* shallowReactive:只处理了对象内最外层属性的响应式(也就是浅响应式)
* shallowRef:只处理了 value 的响应式,不进行对象的 reactive 处理(也就是浅响应式)
* 什么时候用浅响应式呢?
* 一般情况下使用 ref 和 reactive 即可
* 如果有一个对象数据,结构比较深,但变化时只是外层属性变化时,可以使用 shallowReactive
* 如果有一个对象数据,后面会产生新的对象来替换时,可以使用 shallowRef
```html
<template>
<!-- 3. 查看界面变化 -->
<h2>reactive: {{ reactive01 }}</h2>
<h2>shallowReactive: {{ shallowReactive01 }}</h2>
<h2>ref: {{ ref01 }}</h2>
<h2>shallowRef: {{ shallowRef01 }}</h2>
<hr />
<button @click="update">更新数据</button>
</template>
<script lang="ts">
import {
defineComponent,
reactive,
ref,
shallowReactive,
shallowRef,
} from "vue";
export default defineComponent({
setup() {
/* 1. 分别用4个不同的函数创建响应式对象 */
const reactive01 = reactive({
name: "张三",
age: 20,
car: {
name: "奔驰",
price: 100,
},
});
const shallowReactive01 = shallowReactive({
name: "张三",
age: 20,
car: {
name: "奔驰",
price: 100,
},
});
const ref01 = ref({
name: "张三",
age: 20,
car: {
name: "奔驰",
price: 100,
},
});
const shallowRef01 = shallowRef({
name: "张三",
age: 20,
car: {
name: "奔驰",
price: 100,
},
});
// 2. 更新第2层的数据
// shallowXX创建的对象第1层是可以被更新并实时渲染到界面,这里就不演示了,主要演示第2层的更新
const update = () => {
// reactive01.car.price += 100
// shallowReactive01.car.price += 100
// ref01.value.car.price += 100
// shallowRef01.value.car.price += 100
};
return {
reactive01,
shallowReactive01,
ref01,
shallowRef01,
update,
};
},
});
</script>
```
<br/>
更新数据时有以下2种组合,每种组合会产生不同的效果。
**1. shallowXX函数创建的对象单独更新**
```js
// 更新第2层的数据
const update = () => {
shallowReactive01.car.price += 100;
shallowRef01.value.car.price += 100;
console.log(shallowReactive01.car.price, shallowRef01.value.car.price);
//200 200
//300 300
//400 400
//500 500
//... ...
};
```
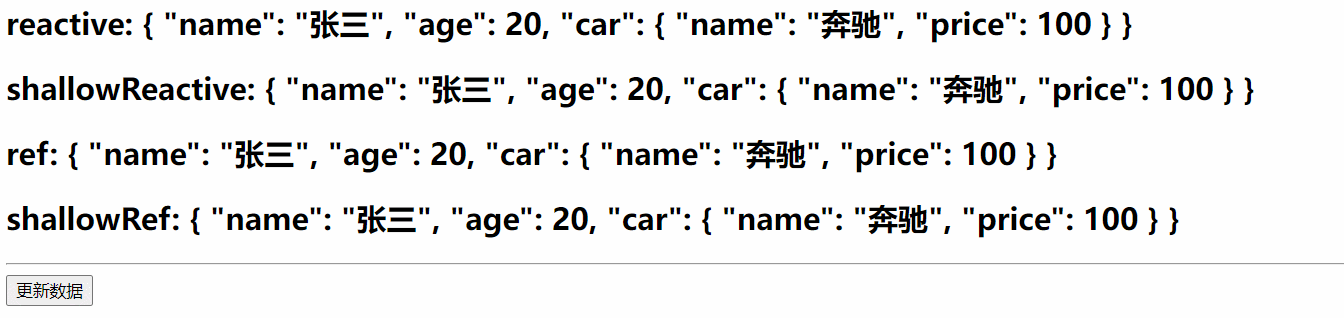
数据确实已经被更新,但是不会显示到界面上。
<br/>
**2. shallowXX与非shallow创建的对象放在一起更新**
```js
// 更新第2层的数据
const update = () => {
//下面三种组合更新效果都是一样的
//(1)reactive与shallowXX放在一起更新
//(2)ref与shallowXX放在一起更新
//(3)reactive、ref、shallowXX放在一起更新
reactive01.car.price += 100
shallowReactive01.car.price += 100;
ref01.value.car.price += 100
shallowRef01.value.car.price += 100;
console.log(shallowReactive01.car.price, shallowRef01.value.car.price);
//200 200
//300 300
//400 400
//500 500
//... ...
}
```
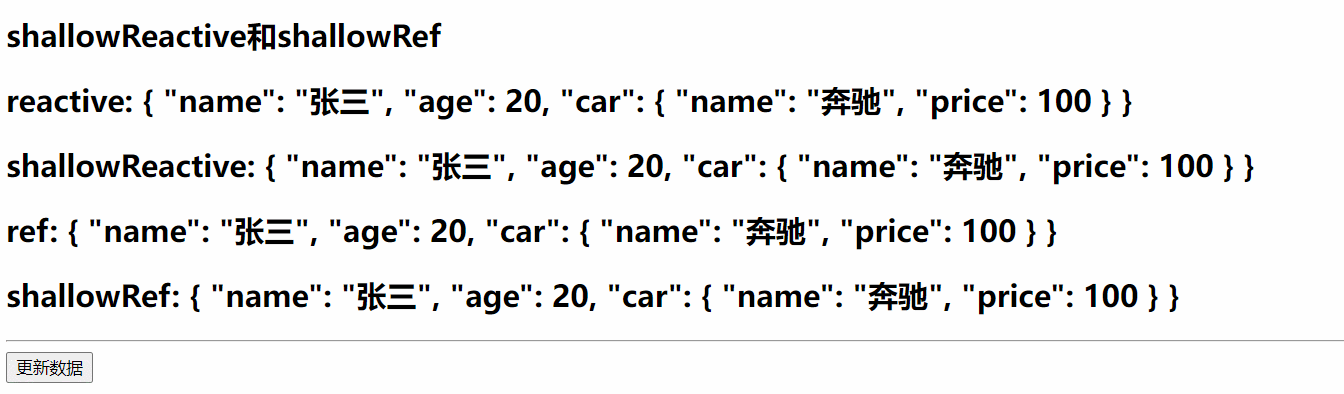
将 shallowXX 函数创建的对象与非 shallow 函数创建的对象放在一起更新,shallowXX 创建的对象的深层会被更新并实时渲染到界面上。
- nodejs
- 同时安装多个node版本
- Vue3
- 创建Vue3项目
- 使用 vue-cli 创建
- 使用 vite 创建
- 常用的Composition API
- setup
- ref
- reactive
- 响应数据原理
- setup细节
- reactive与ref细节
- 计算属性与监视
- 生命周期函数
- toRefs
- 其它的Composition API
- shallowReactive与shallowRef
- readonly与shallowReadonly
- toRaw与markRaw
- toRef
- customRef
- provide与inject
- 响应式数据的判断
- 组件
- Fragment片断
- Teleport瞬移
- Suspense
- ES6
- Promise对象
- Promise作用
- 状态与过程
- 基本使用
- 常用API
- async与await
- Axios