>[success] # 关于继承
1. 在 JavaScript 中,是通过**遍历原型链**的方式,**来访问对象的方法和属性**,简单的说就是**我自身没有我就通过'_\_proto__' 找到我的构造函数的'prototype'上**,构造函数原型对象没有他就去找**构造函数上'_\_proto__' 链接的原型对象**一直都没找到的情况下,最后找到**null 终止**
2. 在原型链上查找属性**比较耗时**,**对性能有副作用**,这在性能要求苛刻的情况下很重要。另外试图访问**不存在**的属性时**会遍历整个原型链**。
~~~
function Person (name) {
this.name = name;
}
var lily = new Person("Lily");
~~~
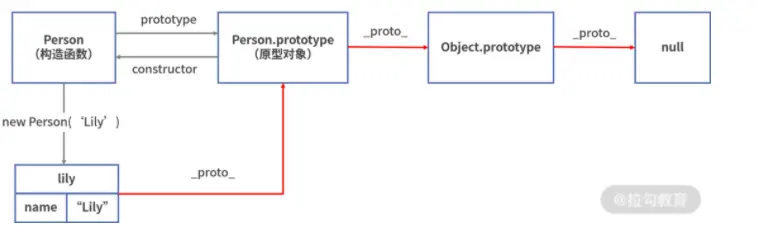
3. **js属性遮蔽**可以理解成就近原则
~~~
function A (name) {
this.name = name
}
A.prototype.name = "原型对象上的name 属性"
const a = new A('w')
console.log(a.name); // w
~~~
* 会找离自身最近属性对应值
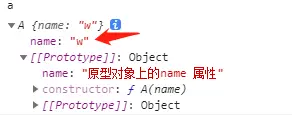
>[danger] ##### hasOwnProperty -- 属性是否是原型对象上
1. 证明打印是自己的而不是原型对象**prototype**上的,使用**hasOwnProperty**返回的是**true** 则使用的是实例对象自己的,**false**怎相反
~~~
function Person() {}
// 每一个函数都有一个原型属性prototype
// 他们都会指向实例对象因此在Person这个
// 构造函数的prototype加属性即可创建的对象共享
Person.prototype.name = 'wang'
const p1 = new Person()
// 当前的name 到底是p1的还是 Person的
console.log(p1.hasOwnProperty('name'))
const p2 = new Person()
p2.name = 'p2'
// 当前的name 到底是p1的还是 Person的
console.log(p2.hasOwnProperty('name'))
打印结果:
false
true
~~~
>[info] ## 继承几种方式
>[danger] ##### 原型链继承
1. 这种继承新实例无法向**父类构造函数传参**
2. 所有新实例都会共享父类实例的属性,会产生的问题两个实例使用的是**同一个原型对象**。它们的内存空间是共享的,如果属性是**引用类型**当一个发生变化的时候,另外一个也随之进行了**变化**
3. 通过直接打印对象是看不到这个属性的,因为我们将父类直接new 生成对象挂在到原型链上,其实当调用时候自身没有的都会去挂在对象去找本事其真正本身属性都是共享属性
~~~
// 第一种 原型链继承
function Parent(){
this.name="parent";
this.play = [1,2,3]
}
function Child(){
this.type="child";
}
Child.prototype = new Parent();
const child1 = new Child()
const child2 = new Child()
child1.play.push(12345)
console.log(child1.play,child2.play); // [ 1, 2, 3, 12345 ] [ 1, 2, 3, 12345 ]
~~~
>[danger] ##### 构造函数继承
1. 此通过 **apply()和call()** 方法 改变函数执行时this 指向,单独声明时候并不会执行只有当new Child 时候此时执行然后给当前this 调用了一个赋值属性的封装方法
~~~
function a(obj){
obj.name = "w"
obj.age = 12
}
const z = {}
a(z)
~~~
2. 这种问题弊端只继承了父类构造函数的属性,没有继承父类原型的属性,因此导致下面的'child.getName()'
调用会报错
~~~
function Parent(age){
this.name="parent";
this.age = age
this.play = [1,2,3]
}
Parent.prototype.getName = function(){
return this.name
}
function Child(age){
// ;(function(){
// this.name = "zzz"
// this.play = [1,2,3]
// }).call(this)
// 上面注释方法和下面等同
Parent.call(this,age)
this.type="child";
}
const child1 = new Child(1)
const child2 = new Child(10)
child1.play.push(12345)
