>[success] # composition API -- VUEX
1. 可以通过 获取`this.$store` 来进行调用里面提供数据对象
* **state**-- 保存数据状态
* **mutations** -- 对`state `中的数据更改都是通过`mutations`中的方法操控,`Vuex `不提倡直接更改`state`中的数据
* **getters** -- 当我们要获取`state`中的方法的时候从`getter`中取值
* **Action** -- 异步获取请求参数赋值,他会操控`mutations`,再让`mutations`给`state`赋值
* **module**-- store 分割成模块(module)。每个模块拥有自己的 state、mutation、action、getter、甚至是嵌套子模块
>[info] ## 综合案例
~~~
// 创建vuex
import { createStore } from "vuex";
const store = createStore({
state: () => {
return {
name: "wwww",
age: 12,
friends: [
{ id: 111, name: "a", age: 20 },
{ id: 112, name: "b", age: 30 },
{ id: 113, name: "c", age: 25 },
],
};
},
getters: {
// 第一个参数当前state
getName(state) {
return state.name;
},
// 第一个参数当前state 第二个参数是getters
getInfo(state, getters) {
return state.age + getters.getName;
},
// 通过返回一个函数达到 让 getter 可以接收参数
getFriendById(state) {
return function (id) {
const friend = state.friends.find((item) => item.id === id);
return friend;
};
},
},
mutations: {
// 第一个参数 state ,第二个参数调用传入的值
changeName(state, payload) {
state.name = payload;
},
},
actions: {
/** 处理函数总是接受 context 作为第一个参数,context 对象包含以下属性
* state, // 等同于 `store.state`,若在模块中则为局部状态
* rootState, // 等同于 `store.state`,只存在于模块中
* commit, // 等同于 `store.commit`
* dispatch, // 等同于 `store.dispatch`
* getters, // 等同于 `store.getters`
* rootGetters // 等同于 `store.getters`,只存在于模块中
*/
incrementAction(context, payload) {
// console.log(context.commit) // 用于提交mutation
// console.log(context.getters) // getters
// console.log(context.state) // state
context.commit("changeName", payload);
},
},
});
export default store;
~~~
>[danger] ##### 特殊说明 -- state
1. `composition Api` 使用方式比较多,但推荐直接使用 `toRefs` 即可
2. 想使用`mapState` 这类 由于其映射出来的对象,所有要获取指定`key`对应的`function`,但要注意 `setup` 此时`this ` 指向问题,你需要手动指定`this`,否则执行失败
* 执行效果图
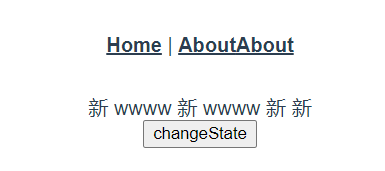
~~~html
<template>
<div>
{{ store.state.name }}
{{ name }}
{{ cname }}
{{ tAname }}
{{ tBname }}
{{ rName }}
</div>
<button @click="changeState">changeState</button>
</template>
<script setup>
import { computed, toRefs } from "vue";
import { useStore, mapState } from "vuex";
const store = useStore();
// 方法一 获取 state 依次赋值
const name = store.state.name; // 非响应
// 方法二 使用计算属性
const cname = computed(() => store.state.name);
// 方法三使用 mapState 但需要自定义this 指向
const tAname = mapState(["name"]).name.apply({ $store: store }); // 非响应
const tBname = computed(mapState(["name"]).name.bind({ $store: store }));
console.log(mapState(["name"]));
// -----------最简单的方法 toRefs 解构--------------
const { name: rName } = toRefs(store.state);
// 强制改变state
function changeState() {
store.state.name = "新";
}
</script>
~~~
* 如果非要使用 `mapState `执行可以封装一个`hooks`
~~~
import { computed } from 'vue'
import { useStore, mapState } from 'vuex'
export default function useState(mapper) {
const store = useStore()
const stateFnsObj = mapState(mapper)
const newState = {}
Object.keys(stateFnsObj).forEach(key => {
newState[key] = computed(stateFnsObj[key].bind({ $store: store }))
})
return newState
}
~~~
~~~
// 使用 封装的 useState,其实和toRefs 一样
const { name, level } = useState(["name", "level"])
