
- 标准库
- builtins
- 三方库
- Ruby
- 标准库
- 三方库
- Python
- 标准库
- future
- abc
- aifc
- argparse
- array
- ast
- asyncio
- windows_events
- events
- coroutines
- futures
- tasks
- locks
- queues
- protocols
- transports
- streams
- subprocess
- threads
- runners
- atexit
- audioop
- base64
- bdb
- binascii
- binhex
- bisect
- builtins
- object
- Exception
- bytes
- bool
- str
- complex
- float
- int
- set
- list
- tuple
- frozenset
- __generator
- __coroutine
- dict
- bytearray
- enumerate
- memoryview
- slice
- range
- type
- super
- bz2
- calendar
- cgi
- cgitb
- chunk
- cmath
- cmd
- code
- codecs
- codeop
- collections
- colorsys
- compileall
- concurrent
- futures
- _base
- process
- thread
- configparser
- contextlib
- contextvars
- copy
- copyreg
- cProfile
- crypt
- csv
- ctypes
- wintypes
- curses
- textpad
- dataclasses
- datetime
- dbm
- decimal
- difflib
- dis
- distutils
- doctest
- parser
- message
- ensurepip
- enum
- errno
- faulthandler
- fcntl
- filecmp
- fileinput
- fnmatch
- fractions
- ftplib
- functools
- gc
- getopt
- getpass
- gettext
- glob
- graphlib
- grp
- gzip
- hashlib
- heapq
- hmac
- html
- http
- client
- server
- cookies
- cookiejar
- imaplib
- imghdr
- importlib
- metadata
- inspect
- io
- ipaddress
- itertools
- json
- encoder
- keyword
- linecache
- locale
- logging
- config
- handlers
- lzma
- mailbox
- mailcap
- marshal
- math
- mimetypes
- mmap
- modulefinder
- msilib
- msvcrt
- multiprocessing
- spawn
- forkserver
- context
- process
- pool
- queues
- managers
- shared_memory
- sharedctypes
- connection
- dummy
- util
- netrc
- nis
- nntplib
- numbers
- operator
- os
- [exp]-os
- linux
- windows
- path
- ossaudiodev
- parser
- pathlib
- pdb
- pickle
- pickletools
- pipes
- pkgutil
- platform
- plistlib
- poplib
- posix
- pprint
- profile
- pstats
- pty
- pwd
- py_compile
- pyclbr
- pydoc
- queue
- quopri
- random
- re
- readline
- reprlib
- resource
- rlcompleter
- runpy
- sched
- secrets
- select
- selectors
- setuptools
- shelve
- shlex
- shutil
- signal
- site
- smtpd
- smtplib
- sndhdr
- socket
- socketserver
- spwd
- sqlite3
- ssl
- stat
- statistics
- string
- stringprep
- struct
- subprocess
- sunau
- symbol
- symtable
- sys
- sysconfig
- syslog
- tabnanny
- tarfile
- telnetlib
- tempfile
- termios
- test
- textwrap
- threading
- time
- timeit
- tkinter
- [exp]-tkinter
- Pack
- Place
- Grid
- Misc
- Wm
- BaseWidget
- Widget
- XView
- Canvas
- PhotoImage
- Toplevel
- Tk
- Menu
- OptionMenu
- Menubutton
- Scrollbar
- PanedWindow
- Frame
- LabelFrame
- Label
- Message
- Entry
- Spinbox
- Text
- Listbox
- Scale
- Button
- Checkbutton
- Radiobutton
- ttk
- [exp]-ttk
- Style
- Separator
- Combobox
- Notebook
- Progressbar
- Treeview
- Sizegrip
- constants
- colorchooser
- font
- dialog
- filedialog
- messagebox
- scrolledtext
- dnd
- token
- tokenize
- trace
- traceback
- tracemalloc
- tty
- turtle
- turtledemo
- types
- typing
- unicodedata
- unittest
- urllib
- request
- parse
- robotparser
- uu
- uuid
- venv
- warnings
- wave
- weakref
- webbrowser
- winreg
- winsound
- wsgiref
- simple_server
- handlers
- headers
- util
- xdrlib
- xml
- xmlrpc
- zipapp
- zipfile
- zipimport
- zlib
- zoneinfo
- 三方库
- aiofiles
- aiohttp
- client_reqrep
- aiomysql
- pool
- connection
- cursors
- aiosqlite
- amzqr
- apispec
- appdirs
- APScheduler
- arrow
- astunparse
- audioread
- awesome-slugify
- Babel
- core
- dates
- bar_chart_race
- base58
- bcrypt
- beautifulsoup4
- better_exceptions
- bitarray
- blinker
- base
- bluepy
- bokeh
- bottle
- bson
- cachelib
- captcha
- case-convert
- camelot
- cefpython3
- celery
- app
- base
- task
- utils
- canvas
- local
- result
- schedules
- certifi
- chardet
- chronyk
- click
- core
- termui
- types
- decorators
- exceptions
- utils
- colorama
- crawley
- crcmod
- crcmod
- predefined
- cryptography
- hazmat
- primitives
- ciphers
- base
- algorithms
- modes
- padding
- backends
- fernet
- dash
- DBUtils
- persistent_db
- pooled_db
- django
- core
- asgi
- wsgi
- urls
- base
- conf
- http
- request
- response
- db
- backends
- sqlite3
- mysql
- models
- fields
- related
- base
- manager
- query
- deletion
- aggregates
- transaction
- views
- generic
- list
- detail
- template
- backends
- django
- context_processors
- loader
- utils
- version
- timezone
- test
- testcases
- contrib
- auth
- models
- context_processors
- messages
- context_processors
- admin
- sites
- options
- shortcuts
- dpark
- delorean
- deprecated
- dnspython
- easygui
- emoji
- emmett
- executing
- evdev
- eventlet
- exrex
- eyed3
- faker
- falcon
- fastapi
- middleware
- cors
- applications
- param_functions
- datastructures
- 生态
- fastapi-mqtt
- ffmpeg-python
- filelock
- filetype
- fire
- flashtext
- flask
- json
- tag
- app
- blueprints
- config
- ctx
- views
- templating
- globals
- helpers
- wrappers
- logging
- sessions
- cli
- utils
- 生态
- flasgger
- base
- utils
- flask-admin
- contrib
- sqla
- view
- ajax
- tools
- form
- fields
- widgets
- fileadmin
- model
- base
- form
- template
- typefmt
- form
- fields
- upload
- rules
- base
- menu
- actions
- helpers
- flask-avatars
- flask-babel
- flask-APScheduler
- flask-babelex
- flask-BasicAuth
- flask-Caching
- flask-ckeditor
- flask-cors
- flask-debugtoolbar
- flask-GraphQL
- flask-HTTPAuth
- flask-login
- login_manager
- mixins
- utils
- flask-Mail
- flask-marshmallow
- flask-migrate
- flask-principal
- flask-restplus
- flask-restful
- flask-seasurf
- flask-security
- flask-session
- flask-socketio
- flask-sockets
- flask-sqlalchemy
- model
- flask-sse
- flask-talisman
- flask-User
- flask_uwsgi_websocket
- flask-wtf
- frida
- core
- frida_tools
- application
- fuzzywuzzy
- GeoAlchemy2
- gevent
- monkey
- pywsgi