console.log(child1.play,child2.play); // [ 1, 2, 3, 12345 ] [ 1, 2, 3 ]
// 报错
// console.log(child.getName())
~~~
>[danger] ##### 组合继承
1. 上面两种各自有对方不能解决的问题,将这两种组合形成继承,弊端就是下面的'Parent' 会执行两次,分别在构造函数时候执行一次在原型链上执行一次浪费性能开销
1.1. 一次在创建子类原型的时候;
1.2. 一次在子类构造函数内部(也就是每次创建子类实例的时候);
2. **所有的子类实例事实上会拥有两份父类的属性**
2.1. 一份在当前的实例自己里面(也就是person本身的),另一份在子类对应的原型对象中(也就是person.\_\_proto\_\_里面),这两份属性我们无需担心访问出现问题,因为默认一定是访问实例本身这一部分的(就近原则)
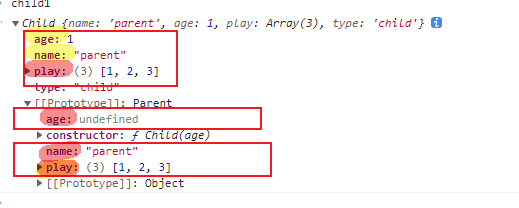
~~~
// 第一种 原型链继承
function Parent(age){
this.name="parent";
this.age = age
this.play = [1,2,3]
}
Parent.prototype.getName = function(){
return this.name
}
function Child(age){
Parent.call(this,age)
this.type="child";
}
// 执行一次
Child.prototype = new Parent()
// 手动挂上构造器,指向自己的构造函数
Child.prototype.constructor = Child;
const child1 = new Child(1) // 触发函数执行了第二次Parent = 》Parent.call(this,age)
const child2 = new Child(10)
child1.play.push(12345)
console.log(child1.play,child2.play); // [ 1, 2, 3, 12345 ] [ 1, 2, 3 ]
~~~
>[danger] ##### 原型继承
**道格拉斯·克罗克福**德在一篇文章中介绍了一种实现继承的方法,**这种方法并没有使用严格意义上的构造函数**。它的想法**是借助原型可以基于已有的对象创建新对象,同时还不必因此创建自定义类型**。为了达到这个目的,他给出了如下函数。
~~~
function object(o){
function F() {}
F.prototype = o;
return new F();
}
~~~
在object()函数内部,**先创建了一个临时性的构造函数,然后将传入的对象作为这个构造函数的原型,最后返回了这个临时类型的一个新实例**。**从本质上讲,object()对传入其中的对象执行了一次浅复制**。
克罗克福德主张的**这种原型式继承**,**要求你必须有一个对象可以作为另一个对象的基础**。
1. ECMAScript5通过新增 **Object.create()** 方法规范化了原形式继承这个方法接收两个参数:一是用作新对象原型的对象、二是为新对象定义额外属性的对象(可选参数),将这个方法拆解可以写作下面
~~~
Object.myCreate = function (obj, properties) {
var F = function () {}
F.prototype = obj
if (properties) {
Object.defineProperties(F, properties)
}
return new F()
}
~~~
2. 如图相当于都挂载到原型了导致数据共享问题,无法实现复用,属于自己的属性需要给create 第二个方法传参
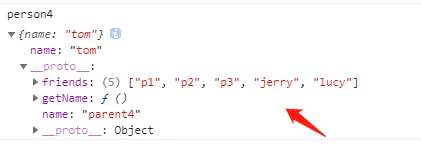
3. 这类思想就是脱离之前构造函数的想法,而是直接给对象做继承,虽然每次伪造了一个 构造函数但对其包装并未暴露
~~~
let parent4 = {
name: "parent4",
friends: ["p1", "p2", "p3"],
getName: function() {
return this.name;
}
};
let person4 = Object.create(parent4);
person4.name = "tom";
person4.friends.push("jerry");
let person5 = Object.create(parent4);
person5.friends.push("lucy");
console.log(person4.name);
console.log(person4.name === person4.getName());
console.log(person5.name);
console.log(person4.friends);
console.log(person5.friends);
打印结果:
tom
true
parent4
[ 'p1', 'p2', 'p3', 'jerry', 'lucy' ]
[ 'p1', 'p2', 'p3', 'jerry', 'lucy' ]
~~~
>[danger] ##### 寄生式继承
