[TOC]
>[success] # uniapp下拉刷新
在 **uniapp** 中有 **2 种下拉刷新** :
1. **下拉刷新整个页面**
2. **数据列表** 的 **下拉刷新**,这种通常与 **上拉加载** 配合使用
>[success] ## 下拉刷新整个页面
如图:
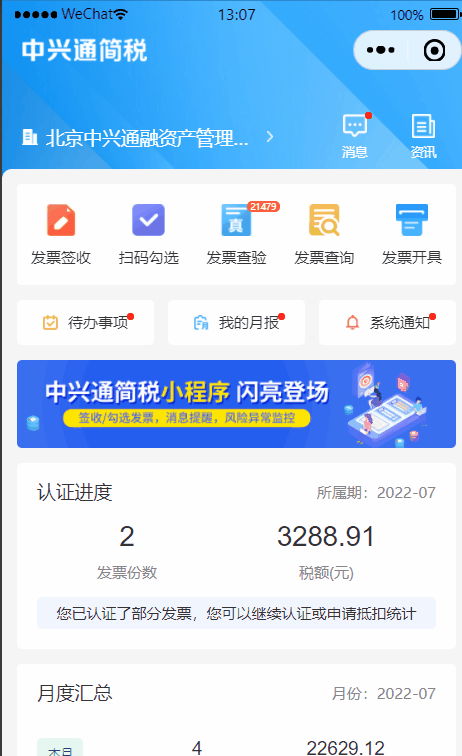
要想实现这个效果,主要需要做 **2个步骤**
1. 需要去 **pages.json** 文件中,找到 **要配置下拉刷新的页面的配置中** ,添加 `"enablePullDownRefresh": true` ,意思是开启页面刷新,如下图:
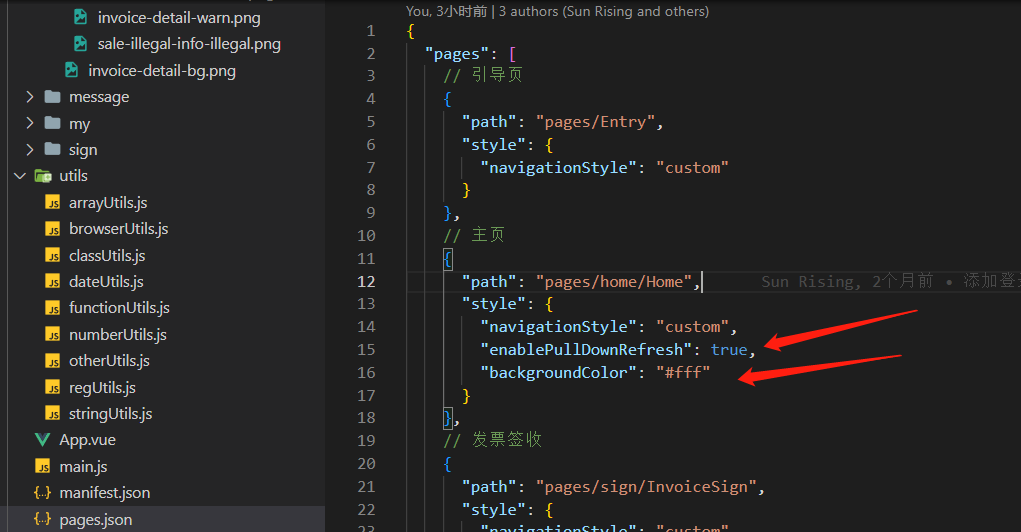
2. 上面我们在 **home** 页面配置了 **下拉刷新** ,我们需要到 **home** 页面通过 **onPullDownRefresh 生命周期函数** ,来 **监听下拉操作** ,我们可以在这个生命周期函数中执行数据接口来达到数据刷新的操作,但是使用时候需要注意,如果反复下拉刷新操作,会反复执行,需要配合 **防抖函数** 来使用,代码如下:
~~~
// 监听下拉刷新
onPullDownRefresh: zdebounce(function () {
// 初始化
this.init();
// 获取认证进度、月度信息、发票查验次数(每次显示首页时都会执行)
this.getPageInfo();
// 停止当前页面下拉刷新
uni.stopPullDownRefresh();
}, 1000),
~~~
这里需要注意,执行完成后接口后,需要手动执行 `uni.stopPullDownRefresh();` 来 **关闭下拉刷新**
>[success] ## 数据列表的下拉刷新与上拉加载
**下拉刷新** 一般都伴随着 **上拉加载** 的功能同时出现,在 **uniapp** 中如果想实现这个效果,需要使用官方提供的 [scroll-view组件](https://uniapp.dcloud.io/component/scroll-view.html#%E8%87%AA%E5%AE%9A%E4%B9%89%E4%B8%8B%E6%8B%89%E5%88%B7%E6%96%B0)来实现这 **2** 个功能,而且 **上拉加载时** 列表底部的 **提示语(没有更多数据了、加载中...)** ,都没有提供,需要我们自己去使用 **uni-ui** 中的 [uni-load-more](https://uniui.dcloud.net.cn/components/load-more.html) 来配合使用完成功能,这里具体代码
vue文件
~~~
<template>
<view class="invoice-list-container">
<!-- 内容区域 -->
<view class="wrap">
<!-- 列表 -->
<scroll-view v-if="invoiceList.length > 0" class="list" scroll-y refresher-enabled :refresher-triggered="triggered" :refresher-threshold="100" @refresherrefresh="onRefresh" @refresherrestore="onRestore" @scrolltolower="loadMore">
<view class="item" v-for="(item, index) in invoiceList" :key="index">
<view class="label">{{ item }}</view>
</view>
<!-- 上拉加载底部文字组件 -->
<uni-load-more v-if="invoiceList.length === 0 || invoiceList.length > 9" :contentText="contentTextObj" :status="loading" />
</scroll-view>
<!-- 自定义无数据样式 -->
<view v-else class="no-data">
<image src="/static/image/list-no-data.png" />
<text>暂无数据</text>
</view>
</view>
</view>
</template>
<script>
/*
* @Author: Wang Jiachong
* @Date: 2022-07-21 13:32:35
* @Last Modified by: Wang Jiachong
* @Last Modified time: 2022-07-29 11:47:47
* @Description: 发票查询列表页面
