## [List](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-list/index.html#list)接口
[TOC]
### List接口简介
List接口继承自Collection接口,是单列集合的一个重要分支,习惯性地会将实现List接口的对象称为List集合。在**List集合中允许出现重复的元素,所有的元素是以一种线性方式存储的,在程序中可以通过索引来访问集合中的指定元素**。另外,**List集合还有一个特点就是元素有序,即元素的存入顺序和取出顺序一致**。
在Kotlin中,List分为可变集合MutableList(Read&Write,Mutable)和不可变集合List(ReadOnly,Immutable)。
* 可变集合MutableList可以对集合中的元素进行增加和删除的操作,
* 不可变集合List对集合中的元素仅提供只读操作。
* 可参考,下一节的[list集合4种创建方式](https://www.kancloud.cn/alex_wsc/android_kotlin/1037817#list4_7)
**在开发过程中,尽可能多用不可变集合List,这样可以在一定程度上提高内存效率**。
#### List接口的继承者-----([Inheritors](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-list/index.html#inheritors))
* [AbstractList](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-abstract-list/index.html#abstractlist)
```
abstract class AbstractList<out E> :
AbstractCollection<E>,
List<E>
```
* [MutableList](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-mutable-list/index.html)
```
interface MutableList<E> : List<E>, MutableCollection<E>
```
### 不可变[List](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-list/index.html#list)
在Kotlin中,不可变List是一个只读操作的集合,只有size属性和get()函数。与Java类似,它继承自Collection进而继承自Iterable。**不可变List是通过listOf()函数创建的,该函数可以创建空的List,也可以创建包含一个或多个元素的List**。示例代码如下:
```
val mList: List<Int> = listOf() //创建空的List
val mList: List<Int> = listOf(0) //创建包含一个元素的List
val mList: List<Int> = listOf(1, 2, 3, 4, 5) //创建包含多个元素的List
```
在上述代码中,创建了3个Int类型的List集合,该集合可以不存放元素,也可以直接存放相应的元素。List集合还可以进行以下几种操作。
#### 查询操作
List集合的查询操作主要有判断集合是否为空,获取集合中元素的个数以及返回集合中的元素的迭代器。为此,Kotlin提供了一系列方法,如表所示

#### 批量操作
在List集合中,经常会判断一个集合中是否包含某个集合,为此,Kotlin提供了一个`contains All(elements:Collection<@UnsafeVariance E>)`方法。
