## **1.创建pojo包,包名为:com.baishenghua200.pojo**
在该包下创建以下类:
### **1.1创建导航菜单类**
>创建导航菜单类Menu200.java
四个个属性:菜单编号、菜单名称、菜单地址和菜单序号。属性名上也加上学号后三位
重写hashCode()和equals()方法
重写toString()方法
实现可序列化接口
创建有参和无参构造方法
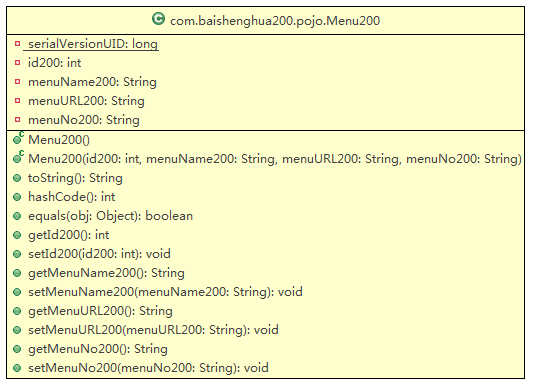
```
package com.baishenghua200.pojo;
import java.io.Serializable;
/**
* Menu200.java(导航菜单类)
* @desc 导航菜单
* @author 柏圣华
* @date 2022-1-3
*
*/
public class Menu200 implements Serializable{
private static final long serialVersionUID = 1L;
private int id200;//导航菜单编号
private String menuName200;//导航菜单名称
private String menuURL200;//导航菜单地址
private String menuNo200;//导航菜单序号
public Menu200() {//无参构造方法
super();
}
//有参构造方法
public Menu200(int id200, String menuName200, String menuURL200,
String menuNo200) {
super();
this.id200 = id200;
this.menuName200 = menuName200;
this.menuURL200 = menuURL200;
this.menuNo200 = menuNo200;
}
@Override
public String toString() {
return "Menu200 [id200=" + id200 + ", menuName200=" + menuName200
+ ", menuURL200=" + menuURL200 + ", menuNo200=" + menuNo200
+ "]";
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + id200;
result = prime * result
+ ((menuName200 == null) ? 0 : menuName200.hashCode());
result = prime * result
+ ((menuNo200 == null) ? 0 : menuNo200.hashCode());
result = prime * result
+ ((menuURL200 == null) ? 0 : menuURL200.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Menu200 other = (Menu200) obj;
if (id200 != other.id200)
return false;
if (menuName200 == null) {
if (other.menuName200 != null)
return false;
} else if (!menuName200.equals(other.menuName200))
return false;
if (menuNo200 == null) {
if (other.menuNo200 != null)
return false;
} else if (!menuNo200.equals(other.menuNo200))
return false;
if (menuURL200 == null) {
if (other.menuURL200 != null)
return false;
} else if (!menuURL200.equals(other.menuURL200))
return false;
return true;
}
public int getId200() {
return id200;
}
public void setId200(int id200) {
this.id200 = id200;
}
public String getMenuName200() {
return menuName200;
}
public void setMenuName200(String menuName200) {
this.menuName200 = menuName200;
}
public String getMenuURL200() {
return menuURL200;
}
public void setMenuURL200(String menuURL200) {
this.menuURL200 = menuURL200;
}
public String getMenuNo200() {
return menuNo200;
}
public void setMenuNo200(String menuNo200) {
this.menuNo200 = menuNo200;
}
}
```
### **1.2创建公司信息类**
>如何抽象设计,降低难度,只设计关于我们这个模块
创建公司信息类CompanyInfomation200.java
6个属性:编号、标题、图片、内容、编辑时间和序号,要求属性名上也加上学号后三位
重写hashCode()和equals()方法
重写toString()方法
实现可序列化接口
生成有参和无参构造方法
生成getter和setter方法
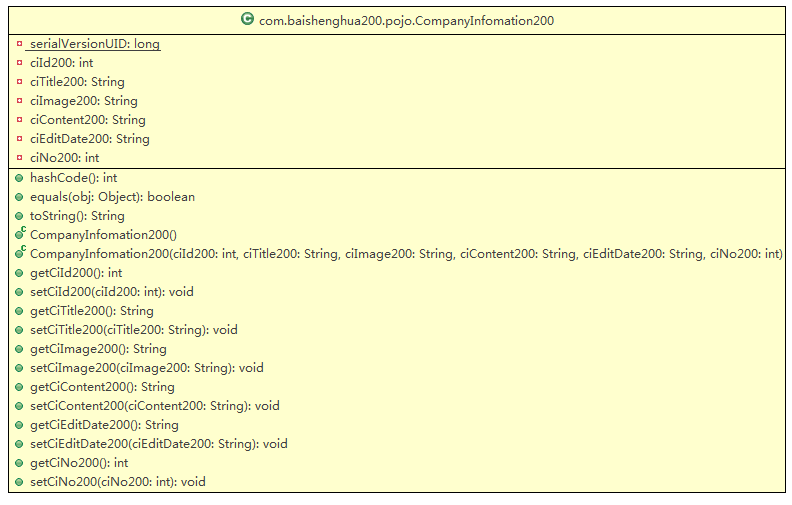
```
package com.baishenghua200.pojo;
import java.io.Serializable;
/**
* CompanyInfomation200.java(公司信息类)
