[TOC]
# 1. 创建gateway服务
gateway需要从nacos获取服务列表和配置
**1. 创建gateway模块**
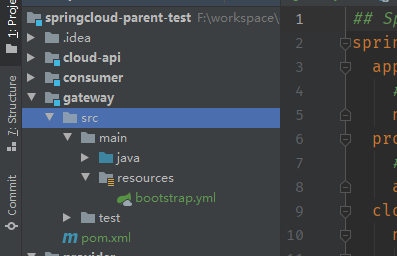
**2. pom**
~~~
<!--需要引入该jar才能使bootstrap配置文件生效-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-context</artifactId>
</dependency>
<!--服务发现-->
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
<!--服务配置-->
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-config</artifactId>
</dependency>
<!--服务网关-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-gateway</artifactId>
</dependency>
~~~
3. 启动类
~~~
@SpringBootApplication
@EnableDiscoveryClient
public class GatewayApplication {
public static void main(String[] args) {
SpringApplication.run(GatewayApplication.class, args);
}
}
~~~
4. bootstrap.yml
~~~
application:
# 应用名称
name: gateway
profiles:
# 环境配置
active: dev
cloud:
nacos:
config:
# 配置中心地址
server-addr: 192.168.56.10:8848
# 配置文件格式
file-extension: yml
group: DEFAULT_GROUP
namespace: 1b2b82fd-96d4-4c10-ac42-80264b0cc2a4
~~~
4. gateway-dev.yml
配置nacos服务注册中心和配置中心
```
server:
port: 8002
spring:
application:
name: tuna-gateway
cloud:
nacos:
discovery:
# 服务注册地址
server-addr: 192.168.56.10:8848
namespace: 1b2b82fd-96d4-4c10-ac42-80264b0cc2a4
gateway:
discovery:
locator:
lowerCaseServiceId: true
enabled: true
routes:
# 认证中心
- id: provider-router
uri: lb://provider
predicates:
- Path=/test/**
```
所有 到gateway上的请求,都会路由到服务名`provider`上
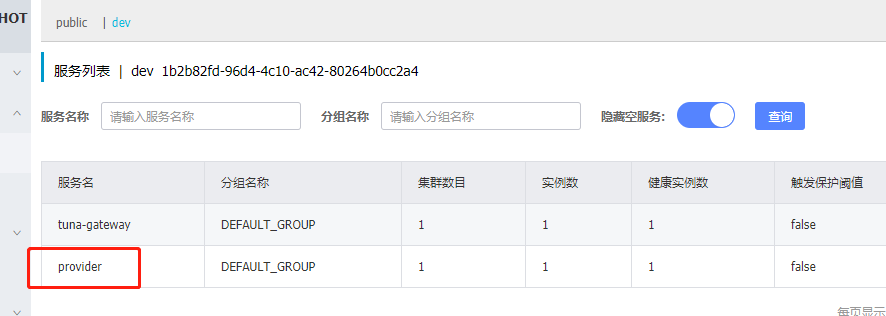
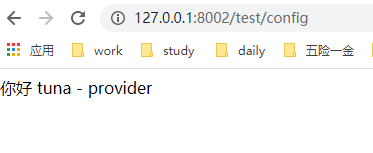
# 2. 路径重写
RewritePath GatewayFilter Factory
The RewritePath GatewayFilter Factory takes a path regexp parameter and a replacement parameter. This uses Java regular expressions for a flexible way to rewrite the request path.
application.yml.
```
spring:
cloud:
gateway:
routes:
# =====================================
- id: rewritepath_route
uri: http://example.org
predicates:
- Path=/foo/**
filters:
- RewritePath=/foo/(?<segment>.*), /$\{segment}
```
For a request path of /foo/bar, this will set the path to /bar before making the downstream request. Notice the $\\ which is replaced with $ because of the YAML spec.