- socket
- greenlet
- timeout
- pool
- queue
- event
- local
- lock
- hub
- 生态
- geventwebsocket
- GitPython
- gmqtt
- gooey
- gpiozero
- graphene
- types
- inputobjecttype
- objecttype
- scalars
- field
- schema
- graphene-sqlalchemy
- greenlet
- hbmqtt
- httpx
- icecream
- img2pdf
- inflect
- inquirer
- irc
- itsdangerous
- exc
- signer
- serializer
- url_safe
- timed
- jws
- jieba
- Jinja2
- environment
- loaders
- runtime
- utils
- jmespath
- joblib
- js2py
- json-rpc
- jsonschema
- keyboard
- _keyboard_event
- kombu
- librosa
- lirc
- lxml
- mako
- MarkupSafe
- marshmallow
- schema
- fields
- validate
- decorators
- exceptions
- utils
- marshmallow-sqlalchemy
- schema
- convert
- manim
- mariadb
- marmir
- matplotlib
- pylab
- pyplot
- container
- figure
- gridspec
- axes
- font_manager
- maya
- mechanicalsoup
- memory_profiler
- mimesis
- moment
- motor
- moviepy
- mutagen
- natsort
- netmiko
- numpy
- core
- arrayprint
- function_base
- fromnumeric
- matrixlib
- defmatrix
- lib
- arraypad
- random
- mtrand
- fft
- objgraph
- opencv-contrib-python
- opencv-python
- openpyxl
- workbook
- workbook
- worksheet
- worksheet
- table
- cell
- cell
- reader
- excel
- strings
- styles
- numbers
- fonts
- utils
- units
- formulas
- formula
- translate
- oslo.config
- paho-mqtt
- client
- pandas
- _libs
- tslibs
- io
- excel
- _base
- formats
- printing
- parsers
- core
- dtypes
- missing
- groupby
- groupby
- generic
- indexes
- base
- datetimes
- range
- multi
- reshape
- concat
- merge
- strings
- accessor
- frame
- series
- paramiko
- parse
- passlib
- path
- pdf2image
- pdfkit
- pdfminer.six
- high_level
- pdfparser
- pdfdocument
- pdfinterp
- converter
- pdfpage
- layout
- utils
- pdfplumber
- page
- peewee
- peewee-async
- pefile
- pexpect
- Pillow
- Image
- ImageFilter
- ImageEnhance
- ImageSequence
- ImageDraw
- ImageFont
- ImageTk
- playsound
- poster3
- pproxy
- progress
- promise
- prompt_toolkit
- psutil
- ptable
- pyaudio
- pyautogui
- pybluez
- pycryptodome
- Random
- Cipher
- _mode_ecb
- AES
- PKCS1_v1_5
- PublicKey
- RSA
- Hash
- MD5
- pydantic
- main
- generics
- fields
- networks
- class_validators
- error_wrappers
- env_settings
- pydub
- audio_segment
- playback
- utils
- pyecharts
- PyExecJS
- pyexiv2
- pygame
- pygatt
- PyGithub
- pygments
- pyinotify
- PyInquirer
- PyJWT
- pylibdmtx
- pymongo
- PyMuPdf
- fitz
- utils
- PyMySQL
- connections
- cursors
- pynput
- mouse
- keyboard
- pyOpenSSL
- crypto
- pyparsing
- PyPDF2
- merger
- pyperclip
- pypinyin
- pyqt5
- pyqt5-tools
- pyquery
- pyramid
- pyscreenshot
- pyserial
- tools
- list_ports
- list_ports_windows
- serialwin32
- pyshark
- pyside2
- PySimpleGUI
- pysocks
- pystrich
- pytesseract
- pytz
- pyyaml
- pyzbar
- python-barcode
- base
- ean
- writer
- python-dateutil
- python-docx
- python-dotenv
- main.py
- python-jose
- python-nmap
- python-memcached
- python-multipart
- python-slugify
- python-stdnum
- pythonnet
- pytime
- pyusb
- backend
- core
- util
- pyv8
- pywin32
- pywin
- win32com
- win32con
- win32ui
- win32gui
- win32api
- win32print
- win32clipboard
- pywinauto
- pywinusb
- qrcode
- redis
- reportlab
- requests
- api
- models
- requests-html
- requests-toolbelt
- routes
- rq
- RPi.GPIO
- retrying
- sanic
- scapy
- schedule
- scikit-learn
- scipy
- scrapy
- seaborn
- segno
- selenium
- setproctitle
- shortuuid
- simpleaudio
- simplejson
- six
- smbus
- spacy
- spidev
- SQLAlchemy
- engine
- base
- create
- cursor
- result
- row
- events
- reflection
- sql
- base
- schema
- ddl
- selectable
- dml
- elements
- expression
- functions
- sqltypes
- orm
- session
- scoping
- events
- base
- decl_api
- instrumentation
- mapper
- interfaces
- properties
- relationships
- strategy_options
- query
- collections
- utils
- event
- api
- ext
- automap
- declarative
- hybrid
- association_proxy
- future
- engine
- dialects
- mysql
- types
- inspection
- schema
- sqlparse
- sql
- starlette
- middleware
- cors
- applications
- responses
- staticfiles
- templating
- sympy
- tensorflow
- terminal-layout
- terminaltables
- tinytag
- tkintertable
- TorMySQL
- tornado
- platform
- asyncio
- twisted
- caresresolver
- wsgi
- ioloop
- concurrent
- gen
- queues
- locks
- log
- options
- escape
- iostream
- tcpserver
- tcpclient
- web
- routing
- template
- httpserver
- httpclient
- websocket
- httputil
- netutil
- tortoise-orm
- tqdm
- twisted
- typer
- typing-extensions
- ujson
- urllib3
- uwsgi
- vibora
- vidgear
- w1thermsensor
- wand
- watchdog
- web.py
- web2py
- webargs
- flaskparser
- websockets
- werkzeug
- middleware
- shared_data
- wrappers
- request
- response
- serving
- exceptions
- routing
- local
- datastructures
- filesystem
- urls
- wsgi
- http
- formparser
- security
- utils
- test
- WeRoBot
- win10toast
- windnd
- wmi
- wordcloud
- WTForms
- widgets
- core
- fields
- core
- simple
- form
- validators
- wxpython
- xdot
- xeger
- xlrd
- xlsxwriter
- xlutils
- xlwings
- xlwt
- xpinyin
- yagmail
- yapf
- zerorpc
- zhconv
- zxing
- TCL
- 标准库
- builtins
- package
- namespace
- encoding
- file
- info
- Tk
- 三方库
- PHP
- 标准库
- Core
- Core_d
- date
- fileinfo
- gd
- iconv
- json
- mbstring
- mysqli
- openssl
- PDO
- session
- SPL
- SPL_f
- standard
- _types
- _standard_manual
- basic
- standard_0
- standard_1
- standard_2
- standard_3
- standard_4
- standard_5
- standard_6
- standard_7
- standard_8
- standard_9
- superglobals
- 三方库
- smarty
- thinkphp
- 生态
- topthink/framework
- topthink/think-helper
- topthink/think-orm
- topthink/think-captcha
- Dart
- 标准库
- 三方库
- flutter
- Rust
- 标准库
- macro
- 三方库
- Web
- Document
- HTML
- 文档元素
- 内容分区
- 表格数据
- 操作表单
- 块状盒子
- 内联语义
- 媒体资源
- 功能标签
- 内嵌插件
- 组件支持
- Emmet
- XML
- SVG
- 容器元素
- 图形元素
- 字体元素