* @desc 导航菜单
* @author 柏圣华
* @date 2022-1-3
*
*/
public class CompanyInfomation200 implements Serializable{
private static final long serialVersionUID = 1L;
private int ciId200;//公司信息编号
private String ciTitle200;//公司信息标题
private String ciImage200;//公司信息图片
private String ciContent200;//公司信息内容
private String ciEditDate200;//公司信息编辑时间
private int ciNo200;//公司信息序号
//生成setter和getter方法
//重写hashCode()、toString()和Equals()方法
//生成有参构造方法和无参构造方法
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result
+ ((ciContent200 == null) ? 0 : ciContent200.hashCode());
result = prime * result
+ ((ciEditDate200 == null) ? 0 : ciEditDate200.hashCode());
result = prime * result + ciId200;
result = prime * result
+ ((ciImage200 == null) ? 0 : ciImage200.hashCode());
result = prime * result + ciNo200;
result = prime * result
+ ((ciTitle200 == null) ? 0 : ciTitle200.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
CompanyInfomation200 other = (CompanyInfomation200) obj;
if (ciContent200 == null) {
if (other.ciContent200 != null)
return false;
} else if (!ciContent200.equals(other.ciContent200))
return false;
if (ciEditDate200 == null) {
if (other.ciEditDate200 != null)
return false;
} else if (!ciEditDate200.equals(other.ciEditDate200))
return false;
if (ciId200 != other.ciId200)
return false;
if (ciImage200 == null) {
if (other.ciImage200 != null)
return false;
} else if (!ciImage200.equals(other.ciImage200))
return false;
if (ciNo200 != other.ciNo200)
return false;
if (ciTitle200 == null) {
if (other.ciTitle200 != null)
return false;
} else if (!ciTitle200.equals(other.ciTitle200))
return false;
return true;
}
@Override
public String toString() {
return "CompanyInfomation200 [ciId200=" + ciId200 + ", ciTitle200="
+ ciTitle200 + ", ciImage200=" + ciImage200 + ", ciContent200="
+ ciContent200 + ", ciEditDate200=" + ciEditDate200
+ ", ciNo200=" + ciNo200 + "]";
}
public CompanyInfomation200() {
super();
}
public CompanyInfomation200(int ciId200, String ciTitle200,
String ciImage200, String ciContent200, String ciEditDate200,
int ciNo200) {
super();
this.ciId200 = ciId200;
this.ciTitle200 = ciTitle200;
this.ciImage200 = ciImage200;
this.ciContent200 = ciContent200;
this.ciEditDate200 = ciEditDate200;
this.ciNo200 = ciNo200;
}
public int getCiId200() {
return ciId200;
}
public void setCiId200(int ciId200) {
this.ciId200 = ciId200;
}
public String getCiTitle200() {
return ciTitle200;
}
public void setCiTitle200(String ciTitle200) {
this.ciTitle200 = ciTitle200;
}
public String getCiImage200() {
return ciImage200;
}
public void setCiImage200(String ciImage200) {
this.ciImage200 = ciImage200;
}
public String getCiContent200() {
return ciContent200;
}
