[TOC]
>[success] # Reflect 使用
~~~
1.Object.getOwnPropertyNames(Reflect) 获取到'Reflect' 上的属性,因为这些属性都是
不可枚举的因此没有使用'Object.keys' 来获取,一共获取了13个静态方法
['defineProperty', 'deleteProperty', 'apply', 'construct', 'get', 'getOwnPropertyDescriptor', 'getPrototypeOf', 'has', 'isExtensible', 'ownKeys', 'preventExtensions', 'set', 'setPrototypeOf']
2.十三个静态方法具体使用规则
Reflect.apply(target, thisArg, args)
Reflect.construct(target, args)
Reflect.get(target, name, receiver)
Reflect.set(target, name, value, receiver)
Reflect.defineProperty(target, name, desc)
Reflect.deleteProperty(target, name)
Reflect.has(target, name)
Reflect.ownKeys(target)
Reflect.isExtensible(target)
Reflect.preventExtensions(target)
Reflect.getOwnPropertyDescriptor(target, name)
Reflect.getPrototypeOf(target)
Reflect.setPrototypeOf(target, prototype)
~~~
>[danger] ##### 为什么要有Reflect
1. 这是因为在早期的ECMA规范中没有考虑到这种对 对象本身 的操作如何设计会更加规范,所以将这些API放到了Object上面
2. 是Object作为一个构造函数,这些操作实际上放到它身上并不合适
3. 还包含一些类似于 in、delete操作符,让JS看起来是会有一些奇怪的
4. 在ES6中新增了Reflect,让我们这些操作都集中到了Reflect对象上
>[danger] ##### Reflect.apply -- 函数内置插槽
~~~
1.Reflect.apply 实际对应'[[call]]' 这个内部插槽,他的用法等同于es5时期'Function.prototype.apply.call',
本意是如果自定义的function 的apply方法被重写,那么可以采用使用'Function.prototype.apply.call'的形式
来实现自定义function触发的是未被重写时候的'apply' 方法,首先需要拆分理解一下'Function.prototype.apply.call'
为什么等同函数自己调用'apply', 'apply(thisArg, [argsArray])' 接受两个参数分别是'函数运行时使用的 this 值',
和'传给参数的函数但需要是数组','call' 与之不同点是他'传给函数参数需要拆分',那此时'Function.prototype.apply'
是一个整体但这个函数需要两个参数,此时call 传递给函数参数位置即确定为apply需要的参数,
call('-------' ,指向函数的this,和函数参数数组),此时是'Function.prototype.apply'调用想让指定到我们自定义函数
此时就需要对call的第一个参数this 去指定call(自定义函数this ,apply指向函数的this,apply函数参数数组)
整体就变成了'自定义函数.apply(apply函数this,参数)'
2.es5 那种绕的写法,对比'Reflect.apply'做的是同样事相对就更容易理解 ,'Reflect.apply(target, thisArg, args)'
即'target目标函数','thisArg target函数调用时绑定的this对象','args target函数调用时传入参数是个数组'
3.如果参数列表为null或undefined,即下面'getName.apply 第二个参数'和'Reflect.apply' 第三个参数,其中
'Reflect.apply' 会报错,如下。
getName.apply(null, null) // 正常执行
Reflect.apply(getName, null, null) // TypeError 异常
Reflect.apply(getName, null, "") // TypeError 异常
Reflect.apply(getName, null) // TypeError 异常
但目标函数确实没有参数使用'Reflect.apply' 需要Reflect.apply(getName, null, {})/Reflect.apply(getName, null, []) 来解决
~~~
* 案例说明
~~~
const obj = { f: 'w' }
var f = 'windows'
function getName(age) {
console.log(age, this.f)
}
getName(12)
getName.apply(obj, [12])
Reflect.apply(getName, null, [12])
Reflect.apply(getName, obj, [12])
Function.prototype.apply.call(getName, obj, [12])
// 打印结果
12 'windows'
12 'w'
12 'windows'
12 'w'
12 'w'
~~~