1. **寄生式(Parasitic)继承是与原型式继承紧密相关的一种思想**, 并且同样由道格拉斯·克罗克福德(Douglas Crockford)提出和推广的,不同在于**手动去增加当前实例的属性和方法**,寄生式继承的思路是结合原型类继承和**工厂模式**的一种方式
~~~
let parent5 = {
name: "parent5",
friends: ["p1", "p2", "p3"],
getName: function() {
return this.name;
}
};
function clone(original) {
let clone = Object.create(original);
clone.getFriends = function() {
return this.friends;
};
return clone;
}
let person5 = clone(parent5);
console.log(person5.getName());
console.log(person5.getFriends());
~~~
>[danger] ##### 寄生组合继承
1. 组合继承是比较理想的继承方式, 但是存在两个问题:
1.1. 构造函数会被调用两次: 一次在创建子类型原型对象的时候, 一次在创建子类型实例的时候.
1.2. 父类型中的属性会有两份: 一份在原型对象中, 一份在子类型实例中
2. **Object.create** 可以挂载原型,这样直接将父类的原型挂载给当前**Child.prototype= new Parent()** 相对于这种相当于少执行一次父类的实例,在利用call 将父类的属性在当前子类的构造函数挂载会来,在这个时候有个疑问为什么不**Child.prototype= Parent.property** 这样的话两者内存共享子类也可以修改父类**new Parent()** 本身就是为了创建一个新的不影响的**Object.create**本质也是创建的新对象
3. 是否可以直接对象的隐式原型去绑定,也就是
~~~
const c = new Child()
c.__proto__ = Parent.property
~~~
这样问题就是`__proto__` 并不是js 提供只是浏览器提供所以这种用法未来会出现兼容性问题
~~~
function clone (parent, child) {
// 这里改用 Object.create 就可以减少组合继承中多进行一次构造的过程
child.prototype = Object.create(parent.prototype);
child.prototype.constructor = child;
}
function Parent6() {
this.name = 'parent6';
this.play = [1, 2, 3];
}
Parent6.prototype.getName = function () {
return this.name;
}
function Child6() {
Parent6.call(this);
this.friends = 'child5';
}
clone(Parent6, Child6);
Child6.prototype.getFriends = function () {
return this.friends;
}
let person6 = new Child6();
console.log(person6);
console.log(person6.getName());
console.log(person6.getFriends());
~~~
>[info] ## 参考
[参考文章](https://www.kancloud.cn/cyyspring/html_js_cs/725347)
[六种继承](https://www.cnblogs.com/Grace-zyy/p/8206002.html)
[参考拉勾教育文章链接](https://kaiwu.lagou.com/course/courseInfo.htm?courseId=601#/detail/pc?id=6176)
- HTTP -- 初始
- HTTP -- 什么是HTTP
- HTTP -- 相关技术
- HTTP -- 相关的协议
- Emmet -- 语法
- HTML -- 补充
- iframe -- 补充
- checkbox 和 radio 细节
- form -- 补充
- html -- html5
- html -- 视频音频
- html -- html5 data-* 全局属性
- css -- 重学
- css -- 单位
- css 知识补充 -- 导入
- css -- 颜色补充
- css --继承性
- css - 元素隐藏
- 标签元素--块/行内/行内块
- css -- 盒子阴影 – 在线查看
- css -边框图形
- css -- Web字体
- css -- 精灵图
- css -- 属性补充
- text-align -- 内容
- line-height -- 行高
- 文字换行
- overflow-溢出
- css -- 选择器
- css -- 伪元素
- css -- 伪类选择器
- 设置高度宽度 和 盒子模型
- css -- 文字溢出
