## 13) 数据传输协议protocol buffer
### 13.1 简介
**Google Protocol Buffer** (简称 Protobuf)是google旗下的一款轻便高效的结构化数据存储格式,平台无关、语言无关、可扩展,可用于通讯协议和数据存储等领域。所以很适合用做数据存储和作为不同应用,不同语言之间相互通信的数据交换格式,只要实现相同的协议格式即同一 proto文件被编译成不同的语言版本,加入到各自的工程中去。这样不同语言就可以解析其他语言通过 protobuf序列化的数据。目前官网提供了 C++,Python,JAVA,GO等语言的支持。google在2008年7月7号将其作为开源项目对外公布。
**tips:**
1. 啥叫平台无关?Linux、mac和Windows都可以用,32位系统,64位系统通吃
2. 啥叫语言无关?C++、Java、Python、Golang语言编写的程序都可以用,而且可以相互通信
3. 那啥叫可扩展呢?就是这个数据格式可以方便的增删一部分字段啦~
4. 最后,啥叫序列化啊?解释得通俗点儿就是把复杂的结构体数据按照一定的规则编码成一个字节切片
### 13.2 数据交换格式
#### A)常用的数据交换格式有三种:
1. `json`: 一般的web项目中,最流行的主要还是 json。因为浏览器对于json 数据支持非常好,有很多内建的函数支持。
2. `xml`: 在 webservice 中应用最为广泛,但是相比于 json,它的数据更加冗余,因为需要成对的闭合标签。json 使用了键值对的方式,不仅压缩了一定的数据空间,同时也具有可读性。
3. `protobuf`: 是后起之秀,是谷歌开源的一种数据格式,适合高性能,对响应速度有要求的数据传输场景。因为 profobuf 是二进制数据格式,需要编码和解码。数据本身不具有可读性。因此只能反序列化之后得到真正可读的数据。
#### B)protobuf的优势与劣势
> 优势:
1:序列化后体积相比Json和XML很小,适合网络传输
2:支持跨平台多语言
3:消息格式升级和兼容性还不错
4:序列化反序列化速度很快,快于Json的处理速速
> 劣势:
1:应用不够广(相比xml和json)
2:二进制格式导致可读性差
3:缺乏自描述
------
### 13.3 protobuf环境安装
##### A) protobuf 编译工具安装
1、下载 protoBuf:
```
cd $GOPATH/src/
git clone https://github.com/protocolbuffers/protobuf.git
```
2、或者直接将压缩包拖入后解压
```
unzip protobuf.zip
```
3、安装依赖库
```
sudo apt-get install autoconf automake libtool curl make g++ unzip libffi-dev -y
```
4、进入目录
```
cd protobuf/
```
5、自动生成configure配置文件:
```
./autogen.sh
```
6、配置环境:
```
./configure
```
7、编译源代码(时间比较长):
```
make
```
8、安装
```
sudo make install
```
9、刷新共享库 (很重要的一步啊)
```
sudo ldconfig
```
10、成功后需要使用命令测试
```
protoc -h
```
#####
### 13.4 protobuf与C++编程
首先创建一个msg.proto文件,里面定义协议的格式
> protobuf_test/msg.proto
```protobuf
syntax="proto3";
package protobuf_test;
message helloworld
{
int32 id = 1; // ID
string str = 2; // str
int32 opt = 3; //optional field
}
```
写好 proto 文件之后就可以用 Protobuf 编译器将该文件编译成目标语言了。本例中我们将使用 C++。
使用如下命令可以生成我们所需要的.cc与.h
```bash
protoc --cpp_out=. ./*.proto
```
执行上述命令将生成msg.pb.cc与msg.pb.h,执行时需要在文件msg.proto所在的目录下执行。
为了方便,我们可以写一个脚本,方便下次执行。
> build.sh
```bash
#!/bin/bash
protoc --cpp_out=. ./*.proto
```
> protobuf_test/write.cpp
```c
#include "msg.pb.h"
#include <fstream>
#include <iostream>
using namespace std;
int main(void)
{