>[danger] ##### Reflect.construct -- 函数内置插槽
~~~
1.'Reflect.construct(target, argumentsList, [, newTarget])'
1.1.target- 代表目标构造函数的第一个参数
1.2.argumentsList- 第二个参数,代表目标构造函数的参数,类数组格式
1.3.newTarget- 作为新创建对象的原型对象的constructor属性,可以选不填默认是target。
2.对应的内部插槽'[[constructor]]',等同于'var obj = new Foo(...args)'
3.和'Reflect.apply' 一样如果不需要对目标函数传参那么需要传一个空对象,否则报错
Reflect.constructor(target, null) // TypeError 异常
Reflect.constructor(target) // TypeError 异常
Reflect.constructor(target, {}) // 不传参
Reflect.constructor(target, []) // 不传参
4.案例二可以看出当使用了第三个参数可以改变对象,[[Prototype]] 指向即改变'__proto__',此时创建
出来的对象构造函数指向了第三个参数,等同于之前
// 生成一个对象[[Prototype]] 指向指向OtherClass.prototype
var obj2 = Object.create(OtherClass.prototype);
// 将target函数this 指向新对象的
OneClass.apply(obj2, args);
只是这种创建其实'OneClass.apply' 激活的是内部插槽'[[Call]]' 只是将this 从windows 指向了我们期望的对象
上,虽然效果一样但是这种创建的'new.target' 为undefined
~~~
* 案例
~~~
class A {
constructor(age) {
this.age = age
}
getAge() {
return this.ages
}
}
const newA = new A(...[10]) // 因为Reflect 第二个参数是伪数组所以为了使用new 更接近使用数组结构
const reflectA = Reflect.construct(A, [10])
~~~
* 改变生成对象原型链指向
~~~
class A {
name = 'a'
age = 'b'
getAge() {}
}
class B {
zz = 12
getName() {}
}
const b = Reflect.construct(A, {}, B)
console.log(b instanceof A) // false
console.log(b instanceof B) // true
~~~
* 如图
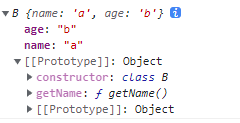
>[danger] ##### Reflect.defineProperty
~~~
1.'Reflect.defineProperty(target, propertyKey, attributes)' 使用的内部插槽'[[DefineOwnProperty]]'
1.1.target目标对象。
1.2.propertyKey要定义或修改的属性的名称。
1.3.attributes要定义或修改的属性的描述。
2.等同于'Object.defineProperty(obj, prop, descriptor)',二者不同点Reflect方法返回布尔值 true(成功)/false(失败)
Object返回的是目标对象
~~~
* 案例
~~~
function MyDate() {
/*…*/
}
// 旧写法
Object.defineProperty(MyDate, 'now', {
value: () => Date.now()
});
// 新写法
Reflect.defineProperty(MyDate, 'now', {
value: () => Date.now()
});
~~~
>[danger] ##### Reflect.deleteProperty
~~~
1.对应的内部插槽'[[Delete]]' 方法等同于delete obj[name],用于删除对象的属性,方法的第一个参数不是对象,会报错
'Reflect.deleteProperty(target, propertyKey)'
~~~
~~~
var obj = { x: 1, y: 2 };
Reflect.deleteProperty(obj, "x"); // true
obj; // { y: 2 }
var arr = [1, 2, 3, 4, 5];
Reflect.deleteProperty(arr, "3"); // true
arr; // [1, 2, 3, , 5]
// 如果属性不存在,返回 true
Reflect.deleteProperty({}, "foo"); // true
// 如果属性不可配置,返回 false
Reflect.deleteProperty(Object.freeze({foo: 1}), "foo"); // false
~~~
>[danger] ##### Reflect.has
~~~
1.Reflect.has方法检查target对象或其原型上的propertyKey属性是否存在。这与in操作符完全相同。如果找到属性,
则返回true,否则返回false,对应内部插槽'[[HasProperty]]'
~~~
* 案例
~~~
'assign' in Object // true
// 新写法
Reflect.has(Object, 'assign') // true
~~~
>[danger] ##### Reflect.ownKeys