- css -- white-space / text-overflow
- css -- 定位
- css -- 浮动
- 浮动 -- 案例
- flex -- 布局
- flex -- 解决等距布局
- flex -- 内容居中
- flex -- 导航栏
- Grid -- 布局
- css -- transform 形变
- css -- 垂直水平居中
- css -- transition 动画
- css -- Animation 动画
- css -- vertical-align
- css -- 函数
- css -- 媒体查询
- 重学案例
- 重新 -- 鼠标常见样式
- 重学 -- 透明边框background-clip
- 重学 -- 多重边框box-shadow
- css -- 预处理器
- 移动端适配
- 前端结构目录说明
- 浏览器 加载过程
- 回流和重绘
- 杂七杂八 -- 小工具方法
- npm包比较网站
- IP定位
- 通过useragent获取手机品牌型号
- 自启本地服务
- BOM -- 常用知识点记录
- window -- 认识
- windows -- 大小滚动
- BOM -- Location
- BOM -- URLSearchParams
- BOM -- history
- BOM -- navigator 和 screen
- 前端存储 -- Cookie、LocalStorage、IndexedDB
- DOM -- 知识总结
- DOM -- 获取元素
- DOM -- 节点属性
- DOM -- 元素属性
- 获取元素style的读取 - getComputedStyle
- DOM -- 元素的操作
- DOM -- 元素大小和滚动
- DOM -- 小练习
- Event -- 事件
- event -- 事件对象
- event -- 案例
- event -- 做一个树
- js -- ajax
- 封装一个ajax
- ajax -- 文件上传
- 倒计时抢购案例
- axios -- 封装
- 跨域
- 前端 -- Fetch API
- js -- 基础篇
- 数据类型
- 数据类型检测
- 类型小知识
- 原始类型的包装类
- 类型转换
- delete -- 运算符
- Date -- 对象
- 函数参数传递
- 对象某个属性不存时候判断
- 操作符
- 函数变量传值
- 访问对象通过点和[]
- 和if等同的一些写法
- for -- 执行顺序
- JS -- 执行过程
- JS中的堆(Heap)栈(Stack)内存
- JS -- 执行上下文
- Js -- ES3 和 ES5+ 后
- let const var
- ES3 -- 案例思考
- 闭包概念
- 浅拷贝和深拷贝
- JS -- 严格模式
- js -- 数组篇
- Array -- 数组基础
- Array -- 小常识检测数组
- Array -- 小技巧将数组转成字符串
- Array -- 自带方法
- Array -- 数组插入总结
- Array -- every是否都符合巧用
- js--Function篇
- Function -- length 属性
- Function -- arguments
- Function -- 也是对象
- Function -- new Function 创建方法
- Function -- 函数作为返回值
- Function -- callee
- 匿名函数
- Function -- 闭包
- 闭包内存查看
- 闭包 -- 使用的案例
- 闭包 -- 使用的案例
- 箭头函数不适用场景
- js -- this、call、apply
- this -- 指向
- call、apply -- 讲解
- 总结 -- this
- 思考题
- Object -- 数据属性/访问器属性
- 新增关于对象方法
- js -- 面向对象
- 对象到底是什么
- 到底什么是js的对象
- js --prototype、__proto__与constructor 1
- JS es5继承
- JS 中的原型继承说明
- JS -- Object是所有类的父类
- 总结
- Object -- 函数构造函数
- Object -- 手动实现一个new
- js -- 函数式编程(目前只是了解后面需要更多时间深入)
- 了解 -- 高阶函数
- 了解 -- 纯函数
- 了解 -- 函数柯里化
- 柯里化 -- 知识点
- 了解 -- 函数组合
- 了解 -- 函子
- js--小知识点
- url -- 将get请求连接参数变成对象
- event -- 一个函数处理多个事件
- try -- 处理异常
- Error -- 前段报错信息传给后台
- JSON -- 序列化
- return -- 返回true和false
- for -- 循环里初始化变量
- 命名 -- get和set怎么命名
- 链式调用
- 利用递归代替循环思路
- JS -- 技巧篇
- 技巧 -- 代码规范
- 技巧 -- 惰性载入函数
- 技巧 -- 防抖函数
- 技巧 -- 节流函数
- 插入补充(防抖/节流)
- 技巧 -- 定时器的使用
- 技巧 -- 回调函数
- 技巧 -- 分时函数
- 技巧 -- 除了return 我怎么跳出多层循环
- 技巧 -- switch 还是 if-else?
