## A到Z个python小技巧
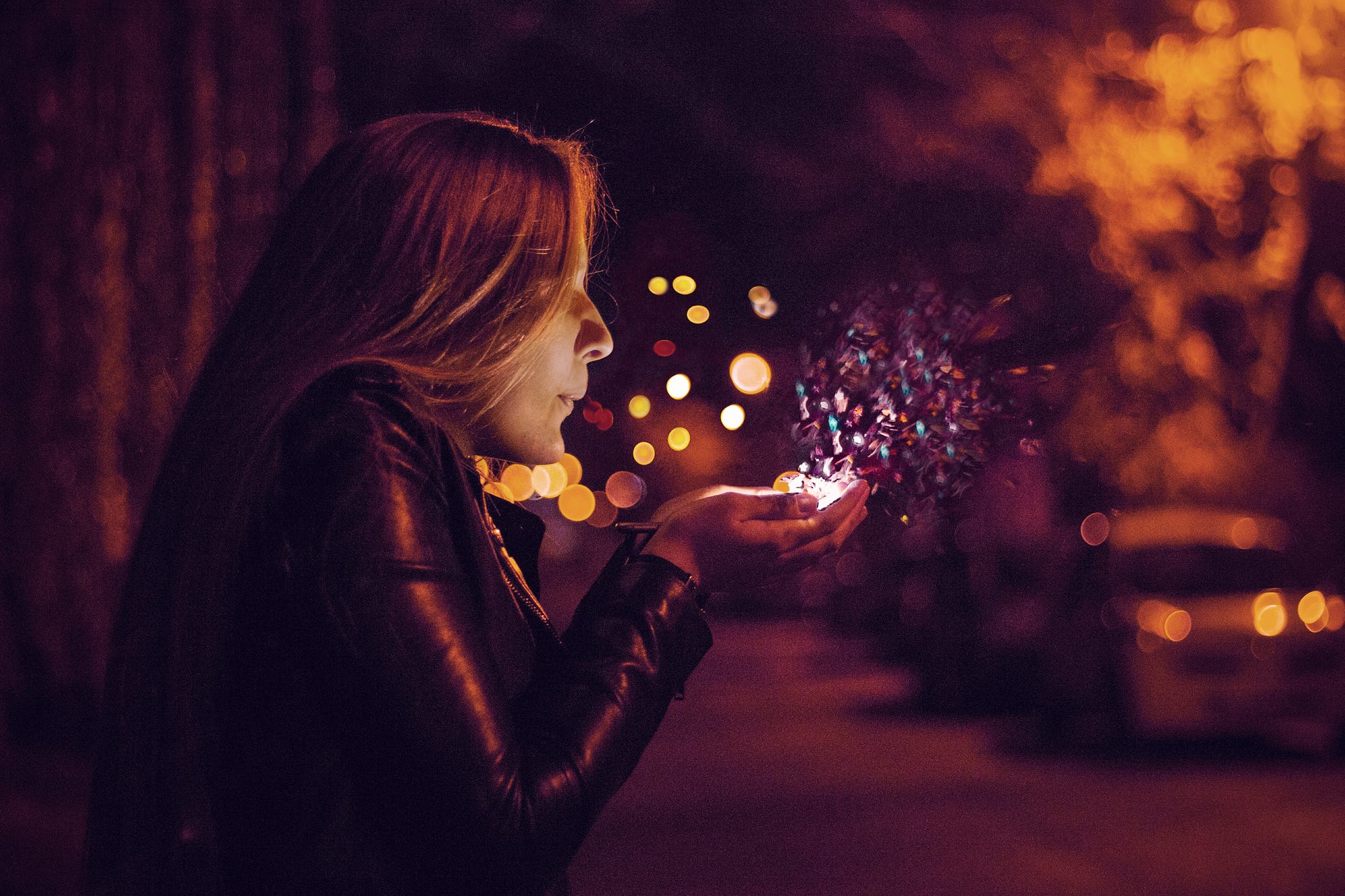
Python是世界上最流行的编程语言之一,这有如下原因:
* python很容易学习
* python有丰富的使用场景
* python有大量的模块和库作为支持
在日常工作中,我使用python完成数据科学的工作,在工作中,我总结了一些有用的技巧。
我尝试把这些技巧在字母a-z进行了分类。下面是具体的小技巧
## all or any
python之所以如此流行的原因是,它的可读性非常高。而且有很多的语法糖,看下面的例子:
~~~
x = [True, True, False]
if any(x):
print("At least one True")
if all(x):
print("Not one False")
if any(x) and not all(x):
print("At least one True and one False")
~~~
## bashplotlib
你想在控制台绘制图表?