~~~
1.'Reflect.ownKeys' 使用的内部插槽 '[[OwnPropertyKeys]]' ,方法返回一个由目标对象自身的属性键组成的数组。
它的返回值等同于Object.getOwnPropertyNames(target).concat(Object.getOwnPropertySymbols(target))。
即自身所有属性(包含可枚举不可枚举和symbol 属性)
~~~
* 案例
~~~
const sy = Symbol('a')
class A {
name = 123;
[sy] = 145
getName() {}
}
class B extends A {}
const b = new B()
// 打印结果
[ 'name', 'sy' ]
~~~
>[danger] ##### Reflect.preventExtensions
~~~
1.'Reflect.preventExtensions(target)' target 必须是一个对象如果是非对象会异常报错,对应的内部插
槽'[[PreventExtensions]]',让对象方法不可以进行扩展,返回一个 Boolean 值表明目标对象是否成功被设置为不可扩展
2.对应的方法,Object.preventExtensions() 方法, 不同点是它对非对象的 target 参数将被强制转换为对象。
~~~
* 案例
~~~
const aa = { name: 1 }
Reflect.preventExtensions(aa)
aa.age = 1
aa.name = 2
// 打印结果
{name:2} // age 属性并没有被添加进去
~~~
>[danger] ##### Reflect.isExtensible
~~~
1.'Reflect.isExtensible(target)' target 必须是一个对象如果是非对象会异常报错,对应的内部插
槽'[[IsExtensible]]',检查对象是否可以进行扩展,返回一个 Boolean 值表明目标对象是否可扩展
2.对应的方法,Object.isExtensible() 方法, 不同点是它对非对象的 target 参数将被强制转换为对象。
~~~
* 案例
~~~
const aa = { name: 1 }
Reflect.preventExtensions(aa)
console.log(Reflect.isExtensible(aa)) // false
~~~
>[danger] ##### Reflect.getOwnPropertyDescriptor
~~~
1.'Reflect.getOwnPropertyDescriptor(target, propertyKey)'trget 必须是一个对象如果是非对象会异常报错,对应的内部插
槽'[[GetOwnProperty]]',返回属性的描述符,并且方法返回目标对象的非继承属性的属性描述符即非'[[Prototype]](__proto)'
上的属性
2.对应的方法,Object.getOwnPropertyDescriptor() 方法, 不同点是它对非对象的 target 参数将被强制转换为对象。
~~~
* 案例
~~~
Reflect.getOwnPropertyDescriptor({x: "hello"}, "x");
// {value: "hello", writable: true, enumerable: true, configurable: true}
Reflect.getOwnPropertyDescriptor({x: "hello"}, "y");
// undefined
Reflect.getOwnPropertyDescriptor([], "length");
// {value: 0, writable: true, enumerable: false, configurable: false}
// ------------------------------ 非继承属性 ---------------------------------------
const sy = Symbol('a')
class A {
name = 123;
[sy] = 145
getName() {}
}
class B extends A {
getAge() {}
}
const b = new B()
console.log(Reflect.getOwnPropertyDescriptor(b, 'getName'))
console.log(Reflect.getOwnPropertyDescriptor(b, 'getAge'))
console.log(Reflect.getOwnPropertyDescriptor(b, 'name'))
// // 打印结果:
// undefined
// undefined
// { value: 123, writable: true, enumerable: true, configurable: true }
~~~
>[danger] ##### Reflect.getPrototypeOf
~~~
1.静态方法 Reflect.getPrototypeOf(target) 与 Object.getPrototypeOf() 方法几乎是一样的。都是返回指定对象的原型
(即内部的 [[Prototype]] 属性的值)。如果目标没有原型,则返回null。Reflect 要求target 必须是对象,Object则不用
会自动进行拆箱转换
~~~
* 案例
~~~
Reflect.getPrototypeOf( null) // TypeError: Reflect.getPrototypeOf called on non-object