- 技巧 -- 将字符串转成对象
- 技巧 -- 函数转换
- 技巧 -- 工作记录数组对象中相同项
- JS -- 数组小文章总结
- 数组类型判断
- includes 和 indexOf
- for ... in,for ... of和forEach之间有什么区别
- map、filter、reduce、find 四种方法
- 多种形式处理数组思考方式
- for...in 和 Object.keys
- 各种知识点集合文章
- 创建数组 -- 总结
- 数组去重 -- 总结
- 获取数组中不重复的元素 -- 总结
- 比较两个数组元素相同 -- 总结
- 出现频率最高的元素 -- 总结
- 两数组交集 -- 总结
- 两数组差集 -- 总结
- 工具方法 - 总结
- 扁平化数组
- JS -- 数组技巧篇 30s
- 30s Array -- 创建数组篇(一)
- 30s Array --过滤(查询)篇章(一)
- 30s Array --过滤篇章(二)
- 30s Array -- 新增篇(一)
- 30s Array--比较篇(一)
- 30s Array -- 分组篇(一)
- 30 Array -- 删除篇(一)
- 30s Array-- 其他篇(一)
- 30s Array -- 个人感觉不常用篇章
- JS -- 对象技巧篇30s
- 30s Object -- 查(一)
- 30s Object -- 增(一)
- 30s Object -- 工具类型小方法
- 30s Object -- 跳过没看系列
- ES -- 新特性
- 变量篇章
- 变量 -- let/const/var声明
- 变量 -- 词法声明和变量声明
- 变量 -- var x = y = 100
- 变量 -- a.x = a = {n:2}
- 带标签的模板字符串
- 函数篇章
- 函数 -- 参数篇章
- 函数 -- 只能通过new创建实例
- 函数 -- 箭头函数
- 函数 -- 尾调优化
- 对象篇章
- 对象 -- 字面量写法的优化/新增方法
- 赋值篇章
- 解构赋值 -- 简单概念
- 解构赋值 -- 对象解构
- 解构赋值 -- 数组解构
- 解构赋值 -- 函数参数
- Symbol 属性
- Set 和 Map
- Set -- 去重/交、并、差集
- Map -- 集合
- 类class篇章
- ES6 和 ES5 的写法比较
- es6 -- mixin函数继承
- es6 -- 创建一个接口
- ES5 VS ES6 -- class 编译
- 数组新功能
- 创建/转换 -- 数组
- 迭代器和生成器
- es6 -- 迭代器
- es6 -- 生成器
- for-of 循环的是什么
- 做一个异步执行器
- 代理和反射
- Proxy -- 使用
- Reflect -- 使用
- Proxy 和 Reflect 结合使用
- 运算符 -- 展开运算符
- ES7 -- 新特性
- ES8 -- 新特性
- ES9 -- 新特性
- ES10 -- 新特性
- ES11 -- 新特性
- ES12 -- 新特性
- ES13 -- 新特性
- ES6 和 ES8 的异步
- js -- 异步、同步、阻塞、非阻塞
- js -- 如何做到异步的
- js -- 引擎线程,GUI渲染线程,浏览器事件触发线程
- js -- 如何通过事件循环运行异步
- js -- 误区任务回调就是异步
- js -- 宏任务和微任务
- 参考文章推荐阅读
- js -- callback 还是 promise
- js -- Promise 初识
- js -- 自己实现一个Promise
- js -- Promise 更多用法
- 再来看setTimeout 和for
- js -- ES8 异步 async await
- js -- 红绿灯问题
- js -- 倒计时
- 异步图片预加载
- 手动触发异步
- 异步题
- JS -- 模块化
- CommonJS -- 在node服务器端
- AMD 模块化规范
- ES Modules
- ES Modules -- 使用特点
- import 和 export 使用
- export 和 import -- 执行