`pip install bashplotlib`
bashplotlib是在控制台画图表的库。
## collections
python有很好的默认集合类,有时候我们需要一些增强的python类来完成对数据的处理,看下面列子:
~~~
from collections import OrderedDict, Counter
# Remembers the order the keys are added!
x = OrderedDict(a=1, b=2, c=3)
# Counts the frequency of each character
y = Counter("Hello World!")
~~~
## dir
你想知道python对象内部有什么东西吗?dir方法可以帮助你查看,看代码:
~~~
dir()
dir("Hello World")
dir(dir)
~~~
这在你使用一个新的包时会非常有用。
## emoji
python的emoji表情表:
`$ pip install emoji`
看代码:
~~~
from emoji import emojize
print(emojize(":thumbs_up:"))
~~~
👍
## from __future__ import
想提前尝试python的新特性,可以使用 __future__语法。
~~~
from __future__ import print_function
print("Hello World!")
~~~
## geopy
python的地理位置包
`$ pip install geopy`
使用这个包可以完成地址和经纬度的转换和距离检测等操作。看代码:
~~~
from geopy import GoogleV3
place = "221b Baker Street, London"
location = GoogleV3().geocode(place)
print(location.address)
print(location.location)
~~~
## howdoi
不知道一些事情该怎么做?用`howdoi `这个命令吧
`$ pip install howdoi`
看使用例子:
~~~
$ howdoi vertical align css
$ howdoi for loop in java
$ howdoi undo commits in git
~~~
## inspect
想知道别人包的代码是是什么?使用inspect模块吧。看代码:
~~~
import inspect
print(inspect.getsource(inspect.getsource))
print(inspect.getmodule(inspect.getmodule))
print(inspect.currentframe().f_lineno)
~~~
## Jedi
python的代码补全器,如果没有用IDE编写python代码,可以尝试一下这个
## **kwargs
python的参数语法糖,看代码:
~~~
dictionary = {"a": 1, "b": 2}
def someFunction(a, b):
print(a + b)
return
# these do the same thing:
someFunction(**dictionary)
someFunction(a=1, b=2)
~~~
## List comprehensions
集合操作的语法糖,是不是很绕 哈哈
~~~
numbers = [1,2,3,4,5,6,7]
evens = [x for x in numbers if x % 2 is 0]
odds = [y for y in numbers if y not in evens]
cities = ['London', 'Dublin', 'Oslo']
def visit(city):
print("Welcome to "+city)
for city in cities:
visit(city)
~~~
## map
函数式编程语法糖,不过现在很少用了
~~~
x = [1, 2, 3]
y = map(lambda x : x + 1 , x)
# prints out [2,3,4]
print(list(y))
~~~
## newspaper3k
用python读新闻,这个太极客了
~~~
$ pip install newspaper3k
~~~
## Operator overloading
操作符重载是python的一个重要特性。在定义一个类时,可以用操作符重载来对两个对象做简单判断。看代码:
~~~
class Thing:
def __init__(self, value):
self.__value = value
def __gt__(self, other):
return self.__value > other.__value
def __lt__(self, other):
return self.__value < other.__value
something = Thing(100)
nothing = Thing(0)
# True
something > nothing
# False
something < nothing
# Error
something + nothing
~~~
## pprint
`print`方法可以做简单输出,如果要输出复杂对象,可以使用`pprint`
~~~
import requests
import pprint
url = 'https://randomuser.me/api/?results=1'
users = requests.get(url).json()
pprint.pprint(users)
~~~
## Queue
python的队列实现
#### \_\_repr\_\_
`__repr__`方法将一个对象用字符串表示。在定一个一个类时,可以重写`__repr__`方法
~~~
class someClass:
def __repr__(self):
return "<some description here>"
someInstance = someClass()
# prints <some description here>
print(someInstance)
~~~
## sh
用python执行shell命令
~~~
from sh import *
sh.pwd()
sh.mkdir('new_folder')
sh.touch('new_file.txt')
sh.whoami()
sh.echo('This is great!')