Reflect.getPrototypeOf( 'hello') // TypeError: Reflect.getPrototypeOf called on non-object
Reflect.getPrototypeOf( {}) // Object {constructor: Object(), __defineGetter__: ƒ, …}
~~~
>[danger] ##### Reflect.setPrototypeOf
~~~
1.'Reflect.setPrototypeOf(target, prototype)',返回一个 Boolean 值表明是否原型已经成功设置,内部的 [[Prototype]] 属性值
对应' Object.setPrototypeOf() '
~~~
* 案例
~~~
const object1 = {};
console.log(Reflect.setPrototypeOf(object1, Object.prototype));
// expected output: true
console.log(Reflect.setPrototypeOf(object1, null));
// expected output: true
const object2 = {};
console.log(Reflect.setPrototypeOf(Object.freeze(object2), null));
// expected output: false
~~~
>[danger] ##### Reflect.get(target, name, receiver)
~~~
1.'Reflect.get(target, propertyKey, [, receiver])'方法与从 对象 (target[propertyKey]) 中读取属性类似,但它是通过一
个函数执行来操作的。如果具有名称propertyKey的属性是中的getter函数,则receiver参数则被当做 this。
如果缺少receiver,则this是target,注意案例二这类改变this 只能是getter函数的
2.调用 reflect.get() 类似于 target[propertykey] 表达式,因为它也搜索 target 原型上的属性值。如果目标上不存在属性,
则返回 undefined。
~~~
* 案例 1
~~~
var computer1 = {
processor: "Intel",
brand: "Dell",
operatingSystem: "windows 7"
};
console.log(computer1);
Reflect.get(computer1, "processor"); // computer1.processor 等同
console.log(computer1.processor);
~~~
* 案例二
~~~
const dinoComputer = {
processor: "Intel",
brand: "Dell",
operatingSystem: "windows 7"
};
Reflect.defineProperty(dinoComputer, "computerDetails", {
get: function() {
return new String().concat(`*********Computer Details********\r\n`,
`****Processor: ${this.processor}***********\r\n`,
`****Brand: ${this.brand}*****************\r\n`,
`****Operating System: ${this.operatingSystem}*\r\n`);
}
});
console.log(dinoComputer);
let oldComputer = Reflect.get(dinoComputer, "computerDetails",
{ processor: "AMD K62",
brand: "Clone",
operatingSystem: "Windows XP" });
console.log(oldComputer);
~~~
>[danger] ##### Reflect.set
~~~
1.'Reflect.set(target, propertyKey, value[, receiver])' 等同于'target[propertyKey] = value'
~~~
>[info] ## 参考文章
[JavaScript Reflection and Reflect API
](https://www.codeproject.com/Articles/5275933/JavaScript-Reflection-and-Reflect-API#get)
[【译】JavaScript元编程之——Reflect API简介
](https://juejin.cn/post/7060056954130923528#heading-17)
[Metaprogramming in ES6: Part 2 - Reflect](https://www.keithcirkel.co.uk/metaprogramming-in-es6-part-2-reflect/)
- HTTP -- 初始
- HTTP -- 什么是HTTP
- HTTP -- 相关技术
- HTTP -- 相关的协议
- Emmet -- 语法
- HTML -- 补充
- iframe -- 补充
- checkbox 和 radio 细节
- form -- 补充
- html -- html5
- html -- 视频音频
- html -- html5 data-* 全局属性
- css -- 重学
- css -- 单位
- css 知识补充 -- 导入
- css -- 颜色补充
- css --继承性