- 文本内容
- 动画元素
- 滤镜元素
- 功能元素
- Latex
- markdown
- reStructuredText
- CSS
- 元素选择
- 内置函数
- 边框背景
- 字体文本
- 规格指定
- 经典布局
- 列表样式
- 表现形式
- 动画操作
- 矢量控制
- 平台兼容
- issue
- JavaScript
- 基础类
- this
- Object
- Boolean
- Number
- String
- Symbol
- BigInt
- 结构类
- Date
- RegExp
- Promise
- Proxy
- URL
- URLSearchParams
- Function
- 集合类
- Array
- TypedArray
- Int8Array
- Int16Array
- Int32Array
- Uint8Array
- Uint8ClampedArray
- Uint16Array
- Uint32Array
- Float32Array
- Float64Array
- ArrayBuffer
- Atomics
- DataView
- Map
- WeakMap
- Set
- WeakSet
- WeakRef
- 异常类
- Error
- EvalError
- RangeError
- ReferenceError
- SyntaxError
- TypeError
- URIError
- IntervalError
- 固有类
- Console
- JSON
- Math
- Reflect
- MessageChannel
- WebAssembly
- FinalizationRegistry
- issue
- CoffeeScript
- 三方库
- TypeScript
- 类型库
- lib.es5.d.ts
- 三方库
- vue
- reflect-metadata
- issue
- Browser
- 宿主库
- DOM类
- Document
- DOMImplementation
- DocumentFragment
- DOMParser
- XMLSerializer
- CustomElementRegistry
- ShadowRoot
- NamedNodeMap
- Attr
- TreeWalker
- NodeIterator
- Node
- ParentNode
- ChildNode
- Element
- DocumentType
- HTMLElement
- HTMLHtmlElement
- HTMLStyleElement
- HTMLIFrameElement
- HTMLMediaElement
- HTMLAudioElement
- HTMLVideoElement
- HTMLImageElement
- HTMLCanvasElement
- HTMLInputElement
- CharacterData
- Text
- Selection
- Range
- CSS
- CSSStyleSheet
- CSSStyleDeclaration
- MediaQueryList
- DOMMatrix
- DOMTokenList
- Animation
- KeyframeEffect
- SVG类
- SVGElement
- SVGGraphicsElement
- SVGSVGElement
- SVGGeometryElement
- 事件类
- EventTarget
- GlobalEventHandlers
- WindowEventHandlers
- Event
- CustomEvent
- UIEvent
- AnimationEvent
- TransitionEvent
- KeyboardEvent
- MouseEvent
- TouchEvent
- DragEvent
- PopStateEvent
- ClipboardEvent
- ProgressEvent
- StorageEvent
- MessageEvent
- BeforeUnloadEvent
- WebGLContextEvent
- 系统类
- WindowOrWorkerGlobalScope
- Window
- WorkerGlobalScope
- Worker
- SharedWorker
- ServiceWorkerContainer
- ServiceWorkerRegistration
- Performance
- Screen
- ScreenOrientation
- History
- Location
- Navigator
- Crypto
- Permissions
- CredentialsContainer
- PasswordCredential
- 媒体类
- TouchList
- Touch
- DataTransfer
- DataTransferItemList
- DataTransferItem
- ReadableStream
- Blob
- FileList
- File
- FileReader
- FileSystemHandle
- FileSystemFileHandle
- FileSystemDirectoryHandle
- FileSystemWritableFileStream
- FormData
- Image
- ImageData
- CanvasRenderingContext2D
- CanvasGradient
- WebGLRenderingContext
- TimeRanges
- Audio
- AudioTrack
- BaseAudioContext
- AudioContext
- AudioBuffer
- AudioNode
- AudioScheduledSourceNode
- MediaElementAudioSourceNode
- AudioBufferSourceNode
- AnalyserNode
- OscillatorNode
- ScriptProcessorNode
- MediaDevices
- MediaSource
- MediaStream
- MediaRecorder
- SpeechSynthesisUtterance
- SpeechSynthesis
- Geolocation
- BatteryManager
- Clipboard
- Bluetooth
- Notification
- 存储类
- SQLite
- indexedDB
- localStorage
- sessionStorage
- 网络类
- fetch
- Headers
- Body
- Request
- Response
- XMLHttpRequest
- XMLHttpRequestUpload
- EventSource
- WebSocket
- 工具类
- PerformanceObserver
- ResizeObserver
- MutationObserver
- IntersectionObserver
- IntersectionObserverEntry
- Intl
- RTCDataChannel
- BroadcastChannel
- MessageChannel
- MessagePort
- Sanitizer
- TextDecoder
- TextEncoder
- TextDecoderStream
- TextEncoderStream
- EyeDropper
- 平台类
- ActiveXObject
- clipboardData
- BarcodeDetector
- 三方库
- CSS
- animate
- magic.css
- frozen
- csshake
- fontawesome
- hover.css
- wickedcss
- spinkit
- bulma
- tailwindcss
- Native
- [Polyfills]
- @babel/standalone
- buble
- eventsource
- event-source-polyfill
- fastclick
- js-polyfills
- history
- html5shiv
- normalize.css
- respond
- core-js
- less
- pathseg
- modernizr
- 生态
- @antv/g6
- @ckeditor/ckeditor5-build-classic
- @docsearch/js
- @flowjs/flow.js
- @mojs/core
- @popperjs/core
- @shopify/draggable
- @toast-ui/editor
- @uppy/core
- @zip.js/zip.js
- ace-builds
- adapterjs
- adaptive-quadratic-curve
- ajax-hook
- alloyfinger
- animejs
- aplayer
- art-template
- array-move
- asciinema-player
- async-validator
- awesome-qr
- await-to-js
- axios
- better-scroll
- big.js
- canvg
- canvas-nest.js
- chai
- chance
- chart.js
- chroma-js
- clipboard
- cnchar
- cnchar-all
- cnchar-poly
- cnchar-order
- cnchar-trad
- cnchar-draw
- cnchar-idiom
- cnchar-xhy
- cnchar-radical
- codemirror
- color-js
- compressorjs
- console.table
- console-ban
- copy-to-clipboard
- countup.js
- cplayer
- create-keyframe-animation
- cropperjs
- croppr
- crunker
- crypto-js
- css-doodle
- d3
- darkmode-js
- dayjs
- deep-diff
- devtools-detect
- devtools-detector
- dom-iterator
- dot
- dom-to-image
- dplayer
- driver.js
- dropzone
- echarts
- editor.md
- element-resize-detector
- eruda
- exif-js
- fabric
- fastscan
- favicon.js
- ffmpeg.js
- file-saver
- filepond
- filesize
- flv.js
- fullpage.js
- fuse.js
- g2
- get-css-translated-position
- good-storage
- gridmanager
- gsap
- [exp]-gsap
- utils
- Tween
- hammer.js
- handsontable
- headroom.js
- highlight.js
- hls.js
- howler
- html2canvas
- humanize-string
- id3js
- image-conversion
- immer
- immutable
- interactjs
- intro.js
- iScroll
- iscroll-lite
- iscroll-probe
- iscroll-zoom
- iscroll-infinite
- jdetects
- js-audio-recorder
- js-base64
- js-cookie
- js-dos
- js-export-excel
- js-qr
- js-web-screen-shot
- jsbarcode
- jsonp
- jspdf
- jsplumb
- konva
- lazyload
- leven
- live2d
- localforage
- lodash
- lodash-es
- lorem-ipsum
- lyric-parser
- markdown-it
- marked
- mathjs
- mescroll.js
- miao-lang
- mitt
- mockjs
- moment
- monaco-editor
- move-js
- mqtt
- namedavatar
- nprogress
- numeral
- omit.js
- op-rec
- p5
- pageswitch
- paho-mqtt
- panzoom
- particles.js
- photoswipe
- phy-touch
- pixi.js
- pluralize
- plyr
- popmotion