#### 检索操作
List集合中的检索操作主要有查询集合中某个位置的元素、返回集合中指定元素首次出现的索引、返回指定元素最后一次出现的索引以及返回集合中指定的索引之间的集合。Kotlin中检索操作的方法如表所示。
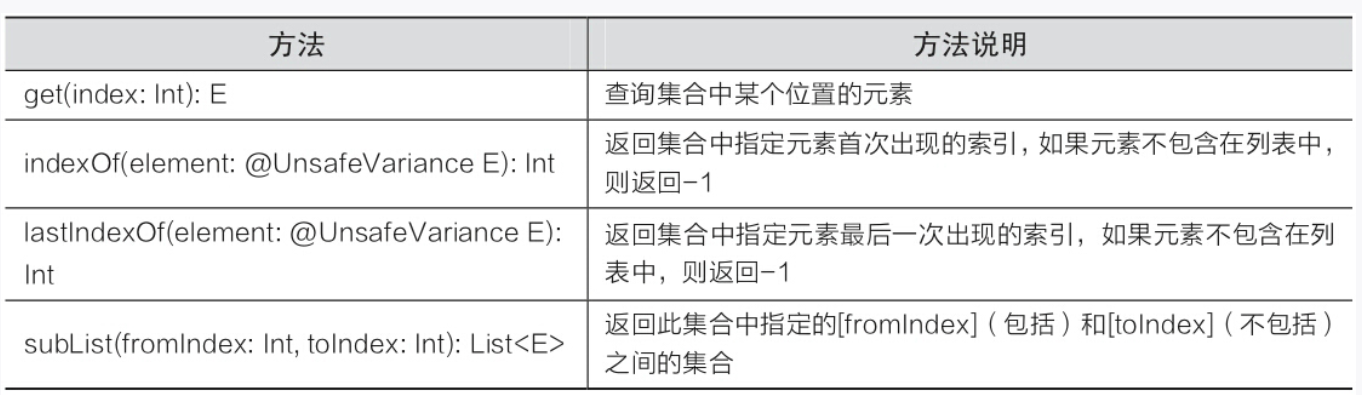
#### 遍历操作
List集合中的遍历操作主要有返回一个集合的迭代器,以及从指定位置开始返回集合的迭代器。获取迭代器的相关方法如表所示。

### 可变[MutableList](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-mutable-list/index.html#mutablelist)接口
```
interface MutableList<E> : List<E>, MutableCollection<E>
```
MutableList是List集合中的可变集合,MutableList<E>接口继承于List<E>接口和Mutable Collection<E>接口,增加了对集合中元素的添加及删除的操作。可变MutableList集合是使用mutableListOf()函数来创建对象的,具体代码如下:
```
val muList: MutableList<Int> = mutableListOf(1, 2, 3)
```
上述代码中创建了一个可变的List集合,这个集合与不可变集合的操作类似,具体如下。
>[info]注意:MutableList接口继承自List接口,所以上面List接口的方法也适用于MutableList接口,具体可查看官网[MutableList](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-mutable-list/index.html#mutablelist)
#### 查询操作
MutableList集合的查询操作与List集合一样,主要有判断集合是否为空、获取集合中元素的数量以及返回集合中的元素的迭代器,相关方法如表所示。
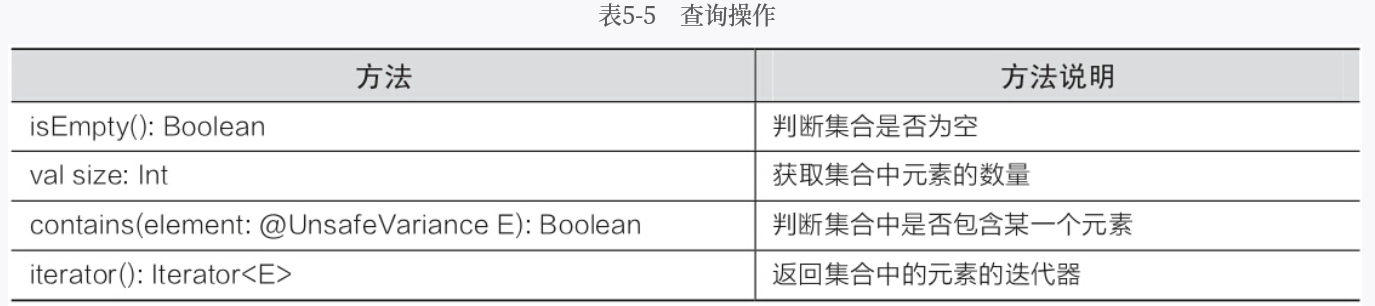
#### 修改操作
MutableList集合可以对该集合中的元素进行修改操作,这些修改操作主要有向集合中添加一个元素、在指定位置添加元素、移除一个元素、移除指定位置的元素以及替换指定位置的元素,相关方法如表所示
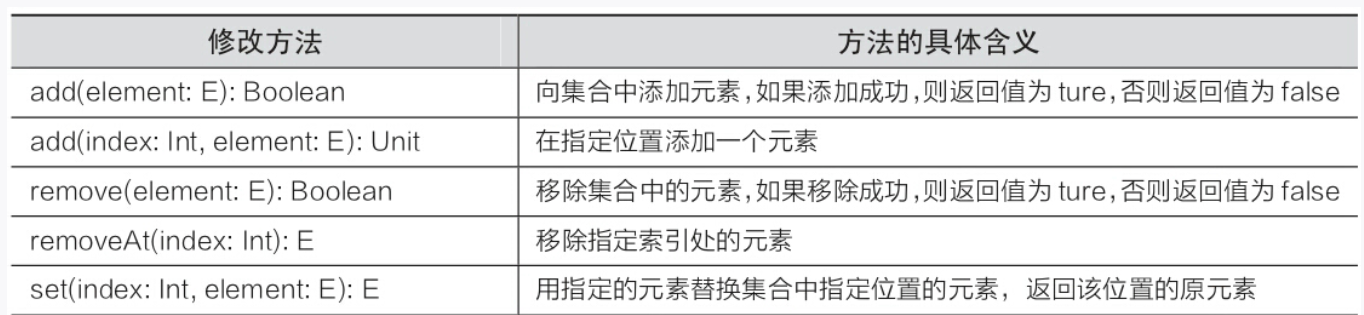
#### 批量操作
MutableList集合的批量操作主要有判断集合中是否包含另一个集合、向集合中添加一个集合、移除集合中的一个集合以及将集合中的所有元素清空,相关方法如表所示。
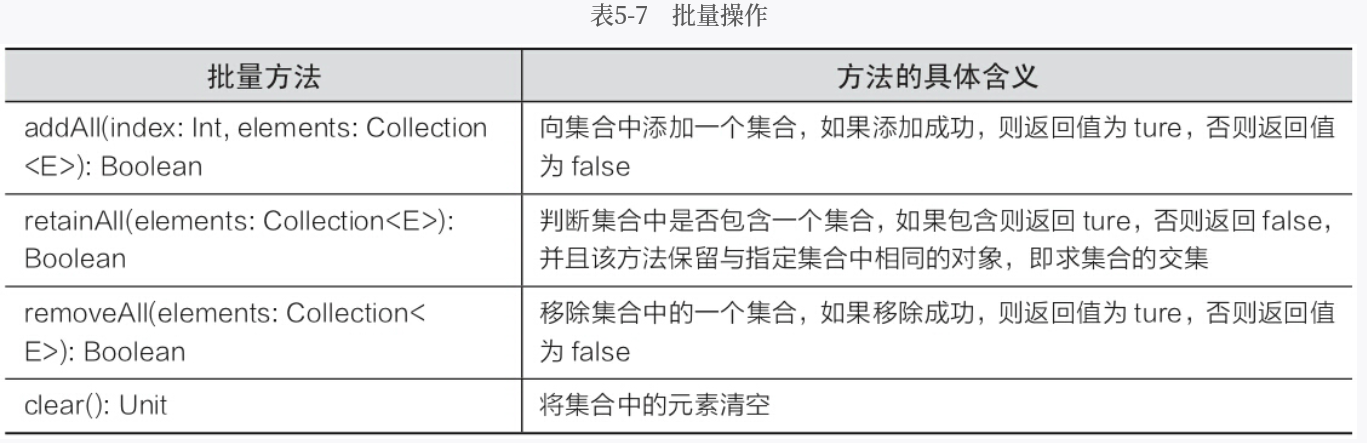
#### 遍历操作
MutableList集合中的遍历操作主要有返回一个集合的迭代器与返回从指定位置开始的集合的迭代器,如表所示。

### MutableList的继承者-----[(Inheritors)](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-mutable-list/index.html#inheritors)
从源码或者[MutableList](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-mutable-list/index.html#mutablelist),知道MutableList的继承者有抽象类[AbstractMutableList](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-abstract-mutable-list/index.html)和[ArrayList](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-array-list/index.html#arraylist)