~~~
>[danger] ##### 其他综合使用
~~~html
<template>
<div>
{{ getName }}
</div>
<button @click="changeName('111')">changeState</button>
<button @click="increment('111')">incrementAction</button>
<button @click="mapActions('111')">mapActions</button>
</template>
<script setup>
import { toRefs } from "vue";
import { useStore, mapMutations, mapActions } from "vuex";
const store = useStore();
const { getName } = toRefs(store.getters);
// 方式 一
const changeName = (name) => store.commit("changeName", name);
// 方式二 手动的映射和绑定
// const mutations = mapMutations(["changeName"]);
// const newMutations = {};
// Object.keys(mutations).forEach((key) => {
// newMutations[key] = mutations[key].bind({ $store: store });
// });
// const { changeName } = newMutations;
// ------------ action ----------
// 1.使用默认的做法
function increment(name) {
store.dispatch("incrementAction", name);
}
// 2.在setup中使用mapActions辅助函数
// const actions = mapActions(["incrementAction", "changeNameAction"])
// const newActions = {}
// Object.keys(actions).forEach(key => {
// newActions[key] = actions[key].bind({ $store: store })
// })
// const { incrementAction, changeNameAction } = newActions
</script>
~~~
- 官网给的工具
- 声明vue2 和 vue3
- 指令速览
- Mustache -- 语法
- v-once -- 只渲染一次
- v-text -- 插入文本
- v-html -- 渲染html
- v-pre -- 显示原始的Mustache标签
- v-cloak -- 遮盖
- v-memo(新)-- 缓存指定值
- v-if/v-show -- 条件渲染
- v-for -- 循环
- v-bind -- 知识
- v-bind -- 修饰符
- v-on -- 点击事件
- v-model -- 双向绑定
- 其他基础知识速览
- 快速使用
- 常识知识点
- key -- 作用 (后续要更新)
- computed -- 计算属性
- watch -- 侦听
- 防抖和节流
- vue3 -- 生命周期
- vue-cli 和 vite 项目搭建方法
- vite -- 导入动态图片
- 组件
- 单文件组件 -- SFC
- 组件通信 -- porp
- 组件通信 -- $emit
- 组件通信 -- Provide / Inject
- 组件通信 -- 全局事件总线mitt库
- 插槽 -- slot
- 整体使用案例
- 动态组件 -- is
- keep-alive
- 分包 -- 异步组价
- mixin -- 混入
- v-model-- 组件
- 使用计算属性
- v-model -- 自定义修饰符
- Suspense -- 实验属性
- Teleport -- 指定挂载
- 组件实例 -- $ 属性
- Option API VS Composition API
- Setup -- 组合API 入口
- api -- reactive
- api -- ref
- 使用ref 和 reactive 场景
- api -- toRefs 和 toRef
- api -- readonly
- 判断性 -- API
- 功能性 -- API
- api -- computed
- api -- $ref 使用
- api -- 生命周期
- Provide 和 Inject
- watch
- watchEffect
- watch vs. watchEffect
- 简单使用composition Api
- 响应性语法糖
- css -- 功能
- 修改css -- :deep() 和 var
- Vue3.2 -- 语法
- ts -- vscode 配置
- attrs/emit/props/expose/slots -- 使用
- props -- defineProps
- props -- defineProps Ts
- emit -- defineEmits
- emit -- defineEmits Ts
- $ref -- defineExpose
- slots/attrs -- useSlots() 和 useAttrs()
- 自定义指令
- Vue -- 插件
- Vue2.x 和 Vue3.x 不同点
- $children -- 移除
- v-for 和 ref
- attribute 强制行为
- 按键修饰符
- v-if 和 v-for 优先级
- 组件使用 v-model -- 非兼容
- 组件
- h -- 函数
- jsx -- 编写
- Vue -- Router
- 了解路由和vue搭配
- vueRouter -- 简单实现
- 安装即使用
- 路由懒加载
- router-view
- router-link
- 路由匹配规则
- 404 页面配置
- 路由嵌套
- 路由组件传参
- 路由重定向和别名
- 路由跳转方法
- 命名路由
- 命名视图
- Composition API
- 路由守卫
- 路由元信息
- 路由其他方法 -- 添加/删除/获取
- 服务器配置映射
- 其他
- Vuex -- 状态管理
- Option Api -- VUEX
- composition API -- VUEX
- module -- VUEX
- 刷新后vuex 数据同步
- 小技巧
- Pinia -- 状态管理
- 开始使用
- pinia -- state
- pinia -- getter
- pinia -- action
- pinia -- 插件 ??
- Vue 源码解读
- 开发感悟
- 练手项目