- prettier
- premonish
- print-js
- prismjs
- pubsub-js
- qrcode
- qrcodejs2
- qs
- recorder-core
- requireJS
- rxjs
- screenfull
- scrollmagic
- scrollreveal
- seajs
- select
- simple-uploader.js
- single-spa
- smartphoto
- sortablejs
- soundjs
- SparkMD5
- spin.js
- stackblur-canvas
- stringify-object
- swiper
- systemjs
- termynal
- three
- tiny-emitter
- tinycolor2
- tinymce
- tippy.js
- toggle-selection
- touchslider
- tui-image-editor
- tween
- typed.js
- updeep
- underscore
- underscore.string
- unescape
- uuid
- uuidjs
- validator.js
- vanilla-lazyload
- vconsole
- vditor
- velocity-animate
- victor
- video.js
- viewerjs
- virtual-dom
- vivusv
- wangEditor
- wavesurfer.js
- web-highlighter
- web-storage-cache
- web-vitals
- wfplayer
- window-watcher
- wowjs
- xe-utils
- xgplayer
- xijs
- xterm
- yup
- zrender
- jQuery
- [UI]
- uikit
- amazeui
- axui
- bootstrap
- Button
- Popover
- Tooltip
- Modal
- bui
- easyui
- frozenUI
- jquerymobile
- jQuerUI
- layui
- Badge
- Breadcrumb
- Button
- Card
- Collapse
- Form
- Grid
- Layer
- Layout
- Nav
- Progress
- Tab
- Table
- Timeline
- Utils
- miniUI
- suiMobile
- weui
- ActionSheet
- Button
- Flex
- Toast
- 生态
- blueimp-file-upload
- bootstrap-table
- jCanvas
- jquery.cookie
- jquery.tmpl
- jquery-editable-select
- jquery-parallax.js
- jquery-pjax
- jquery-scrollify
- jquery-validation
- jsGrid
- jstree
- parsleyjs
- simplePagination
- WebUploader
- zepto
- zTree
- Angular
- 生态
- @angular/common
- @angular/core
- @angular/platform-browser
- @angular/platform-browser-dynamic
- @angular/router
- React
- [UI]
- @material-ui/core
- Button
- @chakra-ui/react
- @douyinfe/semi-ui
- @jetbrains/ring-ui
- bfd-ui
- react-bootstrap
- react-weui
- zarm
- 框架
- umi
- @umijs/plugin-antd
- antd
- @ant-design/icons
- @ant-design/pro-provider
- @ant-design/pro-form
- @ant-design/pro-table
- @ant-design/pro-list
- @ant-design/pro-descriptions
- @ant-design/pro-card
- antd-img-crop
- Anchor
- BackTop
- Button
- Card
- ConfigProvider
- Divider
- Dropdown
- Form
- Grid
- Layout
- Select
- Space
- Table
- Tabs
- Typography
- Menu
- Modal
- Upload
- @umijs/plugin-dva
- dva
- @umijs/plugin-helmet
- @umijs/plugin-initial-state
- @umijs/plugin-layout
- @ant-design/pro-layout
- @umijs/plugin-locale
- @umijs/plugin-model
- @umijs/plugin-qiankun
- qiankun
- @umijs/plugin-request
- umi-request
- @umijs/route-utils
- umi-plugin-antd-icon-config
- 生态
- @alitajs/dform
- @loadable/component
- ahooks
- useRequest
- amis-editor
- braft-editor
- braft-utils
- chart-race-react
- classnames
- clsx
- connected-react-router
- constate
- component-playground
- draft-js
- formik
- gg-editor
- griffith
- html-react-parser
- jsx-transform
- mobx
- mobx-react
- polished
- preact
- prism-react-renderer
- prop-types
- qrcode.react
- rc-form
- react-ace
- react-activation
- react-async-component
- react-audio-player
- react-beautiful-dnd
- react-code-view
- react-codemirror
- react-color
- react-copy-to-clipboard
- react-document-title
- react-dom
- react-dnd
- react-dnd-html5-backend
- react-draggable
- react-filepond
- react-jsx-parser
- react-highlight-words
- react-height
- react-helmet
- react-hook-form
- react-infinite-scroller
- react-input-autosize
- react-intl
- react-json-view
- react-keep-alive
- react-lazy-load-image-component
- react-lazyload
- react-list
- react-live
- react-loadable
- react-motion
- react-photo-view
- react-qrbtf
- react-query
- react-quill
- react-sortable-hoc
- react-rnd
- react-resizable
- react-router
- react-router-config
- react-router-dom
- react-router-transition
- react-scroll
- react-simple-code-editor
- react-sticky
- react-table
- react-tabs
- react-tag-input
- react-tagsinput
- react-text-loop
- react-textarea-autosize
- react-transition-group
- react-use
- react-use-hover
- react-useportal
- react-viewer-mobile
- react-virtualized
- react-window
- react-zmage
- redux
- 生态
- @reduxjs/toolkit
- reselect
- redux-immutable
- redux-logger
- redux-persist
- redux-saga
- redux-thunk
- react-redux
- slate
- slate-react
- styled-components
- swr
- use-debounce
- use-http
- use-media
- use-merge-value
- video-react
- Svelte
- 生态
- nervjs
- 生态
- ef.js
- 生态
- Vue
- [UI]
- element-ui
- el-container
- el-menu
- el-row
- el-button
- el-form
- el-table
- el-upload
- el-scrollbar
- el-collapse-transition
- vant
- van-button
- van-list
- van-nav-bar
- van-search
- mint-ui
- mt-loadmore
- cube-ui
- vuetify
- vux
- iview
- view-design
- nutui
- muse-ui
- buefy
- vonic
- mand-mobile
- vue-ydui
- wot-design
- 生态
- @chenfengyuan/vue-qrcode
- @tinymce/tinymce-vue
- awe-dnd
- carddragger
- img-vuer
- mavon-editor
- qrcode.vue
- v-charts
- v-viewer
- vue-3d-model
- vue-awesome-swiper
- vue-class-component
- vue-clipboard2
- vue-count-to
- vue-croppa
- vue-cropper
- vue-draggable-resizable
- vue-fullpage.js
- vue-js-modal
- vue-i18n
- vue-lazyload
- vue-meditor
- vue-photo-preview
- vue-property-decorator
- vue-qr
- vue-qrcode-reader
- vue-router
- vue-simple-uploader
- vue-splitpane
- vue-meta-info
- vue-video-player
- vue-virtual-scroll-list
- vuep
- vuescroll
- vuex
- vuex-class
- vuex-persist
- vuex-persistedstate
- vxe-table
- vue-cookie
- vue-core-video-player
- vuedraggable
- Vue3
- [UI]
- @arco-design/web-vue
- @varlet/ui
- element-plus
- ant-design-vue
- vant
- naive-ui
- idux
- 生态
- @cloudgeek/vue3-video-player
- @vueuse/core
- qrcode.vue
- responsive-storage
- swiper
- splitpanes
- v-contextmenu
- vue-i18n
- vue-router
- vueuse
- vuex
- vuedraggable
- vue-grid-layout
- vue-multiselect
- vue-request
- vue-virtual-scroller
- vue-web-screen-shot
- vue-drag-verify2
- issue
- NodeJS
- 宿主库
- assert
- async_hooks
- buffer
- child_process
- cluster
- console
- crypto
- dgram
