[TOC]
>[success] # 处理get请求
* **get请求**,即 **客户端** 要向 **server 端** 获取数据,如查询博客列表,**查询都用get**
* 通过 **querystring** 来传递数据,如 **a.html?a=100&b=200**
* **浏览器输入url手动在后面拼接参数,直接访问,就发送get请求**
>[success] ## get请求和 querystring
1. 首先初始化项目,执行 **npm init -y** ,然后会生成初始化的 **package.json** 文件,把里面的 **main** 入口配置文件名改成 **app.js**
**package.json**
~~~
{
"name": "node",
"version": "1.0.0",
"description": "",
"main": "app.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
~~~
2. 创建 **app.js** ,然后写处理 **get请求** 的逻辑
**app.js**
~~~
// 1. 引入node自带的http模块
const http = require('http')
// 2. 引入querystring模块
const querystring = require('querystring')
// 3. 通过http创建服务
const server = http.createServer((req, res) => {
console.log(req.method) // GET
// 4. 获取请求的完整 url ,http://127.0.0.1:8000/?a=100&b=200
const url = req.url
// 5. 把 a=100&b=200 解析成对象 {"a":"100","b":"200"}
req.query = querystring.parse(url.split('?')[1])
// 6. 把参数转换成json字符串返回
res.end(JSON.stringify(req.query))
})
// 7. 监听8000端口
server.listen(8000)
~~~
在编辑器终端执行 **node app.js** 启动该文件的服务,然后在浏览器输入 **localhost:8000或127.0.0.1** ,因为这里的 **http** 服务是 **8000** 端口,此时能看到页面有一个空对象
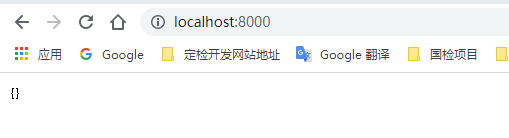
在 **url** 后面拼接参数,就可以看到拼接的参数被后台成功返回,并且展示到了页面上
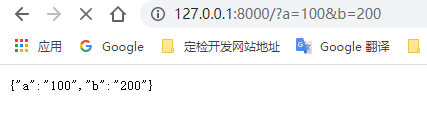
这样就成功的解析到了 **get** 请求的参数。
- NodeJS基础
- 什么是NodeJS
- npm
- Node.js+Express+Koa2+开发Web Server博客
- 下载和安装node
- nodejs和js的区别
- commonjs-演示
- nodejs如何debugger
- server端与前端的区别
- 项目需求分析
- 开发接口(不使用任何框架)
- http-概述
- 处理get请求
- 处理post请求
- 处理http请求的综合示例
- 搭建开发环境
- 初始化并且开发路由
- 开发博客项目之数据存储
- MySql介绍
- 数据库操作(创建和增、删、查)
- Nodejs 操作 Mysql
- Nodejs 链接 mysql 做成工具
- API 对接 MySQL
- 开发博客项目之登陆
- cookie-介绍
- cookie用于登录验证
- cookie做限制