## 图的表达方式及创建
### 二维数组表示法
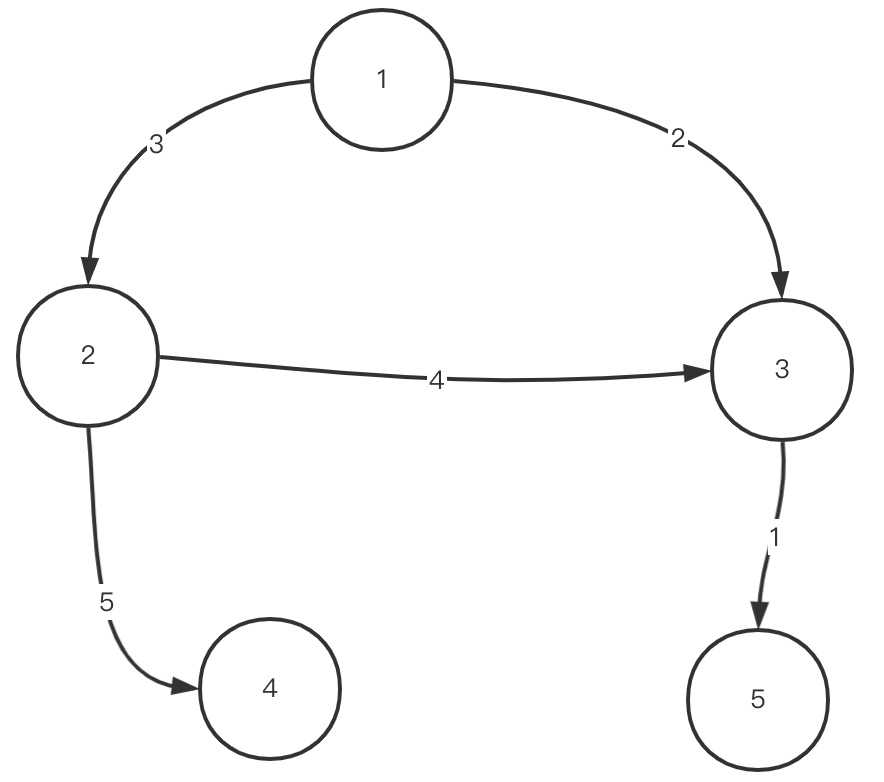
~~~
int[][] data = {
{1,2,3}, // 顶点1到顶点2有一条权值为3的边
{2,3,4},// 顶点2到顶点3有一条权值为4的边
{1,3,2},
{2,4,5},
{3,5,1}
};
~~~
### 按数据创建无向图
~~~
Graph graph = Graph.createGraphUnDirect(data);
~~~
~~~
/**
* 从数组场景创建无向图
* @param data eg,{{1,3,2},{2,3,3}}
* 表示 从顶点1到顶点3有权值为2的边;顶点3到顶点1权值为2的边
* 从顶点2到顶点3有权值为3的边;顶点3到顶点2权值为3的边
* @return
*/
public static Graph createGraphUnDirect(int[][] data){
Graph graph = new Graph();
for(int i=0;i<data.length;i++){
String from = data[i][0] + "";
String to = data[i][1] + "";
Integer weight = data[i][2];
// 将from顶点和to顶点加入到图里
if(!graph.vertexs.containsKey(from)){
graph.vertexs.put(from,new Vertex(from));
}
if(!graph.vertexs.containsKey(to)){
graph.vertexs.put(to,new Vertex(to));
}
// 从图里获取from和to顶点
Vertex fromVertex = graph.vertexs.get(from);
Vertex toVertex = graph.vertexs.get(to);
// 创建从from顶点到to顶点的边
Edge edge = new Edge(weight,fromVertex,toVertex);
// 创建反向的边
Edge reverseEdge = new Edge(weight,toVertex,fromVertex);
// from和to顶点的入度和出度都+1
fromVertex.out++;
fromVertex.in++;
toVertex.out++;
toVertex.in++;
// 将to顶点加入到from顶点里的点集nexts里
fromVertex.nexts.add(toVertex);
// 将from顶点加入到to顶点里的点集nexts里
toVertex.nexts.add(fromVertex);
// 将边加入到from顶点的边集里
fromVertex.edges.add(edge);
// 将反向边加入到to顶点的边集里
toVertex.edges.add(reverseEdge);
// 将边加入到图里
graph.edges.add(edge);
// 将反向边加入到图里
graph.edges.add(reverseEdge);
}
return graph;
}
~~~
### 按数组创建有向图
~~~
Graph graph = Graph.createGraph(data);
~~~
~~~
/**
* 从数组场景创建有向图
* @param data eg,{{1,3,2},{2,3,3}}
* 表示 从顶点1到顶点3有权值为2的边;
* 从顶点2到顶点3有权值为3的边
* @return
*/
public static Graph createGraph(int[][] data){
Graph graph = new Graph();
for(int i=0;i<data.length;i++){
String from = data[i][0] + "";
String to = data[i][1] + "";
Integer weight = data[i][2];
// 将from顶点和to顶点加入到图里
if(!graph.vertexs.containsKey(from)){
graph.vertexs.put(from,new Vertex(from));
}