- diagnostics_channel
- dns
- events
- fs
- [exp]-fs
- constants
- promises
- FileHandle
- Dir
- Dirent
- FSWatcher
- ReadStream
- WriteStream
- Stats
- http
- http2
- https
- inspector
- module
- net
- os
- path
- perf_hooks
- process
- querystring
- readline
- repl
- stream
- string_decoder
- timer
- tls
- tty
- url
- util
- v8
- vm
- wasi
- worker_threads
- zlib
- 三方库
- @antfu/install-pkg
- @serialport/parser-readline
- @vue/test-utils
- acorn
- anchorme
- ast-query
- babel
- 生态
- @babel/code-frame
- @babel/core
- @babel/generator
- @babel/parser
- @babel/template
- @babel/traverse
- @babel/types
- blitz
- body-parser
- browserslist
- canvas
- camelcase
- cdigit
- chalk
- chalk-pipe
- cheerio
- chokidar
- cli-cursor
- cli-table3
- cmd-shim
- co
- commander
- compression
- compressing
- connect
- connect-livereload
- cross-port-killer
- cross-spawn
- csv
- debug
- deep-extend
- degit
- del
- directory-tree
- dotenv
- dotenv-expand
- download
- download-git-repo
- egg
- ejs
- enzyme
- espree
- esprima
- execa
- express
- 生态
- cors
- express-graphql
- express-http-proxy
- express-session
- express-validator
- eventemitter3
- fast-glob
- favicons
- ffi
- figures
- find-up
- fkill
- follow-redirects
- form-data
- fs-extra
- glob
- glob-parent
- globby
- graphql
- handlebars
- hash-sum
- http-assert
- http-errors
- http-proxy
- http-proxy-middleware
- iconv-lite
- inquirer
- inquirer-directory
- inquirer-npm-name
- ip
- jest
- jsdom
- jsdom-global
- jshint
- json-schema
- json-server
- jws
- htmlparser2
- kind-of
- koa
- 生态
- koa-body
- koa2-cors
- koa-nunjucks-2
- koa-router
- koa-send
- koa-server-http-proxy
- koa-sse-stream
- koa-sslify
- koa-static
- koa-ts-controllers
- koa-views
- less
- log4js
- lorem-ipsum
- markdown-it
- mem-fs
- mem-fs-editor
- meow
- minimist
- mkdirp
- mocha
- mongoose
- morgan
- multer
- mysql
- mysql2
- nanoid
- nest
- next
- node-abi
- node-notifier
- node-sass
- nopt
- nunjucks
- nuxt
- opencc
- open
- ora
- os-locale
- p-limit
- passport
- path-to-regexp
- pretty-bytes
- pretty-ms
- pdfkit
- phantom
- portfinder
- postcss
- prettier
- progress
- prompt
- pug
- puppeteer
- pushstate-server
- python-shell
- qrcode
- qrcode-terminal
- react-test-renderer
- regulex
- requires-port
- request
- rpio2
- sass
- serialize-javascript
- serialport
- sequelize
- semver
- serve-static
- shelljs
- shortid
- slash
- socket.io
- sqlite3
- string
- string-format
- stringz
- stylus
- superb
- tapable
- the-answer
- through2
- touch
- undertaker
- uniq
- universalify
- utility
- v8flags
- validate-npm-package-name
- vinyl
- voca
- webpack
- lib
- rules
- Chunk
- ExternalModule
- JavascriptParser
- LorderContext
- NormalModuleFactory
- NormalModule
- Stats
- 生态
- webpack-dev-server
- webpack-dev-middleware
- webpack-sources
- schema-utils
- loader-runner
- loader-utils
- html-webpack-plugin
- ws
- xlsx
- xml2js
- yargs-parser
- yeoman-generator
- yosay
- issue
- ApiCloud
- AVM
- 宿主库
- api
- 三方库
- apijs
- DCloud
- 宿主库
- plus
- 三方库
- mui
- Cordova
- 宿主库
- cordova
- 三方库
- Ionic
- framework7
- Meteor
- 宿主库
- Weex
- 宿主库
- 三方库
- weex-ui
- ReactNative
- 宿主库
- react-native
- StyleSheet
- LogBox
- Platform
- Dimensions
- AppRegistry
- 三方库
- @ant-design/react-native
- Remax
- 宿主库
- Taro
- 宿主库
- Omi
- 宿主库
- uni-app
- 内置组件
- 宿主库
- 三方库
- [UI]
- uni-ui
- uView
- WePublic
- 宿主库
- jssdk
- issue
- WeMini
- WXML
- WXS
- 宿主库
- wx
- App
- Page
- Component
- Behavior
- wx-server-sdk
- 三方库
- minui
- mpvue
- wxDraw
- WPS
- 宿主库
- wps-jsapi
- NW.js
- 宿主库
- Electron
- 宿主库
- electron
- remote
- app
- Menu
- BrowserWindow
- BrowserView
- WebContents
- ipcMain
- ipcRenderer
- dialog
- shell
- clipboard
- globalShortcut
- protocol
- session
- 三方库
- electron-is-dev
- electron-store
- devtron
- issue
- Espruino
- 宿主库
- 三方库
- System
- Windows
- 通用命令
- appwiz.cpl
- arp.exe
- attrib.exe
- bitsadmin.exe
- calc.exe
- certlm.msc
- certmgr.msc
- charmap.exe
- chcp.exe
- chkdsk.exe
- choice
- cleanmgr.exe
- cliconfg.exe
- clip.exe
- cmd.exe
- compmgmt.msc
- control.exe
- credwiz.exe
- dcomcnfg.exe
- desk.cpl
- devmgmt.msc
- dfrg.msc
- dfrgui.exe
- dialer.exe
- diskmgmt.msc
- diskpart.exe
- dvdplay.exe
- dxdiag.exe
- eudcedit.exe
- eventvwr.exe
- explorer.exe
- fc.exe
- find.exe
- findstr.exe
- firewall.cpl
- format.exe
- fsmgmt.msc
- ftp.exe
- gpedit.msc
- hdwwiz.cpl
- icacls.exe
- iexpress.exe
- inermgr.exe
- inetcpl.cpl
- inetmgr.exe
- intl.cpl
- ipconfig.exe
- joy.cpl
- label.exe
- logoff.exe
- lpksetup.exe
- lusrmgr.msc
- main.cpl
- mip.exe
- mmc.exe
- mmsys.cpl
- mobsync.exe
- mode.exe
- more.exe
- mplayer2.exe
- msconfig.exe
- msdt.exe
- mshta.exe
- msinfo32.exe
- mspaint.exe
- msra.exe
- mstsc.exe
- narrator.exe
- ncpa.cpl
- net.exe
- netplwiz.exe
- netsh.exe
- netstat.exe
- notepad.exe
- nslookup.exe
- nvidia-smi.exe
- odbcad32.exe
- optionalfeatures.exe
- osk.exe
- perfmon.msc
- perfmon.exe
- ping.exe
- powercfg.cpl
- powershell.exe
- print.exe
- printmanagement.msc
- psr.exe
- regedit.exe
- rasphone.exe
- regsvr32.exe
- resmon.exe
- robocopy.exe
- route.exe
- rsop.msc
- runas.exe
- rundll32.exe
- sc.exe
- schtasks.exe
- secpol.msc
- services.msc
- sfc.exe
- shrpubw.exe
- shutdown.exe
- slmgr.vbs
- snippingtool.exe
- soundrecorder.exe
- ssh.exe
- stikynot.exe
- subst.exe
- sysdm.cpl
- syskey.exe
- systeminfo.exe
- taskkill.exe
- tasklist.exe
- taskmgr.exe
- taskschd.msc
- telnet.exe
- timedate.cpl
- tracert.exe
- tree.exe
- utilman.exe
- wf.msc
- wfs.exe
- where.exe
- whoami.exe
- wiaacmgr.exe
- winver.exe
- wmimgmt.msc
- write.exe
- wscript.exe
- wscui.cpl
- wuapp.exe
- xcopy.exe
- 注册表项
- HKEY_CLASSES_ROOT
- HKEY_CURRENT_USER
- HKEY_LOCAL_MACHINE
- HKEY_USERS
- HKEY_CURRENT_CONFIG
- issue
- 系统驱动
- accessDatabaseEngine