*/
// 引入组件
import uniLoadMore from '@/components/uni-load-more/uni-load-more';
// 接口
import invoiceApi from '@/api/deduction';
// 防抖函数
import { zdebounce } from '@/utils/functionUtils';
export default {
name: 'InvoiceList',
components: {
uniLoadMore,
},
data() {
return {
// 分页配置
page: {
pageNo: 1,
pageSize: 10
},
invoiceList: [], // 发票列表
loading: 'loading', // 默认显示【加载中】
// 发票对象信息
invoiceInfoObj: null,
// 加载文字配置对象
contentTextObj: {
contentnomore: '您已到底了~'
},
triggered: false // 设置当前下拉刷新状态,true 表示下拉刷新已经被触发,false 表示下拉刷新未被触发
};
},
created() {
// 初始化获取列表
this.getList();
},
methods: {
// 上拉加载事件
loadMore: zdebounce(function () {
// 无数据时 return
if (this.loading === 'noMore') return;
this.page.pageNo++;
this.getList();
}, 1000),
// 获取列表
async getList() {
try {
// 发票类型key集合
let invoiceKeys = this.fplxList.map((i) => i.key);
const params = {
data: {
orgId: this.currentCompany.orgId, // 当前组织 id
source: '1', // 1发票池 2发票签收 3勾选认证列表 4认证发票清单列表 5认证记录(勾选)6认证记录(暂不抵扣)7勾选认证智能匹配 8异常发票9:发票池异常发票查询
kprqStart: this.kprqStart, // 开票日期(开始)
kprqEnd: this.kprqEnd, // 开票日期(结束)
invoiceNumber: this.invoiceNumber, // 发票号码查询条件
xsfMc: this.xsfMc, // 销方名称查询条件
fplxList: invoiceKeys.length > 0 ? invoiceKeys : null // 发票类型条件(多选)
},
...this.page
};
const res = await invoiceApi.getInvoiceList(params);
const { data } = res;
// 发票数据列表
const list = data.list || [];
// 无数据
if (list.length === 0) {
// 无数据时关闭
this.loading = 'noMore';
}
// 下拉刷新
if (this.page.pageNo === 1) {
this.invoiceList = list;
} else {
// 上拉加载
// 每次请求到值,将新值与push到后面
this.invoiceList = [...this.invoiceList, ...list];
}
} catch (e) {
console.warn(e);
this.$message.showToast({
title: '获取发票列表数据失败'
});
} finally {
this.triggered = false;
}
},
// 自定义下拉刷新被触发
onRefresh() {
if (this.triggered) return;
this.triggered = true;
this.page.pageNo = 1;
this.loading = 'loading';
this.getList();
},
// 自定义下拉刷新被复位
onRestore() {
// 需要重置
this.triggered = false;
}
}
};
</script>
~~~
需要注意:**在上拉加载到最后一页到底了,并且页面提示【已经没有更多数据】了,如果此时进行【下拉刷新操作】,一定要把 ` this.loading = 'loading';` 改回到 `loading` 的状态** ,不然会导致列表始终只能显示第 **1** 页的数据,无法触发 **loadMore** 的方法的后续逻辑,**因为该方法中判断了 loading 等于 noMore 就 return**