- css - 元素隐藏
- 标签元素--块/行内/行内块
- css -- 盒子阴影 – 在线查看
- css -边框图形
- css -- Web字体
- css -- 精灵图
- css -- 属性补充
- text-align -- 内容
- line-height -- 行高
- 文字换行
- overflow-溢出
- css -- 选择器
- css -- 伪元素
- css -- 伪类选择器
- 设置高度宽度 和 盒子模型
- css -- 文字溢出
- css -- white-space / text-overflow
- css -- 定位
- css -- 浮动
- 浮动 -- 案例
- flex -- 布局
- flex -- 解决等距布局
- flex -- 内容居中
- flex -- 导航栏
- Grid -- 布局
- css -- transform 形变
- css -- 垂直水平居中
- css -- transition 动画
- css -- Animation 动画
- css -- vertical-align
- css -- 函数
- css -- 媒体查询
- 重学案例
- 重新 -- 鼠标常见样式
- 重学 -- 透明边框background-clip
- 重学 -- 多重边框box-shadow
- css -- 预处理器
- 移动端适配
- 前端结构目录说明
- 浏览器 加载过程
- 回流和重绘
- 杂七杂八 -- 小工具方法
- npm包比较网站
- IP定位
- 通过useragent获取手机品牌型号
- 自启本地服务
- BOM -- 常用知识点记录
- window -- 认识
- windows -- 大小滚动
- BOM -- Location
- BOM -- URLSearchParams
- BOM -- history
- BOM -- navigator 和 screen
- 前端存储 -- Cookie、LocalStorage、IndexedDB
- DOM -- 知识总结
- DOM -- 获取元素
- DOM -- 节点属性
- DOM -- 元素属性
- 获取元素style的读取 - getComputedStyle
- DOM -- 元素的操作
- DOM -- 元素大小和滚动
- DOM -- 小练习
- Event -- 事件
- event -- 事件对象
- event -- 案例
- event -- 做一个树
- js -- ajax
- 封装一个ajax
- ajax -- 文件上传
- 倒计时抢购案例
- axios -- 封装
- 跨域
- 前端 -- Fetch API
- js -- 基础篇
- 数据类型
- 数据类型检测
- 类型小知识
- 原始类型的包装类
- 类型转换
- delete -- 运算符
- Date -- 对象
- 函数参数传递
- 对象某个属性不存时候判断
- 操作符
- 函数变量传值
- 访问对象通过点和[]
- 和if等同的一些写法
- for -- 执行顺序
- JS -- 执行过程
- JS中的堆(Heap)栈(Stack)内存
- JS -- 执行上下文
- Js -- ES3 和 ES5+ 后
- let const var
- ES3 -- 案例思考
- 闭包概念
- 浅拷贝和深拷贝
- JS -- 严格模式
- js -- 数组篇
- Array -- 数组基础
- Array -- 小常识检测数组
- Array -- 小技巧将数组转成字符串
- Array -- 自带方法
- Array -- 数组插入总结
- Array -- every是否都符合巧用
- js--Function篇
- Function -- length 属性
- Function -- arguments
- Function -- 也是对象
- Function -- new Function 创建方法
- Function -- 函数作为返回值
- Function -- callee
- 匿名函数
- Function -- 闭包
- 闭包内存查看
- 闭包 -- 使用的案例
- 闭包 -- 使用的案例
- 箭头函数不适用场景
- js -- this、call、apply
- this -- 指向
- call、apply -- 讲解
- 总结 -- this
- 思考题
- Object -- 数据属性/访问器属性
- 新增关于对象方法
- js -- 面向对象
- 对象到底是什么
- 到底什么是js的对象
- js --prototype、__proto__与constructor 1
- JS es5继承
- JS 中的原型继承说明
- JS -- Object是所有类的父类
- 总结
- Object -- 函数构造函数
- Object -- 手动实现一个new
- js -- 函数式编程(目前只是了解后面需要更多时间深入)
- 了解 -- 高阶函数
- 了解 -- 纯函数
- 了解 -- 函数柯里化
- 柯里化 -- 知识点
- 了解 -- 函数组合
- 了解 -- 函子
- js--小知识点
- url -- 将get请求连接参数变成对象
- event -- 一个函数处理多个事件
- try -- 处理异常
- Error -- 前段报错信息传给后台
- JSON -- 序列化
- return -- 返回true和false
- for -- 循环里初始化变量
- 命名 -- get和set怎么命名
- 链式调用
- 利用递归代替循环思路
- JS -- 技巧篇
- 技巧 -- 代码规范
- 技巧 -- 惰性载入函数
- 技巧 -- 防抖函数
- 技巧 -- 节流函数
- 插入补充(防抖/节流)
- 技巧 -- 定时器的使用
- 技巧 -- 回调函数
- 技巧 -- 分时函数
- 技巧 -- 除了return 我怎么跳出多层循环
- 技巧 -- switch 还是 if-else?
