成功的对表单进行输入后,本节我们开始按规范开发数据的提交功能。
## 提交班级信息
```
export class AddComponent implements OnInit {
/*当发生请求错误时,显示该信息*/
public static errorMessage = '数据保存失败,这可能是由于网络的原因引起的';
name: FormControl;
teacherId: FormControl;
/*当该值不为空时,可以显示在前台并提示用户*/
message: string;
constructor(private httpClient: HttpClient) {
}
ngOnInit() {
this.name = new FormControl('');
this.teacherId = new FormControl();
}
onSubmit(): void {
const url = 'http://localhost:8080/Klass';
const klass = new Klass(undefined, this.name.value,
new Teacher(parseInt(this.teacherId.value➊, 10), undefined, undefined)
);
this.httpClient.post(url, klass)
.subscribe(() => {
console.log('保存成功');
}, (response) => {
console.log(`向${url}发起的post请求发生错误` + response);
this.setMessage(AddComponent.errorMessage);
});
}
/**
* 使用传的值来设置message,并在1.5秒后将消息置空
* @param message 消息
*/
private setMessage(message: string): void {
this.message = message;
setTimeout(() => {
this.message = '';
}, 1500);
}
}
```
* ➊ FormController获取的值的类型为string,Teacher中的ID的类型为number。使用parseInt(字符串,进制)将字符串转为number使类型相匹配。
## 测试
我们测试点保存按钮点击后,组件是否按预期提交了相应的请求:
klass/add/add.component.spec.ts
```
/**
* 设置表单数据
* 点击按钮发起请求
* 断言:请求地址、请求方法、请求主体数据
*/
fit('保存按钮点击后,提交相应的http请求', () => {
httpTestingController = TestBed.get(HttpTestingController);
expect(component).toBeTruthy();
component.name.setValue('test3');
component.teacherId.setValue('3');
fixture.whenStable().then(() => {
const debugElement: DebugElement = fixture.debugElement;
const submitButtonElement = debugElement.query(By.css('button'));
const submitButton: HTMLButtonElement = submitButtonElement.nativeElement;
submitButton.click();
const req = httpTestingController.expectOne('http://localhost:8080/Klass');
expect(req.request.method).toEqual('POST'); ➊
const klass: Klass = req.request.body.valueOf(); ➋
console.log(klass);➌
expect(klass.name).toEqual('test3');➍
expect(klass.teacher.id).toEqual(3);➍
});
});
```
* ➊ 断言请求方法为POST
* ➋ 获取请求主体数据
* ➌ 在终端及浏览器控制中中打印klass数据信息
* ➍ 断言成功传入了名称及教师ID
```
LOG: Klass{id: undefined, name: 'test3', teacher: Teacher{id: 3, name: undefined, username: undefined, email: undefined, sex: undefined}} ➊
Chrome 78.0.3904 (Mac OS X 10.13.6): Executed 1 of 10 SUCCESS (0 secs / 0.258 secs)
