## String to Int Function
The [toInt()](https://www.arduino.cc/en/Reference/StringToInt) function allows you to convert a String to an integer number.
In this example, the board reads a serial input string until it sees a newline, then converts the string to a number if the characters are digits. Once you've uploaded the code to your board, open the Arduino IDE serial monitor, enter some numbers, and press send. The board will repeat these numbers back to you. Observe what happens when a non-numeric character is sent.
### Hardware Required:
* Arduino or Genuino Board
### Circuit
There is no circuit for this example, though your board must be connected to your computer via USB and the serial monitor window of the Arduino Software (IDE) should be open.
[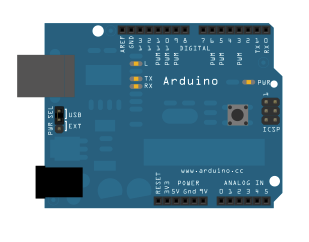](https://www.arduino.cc/en/uploads/Tutorial/Arduino_bb.png)
image developed using [Fritzing](http://www.fritzing.org/). For more circuit examples, see the [Fritzing project page](http://fritzing.org/projects/)
### Code
/*
String to Integer conversion
Reads a serial input string until it sees a newline, then converts the string
to a number if the characters are digits.
The circuit:
- No external components needed.
created 29 Nov 2010
by Tom Igoe
This example code is in the public domain.
http://www.arduino.cc/en/Tutorial/StringToInt
*/
String inString = ""; // string to hold input
void setup() {
// Open serial communications and wait for port to open:
Serial.begin(9600);
while (!Serial) {
; // wait for serial port to connect. Needed for native USB port only
}
// send an intro:
Serial.println("\n\nString toInt():");
Serial.println();
}
void loop() {
// Read serial input:
while (Serial.available() > 0) {
int inChar = Serial.read();
if (isDigit(inChar)) {
// convert the incoming byte to a char and add it to the string:
inString += (char)inChar;
}
// if you get a newline, print the string, then the string's value:
if (inChar == '\n') {
Serial.print("Value:");
Serial.println(inString.toInt());
Serial.print("String: ");
Serial.println(inString);
// clear the string for new input:
inString = "";
}
}
}
[[Get Code]](https://www.arduino.cc/en/Tutorial/StringToInt?action=sourceblock&num=1)
### See Also
* [String object](https://www.arduino.cc/en/Reference/StringObject) – Your Reference for String objects
* [CharacterAnalysis](https://www.arduino.cc/en/Tutorial/CharacterAnalysis) - We use the operators that allow us to recognise the type of character we are dealing with.
* [StringAdditionOperator](https://www.arduino.cc/en/Tutorial/StringAdditionOperator) - Add strings together in a variety of ways.
* [StringAppendOperator](https://www.arduino.cc/en/Tutorial/StringAppendOperator) - Use the += operator and the concat() method to append things to Strings
* [StringCaseChanges](https://www.arduino.cc/en/Tutorial/StringCaseChanges) - Change the case of a string.
* [StringCharacters](https://www.arduino.cc/en/Tutorial/StringCharacters) - Get/set the value of a specific character in a string.
* [StringComparisonOperators](https://www.arduino.cc/en/Tutorial/StringComparisonOperators) - Get/set the value of a specific character in a string.
* [StringConstructors](https://www.arduino.cc/en/Tutorial/StringConstructors) - Initialize string objects.
* [StringIndexOf](https://www.arduino.cc/en/Tutorial/StringIndexOf) - Look for the first/last instance of a character in a string.
* [StringLength](https://www.arduino.cc/en/Tutorial/StringLength) - Get the length of a string.
* [StringLengthTrim](https://www.arduino.cc/en/Tutorial/StringLengthTrim) - Get and trim the length of a string.
* [StringReplace](https://www.arduino.cc/en/Tutorial/StringReplace) - Replace individual characters in a string.
* [StringStartsWithEndsWith](https://www.arduino.cc/en/Tutorial/StringStartsWithEndsWith) - Check which characters/substrings a given string starts or ends with.
* [StringSubstring](https://www.arduino.cc/en/Tutorial/StringSubstring) - Look for "phrases" within a given string.
- 说明
- 系统示例文件目录结构及说明
- 01.Basics
- AnalogReadSerial
- BareMinimum
- Blink
- DigitalReadSerial
- Fade
- ReadAnalogVoltage
- 02.Digital
- BlinkWithoutDelay
- Button
- Debounce
- DigitalInputPullup
- StateChangeDetection
- toneKeyboard
- toneMelody
- toneMultiple
- tonePitchFollower
- 03.Analog
- AnalogInOutSerial
- AnalogInput
- AnalogWriteMega
- Calibration
- Fading
- Smoothing
- 04.Communication
- ASCIITable
- Dimmer
- Graph
- Midi
- MultiSerial
- PhysicalPixel
- ReadASCIIString
- SerialCallResponse
- SerialCallResponseASCII
- SerialEvent
- SerialPassthrough
- VirtualColorMixer
- 05.Control
- Arrays
- ForLoopIteration
- IfStatementConditional
- switchCase
- switchCase2
- WhileStatementConditional
- 06.Sensors
- ADXL3xx
- Knock
- Memsic2125
- Ping
- 07.Display
- barGraph
- RowColumnScanning
- 08.Strings
- CharacterAnalysis
- StringAdditionOperator
- StringAppendOperator
- StringCaseChanges
- StringCharacters
- StringComparisonOperators
- StringConstructors
- StringIndexOf
- StringLength
- StringLengthTrim
- StringReplace
- StringStartsWithEndsWith
- StringSubstring
- StringToInt
- 09.USB
- Keyboard
- KeyboardLogout
- KeyboardMessage
- KeyboardReprogram
- KeyboardSerial
- KeyboardAndMouseControl
- Mouse
- ButtonMouseControl
- JoystickMouseControl
- 10.StarterKit_BasicKit (与特定硬件相关,暂无)
- p02_SpaceshipInterface
- p03_LoveOMeter
- p04_ColorMixingLamp
- p05_ServoMoodIndicator
- p06_LightTheremin
- p07_Keyboard
- p08_DigitalHourglass
- p09_MotorizedPinwheel
- p10_Zoetrope
- p11_CrystalBall
- p12_KnockLock
- p13_TouchSensorLamp
- p14_TweakTheArduinoLogo
- p15_HackingButtons
- 11.ArduinoISP(暂无)
- ArduinoISP