public void setCiContent200(String ciContent200) {
this.ciContent200 = ciContent200;
}
public String getCiEditDate200() {
return ciEditDate200;
}
public void setCiEditDate200(String ciEditDate200) {
this.ciEditDate200 = ciEditDate200;
}
public int getCiNo200() {
return ciNo200;
}
public void setCiNo200(int ciNo200) {
this.ciNo200 = ciNo200;
}
}
```
### **1.3创建用户信息类**
>如何抽象设计,降低难度,只设计关于我们这个模块
创建用户信息类User200.java
6个属性:编号、姓名、性别、年龄、地址、QQ、邮箱、账号和密码 ,要求属性名上也加上学号后三位
重写hashCode()和equals()方法
重写toString()方法
实现可序列化接口
生成有参和无参构造方法
生成getter和setter方法
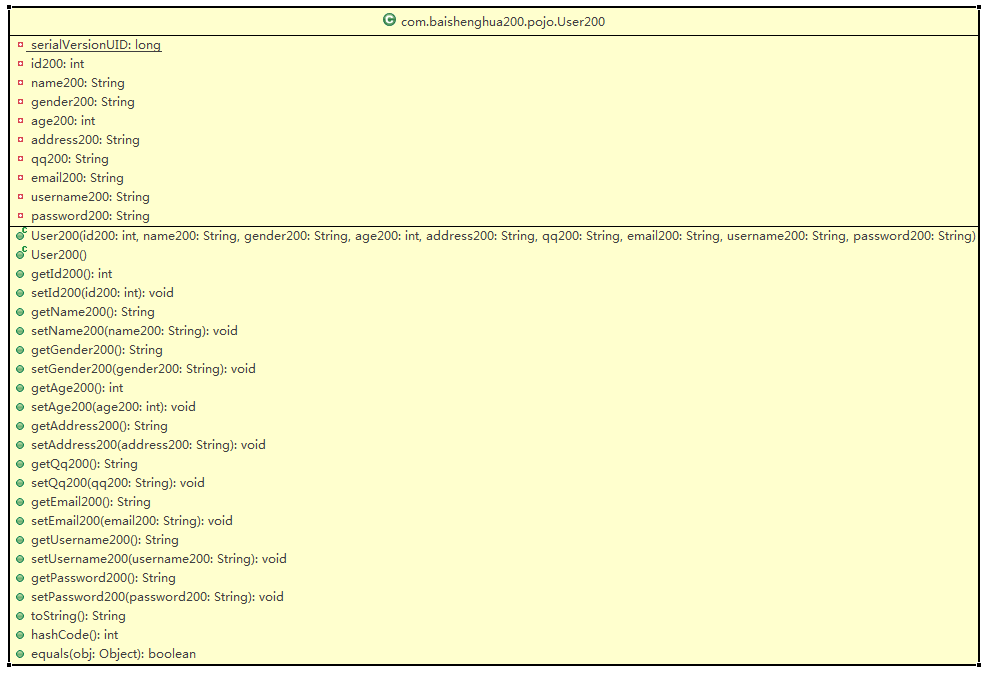
```
package com.baishenghua200.pojo;
import java.io.Serializable;
/**
* User200.java(用户类)
* @desc 描述用户的属性和方法
* @author 柏圣华
* @date 2022-1-3
*
*/
public class User200 implements Serializable{
private static final long serialVersionUID = 1L;
private int id200;
private String name200;
private String gender200;
private int age200;
private String address200;
private String qq200;
private String email200;
private String username200;
private String password200;
//生成setter和getter方法
//重写hashCode()、toString()和Equals()方法
//生成有参构造方法和无参构造方法
//实现可序列化接口
public User200(int id200, String name200, String gender200, int age200,
String address200, String qq200, String email200,
String username200, String password200) {
super();
this.id200 = id200;
this.name200 = name200;
this.gender200 = gender200;
this.age200 = age200;
this.address200 = address200;
this.qq200 = qq200;
this.email200 = email200;
this.username200 = username200;
this.password200 = password200;
}
public User200() {
super();
}
public int getId200() {
return id200;
}
public void setId200(int id200) {
this.id200 = id200;
}
public String getName200() {
return name200;
}
public void setName200(String name200) {
this.name200 = name200;
}
public String getGender200() {
return gender200;
}
public void setGender200(String gender200) {
this.gender200 = gender200;
}
public int getAge200() {
return age200;
}
public void setAge200(int age200) {
this.age200 = age200;
}
public String getAddress200() {
return address200;
}
public void setAddress200(String address200) {
this.address200 = address200;
}
public String getQq200() {
return qq200;
}
public void setQq200(String qq200) {
this.qq200 = qq200;
}
public String getEmail200() {
return email200;
}
public void setEmail200(String email200) {
this.email200 = email200;
}
public String getUsername200() {
return username200;
}
public void setUsername200(String username200) {
this.username200 = username200;
}
public String getPassword200() {
return password200;
}
public void setPassword200(String password200) {
this.password200 = password200;
}
@Override
public String toString() {