- dxwebsetup
- font
- 类库文件
- advapi32.dll
- comdlg32.dll
- credui.dll
- gdi32.dll
- kernel32.dll
- msvcrt.dll
- netapi32.dll
- ntdll.dll
- shcore.dll
- shell32.dll
- shlwapi.dll
- user32.dll
- winmm.dll
- winspool.drv
- 软件应用
- adb
- 指令指南
- another-redis-desktop-manager
- 软件配置
- apache2
- 指令指南
- 软件配置
- Core
- 软件配置
- mod_so
- 软件配置
- mod_mime
- 软件配置
- mod_rewrite
- 软件配置
- mod_authz_core
- 软件配置
- mod_access_compat
- 软件配置
- mod_negotiation
- 软件配置
- issue
- apifox
- 指南指南
- apktool
- 指令指南
- apicloudstudio2
- 指令指南
- 软件配置
- aria2
- 指令指南
- ascgen2
- 指令指南
- axurepr
- 指令指南
- balenaEtcher
- 指令指南
- bartender
- 指令指南
- burpsuite
- 指令指南
- ccproxy
- 指令指南
- charles-proxy
- 指令指南
- cheatEngine
- 指令指南
- choco
- 指令指南
- chipeasy
- 指令指南
- chrome
- 指令指南
- cisco packet tracer
- 指令指南
- cmake
- 指令指南
- 软件配置
- cmder
- 指令指南
- 软件配置
- C-C++
- conan
- 指令指南
- 软件配置
- cpu-z
- 指令指南
- CSharp
- msbuild
- 软件配置
- nuget
- 指令指南
- dotnet
- 指令指南
- IronPython
- cura
- 指令指南
- Dart
- 指令指南
- flutter
- data-retriever
- 指令指南
- dbeaver
- 指令指南
- deskPins
- 软件配置
- dex2jar
- 指令指南
- discuzX
- 软件配置
- disk genius
- 指令指南
- dosbox
- 指令指南
- easyBCD
- 指令指南
- eDEX-UI
- 指令指南
- emq
- 指令指南
- 软件配置
- eNSP
- 指令指南
- Erlang
- 指令指南
- rebar
- 指令指南
- 软件配置
- etcher
- 指令指南
- emu8086
- 指令指南
- everything
- 指令指南
- exe4j
- 指令指南
- fastgithub
- 指令指南
- ffmpeg
- 指令指南
- fiddler
- 指令指南
- filezilla
- 软件配置
- finalshell
- 指令指南
- foxmail
- 软件配置
- fontCreator
- 软件配置
- fscapture
- 指令指南
- git
- 指令指南
- 软件配置
- git-flow
- 指令指南
- git-lfs
- 指令指南
- issue
- gimp
- 软件配置
- gitblit
- 软件配置
- Go
- 指令指南
- gogs
- 软件配置
- issue
- gpu-z
- 指令指南
- graphviz
- 指令指南
- hedit
- 指令指南
- hex
- 指令指南
- hex-editor
- 指令指南
- hexchat
- 软件配置
- imageMagick
- 指令指南
- Java
- 指令指南
- ant
- 指令指南
- 软件配置
- maven
- 指令指南
- 软件配置
- maven-jetty-plugin
- tomcat7-maven-plugin
- mybatis-generator-maven-plugin
- spring-boot-maven-plugin
- gradle
- 指令指南
- 软件配置
- Groovy
- 指令指南
- issue
- jd-gui
- 指令指南
- jenkins
- 指令指南
- jetbrains
- 指令指南
- 软件配置
- AndroidStudio
- Intellij
- CLion
- plugin
- koala
- 指令指南
- listdlls
- 指令指南
- installshield
- 指令指南
- m3u8DL
- 指令指南
- masm
- 指令指南
- memcached
- 指令指南
- microsoft office
- 指令指南
- miniconda
- 指令指南
- mongodb
- 指令指南
- mqttx
- 指令指南
- msys2
- 指令指南
- base-devel
- pkg-config
- mingw-w64-x86_64-toolchain
- mingw-w64-x86_64-python3-gobject
- mingw-w64-x86_64-glade
- mingw-w64-x86_64-gtk3
- mingw-w64-x86_64-adwaita-icon-theme
- mingw-w64-x86_64-hicolor-icon-theme
- mysql
- 软件配置
- 指令指南
- navicat
- 指令指南
- netassist
- 指令指南
- nexus3
- 指令指南
- nrfconnect
- 指令指南
- nginx
- 指令指南
- 软件配置
- ngx_http_core_module
- 软件配置
- ngx_http_map_module
- 软件配置
- ngx_http_log_module
- 软件配置
- ngx_http_ssl_module
- 软件配置
- ngx_http_charset_module
- 软件配置
- ngx_http_headers_module
- 软件配置
- ngx_http_index_module
- 软件配置
- ngx_http_autoindex_module
- 软件配置
- ngx_http_rewrite_module
- 软件配置
- ngx_http_proxy_module
- 软件配置
- ngx_http_uwsgi_module
- 软件配置
- ngx_http_upstream_module
- 软件配置
- ngx_http_api_module
- 软件配置
- ngx_http_lua_module
- 软件配置
- nginx-rtmp-module
- 软件配置
- nginx-upload-module
- 软件配置
- issue
- ninja
- 指令指南
- 软件配置
- NodeJS
- 指令指南
- @angular/cli
- 指令指南
- @antfu/ni
- 指令指南
- @babel/core
- 指令指南
- 软件配置
- core-js
- regenerator-runtime
- @babel/polyfill
- @babel/runtime
- @babel/runtime-corejs2
- @babel/runtime-corejs3
- @babel/plugin-proposal-class-properties
- @babel/plugin-proposal-decorators
- @babel/plugin-transform-modules-commonjs
- @babel/plugin-transform-runtime
- @babel/plugin-transform-typescript
- @babel/plugin-transform-react-jsx
- @babel/plugin-syntax-jsx
- babel-plugin-import
- babel-plugin-component
- babel-plugin-transform-remove-console
- babel-plugin-dynamic-import-node
- babel-plugin-syntax-jsx
- babel-plugin-transform-react-jsx
- babel-plugin-transform-vue-jsx
- @babel/preset-env
- @babel/preset-typescript
- @babel/preset-react
- @vue/babel-helper-vue-jsx-merge-props
- @vue/babel-plugin-jsx
- @vue/babel-plugin-transform-vue-jsx
- @vue/babel-preset-app
- issue
- @commitlint/cli
- 指令指南
- 软件配置
- @commitlint/config-conventional
- @docusaurus/init
- 指令指南
- 软件配置
- @ionic/cli
- 指令指南
- @nestjs/cli
- 指令指南
- @tarojs/cli
- 指令指南
- @umijs/create-umi-app
- 指令指南
- 软件配置
- @umijs/preset-ui
- @umijs/preset-react
- @umijs/preset-dumi
- @vue/cli
- 指令指南
- 软件配置
- @vue/cli-service
- 指令指南
- @vue/cli-service-global
- 指令指南
- @vue/cli-plugin-babel
- 指令指南
- @vue/cli-plugin-eslint
- 指令指南
- @vue/cli-plugin-router
- @vue/cli-plugin-vuex
- @vue/cli-plugin-typescript
- @vue/cli-plugin-unit-jest
- vue-cli-plugin-electron-builder
- vue-cli-plugin-element
- @vue/compiler-sfc
- apidoc
- 指令指南
- bower
- 指令指南
- browserify
- 指令指南
- browsersync
- 指令指南
- budo
- 指令指南
- caz
- 指令指南
- concurrently
- 指令指南
- cordova
- 指令指南
- 软件配置
- cordova-res
- create-html5-boilerplate
- 指令指南
- creat-react-app
- 指令指南
- babel-preset-react-app
- react-dev-utils
- craco
- 软件配置
- customize-cra
- 软件配置
- http-proxy-middleware
- 软件配置
- react-app-rewired
- 软件配置
- react-app-rewire-hot-loader
- issue
- create-next-app
- 指令指南
- create-umi
- 指令指南
- umi-serve
- create-vite
- 指令指南
- vite
- @vitejs/plugin-vue
- @vitejs/plugin-react
- @vitejs/plugin-vue-jsx
- @vitejs/plugin-legacy
- vite-plugin-element-plus
- vite-plugin-babel-import
- vite-plugin-imp
- vite-plugin-mock
- vite-plugin-restart
- vite-plugin-svg-icons
- unplugin-auto-import
- unplugin-vue-components
- check-for-leaks
- 指令指南
- copyfiles
- 指令指南
- corepack
- 指令指南
- cross-env
- 指令指南
- degit
- 指令指南
- docsify-cli
- 指令指南
- download-cli
- 指令指南
- dva-cli
- 指令指南
- electron
- 指令指南
- electron-rebuild
- 指令指南
- electron-builder
- 指令指南
- 软件配置
- electron-packager
- 指令指南
- 软件配置
- ember-cli
- 指令指南
- esbuild
- 指令指南
- esbuild-wasm
- eslint
- 指令指南
- 软件配置
- babel-eslint
- eslint-formatter-pretty
- @typescript-eslint/parser
- @babel/eslint-parser
- @typescript-eslint/eslint-plugin