- 其他用法
- systemjs
- 对比区别
- 使用babel 或webpack进行模块打包
- Jq -- 细节
- JS -- 性能优化
- 图片预加载
- js -- 正则
- 设计原则和编程技巧
- 设计原则 -- 单一职责
- js -- 设计模式
- 设计模式 -- 简单理解
- 一、鸭子类型
- 1.1 多态概念
- 1.2 小章节记录 -- 封装
- 1.3.多态和鸭子
- 设计模式 -- 单例模式(透明、惰性)
- 单例模式 -- js单例和惰性单例
- ES6 -- 写单例模式
- 设计模式 -- 策略模式
- 策略模式 -- 表单验证
- 策略模式 -- 表单验证多规则
- 策略模式和多态区别
- 设计模式 -- 代理模式(代理被代理相同方法)
- 代理模式 -- 分类
- 代理模式 -- 总结
- 设计模式 -- 迭代器模式
- 设计模式 -- 观察者(发布订阅)模式
- 观察者 和 发布订阅区别
- 发布订阅模式 -- 现在通用模型
- 发布订阅模式 -- 书中实际案例
- 发布订阅模式--全局发布订阅对象
- 发布订阅模式 -- vue
- 设计模式 -- 命令模式(对象方法拆分指令)
- 命令模式 -- js 自带
- 命令模式 -- 撤销和重做
- 命令模式 -- 命令和策略的区别
- 设计模式 -- 组合模式(树)
- 组合模式 -- 拆分了解
- 组合模式 -- java角度
- 组合模式 -- 书中案例扫描文件夹
- 组合模式 -- 注意点
- 组合模式 -- 引用父对象(书中案例)
- 组合模式 -- 小的案例
- 组合模式 -- 总结
- 设计模式 -- 模板方法(抽象类继承)
- 模板方法 -- 前端角度思考
- 模板方法 -- java 思考方式
- 模板方法 -- js没有抽象类
- 模板方法 -- 钩子方法
- 模板方法 -- 用js方式来实现
- 设计模式 -- 享元模式
- 享元、单例、对象池
- 享元模式 -- 前端角度思考
- 享元模式 -- 代码案例
- 享元模式 -- 适用和内外部状态
- 额外扩展 -- 对象池
- 设计模式 -- 职责链模式
- 职责链 -- 前端角度
- 职责链和策略
- 职责链 -- 异步的职责链
- 职责链 -- AOP
- 职责链 -- 改造迭代器模式的代码
- 设计模式 -- 中介者模式
- 中介者模式 -- 前端角度(一)
- 中介者模式 -- 前端角度(二)
- 中介者模式 -- 前端角度(三)
- 中介者模式 -- 总结
- 设计模式 -- 装饰者模式
- 装饰者模式 -- es5 解决方案
- 装饰器模式 -- 和代理模式区别
- 设计模式 -- 状态模式
- 状态模式 -- 前端思想
- 状态模式 -- 文件上传案例
- 状态模式 -- 和策略模式的区别
- 设计模式 -- 适配器模式
- js -- 代码重构
- 重构 -- 方法封装
- 重构 -- 抽象函数
- 高阶函数 -- 范式
- 状态管理方案
- Node -- 学习篇
- Node -- 服务端运行的js
- node -- Global(全局成员)
- node -- Buffer缓冲器
- node -- 路径操作
- node -- 文件的读写
- node -- 目录操作
- node -- HTTP
- HTTP -- 响应头常见类型
- HTTP -- 处理Get
- HTTP -- 处理Post
- HTTP -- 简单的案例
- Express
- Express -- 中间件
- Express -- 处理post/get
- Express -- 模板引擎
- Express -- 简单案例和目录搭建
- Express -- 数据库service.js
- Express -- json/url
- Express -- 配合数据库
- 配合数据库 -- 简单的登录
- npm -- js包管理
- npm -- 淘宝镜像
- nrm -- 更多镜像的选择
- yarn -- 包管理
- yarn -- 清理缓存
- WebPack -- 模块化开发
- webPack -- 安装、使用、打包
- webPack -- 使用配置文件
- 延伸 -- base64图片优劣
- webPack -- 完整配置