[TOC]
## **一、运算符及优先级**
Python 运算符(算术运算、比较运算、赋值运算、逻辑运算、成员运算)
**1、算数运算符**
| 运算符 | 描述 | 实例,a=20,b=10 |
| --- | --- |---|
| + | 加 | a+b输出结果30 |
| - | 减 | a-b输出结果10 |
| * | 乘 | a*b 输出结果200 |
| / | 除 | a/b输出结果2 |
| % | 取模 | a%b输出结果0 |
| ** | 取幂 | a**b输出结果20的10次方 |
| // | 取整除 | 9/2输出结果4,9.0/2.0输出结果4.0 |
**2.比较运算符**
| 运算符 | 描述 | 实例 |
| --- | --- |--- |
| == | 等于 | (a==b)返回False |
| != | 不等于 | (a!=b)返回True |
| <> | 不等于 | (a<>b)返回True |
| > | 大于 | (a>b)返回True |
| >= | 大于等于 | (a>=b)返回True |
| < | 小于 | (a<b)返回False |
| <= | 小于等于 | (a<=b)返回False |
**3.赋值运算符**
| 运算符 | 描述 | 实例 |
| --- | --- |--- |
| = | 简单的赋值运算符 | c=a+b,将a+b的运算结果赋值给c(30) |
| += | 加法赋值运算符 | c+=a 等效于c=c+a |
| -= | 减法赋值运算符 | c-=a 等效于c=c-a |
| *= | 乘法赋值运算符 | c*=a 等效于c=c*a |
| /= | 除法赋值运算符 | c/=a 等效于c=c/a |
| %= | 取模赋值运算符 | c%=a 等效于c=c%a |
| **= | 取幂赋值运算符 | c**=a 等效于c=c**a |
| //= | 取整除赋值运算符 | c//=a 等效于c=c//a |
**4.逻辑运算符**
| 运算符 | 描述 | 实例 |
| --- | --- |--- |
| and | 布尔“与” | (a and b)返回True |
| or | 布尔“或” | (a or b)返回True |
| not | 布尔“非” | not(a and b)返回False |
**5.成员运算符**
| 成员运算符 | 描述 | 实例 |
| --- | --- | --- |
| in | 如果再指定的序列中找到值,返回True,否则返回False | x在y序列中,如果x在y序列中返回True |
| not in | 如果再指定的序列中找不到值,返回True,否则返回False | x在y序列中,如果x在y序列中返回False |
```
a="abcdefg"
b="b"
if b in a :
print("b元素在a字符串中")
else:
print("b元素不在a字符串")
```
**6.位运算符**
| 运算符 | 描述 | 实例 a=00001010 b=00010100 |
| --- | --- | --- |
| & | 按位与运算符 | (a&b)输出结果0 |
| I | 按位或运算符 | (a|b)输出结果30 |
| ^ | 按位异或运算符 | (a^b)输出结果30 |
| ~ | 按位取反运算符 | ~10输出结果-11 |
| << | 左移运算符 | a<<2输出结果40 |
| >> | 右移运算符 | a>>2输出结果2 |
**7.身份运算符**
| 运算符 | 描述 | 实例 |
| --- | --- |--- |
| is | is是判断两个标识符是不是引用自一个对象 | x is y,如果id(x)等于id(y),is返回结果1 |
| is not | is not是判断两个标识符是不是引用不同对象 | x is not y,如果id(x)不等于id(y),is not返回1 |
**8.三目运算符**
三目运算符可以简化条件语句的缩写,可以使代码看起来更加简洁,三目可以简单的理解为有三个变量,它的形式是这样的 name= k1 if 条件 else k2 ,如果条件成立,则 name=k1,否则name=k2,下面从代码里面来加深一下理解,从下面的代码明显可以看出三目运算符可以使代码更加简洁。
```