LOG: Klass{id: undefined, name: 'test3', teacher: Teacher{id: 3, name: undefined, username: undefined, email: undefined, sex: undefin➋
Chrome 78.0.3904 (Mac OS X 10.13.6): Executed 1 of 10 (skipped 9) SUCCESS (3.672 secs / 0.258 secs)
TOTAL: 1 SUCCESS
TOTAL: 1 SUCCESS
```
* ➊ 终端中打印的测试数据
* ➋ 浏览器打印日志
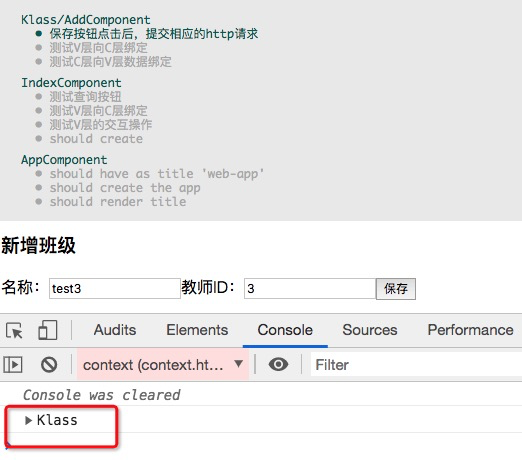
## 模拟响应数据
klass/add/add.component.spec.ts
```
expect(klass.name).toEqual('test3');
expect(klass.teacher.id).toEqual(3);
req.flush(null➊, {status: 201➋, statusText: 'Created'➌}); ✚
});
```
* ➊ 返回空数据。
* ➋ 新增成功,返回状态码201(行业惯例)。
* ➌ 返回对状态码的说明。
测试:
```
LOG: Klass{id: undefined, name: 'test3', teacher: Teacher{id: 3, name: undefined, username: undefined, email: undefined, sex: undefined}}
Chrome 78.0.3904 (Mac OS X 10.13.6): Executed 1 of 10 SUCCESS (0 secs / 0.097 secs)
LOG: Klass{id: undefined, name: 'test3', teacher: Teacher{id: 3, name: undefined, username: undefined, email: undefined, sex: undefinLOG: '保存成功'
Chrome 78.0.3904 (Mac OS X 10.13.6): Executed 1 of 10 SUCCESS (0 secs / 0.097 secs)
Chrome 78.0.3904 (Mac OS X 10.13.6): Executed 1 of 10 (skipped 9) SUCCESS (0.121 secs / 0.097 secs)
TOTAL: 1 SUCCESS
TOTAL: 1 SUCCESS
```
> 最后,我们将测试完毕的方法名由`fit`变更为`it`。
# 参考文档
| 名称 | 链接 | 预计学习时长(分) |
| --- | --- | --- |
| 源码地址 | [https://github.com/mengyunzhi/spring-boot-and-angular-guild/releases/tag/step3.3.2](https://github.com/mengyunzhi/spring-boot-and-angular-guild/releases/tag/step3.3.2) | - |
| 测试http请求 | [https://www.angular.cn/guide/http#testing-http-requests](https://www.angular.cn/guide/http#testing-http-requests) | 10 |
| TestRequest | [https://www.angular.cn/api/common/http/testing/TestRequest#request](https://www.angular.cn/api/common/http/testing/TestRequest#request) | 5 |
- 序言
- 第一章:Hello World
- 第一节:Angular准备工作
- 1 Node.js
- 2 npm
- 3 WebStorm
- 第二节:Hello Angular
- 第三节:Spring Boot准备工作
- 1 JDK
- 2 MAVEN
- 3 IDEA
- 第四节:Hello Spring Boot
- 1 Spring Initializr
- 2 Hello Spring Boot!
- 3 maven国内源配置
- 4 package与import
- 第五节:Hello Spring Boot + Angular
- 1 依赖注入【前】
- 2 HttpClient获取数据【前】
- 3 数据绑定【前】
- 4 回调函数【选学】
- 第二章 教师管理
- 第一节 数据库初始化
- 第二节 CRUD之R查数据
- 1 原型初始化【前】
- 2 连接数据库【后】
- 3 使用JDBC读取数据【后】