#### [AbstractMutableList](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-abstract-mutable-list/index.html)
```
abstract class AbstractMutableList<E> : MutableList<E>//kotlin 1.2
```
它的继承者[(Inheritors)](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-abstract-mutable-list/index.html#inheritors)是[ArrayList](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-array-list/index.html#arraylist),不过这是kotlin1.1时(适用于js)的,kotlin1.3时,ArrayList的继承框架结构就变成下面的结构了。详情可查看官方网站[ArrayList](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-array-list/index.html#arraylist)
#### [ArrayList](https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-array-list/index.html#arraylist)
```
//kotlin 1.3
class ArrayList<E> :
MutableList<E>,
RandomAccess,
AbstractMutableCollection<E>
```
可以看出:类ArrayList,实现了MutableList接口和RandomAccess接口,而且还继承自AbstractMutableCollection抽象类
- 前言
- Kotlin简介
- IntelliJ IDEA技巧总结
- idea设置类注释和方法注释模板
- 像Android Studion一样创建工程
- Gradle
- Gradle入门
- Gradle进阶
- 使用Gradle创建一个Kotlin工程
- 环境搭建
- Androidstudio平台搭建
- Eclipse的Kotlin环境配置
- 使用IntelliJ IDEA
- Kotlin学习路线
- Kotlin官方中文版文档教程
- 概述
- kotlin用于服务器端开发
- kotlin用于Android开发
- kotlin用于JavaScript开发
- kotlin用于原生开发
- Kotlin 用于数据科学
- 协程
- 多平台
- 新特性
- 1.1的新特性
- 1.2的新特性
- 1.3的新特性
- 开始
- 基本语法
- 习惯用法
- 编码规范
- 基础
- 基本类型
- 包与导入
- 控制流
- 返回与跳转
- 类与对象
- 类与继承
- 属性与字段
- 接口
- 可见性修饰符
- 扩展
- 数据类
- 密封类
- 泛型
- 嵌套类
- 枚举类
- 对象
- 类型别名
- 内嵌类
- 委托
- 委托属性
- 函数与Lambda表达式
- 函数
- Lambda表达式
- 内联函数
- 集合