a=1
b=2
if a<b: #一般条件语句的写法
k=a
else:
k=b
k=a if a<b else b #三目运算符的写法
```
**9.运算符优先级**
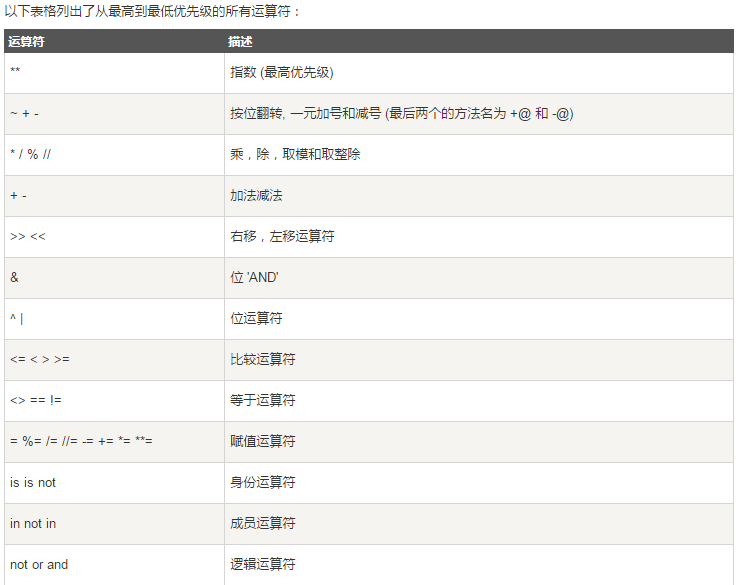
<br />
## **二、数据类型及常用操作(数字, bool, str, list, tuple, dict, set)**
数据类型:数字、字符串、布尔值、字符串、列表、元组、字典、集合
序列:字符串、列表、元组
散列:集合、字典
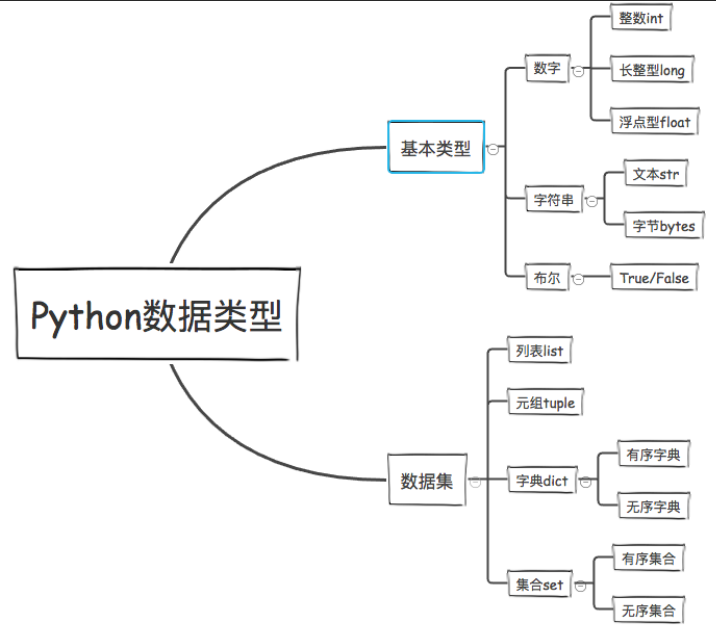
查看不同数据类型的方法,dir(),列如:dir(list)
\>>> dir(list) \['\_\_add\_\_', '\_\_class\_\_', '\_\_contains\_\_', '\_\_delattr\_\_', '\_\_delitem\_\_', '\_\_delslice\_\_', '\_\_doc\_\_', '\_\_eq\_\_', '\_\_format\_\_', '\_\_ge\_\_', '\_\_getattribute\_\_', '\_\_getitem\_\_', '\_\_getslice\_\_', '\_\_gt\_\_', '\_\_hash\_\_', '\_\_iadd\_\_', '\_\_imul\_\_', '\_\_init\_\_', '\_\_iter\_\_', '\_\_le\_\_', '\_\_len\_\_', '\_\_lt\_\_', '\_\_mul\_\_', '\_\_ne\_\_', '\_\_new\_\_', '\_\_reduce\_\_', '\_\_reduce\_ex\_\_', '\_\_repr\_\_', '\_\_reversed\_\_', '\_\_rmul\_\_', '\_\_setattr\_\_', '\_\_setitem\_\_', '\_\_setslice\_\_', '\_\_sizeof\_\_', '\_\_str\_\_', '\_\_subclasshook\_\_', 'append', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort'\]
**1、数字**
**int**
Python可以处理任意大小的正负整数,但是实际中跟我们计算机的内存有关,
在32位机器上,整数的位数为32位,取值范围为-2\*\*31~2\*\*31-1,即-2147483648~2147483647
在64位系统上,整数的位数为64位,取值范围为-2\*\*63~2\*\*63-1,即-9223372036854775808~9223372036854775807。
**long**
跟C语言不同,Python的长整数没有指定位宽,即:Python没有限制长整数数值的大小,但实际上由于机器内存有限,我们使用的长整数数值不可能无限大。
注意,自从Python2.2起,如果整数发生溢出,Python会自动将整数数据转换为长整数,所以如今在长整数数据后面不加字母L也不会导致严重后果了。
注意:在Python3里不再有long类型了,全都是int。
py2中

py3中
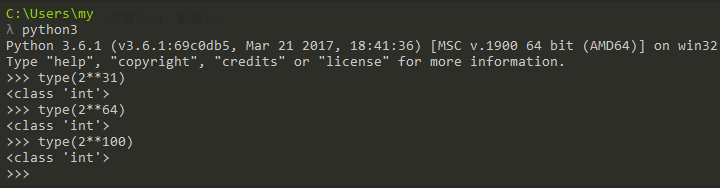
**float**
浮点数也就是小数,之所以称为浮点数,是因为按照科学记数法表示时,一个浮点数的小数点位置是可变的,比如,1.23x109和12.3x108是相等的。浮点数可以用数学写法,如1.23,3.14,-9.01,等等。但是对于很大或很小的浮点数,就必须用科学计数法表示,把10用e替代,1.23x109就是1.23e9,或者12.3e8,0.000012可以写成1.2e-5,等等。
**2、布尔值(bool)**
布尔类型很简单,就两个值 ,一个True(真),一个False(假),,主要用记逻辑判断
也可以将bool值归类为数字,是因为我们也习惯用1表示True,0表示False
```
class bool([x])