- 4 前后台对接
- 5 ng-if【前】
- 6 日期管道【前】
- 第三节 CRUD之C增数据
- 1 新建组件并映射路由【前】
- 2 模板驱动表单【前】
- 3 httpClient post请求【前】
- 4 保存数据【后】
- 5 组件间调用【前】
- 第四节 CRUD之U改数据
- 1 路由参数【前】
- 2 请求映射【后】
- 3 前后台对接【前】
- 4 更新数据【前】
- 5 更新某个教师【后】
- 6 路由器链接【前】
- 7 观察者模式【前】
- 第五节 CRUD之D删数据
- 1 绑定到用户输入事件【前】
- 2 删除某个教师【后】
- 第六节 代码重构
- 1 文件夹化【前】
- 2 优化交互体验【前】
- 3 相对与绝对地址【前】
- 第三章 班级管理
- 第一节 JPA初始化数据表
- 第二节 班级列表
- 1 新建模块【前】
- 2 初识单元测试【前】
- 3 初始化原型【前】
- 4 面向对象【前】
- 5 测试HTTP请求【前】
- 6 测试INPUT【前】
- 7 测试BUTTON【前】
- 8 @RequestParam【后】
- 9 Repository【后】
- 10 前后台对接【前】
- 第三节 新增班级
- 1 初始化【前】
- 2 响应式表单【前】
- 3 测试POST请求【前】
- 4 JPA插入数据【后】
- 5 单元测试【后】
- 6 惰性加载【前】
- 7 对接【前】
- 第四节 编辑班级
- 1 FormGroup【前】
- 2 x、[x]、{{x}}与(x)【前】
- 3 模拟路由服务【前】
- 4 测试间谍spy【前】
- 5 使用JPA更新数据【后】
- 6 分层开发【后】
- 7 前后台对接
- 8 深入imports【前】
- 9 深入exports【前】
- 第五节 选择教师组件
- 1 初始化【前】
- 2 动态数据绑定【前】
- 3 初识泛型
- 4 @Output()【前】
- 5 @Input()【前】
- 6 再识单元测试【前】
- 7 其它问题
- 第六节 删除班级
- 1 TDD【前】
- 2 TDD【后】
- 3 前后台对接
- 第四章 学生管理
- 第一节 引入Bootstrap【前】
- 第二节 NAV导航组件【前】
- 1 初始化
- 2 Bootstrap格式化
- 3 RouterLinkActive
- 第三节 footer组件【前】
- 第四节 欢迎界面【前】
- 第五节 新增学生
- 1 初始化【前】
- 2 选择班级组件【前】
- 3 复用选择组件【前】
- 4 完善功能【前】
- 5 MVC【前】
- 6 非NULL校验【后】
- 7 唯一性校验【后】
- 8 @PrePersist【后】
- 9 CM层开发【后】
- 10 集成测试
- 第六节 学生列表
- 1 分页【后】
- 2 HashMap与LinkedHashMap
- 3 初识综合查询【后】
- 4 综合查询进阶【后】
- 5 小试综合查询【后】
- 6 初始化【前】
- 7 M层【前】
- 8 单元测试与分页【前】
- 9 单选与多选【前】
- 10 集成测试
- 第七节 编辑学生
- 1 初始化【前】
- 2 嵌套组件测试【前】
- 3 功能开发【前】
- 4 JsonPath【后】
- 5 spyOn【后】
- 6 集成测试
- 7 @Input 异步传值【前】
- 8 值传递与引入传递
- 9 @PreUpdate【后】
- 10 表单验证【前】
- 第八节 删除学生
- 1 CSS选择器【前】
- 2 confirm【前】
- 3 功能开发与测试【后】
- 4 集成测试
- 5 定制提示框【前】
- 6 引入图标库【前】
- 第九节 集成测试
- 第五章 登录与注销
- 第一节:普通登录
- 1 原型【前】
- 2 功能设计【前】
- 3 功能设计【后】
- 4 应用登录组件【前】
- 5 注销【前】
- 6 保留登录状态【前】
- 第二节:你是谁
- 1 过滤器【后】
- 2 令牌机制【后】
- 3 装饰器模式【后】
- 4 拦截器【前】
- 5 RxJS操作符【前】
- 6 用户登录与注销【后】
- 7 个人中心【前】
- 8 拦截器【后】
- 9 集成测试
- 10 单例模式
- 第六章 课程管理
- 第一节 新增课程
- 1 初始化【前】
- 2 嵌套组件测试【前】
- 3 async管道【前】
- 4 优雅的测试【前】
- 5 功能开发【前】
- 6 实体监听器【后】
- 7 @ManyToMany【后】
- 8 集成测试【前】
- 9 异步验证器【前】
- 10 详解CORS【前】
- 第二节 课程列表
- 第三节 果断
- 1 初始化【前】
- 2 分页组件【前】
- 2 分页组件【前】
- 3 综合查询【前】
- 4 综合查询【后】
- 4 综合查询【后】
- 第节 班级列表
- 第节 教师列表
- 第节 编辑课程
- TODO返回机制【前】
- 4 弹出框组件【前】
- 5 多路由出口【前】
- 第节 删除课程
- 第七章 权限管理
- 第一节 AOP
- 总结
- 开发规范
- 备用