修改
```
routes:
# 认证中心
- id: provider-router
uri: lb://provider
predicates:
- Path=/gateway/**
filters:
- RewritePath=/gateway/(?<segment>.*), /$\{segment}
```
RewritePath要与 predicates:
中的path相同
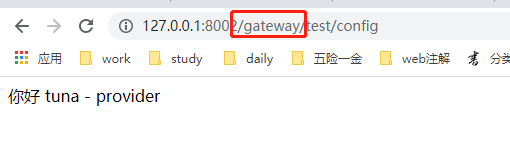
# 3. 加入filter
## 3.1 普通filter
1. 定义filter,继承
~~~
@Component
public class ValidateCodeFilter extends AbstractGatewayFilterFactory<Object> {
private final static String AUTH_URL = "/login";
private static final String CODE = "code";
@Override
public GatewayFilter apply(Object config) {
HashMap<Object, Object> res = new HashMap<>();
return (exchange, chain) -> {
ServerHttpRequest request = exchange.getRequest();
// 非登录请求,不处理
if (!StringUtils.containsIgnoreCase(request.getURI().getPath(), AUTH_URL)) {
return chain.filter(exchange);
}
try {
List<String> code = exchange.getRequest().getQueryParams().get("code");
if (code.size() == 0)
throw new RuntimeException("非法用户");
} catch (Exception e) {
ServerHttpResponse response = exchange.getResponse();
response.getHeaders().add("Content-Type", "application/json;charset=UTF-8");
return exchange.getResponse().writeWith(
Mono.just(response.bufferFactory().wrap(JSON.toJSONBytes(res.put("message", "请求失败")))));
}
return chain.filter(exchange);
};
}
}
~~~
2. 修改配置,加入filter
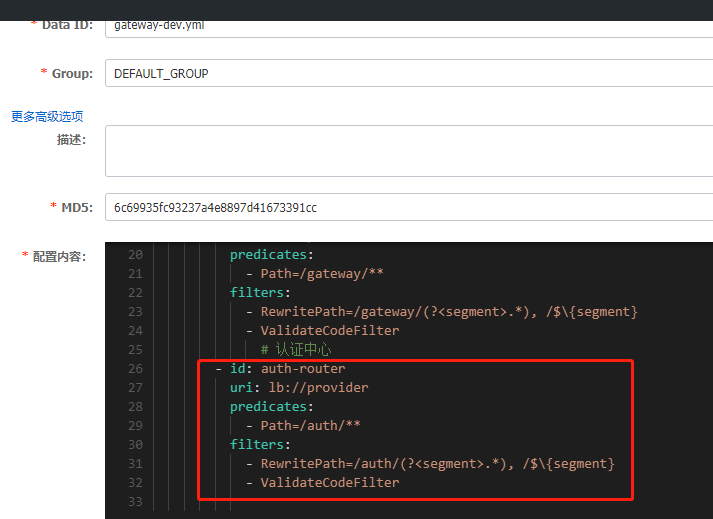
如图配置,只对/auth/开头的请求router进行过滤
请求成功
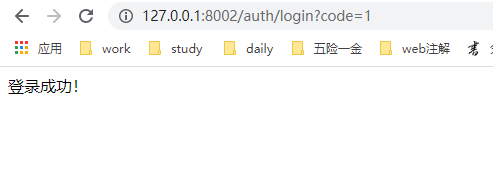
请求失败
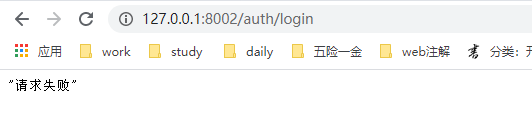
## 3.2 自定义全局filter
**无需配置,对所有的route都有效,可进行流量控制,权限等校验**
~~~
@Component
public class TokenFilter implements GlobalFilter, Ordered {
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
String token = exchange.getRequest().getQueryParams().getFirst("token");
if (token == null || token.isEmpty()) {
exchange.getResponse().setStatusCode(HttpStatus.UNAUTHORIZED);
return exchange.getResponse().setComplete();
}
return chain.filter(exchange);
}
@Override
public int getOrder() {
return -100;
}
}
~~~
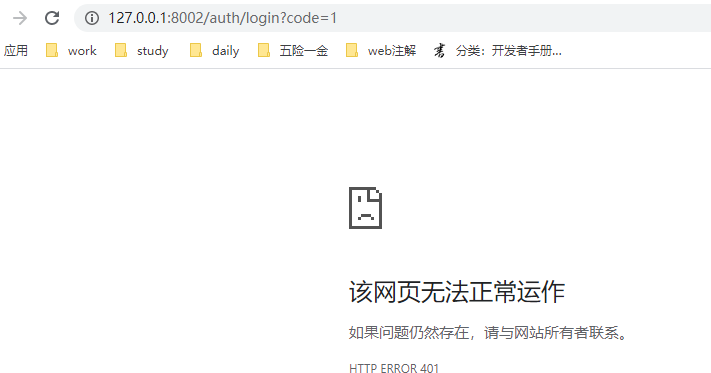
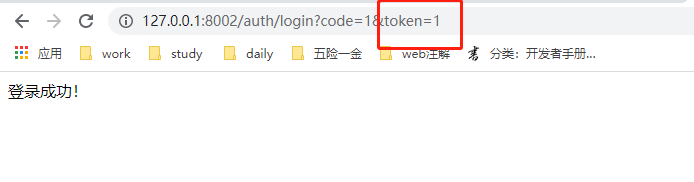
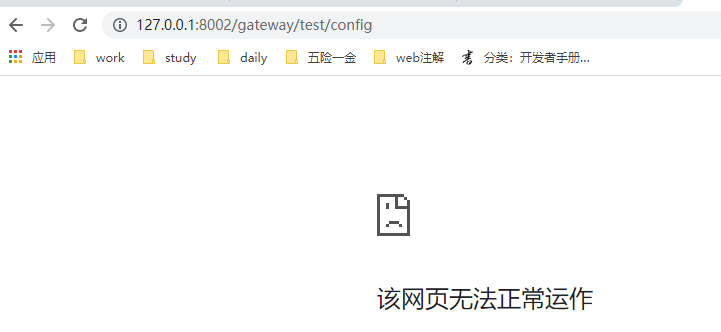
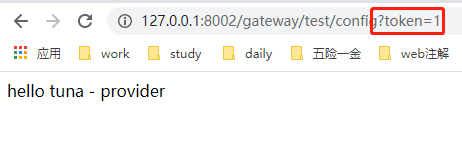
对auth和gateway两个route都进行了拦截
## 3.3 自定义局部过滤器
局部过滤器,**需要在配置文件中配置,如果配置,则该过滤器才会生效**。
**主要实现GatewayFilter, Ordered接口,并通过AbstractGatewayFilterFactory的子类注册到spring容器中,当然也可以直接继承AbstractGatewayFilterFactory,在里面写过滤器逻辑,还可以从配置文件中读取外部数据。**
只需要在亲请求处理之前和之后标记时间即可。注意此处演示的是使用配置类的形式:
~~~
package com.yefengyu.gateway.globalFilter;