~~~
## Type hints
python是一个弱类型语言,为了安全,还可以对方法参数声明类型。
~~~
def addTwo(x : Int) -> Int:
return x + 2
~~~
## uuid
生成uuid
~~~
import uuid
user_id = uuid.uuid4()
print(user_id)
~~~
## Virtual environments
为自己的项目虚拟一个独立的python环境,防止模块污染
~~~
python -m venv my-project
source my-project/bin/activate
pip install all-the-modules
~~~
## wikipedia
python访问维基百科
~~~
import wikipedia
result = wikipedia.page('freeCodeCamp')
print(result.summary)
for link in result.links:
print(link)
~~~
## xkcd
Humour is a key feature of the Python language — after all, it is named after the British comedy sketch show [Monty Python’s Flying Circus](https://en.wikipedia.org/wiki/Monty_Python%27s_Flying_Circus). Much of Python’s official documentation references the show’s most famous sketches.
The sense of humour isn’t restricted to the docs, though. Have a go running the line below:
import antigravity
Never change, Python. Never change.
## YAML
ymal数据的python客户端
`$ pip install pyyaml`
`import yaml`
PyYAML lets you store Python objects of any datatype, and instances of any user-defined classes also.
## zip
将两个集合变为一个字典
~~~
keys = ['a', 'b', 'c']
vals = [1, 2, 3]
zipped = dict(zip(keys, vals))
~~~
python是一个非常灵活的语言。里面还有很多内置的语法糖,一方面方便了我们的编码,另外一方面也会造成一些困惑。有奇怪的语法记得多谷歌。
**阿达老师-孩子身边的编程专家**
*完整课程请关注阿达老师,主页里有完整的课程目录和观看地址*
- 空白目录
- 8.21 做自媒体我学到了什么
- scratch技巧分享系列-调试技巧
- 8.23 论scratch的缺陷
- 9.4 孩子为什么要学编程
- 9.4 好榜样
- 9.12 python a-z
- 开发网页很难吗?
- 9.14 用python识别微表情
- 9.14 todo,给孩子搭建一个自己的网站吧
- 9.16 scratch模拟台风
- 9.17 python好文分享-列表详解
- 9.17 台风怎么形成的,阿达老师做给你
- 9.18 阿达老师科学课-什么是生物
- 9.18 进位加法怎么做?阿达老师用Scratch教给你
- 9.19 树叶为什么会变黄?和阿达老师一起看下
- 9.19 用Scratch做100以内的减法
- 9.19 小草和山羊的斗智斗勇
- 9.19习大大主持开幕的人工智能大会讲了啥
- 9.24 中秋节的月亮为什么那么圆
- 9.27 编程还可以写歌?你没看错
- 10.10
- 10.11 用编程玩物理-什么是引力
- 10.16 jupyter使用
- 10.17 什么是火
- 1024 长度换算
- 你会叠飞机吗
- 和孩子们一起做绘本-沙漠版小红帽
- 一分钟学编程系列-下雪啦
- 一分钟学编程系列-光合作用
- 一分钟学编程系列-挂满礼物的圣诞树
- 一分钟学编程系列-太阳系里的星球(一)
- 一分钟学编程系列-太阳系里的星球(二)
- 为什么学生不喜欢上学(二)-事实性知识的重要性
- 为什么学生不喜欢上学(三)-为什么学生能记住电视里的所有细节, 却记不住我们告诉他的任何知识?
- 为什么学生不喜欢上学(四)- 抽象概念为什么这么难
- 一分钟学编程计划-圣诞节的礼物派对
- 一分钟学编程系列-火星营救(一)
- 为什么孩子不喜欢上学(五)- 题海战术有用吗
- 为什么孩子不喜欢上学(六)- 思考的秘诀是什么