- eslint-plugin-vue
- eslint-plugin-react
- eslint-plugin-react-hooks
- eslint-plugin-import
- eslint-plugin-unicorn
- eslint-plugin-promise
- eslint-plugin-babel
- eslint-plugin-jest
- @vue/eslint-config-airbnb
- @vue/eslint-config-typescript
- eslint-config-airbnb-base
- eslint-config-google
- eslint-config-prettier
- express-generator
- 指令指南
- father
- 指令指南
- framework7-cli
- 指令指南
- fuzhi
- 指令指南
- gatsby-cli
- 指令指南
- ghost-cli
- 指令指南
- grunt
- 指令指南
- 软件配置
- grunt-cli
- grunt-init
- load-grunt-tasks
- load-grunt-configs
- time-grunt
- grunt-contrib-clean
- grunt-contrib-copy
- grunt-contrib-requirejs
- grunt-contrib-watch
- grunt-contrib-jshint
- grunt-contrib-nodeunit
- grunt-contrib-concat
- grunt-contrib-uglify
- grunt-contrib-cssmin
- grunt-contrib-htmlmin
- grunt-contrib-imagemin
- grunt-contrib-connect
- grunt-contrib-compass
- grunt-contrib-sass
- grunt-contrib-less
- grunt-wiredep
- grunt-concurrent
- grunt-autoprefixer
- grunt-newer
- grunt-webpack
- grunt-postcss
- grunt-ejs-render
- grunt-premailer
- grunt-preprocess
- grunt-bootlint
- gulp
- 指令指南
- 软件配置
- gulp-cli
- gulp-concat
- gulp-load-plugins
- gulp-replace
- gulp-shell
- hexo-cli
- 指令指南
- http-server
- 指令指南
- husky
- 指令指南
- 软件配置
- jest
- 指令指南
- 软件配置
- jsdoc
- 指令指南
- jsdoc-to-markdown
- 指令指南
- json-server
- 指令指南
- 软件配置
- jsonlint
- 指令指南
- lerna
- 指令指南
- 软件配置
- less
- 指令指南
- less-plugin-autoprefix
- less-plugin-est
- lesshat
- less-plugin-clean-css
- lint-staged
- 指令指南
- live-server
- 指令指南
- meteor
- 指令指南
- mocha
- 指令指南
- mrm
- 指令指南
- nexe
- 指令指南
- node-gyp
- 指令指南
- nodemon
- 指令指南
- 软件配置
- npe
- 指令指南
- npm
- 指令指南
- 软件配置
- np
- 指令指南
- npm-check
- 指令指南
- npm-run-all
- 指令指南
- npkill
- 指令指南
- nrm
- 指令指南
- nvm
- 指令指南
- 软件配置
- parcel
- 指令指南
- 软件配置
- @parcel/config-default
- @parcel/transformer-raw
- @parcel/transformer-typescript-tsc
- @parcel/resolver-glob
- phantomjs
- 指令指南
- plop
- 指令指南
- 软件配置
- pm2
- 指令指南
- 软件配置
- pnpm
- 指令指南
- postcss
- 指令指南
- postcss-cli
- postcss-comment
- postcss-preset-env
- postcss-px-to-viewport
- postcss-pxtore
- autoprefixer
- postcss-import
- issue
- prettier
- 指令指南
- 软件配置
- pug-cli
- 指令指南
- react-native
- 指令指南
- issue
- rimraf
- 指令指南
- rjs
- 指令指南
- 软件配置
- roadhog
- 软件配置
- rollup
- 指令指南
- 软件配置
- @rollup/plugin-babel
- @rollup/plugin-json
- @rollup/plugin-node-resolve
- @rollup/plugin-commonjs
- @rollup/plugin-typescript
- @rollup/plugin-replace
- rollup-plugin-babel
- rollup-plugin-json
- rollup-plugin-node-resolve
- rollup-plugin-commonjs
- rollup-plugin-eslint
- rollup-plugin-replace
- rollup-plugin-uglify
- rollup-plugin-terser
- rollup-plugin-vue
- rollup-plugin-postcss
- runjs
- 指令指南
- sass
- 指令指南
- mathsass
- serve
- 指令指南
- stylelint
- 指令指南
- 软件配置
- stylelint-config-standard
- stylelint-config-idiomatic-order
- stylelint-config-css-modules
- stylelint-config-recommended-scss
- stylelint-config-prettier
- stylelint-order
- stylelint-declaration-block-no-ignored-properties
- stylus
- 指令指南
- surge
- 指令指南
- svgo
- 指令指南
- 软件配置
- tailwindcss
- 指令指南
- 软件配置
- terser
- 指令指南
- 软件配置
- touch
- 指令指南
- typescript
- 指令指南
- 软件配置
- ts-node
- ts-node-dev
- uglify-js
- 指令指南
- unplugin
- 软件配置
- vuepress
- 指令指南
- wait-on
- 指令指南
- webpack
- 指令指南
- 软件配置
- webpack-cli
- webpack-dev-server
- webpack-merge
- webpack-chain
- webpackbar
- webpack-node-externals
- ProfilingPlugin
- IgnorePlugin
- DefinePlugin
- ProvidePlugin
- HotModuleReplacementPlugin
- webpack-bundle-analyzer
- windicss-webpack-plugin
- common-config-webpack-plugin
- friendly-errors-webpack-plugin
- speed-measure-webpack-plugin
- antd-dayjs-webpack-plugin
- uglifyjs-webpack-plugin
- clean-webpack-plugin
- copy-webpack-plugin
- html-webpack-plugin
- page-skeleton-webpack-plugin
- add-asset-html-cdn-webpack-plugin
- html-webpack-inline-source-plugin
- script-ext-html-webpack-plugin
- favicons-webpack-plugin
- mini-css-extract-plugin
- optimize-css-assets-webpack-plugin
- terser-webpack-plugin
- preload-webpack-plugin
- prerender-spa-plugin
- sw-precache-webpack-plugin
- workbox-webpack-plugin
- pnp-webpack-plugin
- extract-text-webpack-plugin
- react-hot-loader
- @hot-loader/react-dom
- vue-loader
- @svgr/webpack
- svg-sprite-loader
- expose-loader
- exports-loader
- script-loader
- raw-loader
- url-loader
- file-loader
- babel-loader
- eslint-loader
- html-loader
- markdown-loader
- isomorphic-style-loader
- style-loader
- css-loader
- postcss-loader
- sass-loader
- less-loader
- thread-loader
- ts-loader
- issue
- weex-toolkit
- 指令指南
- wpsjs
- 指令指南
- yarn
- 指令指南
- yo
- 指令指南
- generator-generator
- generator-fountain-webapp
- yorkie
- 指令指南
- nox
- 指令指南
- nmap
- 指令指南
- nsis
- 指令指南
- nssm
- 指令指南
- oleview
- 指令指南
- osfmount
- 指令指南
- PHP
- 指令指南
- 软件配置
- composer
- 指令指南
- wordpress
- 软件配置
- xampp-windows
- 指令指南
- wampserver
- 指令指南
- pinginfoview
- 软件配置
- postman
- 指令指南
- putty
- 软件配置
- Python
- 指令指南
- alembic
- 指令指南
- apistar
- 指令指南
- compileall
- 指令指南
- cookiecutter
- 指令指南
- flower
- 指令指南
- frida-tools
- 指令指南
- httpie
- 指令指南
- ipython
- 指令指南
- isort
- 指令指南
- jupyter
- 指令指南
- IronPython
- 指令指南
- memory_profiler
- 指令指南
- mitmproxy
- 指令指南
- mypy
- 指令指南
- pdb
- 指令指南
- pdfminer.six
- 指令指南
- pyLauncher
- 指令指南
- pyLoris
- 指令指南
- pip-autoremove
- 指令指南
- pip-tools
- 指令指南
- pip
- 指令指南
- pipenv
- 指令指南
- 软件配置
- poetry
- 指令指南
- pyflow
- 指令指南
- pynsist
- 软件配置
- py2exe
- 软件配置
- pyenv
- 指令指南
- pydoc
- 指令指南
- pyinstaller
- 指令指南
- 软件配置
- pypiserver
- 指令指南
- roapi-http
- 指令指南
- setuptools
- 指令指南
- 软件配置
- sphinx
- 指令指南
- 软件配置
- sphinx-autobuild
- sphinx_rtd_theme
- furo
- sqlacodegen
- 指令指南
- sqlmap
- 指令指南
- twine
- 指令指南
- 软件配置
- virtualenv
- 指令指南
- viztracer
- 指令指南
- Ruby
- 指令指南
- rdm
- 指令指南
- rufus
- 指令指南
- saneTwain
- 指令指南
- sdformatter
- 软件配置
- snipaste
- 指令指南
- sourceTree
- 指令指南