return "User200 [id200=" + id200 + ", name200=" + name200
+ ", gender200=" + gender200 + ", age200=" + age200
+ ", address200=" + address200 + ", qq200=" + qq200
+ ", email200=" + email200 + ", username200=" + username200
+ ", password200=" + password200 + "]";
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result
+ ((address200 == null) ? 0 : address200.hashCode());
result = prime * result + age200;
result = prime * result
+ ((email200 == null) ? 0 : email200.hashCode());
result = prime * result
+ ((gender200 == null) ? 0 : gender200.hashCode());
result = prime * result + id200;
result = prime * result + ((name200 == null) ? 0 : name200.hashCode());
result = prime * result
+ ((password200 == null) ? 0 : password200.hashCode());
result = prime * result + ((qq200 == null) ? 0 : qq200.hashCode());
result = prime * result
+ ((username200 == null) ? 0 : username200.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
User200 other = (User200) obj;
if (address200 == null) {
if (other.address200 != null)
return false;
} else if (!address200.equals(other.address200))
return false;
if (age200 != other.age200)
return false;
if (email200 == null) {
if (other.email200 != null)
return false;
} else if (!email200.equals(other.email200))
return false;
if (gender200 == null) {
if (other.gender200 != null)
return false;
} else if (!gender200.equals(other.gender200))
return false;
if (id200 != other.id200)
return false;
if (name200 == null) {
if (other.name200 != null)
return false;
} else if (!name200.equals(other.name200))
return false;
if (password200 == null) {
if (other.password200 != null)
return false;
} else if (!password200.equals(other.password200))
return false;
if (qq200 == null) {
if (other.qq200 != null)
return false;
} else if (!qq200.equals(other.qq200))
return false;
if (username200 == null) {
if (other.username200 != null)
return false;
} else if (!username200.equals(other.username200))
return false;
return true;
}
}
```
### **1.4创建留言信息类**
>如何抽象设计,降低难度,只设计关于我们这个模块
创建留言信息类Message200.java
3个属性:编号、标题和内容,要求属性名上也加上学号后三位
重写hashCode()和equals()方法
重写toString()方法
实现可序列化接口
生成有参和无参构造方法
生成getter和setter方法
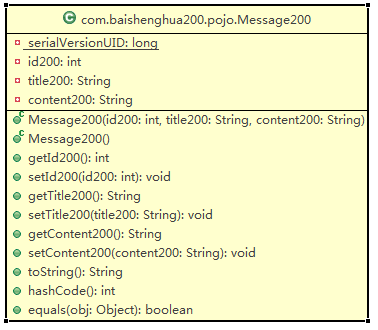
```
package com.baishenghua200.pojo;
import java.io.Serializable;
/**
* Message200.java(留言类)
* @desc 描述留言的属性和方法
* @author 柏圣华
* @date 2022-1-4
*
*/
public class Message200 implements Serializable{
private static final long serialVersionUID = 1L;
private int id200;//留言编号
private String title200;//留言标题
private String content200;//留言内容
public Message200(int id200, String title200, String content200) {
super();
this.id200 = id200;
this.title200 = title200;
this.content200 = content200;
}
public Message200() {
super();
}