Return a Boolean value, i.e. one of True or False. x is converted using the standard truth testing procedure. If x is false or omitted, this returns False; otherwise it returns True. The bool class is a subclass of int (see Numeric Types — int, float, complex). It cannot be subclassed further. Its only instances are False and True (see Boolean Values).
```
```
# 参数如果缺省,则返回False
print(bool()) # False
# 传入布尔类型时,按原值返回
print(bool(True)) # True
print(bool(False)) # False
# 传入字符串时,空字符串返回False,否则返回True
print(bool('')) # False
print(bool('0')) # True
# 传入数值时,0值返回False,否则返回True
print(bool(0)) # False
print(bool(0,)) # False
print(bool(1)) # True
# 传入元组、列表、字典等对象时,元素个数为空返回False,否则返回True
print(bool(())) # False
print(bool((0,))) # True
print(bool([])) # False
print(bool([0])) # True
print(bool({})) # False
print(bool({'name':'jack'})) # True
```
- Linux
- Linux 文件权限概念
- 重点总结
- Linux 文件与目录管理
- 2.1 文件与目录管理
- 2.2 文件内容查阅
- 文件与文件系统的压缩,打包与备份
- 3.1 Linux 系统常见的压缩指令
- 3.2 打包指令: tar
- vi/vim 程序编辑器
- 4.1 vi 的使用
- 4.2 vim编辑器删除一行或者多行内容
- 进程管理
- 5.1 常用命令使用技巧
- 5.2 进程管理
- 系统服务 (daemons)
- 6.1 通过 systemctl 管理服务
- Linux 系统目录结构
- Linux yum命令
- linux系统查看、修改、更新系统时间(自动同步网络时间)
- top linux下的任务管理器
- Linux基本配置
- CentOS7开启防火墙
- CentOS 使用yum安装 pip
- strace 命令
- Linux下设置固定IP地址
- 查看Linux磁盘及内存占用情况
- Mysql
- 关系数据库概述
- 数据库技术
- 数据库基础语句
- 查询语句(--重点--)
- 约束
- 嵌套查询(子查询)
- 表emp
- MySQL数据库练习
- 01.MySQL数据库练习数据
- 02.MySQL数据库练习题目
- 03.MySQL数据库练习-答案
- Mysql远程连接数据库
- Python
- python基础
- Python3中字符串、列表、数组的转换方法
- python字符串
- python安装、pip基本用法、变量、输入输出、流程控制、循环
- 运算符及优先级、数据类型及常用操作、深浅拷贝
- 虚拟环境(virtualenv)
- 网络编程
- TCP/IP简介
- TCP编程
- UDP编程
- 进程和线程
- 访问数据库
- 使用SQLite
- 使用MySQL
- Web开发
- HTML简介
- Python之日志处理(logging模块)
- 函数式编程
- 高阶函数
- python报错解决
- 启动Python时报“ImportError: No module named site”错误
- python实例
- 01- 用python解决数学题
- 02- 冒泡排序
- 03- 邮件发送(smtplib)
- Django
- 01 Web应用
- Django3.2 教程
- Django简介
- Django环境安装
- 第一个Django应用
- Part 1:请求与响应
- Part 2:模型与后台
- Part 3:视图和模板
- Part 4:表单和类视图
- Part 5:测试