- 技巧 -- 将字符串转成对象
- 技巧 -- 函数转换
- 技巧 -- 工作记录数组对象中相同项
- JS -- 数组小文章总结
- 数组类型判断
- includes 和 indexOf
- for ... in,for ... of和forEach之间有什么区别
- map、filter、reduce、find 四种方法
- 多种形式处理数组思考方式
- for...in 和 Object.keys
- 各种知识点集合文章
- 创建数组 -- 总结
- 数组去重 -- 总结
- 获取数组中不重复的元素 -- 总结
- 比较两个数组元素相同 -- 总结
- 出现频率最高的元素 -- 总结
- 两数组交集 -- 总结
- 两数组差集 -- 总结
- 工具方法 - 总结
- 扁平化数组
- JS -- 数组技巧篇 30s
- 30s Array -- 创建数组篇(一)
- 30s Array --过滤(查询)篇章(一)
- 30s Array --过滤篇章(二)
- 30s Array -- 新增篇(一)
- 30s Array--比较篇(一)
- 30s Array -- 分组篇(一)
- 30 Array -- 删除篇(一)
- 30s Array-- 其他篇(一)
- 30s Array -- 个人感觉不常用篇章
- JS -- 对象技巧篇30s
- 30s Object -- 查(一)
- 30s Object -- 增(一)
- 30s Object -- 工具类型小方法
- 30s Object -- 跳过没看系列
- ES -- 新特性
- 变量篇章
- 变量 -- let/const/var声明
- 变量 -- 词法声明和变量声明
- 变量 -- var x = y = 100
- 变量 -- a.x = a = {n:2}
- 带标签的模板字符串
- 函数篇章
- 函数 -- 参数篇章
- 函数 -- 只能通过new创建实例
- 函数 -- 箭头函数
- 函数 -- 尾调优化
- 对象篇章
- 对象 -- 字面量写法的优化/新增方法
- 赋值篇章
- 解构赋值 -- 简单概念
- 解构赋值 -- 对象解构
- 解构赋值 -- 数组解构
- 解构赋值 -- 函数参数
- Symbol 属性
- Set 和 Map
- Set -- 去重/交、并、差集
- Map -- 集合
- 类class篇章
- ES6 和 ES5 的写法比较
- es6 -- mixin函数继承
- es6 -- 创建一个接口
- ES5 VS ES6 -- class 编译
- 数组新功能
- 创建/转换 -- 数组
- 迭代器和生成器
- es6 -- 迭代器
- es6 -- 生成器
- for-of 循环的是什么
- 做一个异步执行器
- 代理和反射
- Proxy -- 使用
- Reflect -- 使用
- Proxy 和 Reflect 结合使用
- 运算符 -- 展开运算符
- ES7 -- 新特性
- ES8 -- 新特性
- ES9 -- 新特性
- ES10 -- 新特性
- ES11 -- 新特性
- ES12 -- 新特性
- ES13 -- 新特性
- ES6 和 ES8 的异步
- js -- 异步、同步、阻塞、非阻塞
- js -- 如何做到异步的
- js -- 引擎线程,GUI渲染线程,浏览器事件触发线程
- js -- 如何通过事件循环运行异步
- js -- 误区任务回调就是异步
- js -- 宏任务和微任务
- 参考文章推荐阅读
- js -- callback 还是 promise
- js -- Promise 初识
- js -- 自己实现一个Promise
- js -- Promise 更多用法
- 再来看setTimeout 和for
- js -- ES8 异步 async await
- js -- 红绿灯问题
- js -- 倒计时
- 异步图片预加载
- 手动触发异步
- 异步题
- JS -- 模块化
- CommonJS -- 在node服务器端
- AMD 模块化规范
- ES Modules
- ES Modules -- 使用特点
- import 和 export 使用
- export 和 import -- 执行