import org.springframework.cloud.gateway.filter.GlobalFilter;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.core.annotation.Order;
import reactor.core.publisher.Mono;
//全局过滤器,使用配置类形式,直接构造bean,使用注解完成Ordered接口功能,统计接口调用时间
@Configuration
public class GlobalGatewayFilterConfig
{
@Bean
@Order(-100)
public GlobalFilter elapsedGlobalFilter()
{
return (exchange, chain) -> {
//调用请求之前统计时间
Long startTime = System.currentTimeMillis();
return chain.filter(exchange).then().then(Mono.fromRunnable(() -> {
//调用请求之后统计时间
Long endTime = System.currentTimeMillis();
System.out.println(
exchange.getRequest().getURI().getRawPath() + ", cost time : " + (endTime - startTime) + "ms");
}));
};
}
}
~~~
局部过滤器-简单的权限检查**
* * *
权限检查一般把信息存储在某处,请求到来之后进行核对,有权限的请求将真正执行。
1、首先编写一个工具类,对权限做管理。
~~~
package com.yefengyu.gateway.utitls;
import java.util.HashMap;
import java.util.Map;
public final class AuthUtil
{
private static Map<String, String> map = new HashMap<>();
private AuthUtil()
{
}
//程序启动的时候加载权限的信息,比如从文件、数据库中加载
public static void init()
{
map.put("tom", "123456");
}
//简单判断
public static boolean isPermitted(String name, String password)
{
return map.containsKey(name) && map.get(name).equals(password);
}
}
~~~
我们简单的将权限信息放到map中保管,init方法是初始化方法,isPermitted是对外提供一个判断是否有权限的方法。
2、服务启动的时候,需要初始化权限map,因此主启动类进行了修改:
~~~
package com.yefengyu.gateway;
import com.yefengyu.gateway.utitls.AuthUtil;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.context.event.ApplicationStartedEvent;
import org.springframework.context.ApplicationListener;
@SpringBootApplication
public class GatewayApplication
{
public static void main(String[] args)
{
SpringApplication springApplication = new SpringApplication(GatewayApplication.class);
springApplication.addListeners(new ApplicationListenerStarted());//增加监听器
springApplication.run(args);
}
private static class ApplicationListenerStarted
implements ApplicationListener<ApplicationStartedEvent>
{
@Override
public void onApplicationEvent(ApplicationStartedEvent applicationStartedEvent)
{
//权限初始化数据
AuthUtil.init();
}
}
}
~~~
3、编写一个局部过滤器,需要实现GatewayFilter, Ordered,实现相关的方法
~~~
package com.yefengyu.gateway.localFilter;
import com.yefengyu.gateway.utitls.AuthUtil;
import org.springframework.cloud.gateway.filter.GatewayFilter;
import org.springframework.cloud.gateway.filter.GatewayFilterChain;
import org.springframework.core.Ordered;
import org.springframework.http.HttpStatus;
import org.springframework.web.server.ServerWebExchange;