- spy++
- 指令指南
- sqlite
- 指令指南
- sqlserver
- 软件配置
- sscom32
- 指令指南
- subversion
- 指令指南
- switchhosts
- 软件配置
- TCL
- 指令指南
- teleport-ultra
- 指令指南
- tesseract-ocr
- 指令指南
- tomcat
- 指令指南
- 软件配置
- issue
- tortoiseGit
- 指令指南
- typora
- 指令指南
- uibot
- 指令指南
- unetbootin
- 指令指南
- upx
- 指令指南
- usbview
- 指令指南
- utools
- 指令指南
- vagrant
- 指令指南
- 软件配置
- virtualBox
- 软件配置
- issue
- visualstudio
- 指令指南
- issue
- vlc
- 软件配置
- vmware
- 指令指南
- vnc-Viewer
- 指令指南
- vscode
- 指令指南
- 软件配置
- issue
- win32diskimager
- 软件配置
- winSCP
- 指令指南
- wireshark
- 指令指南
- xftp
- 指令指南
- xiaowan
- 指令指南
- xmind
- 指令指南
- xshell
- 指令指南
- zadig
- 指令指南
- zookeeper
- 指令指南
- 软件配置
- 吾爱破解专用版Ollydbg
- 指令指南
- Windows10
- 软件应用
- docker
- 指令指南
- issue
- ext2fsd
- 软件配置
- wsl
- 指令指南
- 软件配置
- openSSH
- 指令指南
- issue
- Windows2019
- issue
- issue
- Linux
- 文件描述
- dev
- opt
- 通用命令
- adduser
- alias
- arch
- awk
- bash
- blkid
- cat
- cd
- chmod
- chown
- col
- compgen
- crontab
- curl
- cut
- date
- declare
- df
- dig
- dmesg
- du
- ethtool
- exec
- export
- eval
- fdisk
- fg
- file
- free
- gpasswd
- grep
- groupadd
- groupdel
- groups
- head
- history
- hostname
- hostnamectl
- id
- ifconfig
- ip
- jobs
- kill
- killall
- last
- ld
- ldd
- less
- ln
- locate
- ls
- lscpu
- lshw
- lsof
- man
- mkdir
- more
- mount
- mv
- netcat
- netplan
- netstat
- newgrp
- nm
- nohup
- passwd
- ping
- ps
- ps1
- pstree
- pwd
- pv
- readelf
- reboot
- rsync
- rm
- route
- scp
- sed
- set
- sort
- source
- su
- systemd
- systemd-analyze
- tac
- tail
- tar
- tcpdump
- tee
- top
- touch
- tr
- type
- tzselect
- ulimit
- uname
- uniq
- unset
- useradd
- userdel
- usermod
- vim
- w
- wc
- wget
- whereis
- which
- who
- xargs
- xclip
- xgettext
- 类库文件
- libsqlite3-dev
- libtool
- 软件应用
- gogs
- 软件配置
- pkg-config
- 指令指南
- Centos
- 文件描述
- etc
- lib
- 通用命令
- alternatives
- chkconfig
- dnf
- firewalld
- firewall-cmd
- getenforce
- nmcli
- pkill
- rpm
- semanage
- setenforce
- systemctl
- yum
- 软件应用
- centos-release-scl
- 指令指南
- cmake
- 指令指南
- cyrus-sasl
- 指令指南
- docker
- 指令指南
- 软件配置
- docker-compose
- 指令指南
- 软件配置
- issue
- dovecot
- 软件配置
- emqx
- 指令指南
- epel-release
- 指令指南
- gcc
- 指令指南
- gdb
- 指令指南
- haproxy
- 指令指南
- iputils
- 指令指南
- Java
- 指令指南
- issue
- keepalived
- 指令指南
- 软件配置
- net-tools
- 指令指南
- nginx
- 指令指南
- 软件配置
- postgresql
- 指令指南
- 软件配置
- NodeJS
- 指令指南
- nvm
- postfix
- 指令指南
- 软件配置
- portainer
- 指令指南
- psmisc
- 指令指南
- Python
- pip3
- jupyter
- redis
- 指令指南
- 软件配置
- sonar
- 软件配置
- texinfo
- 指令指南
- mysql
- 指令指南
- 软件配置
- issue
- yum-utils
- 指令指南
- zookeeper
- 软件配置
- issue
- Debian
- 文件描述
- ~
- etc
- lib
- proc
- root
- 通用命令
- apt-get
- dpkg-reconfigure
- hciconfig
- hcitool
- iptables
- lsusb
- service
- systemctl
- ufw
- update-alternatives
- update-rc.d
- 软件应用
- aList
- 指令指南
- auto-apt
- 指令指南
- autoconf
- 指令指南
- automake
- 指令指南
- autossh
- 指令指南
- baota
- 指令指南
- build-essential
- 指令指南
- checkInstall
- 指令指南
- dovecot
- 软件配置
- dovecot-core
- dovecot-imapd
- dovecot-pop3d
- dos2unix
- 指令指南
- freetype
- 指令指南
- frp
- 指令指南
- 软件配置
- Java
- 指令指南
- issue
- gcc
- 指令指南
- gdb
- 指令指南
- git
- 指令指南
- git-flow
- 指令指南
- issue
- gitblit
- 软件配置
- gitlab
- 指令指南
- issue
- glibc-devel
- 指令指南
- iproute2
- 指令指南
- mailx
- 指令指南
- 软件配置
- make
- 指令指南
- mercurial
- 指令指南
- memcached
- 指令指南
- mongodb
- 指令指南
- 软件配置
- issue
- mysql
- 指令指南
- 软件配置
- issue
- mysqldump
- 指令指南
- net-tools
- 指令指南
- nextCloud
- 软件配置
- NodeJS
- 指令指南
- n
- npm
- openssh-server
- 指令指南
- openssh-client
- 指令指南
- 软件配置
- openssl
- 指令指南
- postfix
- 指令指南
- 软件配置
- privoxy
- 指令指南
- prometheus
- 指令指南
- Python
- 指令指南
- asciinema
- 指令指南
- gunicorn
- 指令指南
- 软件配置
- hypercorn
- 指令指南
- p
- 指令指南
- pip3
- 指令指南
- python3-tk
- 指令指南
- supervisor
- 指令指南
- 软件配置
- virtualenv
- 指令指南
- uwsgi
- 指令指南
- 软件配置
- uvicorn
- 指令指南
- rap2-delos
- 指令指南
- realvnc
- 指令指南
- 软件配置
- redis
- 指令指南
- samba
- 指令指南
- smbclient
- sensors
- 指令指南
- software-properties-common
- 指令指南
- tinyproxy
- 指令指南
- 软件配置
- unixbench
- 指令指南
- vsftpd
- 指令指南
- xsel
- 指令指南
- zabbix
- 指令指南
- issue
- Ubuntu
- issue
- Raspbian
- 文件描述
- boot
- dev
- etc
- proc
- 通用命令
- gpio
- raspi-config
- xrandr
- sdptool
- 软件应用
- bluetoothctl
- 指令指南
- CSharp
- 指令指南
- cups
- 指令指南
- 软件配置
- issue
- i2cTools
- 指令指南
- lcd
- 指令指南
- issue
- lirc
- 指令指南
- minicom
- 指令指南
- nginx
- 软件配置
- issue
- NodeJS
- nvm
- electron
- issue
- PHP
- php-fpm
- picocom
- 指令指南
- Python3
- pip3
- raspistill
- 指令指南
- sane
- 指令指南
- 软件配置
- issue
- xgcom
- 指令指南
- issue
- Kali
- 软件应用
- fcitx
- 软件配置
- fcitx-googlepinyin
- mtr
- 指令指南
- issue
- Synology
- issue
- issue
- MacOS
- 文件描述
- Applications
- Library
- Users
- 通用命令
- codesign
- fc-list
- lsof
- open
- spctl
- xattr
- 软件应用
- fastgithub
- 指令指南
- fig
- 指令指南
- hbuilderX
- 指令指南
- homebrew
- 指令指南
- issue
- iTerm2
- 指令指南
- mkcert
- 指令指南
- oh-my-zsh
- 指令指南
- PHP
- 软件配置
- php-fpm
- 指令指南
- phpize
- 指令指南
- Ruby
- 指令指南
- gem
- 指令指南
- rbenv
- 指令指南
- Rust
- 软件配置
- rustup
- 指令指南
- cargo
- 指令指南
- vscode
- 指令指南
- xcode
- 指令指南
- 访达
- 指令指南
- issue
- Database
- sqlite
- 系统变量
- 系统函数
- issue
- mysql
- 系统变量
- 系统函数
- issue
- postgresql
- 系统变量
- 系统函数
- issue
- sqlserver
- 系统变量
- 系统函数
- issue
- mongodb
- issue
- memcached
- issue
- redis
- issue
- mqtt
- issue
- kafka
- issue
- rabbitmq
- issue
- rocketmq
- issue
- msmq
- issue
- Electronic
- standard
- HID
- IR
- UART
- USB
- UHF
- BLUETOOTH
- IC
- 74HC595
- L293D
- NE555
- module
- CP2102
- HC-05
- UPS18650
- Hilt
- Science
- Application
- 计算机图形学
- 编译原理
- 算法导论
- 校验加密
- 排序算法
- 设计模式
- 编码解析
- 消息队列
- 邮件收发
- 远程窗口
- 文件共享
- 视频推流
- 网站相关
- Presentation
- Session
- Transfer
- Network
- DataLink
- Physical
- 微机原理与接口技术
- Mathematics
- 高等代数
- 线性代数
- 三角函数
- 常微分方程
- 偏微分方程
- 复变函数
- 实分析
- 实变函数
- 微分几何
- 现世代数
- 泛函分析
- 数学分析
- 实用工具
- 对数
- Physics
- 力学
- 声学
- Ornament
- 工具
- 资源
- 接口
- Index