- 其他用法
- systemjs
- 对比区别
- 使用babel 或webpack进行模块打包
- Jq -- 细节
- JS -- 性能优化
- 图片预加载
- js -- 正则
- 设计原则和编程技巧
- 设计原则 -- 单一职责
- js -- 设计模式
- 设计模式 -- 简单理解
- 一、鸭子类型
- 1.1 多态概念
- 1.2 小章节记录 -- 封装
- 1.3.多态和鸭子
- 设计模式 -- 单例模式(透明、惰性)
- 单例模式 -- js单例和惰性单例
- ES6 -- 写单例模式
- 设计模式 -- 策略模式
- 策略模式 -- 表单验证
- 策略模式 -- 表单验证多规则
- 策略模式和多态区别
- 设计模式 -- 代理模式(代理被代理相同方法)
- 代理模式 -- 分类
- 代理模式 -- 总结
- 设计模式 -- 迭代器模式
- 设计模式 -- 观察者(发布订阅)模式
- 观察者 和 发布订阅区别
- 发布订阅模式 -- 现在通用模型
- 发布订阅模式 -- 书中实际案例
- 发布订阅模式--全局发布订阅对象
- 发布订阅模式 -- vue
- 设计模式 -- 命令模式(对象方法拆分指令)
- 命令模式 -- js 自带
- 命令模式 -- 撤销和重做
- 命令模式 -- 命令和策略的区别
- 设计模式 -- 组合模式(树)
- 组合模式 -- 拆分了解
- 组合模式 -- java角度
- 组合模式 -- 书中案例扫描文件夹
- 组合模式 -- 注意点
- 组合模式 -- 引用父对象(书中案例)
- 组合模式 -- 小的案例
- 组合模式 -- 总结
- 设计模式 -- 模板方法(抽象类继承)
- 模板方法 -- 前端角度思考
- 模板方法 -- java 思考方式
- 模板方法 -- js没有抽象类
- 模板方法 -- 钩子方法
- 模板方法 -- 用js方式来实现
- 设计模式 -- 享元模式
- 享元、单例、对象池
- 享元模式 -- 前端角度思考
- 享元模式 -- 代码案例
- 享元模式 -- 适用和内外部状态
- 额外扩展 -- 对象池
- 设计模式 -- 职责链模式
- 职责链 -- 前端角度
- 职责链和策略
- 职责链 -- 异步的职责链
- 职责链 -- AOP
- 职责链 -- 改造迭代器模式的代码
- 设计模式 -- 中介者模式
- 中介者模式 -- 前端角度(一)
- 中介者模式 -- 前端角度(二)
- 中介者模式 -- 前端角度(三)
- 中介者模式 -- 总结
- 设计模式 -- 装饰者模式
- 装饰者模式 -- es5 解决方案
- 装饰器模式 -- 和代理模式区别
- 设计模式 -- 状态模式
- 状态模式 -- 前端思想
- 状态模式 -- 文件上传案例
- 状态模式 -- 和策略模式的区别
- 设计模式 -- 适配器模式
- js -- 代码重构
- 重构 -- 方法封装
- 重构 -- 抽象函数
- 高阶函数 -- 范式
- 状态管理方案
- Node -- 学习篇
- Node -- 服务端运行的js
- node -- Global(全局成员)
- node -- Buffer缓冲器
- node -- 路径操作
- node -- 文件的读写
- node -- 目录操作
- node -- HTTP
- HTTP -- 响应头常见类型
- HTTP -- 处理Get
- HTTP -- 处理Post
- HTTP -- 简单的案例
- Express
- Express -- 中间件
- Express -- 处理post/get
- Express -- 模板引擎
- Express -- 简单案例和目录搭建
- Express -- 数据库service.js
- Express -- json/url
- Express -- 配合数据库
- 配合数据库 -- 简单的登录
- npm -- js包管理
- npm -- 淘宝镜像
- nrm -- 更多镜像的选择
- yarn -- 包管理
- yarn -- 清理缓存
- WebPack -- 模块化开发
- webPack -- 安装、使用、打包
- webPack -- 使用配置文件
- 延伸 -- base64图片优劣
- webPack -- 完整配置