if(!graph.vertexs.containsKey(to)){
graph.vertexs.put(to,new Vertex(to));
}
// 从图里获取from和to顶点
Vertex fromVertex = graph.vertexs.get(from);
Vertex toVertex = graph.vertexs.get(to);
// 创建从from顶点到to顶点的边
Edge edge = new Edge(weight,fromVertex,toVertex);
// from顶点的出度+1,to顶点的入度+1
fromVertex.out++;
toVertex.in++;
// 将to顶点加入到from顶点里的nexts里
fromVertex.nexts.add(toVertex);
// 将边加入到from顶点的边集里
fromVertex.edges.add(edge);
// 将边加入到图里
graph.edges.add(edge);
}
return graph;
}
~~~
### 字符箭头数组表示法
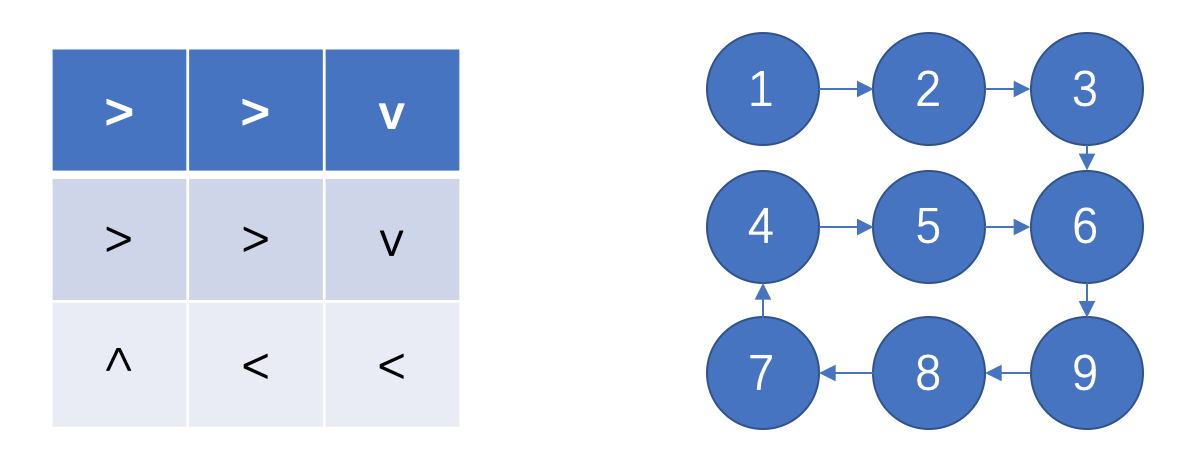
~~~
String[] arrows = {">>v",">>v","^<<"};
~~~
~~~
/**
* 从箭头数组场景创建有向图
* @param arrows eg, 自主编号
* {
* ">>v", 1,2,3
* "v^<", 4,5,6
* "<><" 7,8,9
* }
* 表示 从顶点1到顶点2有边;顶点2到顶点3有边;顶点3到顶点6有边
* 顶点4到顶点7有边;顶点5到顶点2有边;顶点6到顶点5有边
* 顶点8到顶点9有边;顶点9到顶点8有边
* @return
*/
public static Graph createGraphFromArrowString(String[] arrows){
Graph graph = new Graph();
int row = arrows.length;
int col = arrows[0].length();
// 设置上下左右的坐标规则
Map<Character,int[]> ruleMap = new HashMap<>();
ruleMap.put('^',new int[]{-1,0});
ruleMap.put('v',new int[]{1,0});
ruleMap.put('<',new int[]{0,-1});
ruleMap.put('>',new int[]{0,1});
for(int i=0;i<row;i++){
for(int j=0;j<col;j++){
String from = (i * col + j + 1) + "";
int[] rule = ruleMap.get(arrows[i].charAt(j));
int nextI = i + rule[0];
int nextJ = j + rule[1];
String to = (nextI * col + (nextJ + 1)) + "";
// 无权,默认为1
Integer weight = 1;
// 判断to点的坐标在合法范围内
if(nextI >=0 && nextI < row && nextJ >=0 && nextJ < col){
// 将from顶点和to顶点加入到图里
if(!graph.vertexs.containsKey(from)){
graph.vertexs.put(from,new Vertex(from));
}
if(!graph.vertexs.containsKey(to)){
graph.vertexs.put(to,new Vertex(to));
}
// 从图里获取from和to顶点
Vertex fromVertex = graph.vertexs.get(from);
Vertex toVertex = graph.vertexs.get(to);
// 创建从from顶点到to顶点的边
Edge edge = new Edge(weight,fromVertex,toVertex);
fromVertex.out++;
toVertex.in++;
// 将to顶点加入到from顶点里的点集nexts里
fromVertex.nexts.add(toVertex);