- 集合概述
- 构造集合
- 迭代器
- 区间与数列
- 序列
- 操作概述
- 转换
- 过滤
- 加减操作符
- 分组
- 取集合的一部分
- 取单个元素
- 排序
- 聚合操作
- 集合写操作
- List相关操作
- Set相关操作
- Map相关操作
- 多平台程序设计
- 平台相关声明
- 以Gradle创建
- 更多语言结构
- 解构声明
- 类型检测与转换
- This表达式
- 相等性
- 操作符重载
- 空安全
- 异常
- 注解
- 反射
- 作用域函数
- 类型安全的构造器
- Opt-in Requirements
- 核心库
- 标准库
- kotlin.test
- 参考
- 关键字与操作符
- 语法
- 编码风格约定
- Java互操作
- Kotlin中调用Java
- Java中调用Kotlin
- JavaScript
- 动态类型
- kotlin中调用JavaScript
- JavaScript中调用kotlin
- JavaScript模块
- JavaScript反射
- JavaScript DCE
- 原生
- 并发
- 不可变性
- kotlin库
- 平台库
- 与C语言互操作
- 与Object-C及Swift互操作
- CocoaPods集成
- Gradle插件
- 调试
- FAQ
- 协程
- 协程指南
- 基础
- 取消与超时
- 组合挂起函数
- 协程上下文与调度器
- 异步流
- 通道
- 异常处理与监督
- 共享的可变状态与并发
- Select表达式(实验性)
- 工具
- 编写kotlin代码文档
- 使用Kapt
- 使用Gradle
- 使用Maven
- 使用Ant
- Kotlin与OSGI
- 编译器插件
- 编码规范
- 演进
- kotlin语言演进
- 不同组件的稳定性
- kotlin1.3的兼容性指南
- 常见问题
- FAQ
- 与Java比较
- 与Scala比较(官方已删除)
- Google开发者官网简介
- Kotlin and Android
- Get Started with Kotlin on Android
- Kotlin on Android FAQ
- Android KTX
- Resources to Learn Kotlin
- Kotlin样品
- Kotlin零基础到进阶
- 第一阶段兴趣入门
- kotlin简介和学习方法
- 数据类型和类型系统
- 入门
- 分类
- val和var
- 二进制基础
- 基础
- 基本语法
- 包
- 示例
- 编码规范
- 代码注释
- 异常
- 根类型“Any”
- Any? 可空类型
- 可空性的实现原理
- kotlin.Unit类型
- kotlin.Nothing类型
- 基本数据类型
- 数值类型
- 布尔类型
- 字符型
- 位运算符
- 变量和常量
- 语法和运算符
- 关键字
- 硬关键字
- 软关键字
- 修饰符关键字
- 特殊标识符
- 操作符和特殊符号
- 算术运算符
- 赋值运算符
- 比较运算符
- 逻辑运算符
- this关键字
- super关键字
- 操作符重载
- 一元操作符
- 二元操作符
- 字符串
- 字符串介绍和属性
- 字符串常见方法操作
- 字符串模板
- 数组
- 数组介绍创建及遍历
- 数组常见方法和属性
- 数组变化以及下标越界问题
- 原生数组类型
- 区间
- 正向区间
- 逆向区间
- 步长
- 类型检测与类型转换
- is、!is、as、as-运算符
- 空安全
- 可空类型变量
- 安全调用符
- 非空断言
- Elvis操作符
- 可空性深入
- 可空性和Java
- 函数
- 函数式编程概述
- OOP和FOP
- 函数式编程基本特性
- 组合与范畴