import reactor.core.publisher.Mono;
public class AuthGatewayFilter implements GatewayFilter, Ordered
{
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain)
{
//获取header的参数
String name = exchange.getRequest().getHeaders().getFirst("name");
String password = exchange.getRequest().getHeaders().getFirst("password");
boolean permitted = AuthUtil.isPermitted(name, password);//权限比较
if (permitted)
{
return chain.filter(exchange);
}
else
{
exchange.getResponse().setStatusCode(HttpStatus.UNAUTHORIZED);
return exchange.getResponse().setComplete();
}
}
@Override
public int getOrder()
{
return 10;
}
}
~~~
4、接着需要把上面自定义的局部过滤器加入到过滤器工厂,并且注册到spring容器中。
~~~
package com.yefengyu.gateway.localFilter;
import org.springframework.cloud.gateway.filter.GatewayFilter;
import org.springframework.cloud.gateway.filter.factory.AbstractGatewayFilterFactory;
import org.springframework.stereotype.Component;
@Component
public class AuthGatewayFilterFactory extends AbstractGatewayFilterFactory<Object>
{
@Override
public GatewayFilter apply(Object config)
{
return new AuthGatewayFilter();
}
}
~~~
5、在配置文件中进行配置,如果不配置则不启用此过滤器规则。
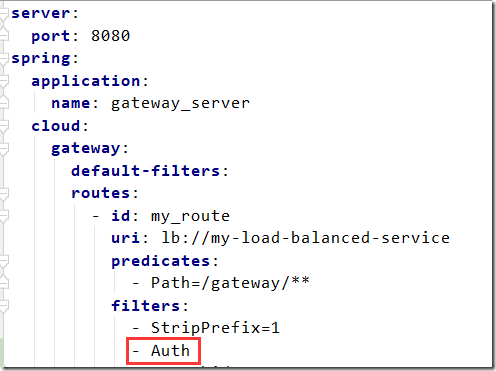
- springcloud
- springcloud的作用
- springboot服务提供者和消费者
- Eureka
- ribbon
- Feign
- feign在微服务中的使用
- feign充当http请求工具
- Hystrix 熔断器
- Zuul 路由网关
- Spring Cloud Config 分布式配置中心
- config介绍与配置
- Spring Cloud Config 配置实战
- Spring Cloud Bus
- gateway
- 概念讲解
- 实例
- GateWay
- 统一日志追踪
- 分布式锁
- 1.redis
- springcloud Alibaba
- 1. Nacos
- 1.1 安装
- 1.2 特性
- 1.3 实例
- 1. 整合nacos服务发现
- 2. 整合nacos配置功能
- 1.4 生产部署方案
- 环境隔离
- 原理讲解
- 1. 服务发现
- 2. sentinel
- 3. Seata事务
- CAP理论
- 3.1 安装
- 分布式协议
- 4.熔断和降级
- springcloud与alibba
- oauth
- 1. abstract
- 2. oauth2 in micro-service
- 微服务框架付费
- SkyWalking
- 介绍与相关资料
- APM系统简单对比(zipkin,pinpoint和skywalking)
- server安装部署
- agent安装
- 日志清理
- 统一日志中心
- docker安装部署
- 安装部署
- elasticsearch 7.x
- logstash 7.x
- kibana 7.x
- ES索引管理
- 定时清理数据
- index Lifecycle Management
- 没数据排查思路
- ELK自身组件监控
- 多租户方案
- 慢查询sql
- 日志审计
- 开发
- 登录认证
- 链路追踪
- elk
- Filebeat
- Filebeat基础
- Filebeat安装部署
- 多行消息Multiline
- how Filebeat works
- Logstash
- 安装
- rpm安装
- docker安装Logstash
- grok调试
- Grok语法调试
- Grok常用表达式
- 配置中常见判断
- filter提取器
- elasticsearch
- 安装
- rpm安装
- docker安装es
- 使用
- 概念
- 基础
- 中文分词
- 统计
- 排序
- 倒排与正排索引
- 自定义dynamic
- 练习
- nested object
- 父子关系模型
- 高亮
- 搜索提示
- kibana
- 安装
- docker安装
- rpm安装
- 整合
- 收集日志
- 慢sql
- 日志审计s
- 云
- 分布式架构
- 分布式锁
- Redis实现
- redisson
- 熔断和降级