protobuf_test::helloworld msg1;
msg1.set_id(101);
msg1.set_str("hello");
fstream output("./log", ios::out | ios::trunc | ios::binary);
if (!msg1.SerializeToOstream(&output)) {
cerr << "Failed to write msg." << endl;
return -1;
}
return 0;
}
```
Msg1 是一个 helloworld 类的对象,set_id() 用来设置 id 的.SerializeToOstream 将对象序列化后写入一个 fstream 流。当担序列化的接口还有很多比如SerializeToString, SerializeToArray等。
> protobuf_test/read.cpp
```c
#include "msg.pb.h"
#include <fstream>
#include <iostream>
using namespace std;
void ListMsg(const protobuf_test::helloworld & msg) {
cout << msg.id() << endl;
cout << msg.str() << endl;
}
int main(int argc, char* argv[]) {
protobuf_test::helloworld msg1;
fstream input("./log", ios::in | ios::binary);
if (!msg1.ParseFromIstream(&input)) {
cerr << "Failed to parse address book." << endl;
return -1;
}
ListMsg(msg1);
}
```
现在进行编译:
```bash
$ g++ msg.pb.cc write.cpp -o write -lprotobuf
$ g++ msg.pb.cc read.cpp -o read -lprotobuf
```
执行,先执行write,再执行read。 write会将数据写进log文件中,read可以通过pb协议解析出数据到内存变量中.
---
### 关于作者:
作者:`Aceld(刘丹冰)`
mail: [danbing.at@gmail.com](mailto:danbing.at@gmail.com)
github: [https://github.com/aceld](https://github.com/aceld)
原创书籍: [https://www.kancloud.cn/@aceld](https://www.kancloud.cn/@aceld)
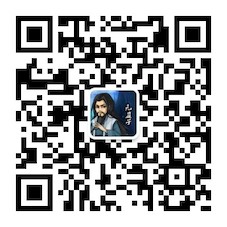
>**原创声明:未经作者允许请勿转载, 如果转载请注明出处**
- 一、Lars系统概述
- 第1章-概述
- 第2章-项目目录构建
- 二、Reactor模型服务器框架
- 第1章-项目结构与V0.1雏形
- 第2章-内存管理与Buffer封装
- 第3章-事件触发EventLoop
- 第4章-链接与消息封装
- 第5章-Client客户端模型
- 第6章-连接管理及限制
- 第7章-消息业务路由分发机制
- 第8章-链接创建/销毁Hook机制
- 第9章-消息任务队列与线程池
- 第10章-配置文件读写功能
- 第11章-udp服务与客户端
- 第12章-数据传输协议protocol buffer
- 第13章-QPS性能测试
- 第14章-异步消息任务机制
- 第15章-链接属性设置功能
- 三、Lars系统之DNSService
- 第1章-Lars-dns简介
- 第2章-数据库创建
- 第3章-项目目录结构及环境构建
- 第4章-Route结构的定义
- 第5章-获取Route信息
- 第6章-Route订阅模式
- 第7章-Backend Thread实时监控
- 四、Lars系统之Report Service
- 第1章-项目概述-数据表及proto3协议定义
- 第2章-获取report上报数据
- 第3章-存储线程池及消息队列
- 五、Lars系统之LoadBalance Agent
- 第1章-项目概述及构建
- 第2章-主模块业务结构搭建
- 第3章-Report与Dns Client设计与实现
- 第4章-负载均衡模块基础设计
- 第5章-负载均衡获取Host主机信息API
- 第6章-负载均衡上报Host主机信息API
- 第7章-过期窗口清理与过载超时(V0.5)
- 第8章-定期拉取最新路由信息(V0.6)
- 第9章-负载均衡获取Route信息API(0.7)
- 第10章-API初始化接口(V0.8)
- 第11章-Lars Agent性能测试工具
- 第12章- Lars启动工具脚本