## 按钮状态检测
> 当你使用按钮时,有时需要知道按钮被按下了多少次,为了这个目的,你需要知道当按钮按下时,状态的改变,并且能够记录统计次数,这就是状态检测或边缘检测。
> 在这个示例中,我们要学会如何检测这种改变,并且把信息发送到串口监视器,并且改变led的亮暗。
### 所需硬件
* Arduino
* 按键
* 10k 电阻
* 导线
* 面包板
### 电路

按钮的一端通过10K下拉电阻接地,并且连接到数字引脚I/O口2,来读取按钮的状态,另外一端连接到5V
当按钮没有被按下的时候,两个引脚之间是断开的,这时候我们的数字引脚通过下拉电阻接地,读出数值LOW,
当按钮按下时,按钮的两个引脚被连接上了,引脚连接到了电压,所以我们读出HIGH,(因为电路中电阻的阻碍作用,所以这个引脚是连接到了+5V)
### 原理图
[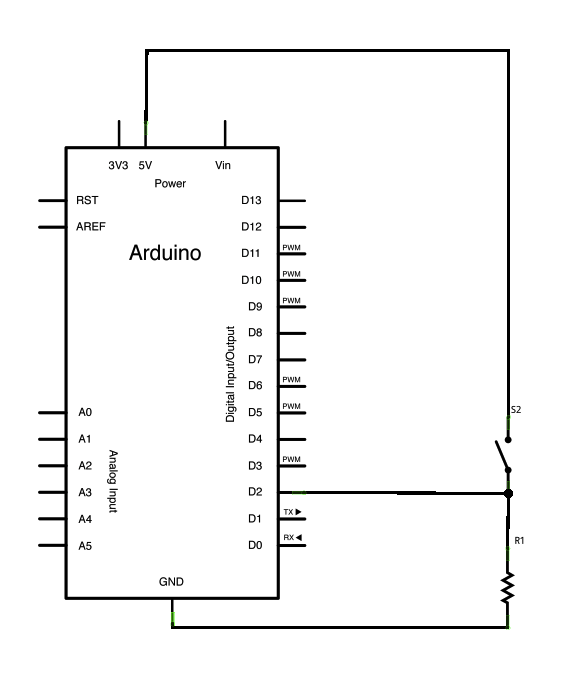](https://www.arduino.cc/en/uploads/Tutorial/button_sch.png)
### 代码
这个程序不断的读取按钮的状态,并且比较当前和以前的按钮状态,如果状态发生了改变,那么按钮被按下,如果按钮按下的次数是四的倍数的时候,改变13脚led的亮灭
/*
State change detection (edge detection)
Often, you don't need to know the state of a digital input all the time, but
you just need to know when the input changes from one state to another.
For example, you want to know when a button goes from OFF to ON. This is called
state change detection, or edge detection.
This example shows how to detect when a button or button changes from off to on
and on to off.
*/
// this constant won't change:
const int buttonPin = 2; // the pin that the pushbutton is attached to
const int ledPin = 13; // the pin that the LED is attached to
// 定义变量:
int buttonPushCounter = 0; // 按钮按下次数计数器,
int buttonState = 0; // 当前按钮的状态,
int lastButtonState = 0; // 上一次按钮的状态,
void setup() {
//初始化按钮作为输入,
pinMode(buttonPin, INPUT);
//初始化led作为输出,:
pinMode(ledPin, OUTPUT);
// 初始化串口,:
Serial.begin(9600);
}
void loop() {
// 读取按钮输入引脚,
buttonState = digitalRead(buttonPin);
// 比较按钮的当前状态和以前的状态,
if (buttonState != lastButtonState) {
// 如果按钮状态发生了改变,增加计数
if (buttonState == HIGH) {
// if the current state is HIGH then the button went from off to on:
buttonPushCounter++;
Serial.println("on");
Serial.print("number of button pushes: ");
Serial.println(buttonPushCounter);
} else {
// if the current state is LOW then the button went from on to off:
Serial.println("off");
}
// Delay a little bit to avoid bouncing
delay(50);
}
// 保存当前的状态,作为下一个循环使用,
lastButtonState = buttonState;
// 如果按钮按下的次数是四的倍数,点亮led,
if (buttonPushCounter % 4 == 0) {
digitalWrite(ledPin, HIGH);
} else {
digitalWrite(ledPin, LOW);
}
}
### 相关资料:
* [pinMode](https://www.arduino.cc/en/Reference/PinMode)()
* [digitalWrite](https://www.arduino.cc/en/Reference/DigitalWrite)()
* [digitalRead](https://www.arduino.cc/en/Reference/DigitalRead)()
* [millis](https://www.arduino.cc/en/Reference/Millis)()
* [if](https://www.arduino.cc/en/Reference/If)
- 说明
- 系统示例文件目录结构及说明
- 01.Basics
- AnalogReadSerial
- BareMinimum
- Blink
- DigitalReadSerial
- Fade
- ReadAnalogVoltage
- 02.Digital
- BlinkWithoutDelay
- Button
- Debounce
- DigitalInputPullup
- StateChangeDetection
- toneKeyboard
- toneMelody
- toneMultiple
- tonePitchFollower
- 03.Analog
- AnalogInOutSerial
- AnalogInput
- AnalogWriteMega
- Calibration
- Fading
- Smoothing
- 04.Communication
- ASCIITable
- Dimmer
- Graph
- Midi
- MultiSerial
- PhysicalPixel
- ReadASCIIString
- SerialCallResponse
- SerialCallResponseASCII
- SerialEvent
- SerialPassthrough
- VirtualColorMixer
- 05.Control
- Arrays
- ForLoopIteration
- IfStatementConditional
- switchCase
- switchCase2
- WhileStatementConditional
- 06.Sensors
- ADXL3xx
- Knock
- Memsic2125
- Ping
- 07.Display
- barGraph
- RowColumnScanning
- 08.Strings
- CharacterAnalysis
- StringAdditionOperator
- StringAppendOperator
- StringCaseChanges
- StringCharacters
- StringComparisonOperators
- StringConstructors
- StringIndexOf
- StringLength
- StringLengthTrim
- StringReplace
- StringStartsWithEndsWith
- StringSubstring
- StringToInt
- 09.USB
- Keyboard
- KeyboardLogout
- KeyboardMessage
- KeyboardReprogram
- KeyboardSerial
- KeyboardAndMouseControl
- Mouse
- ButtonMouseControl
- JoystickMouseControl
- 10.StarterKit_BasicKit (与特定硬件相关,暂无)
- p02_SpaceshipInterface
- p03_LoveOMeter
- p04_ColorMixingLamp
- p05_ServoMoodIndicator
- p06_LightTheremin
- p07_Keyboard
- p08_DigitalHourglass
- p09_MotorizedPinwheel
- p10_Zoetrope
- p11_CrystalBall
- p12_KnockLock
- p13_TouchSensorLamp
- p14_TweakTheArduinoLogo
- p15_HackingButtons
- 11.ArduinoISP(暂无)
- ArduinoISP