// 将边加入到from顶点的边集里
fromVertex.edges.add(edge);
// 将边加入到图里
graph.edges.add(edge);
}
}
}
return graph;
}
~~~
- Redis来回摩擦
- redis的数据结构SDS和DICT
- redis的持久化和事件模型
- Java
- 从何而来之Java IO
- 发布Jar包到公共Maven仓库
- Java本地方法调用
- 面试突击
- Linux
- Nginx
- SpringBoot
- Springboot集成Actuator和SpringbootAdminServer监控
- SpringCloud
- Spring Cloud初识
- Spring Cloud的5大核心组件
- Spring Cloud的注册中心
- Spring Cloud注册中心之Eureka
- Spring Cloud注册中心之Consul
- Spring Cloud注册中心之Nacos
- Spring Cloud的负载均衡之Ribbon
- Spring Cloud的服务调用之Feign
- Spring Cloud的熔断器
- Spring Cloud熔断器之Hystrix
- Spring Cloud的熔断器监控
- Spring Cloud的网关
- Spring Cloud的网关之Zuul
- Spring Cloud的配置中心
- Spring Cloud配置中心之Config Server
- Spring Cloud Config配置刷新
- Spring Cloud的链路跟踪
- Spring Cloud的链路监控之Sleuth
- Spring Cloud的链路监控之Zipkin
- Spring Cloud集成Admin Server
- Docker
- docker日常基本使用
- docker-machine的基本使用
- Kubernetes
- kubernetes初识
- kubeadm安装k8s集群
- minikube安装k8s集群
- k8s的命令行管理工具
- k8s的web管理工具
- k8s的相关发行版
- k3s初识及安装
- rancher的安装及使用
- RaspberryPi
- 运维
- 域名证书更新
- 腾讯云主机组建内网
- IDEA插件开发
- 第一个IDEA插件hello ide开发
- 千呼万唤始出来的IDEA笔记插件mdNote
- 大刚学算法
- 待整理
- 一些概念和知识点
- 位运算
- 数据结构
- 字符串和数组
- LC242-有效的字母异位词
- 链表
- LC25-K个一组翻转链表
- LC83-删除有序单链表重复的元素
- 栈
- LC20-有效的括号
- 队列
- 双端队列
- 优先队列
- 树
- 二叉树
- 二叉树的遍历
- 二叉树的递归序
- 二叉树的前序遍历(递归)
- 二叉树的前序遍历(非递归)
- 二叉树的中序遍历(递归)
- 二叉树的中序遍历(非递归)
- 二叉树的后序遍历(递归)
- 二叉树的后序遍历(非递归)
- 二叉树的广度优先遍历(BFS)
- 平衡二叉树
- 二叉搜索树
- 满二叉树
- 完全二叉树
- 二叉树的打印(二维数组)
- 树的序列化和反序列化
- 前缀树
- 堆
- Java系统堆优先队列
- 集合数组实现堆
- 图
- 图的定义
- 图的存储方式
- 图的Java数据结构(邻接表)
- 图的表达方式及对应场景创建
- 图的遍历
- 图的拓扑排序
- 图的最小生成树之Prim算法
- 图的最小生成树之Kruskal算法
- 图的最小单元路径之Dijkstra算法
- 位图
- Java实现位图
- 并查集
- Java实现并查集
- 滑动窗口
- 单调栈
- 排序
- 冒泡排序BubbleSort
- 选择排序SelectSort
- 插入排序InsertSort
- 插入排序InsertXSort
- 归并排序MergeSort
- 快速排序QuickSort
- 快速排序优化版QuickFastSort
- 堆排序HeapSort
- 哈希Hash
- 哈希函数
- guava中的hash函数
- hutool中的hash函数
- 哈希表实现
- Java之HashMap的实现
- Java之HashSet的实现
- 一致性哈希算法
- 经典问题
- 荷兰国旗问题
- KMP算法
- Manacher算法
- Go