- 在Kotlin中使用函数式编程
- 函数入门
- 函数作用域
- 函数加强
- 命名参数
- 默认参数
- 可变参数
- 表达式函数体
- 顶层、嵌套、中缀函数
- 尾递归函数优化
- 函数重载
- 控制流
- if表达式
- when表达式
- for循环
- while循环
- 循环中的 Break 与 continue
- return返回
- 标签处返回
- 集合
- list集合
- list集合介绍和操作
- list常见方法和属性
- list集合变化和下标越界
- set集合
- set集合介绍和常见操作
- set集合常见方法和属性
- set集合变换和下标越界
- map集合
- map集合介绍和常见操作
- map集合常见方法和属性
- map集合变换
- 集合的函数式API
- map函数
- filter函数
- “ all ”“ any ”“ count ”和“ find ”:对集合应用判断式
- 别样的求和方式:sumBy、sum、fold、reduce
- 根据人的性别进行分组:groupBy
- 扁平化——处理嵌套集合:flatMap、flatten
- 惰性集合操作:序列
- 区间、数组、集合之间转换
- 面向对象
- 面向对象-封装
- 类的创建及属性方法访问
- 类属性和字段
- 构造器
- 嵌套类(内部类)
- 枚举类
- 枚举类遍历&枚举常量常用属性
- 数据类
- 密封类
- 印章类(密封类)
- 面向对象-继承
- 类的继承
- 面向对象-多态
- 抽象类
- 接口
- 接口和抽象类的区别
- 面向对象-深入
- 扩展
- 扩展:为别的类添加方法、属性
- Android中的扩展应用
- 优化Snackbar
- 用扩展函数封装Utils
- 解决烦人的findViewById
- 扩展不是万能的
- 调度方式对扩展函数的影响
- 被滥用的扩展函数
- 委托
- 委托类
- 委托属性
- Kotlin5大内置委托
- Kotlin-Object关键字
- 单例模式
- 匿名类对象
- 伴生对象
- 作用域函数
- let函数
- run函数
- with函数
- apply函数
- also函数
- 标准库函数
- takeIf 与 takeUnless
- 第二阶段重点深入
- Lambda编程
- Lambda成员引用高阶函数
- 高阶函数
- 内联函数
- 泛型
- 泛型的分类
- 泛型约束
- 子类和子类型
- 协变与逆变
- 泛型擦除与实化类型
- 泛型类型参数
- 泛型的背后:类型擦除
- Java为什么无法声明一个泛型数组
- 向后兼容的罪
- 类型擦除的矛盾
- 使用内联函数获取泛型
- 打破泛型不变
- 一个支持协变的List
- 一个支持逆变的Comparator
- 协变和逆变
- 第三阶段难点突破
- 注解和反射
- 声明并应用注解
- DSL
- 协程
- 协程简介
- 协程的基本操作
- 协程取消
- 管道
- 慕课霍丙乾协程笔记
- Kotlin与Java互操作
- 在Kotlin中调用Java
- 在Java中调用Kotlin
- Kotlin与Java中的操作对比
- 第四阶段专题练习
- 朱凯Kotlin知识点总结
- Kotlin 基础
- Kotlin 的变量、函数和类型
- Kotlin 里那些「不是那么写的」
- Kotlin 里那些「更方便的」
- Kotlin 进阶
- Kotlin 的泛型
- Kotlin 的高阶函数、匿名函数和 Lambda 表达式
- Kotlin协程
- 初识
- 进阶
- 深入
- Kotlin 扩展
- 会写「18.dp」只是个入门——Kotlin 的扩展函数和扩展属性(Extension Functions / Properties)
- Kotlin实战-开发Android