- Part 6:静态文件
- Part 7:自定义admin
- 第一章:模型层
- 实战一:基于Django3.2可重用登录与注册系统
- 1. 搭建项目环境
- 2. 设计数据模型
- 3. admin后台
- 4. url路由和视图
- 5. 前端页面设计
- 6. 登录视图
- 7. Django表单
- 8. 图片验证码
- 9. session会话
- 10. 注册视图
- 实战二:Django3.2之CMDB资产管理系统
- 1.项目需求分析
- 2.模型设计
- 3.数据收集客户端
- 4.收集Windows数据
- 5.Linux下收集数据
- 6.新资产待审批区
- 7.审批新资产
- django 快速搭建blog
- imooc-Django全栈项目开发实战
- redis
- 1.1 Redis简介
- 1.2 安装
- 1.3 配置
- 1.4 服务端和客户端命令
- 1.5 Redis命令
- 1.5.1 Redis命令
- 1.5.2 键(Key)
- 1.5.3 字符串(string)
- 1.5.4 哈希(Hash)
- 1.5.5 列表(list)
- 1.5.6 集合(set)
- 1.5.7 有序集合(sorted set)
- Windows
- Win10安装Ubuntu子系统
- win10远程桌面身份验证错误,要求的函数不受支持
- hm软件测试
- 02 linux基本命令
- Linux终端命令格式
- Linux基本命令(一)
- Linux基本命令(二)
- 02 数据库
- 数据库简介
- 基本概念
- Navicat使用
- SQL语言
- 高级
- 03 深入了解软件测试
- day01
- 04 python基础
- 语言基础
- 程序中的变量
- 程序的输出
- 程序中的运算符
- 数据类型基础
- 数据序列
- 数据类型分类
- 字符串
- 列表
- 元组
- 字典
- 列表与元组的区别详解
- 函数
- 案例综合应用
- 列表推导式
- 名片管理系统
- 文件操作
- 面向对象基础(一)
- 面向对象基础(二)
- 异常、模块
- 05 web自动化测试
- Day01
- Day02
- Day03
- Day04
- Day05
- Day06
- Day07
- Day08
- 06 接口自动化测试
- 软件测试面试大全2020
- 第一章 测试理论
- 软件测试面试
- 一、软件基础知识
- 二、网络基础知识
- 三、数据库
- SQL学生表 — 1
- SQL学生表 — 2
- SQL查询 — 3
- SQL经典面试题 — 4
- 四、linux
- a. linux常用命令
- 五、自动化测试
- 自动化测试
- python 笔试题
- selenium面试题
- 如何判断一个页面上元素是否存在?
- 如何提高脚本的稳定性?
- 如何定位动态元素?
- 如何通过子元素定位父元素?
- 如果截取某一个元素的图片,不要截取全部图片
- 平常遇到过哪些问题?如何解决的
- 一个元素明明定位到了,点击无效(也没报错),如果解决?
- selenium中隐藏元素如何定位?(hidden、display: none)
- 六、接口测试
- 接口测试常规面试题
- 接口自动化面试题
- json和字典dict的区别?
- 测试的数据你放在哪?
- 什么是数据驱动,如何参数化?
- 下个接口请求参数依赖上个接口的返回数据
- 依赖于登录的接口如何处理?
- 依赖第三方的接口如何处理
- 不可逆的操作,如何处理,比如删除一个订单这种接口如何测试
- 接口产生的垃圾数据如何清理
- 一个订单的几种状态如何全部测到,如:未处理,处理中,处理失败,处理成功
- python如何连接数据库操作?
- 七、App测试
- 什么是activity?
- Activity生命周期?
- Android四大组件
- app测试和web测试有什么区别?
- android和ios测试区别?
- app出现ANR,是什么原因导致的?
- App出现crash原因有哪些?
- app对于不稳定偶然出现anr和crash时候你是怎么处理的?
- app的日志如何抓取?
- logcat查看日志步骤
- 你平常会看日志吗, 一般会出现哪些异常
- 抓包工具
- fiddler
- Wireshark
- 安全/渗透测试
- 安全性测试都包含哪些内容?