public int getId200() {
return id200;
}
public void setId200(int id200) {
this.id200 = id200;
}
public String getTitle200() {
return title200;
}
public void setTitle200(String title200) {
this.title200 = title200;
}
public String getContent200() {
return content200;
}
public void setContent200(String content200) {
this.content200 = content200;
}
@Override
public String toString() {
return "Message200 [id200=" + id200 + ", title200=" + title200
+ ", content200=" + content200 + "]";
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result
+ ((content200 == null) ? 0 : content200.hashCode());
result = prime * result + id200;
result = prime * result
+ ((title200 == null) ? 0 : title200.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Message200 other = (Message200) obj;
if (content200 == null) {
if (other.content200 != null)
return false;
} else if (!content200.equals(other.content200))
return false;
if (id200 != other.id200)
return false;
if (title200 == null) {
if (other.title200 != null)
return false;
} else if (!title200.equals(other.title200))
return false;
return true;
}
}
```
- Java Web项目开发学习手册
- 一、B/S开发环境搭建
- 1.1 tomcat服务器目录结构及作用
- 1.2 在IDE开发工具上配置tomcat服务器
- 1.3 简单web项目在tomcat服务器上运行的方法
- 1.4 开发工具设置
- 1.5 总结
- 二、Servlet技术应用
- 2.1 HttpServlet中的主要方法及应用
- 2.1.1 基于Eclipse完成一个JavaWeb项目
- 2.2 HttpRequest,HttpResponse的应用
- 2.2.1客户端请求
- 2.2.2服务器响应
- 2.2.3Servlet HTTP 状态码
- 2.2.4图片验证码类
- 2.2.5注册模拟实现(带验证码)
- 2.3 ServletConfig对象和ServletContext对象的概念
- 2.4 总结
- 三、JSP技术应用
- 3.1 JSP基本语法
- 3.2 JSP标签和指令
- 3.3 JSP中的隐式对象
- 3.4 常用应用操作
- 3.4.1 JSP客户端请求
- 3.4.2 JSP服务器响应
- 3.4.3 HTTP状态码
- 3.4.4 表单处理
- 3.4.5 过滤器
- 3.4.6 Cookie处理
- 3.4.7 Session处理
- 3.4.8 文件上传
- 3.4.9 日期处理
- 3.4.10 页面重定向
- 3.4.11 点击量统计
- 3.4.12 自动刷新
- 3.4.13 发送邮件
- 3.5 JSP高级应用
- 3.5.1 JSP标准标签库(JSTL)
- 3.5.2 JSP连接数据库
- 3.5.3 JSP XML数据处理
- 3.5.4 JSP JavaBean
- 3.5.5 自定义标签
- 3.5.6 表达式语言
- 3.5.7 异常处理
- 3.5.8 调试
- 3.5.9 JSP国际化
- 3.6 实践代码
- 3.6.1 实践代码
- 3.6.2 项目实战
- 3.7 总结
- 四、MVC思想的理解和搭建MVC
- 4.1 MVC设计模式的思想
- 4.2 MVC设计模式的实现步骤
- 4.3 项目实践
- 4.4 总结
- 五、EL表达式和JSTL技术
- 5.1 EL表达式及其应用
- 5.2 常用的JSTL标签的应用
- 5.3 项目实践
- 5.4 总结
- 六、Cookie和Session
- 6.1 cookie对象的概念和应用
- 6.2 session对象的概念和应用
- 6.3 项目实践
- 6.4 总结
- 七、过滤器技术应用
- 7.1 Filter的概念及应用
- 7.2 Filter、FilterChain、FilterConfig 介绍
- 7.3 用户登录过滤案例
- 7.4 项目实战
- 7.5总结
- 八、异步请求技术
- 8.1 JSON数据格式
- 8.2 使用AJAX实现异步请求
- 8.3 用户名校验案例
- 8.4小结
- 综合项目技术实训
- 1.BS项目开发项目实战
- 2.项目需求分析和系统设计
- 2.1需求分析
- 2.2类型模型设计
- 2.3原型设计
- 3.项目数据库分析和系统设计
- 4.BS项目编程实现
- 4.1搭建框架和命名规约
- 4.2实现步骤
- 4.2.1创建实体类
- 4.2.2创建过滤器类
- 4.2.3创建工具类
- 4.2.4创建DAO接口及其实现类
- 4.2.5创建Service接口及其实现类
- 4.2.6创建测试类
- 4.2.7创建控制器类
- 5.企业开发流程规范
- 6.总结
- 九、练习题及答案
- 企业开发常用技术
- 1.Maven技术
- Java命名规范解读
- 参考资料
- 开发中常用的应用服务器和Web服务器