- 开放性思维题
- 面试题
- 字节测试面试
- 一、计算机网络
- 二、操作系统
- 三、数据库
- 四、数据结构与算法
- 五、Python
- 六、Linux
- 七、测试用例
- 八、智力/场景题
- 九、开放性问题
- python3_收集100+练习题(面试题)
- python3_100道题目答案
- 接口测试
- 接口测试实例_01
- python+requests接口自动化测试框架实例详解
- 性能测试
- 性能测试流程
- 性能测试面试题
- 如何编写性能测试场景用例
- 性能测试:TPS和QPS的区别
- jmeter
- jmeter安装配置教程
- Jmeter性能测试 入门
- PyCharm
- 快捷工具
- 1-MeterSphere
- 一、安装和升级
- 2- MobaXterm 教程
- 3-fiddler抓包
- 4-Xshell
- Xshell的安装和使用
- Xshell远程连接失败怎么解决
- 5-Vmware
- Vmware提示以独占方式锁定此配置文件失败
- Windows10彻底卸载VMWare虚拟机步骤
- VM ware无法关机,虚拟机繁忙
- VMware虚拟机下载与安装
- 解决VM 与 Device/Credential Guard 不兼容。在禁用 Device/Credential Guard 后,可以运行 VM 的方法
- VMware虚拟机镜像克隆与导入
- 6-WPS
- 1.WPS文档里的批注怎么删除
- 2.wps表格中设置图表的坐标
- 3. wps快速绘制数学交集图
- 7-MongoDB
- Win10安装配置MongoDB
- Navicat 15.x for MongoDB安装破解教程
- Apache
- apache层的账户权限控制,以及apache黑名单白名单过滤功能
- HTTP / HTTPS协议
- HTTP协议详解
- 代理
- 状态码详解
- HTTPS详解
- Selenium3+python3
- (A) selenium
- selenium自动化环境搭建(Windows10)
- 火狐firebug和firepath插件安装方法(最新)
- 元素定位工具和方法
- Selenium3+python3自动化
- 新手学习selenium路线图---学前篇
- 1-操作浏览器基本方法
- 2-八种元素定位方法
- 3-CSS定位语法
- 4-登录案例
- 5-定位一组元素find_elements
- 6-操作元素(键盘和鼠标事件)
- 7-多窗口、句柄(handle)
- 8-iframe
- 9-select下拉框
- 10-alert\confirm\prompt
- 11-JS处理滚动条
- 12-单选框和复选框(radiobox、checkbox)
- 13-js处理日历控件(修改readonly属性)
- 14-js处理内嵌div滚动条
- 15-table定位
- 16-js处理多窗口
- 17-文件上传(send_keys)
- 18-获取百度输入联想词
- 19-处理浏览器弹窗
- 20-获取元素属性
- 21-判断元素存在
- 22-爬页面源码(page_source)
- 23-显式等待(WebDriverWait)
- 24-关于面试的题
- 25-cookie相关操作
- 26-判断元素(expected_conditions)
- 27-判断title(title_is)
- 28-元素定位参数化(find_element)
- 29-18种定位方法(find_elements)
- 30- js解决click失效问题
- 31- 判断弹出框存在(alert_is_present)
- 32- 登录方法(参数化)
- 33- 判断文本(text_to_be_present_in_element)
- 34- unittest简介
- 35- unittest执行顺序
- 36- unittest之装饰器(@classmethod)
- 37- unittest之断言(assert)
- 38- 捕获异常(NoSuchElementException)
- 39- 读取Excel数据(xlrd)
- 40- 数据驱动(ddt)
- 41- 异常后截图(screenshot)
- 42- jenkins持续集成环境搭建
- 43- Pycharm上python和unittest两种运行方式
- 44- 定位的坑:class属性有空格
- 45- 只截某个元素的图
- 46- unittest多线程执行用例
- 47- unittest多线程生成报告(BeautifulReport)
- 48- 多线程启动多个不同浏览器
- (B) python3+selenium3实现web UI功能自动化测试框架
- (C) selenium3常见报错处理
- 书籍
- (D)Selenium3自动化测试实战--基于Python语
- 第4章 WebDriver API
- 4.1 从定位元素开始
- 4.2 控制浏览器
- 4.3 WebDriver 中的常用方法
- 4.4 鼠标操作
- 4.5 键盘操作
- 4.6 获得验证信息
- 4.7 设置元素等待
- 4.8 定位一组元素
- 4.9 多表单切换
- 4.10 多窗口切换
- 4.11 警告框处理
- 4.12 下拉框处理
- 4.13 上传文件
- 4.14 下载文件
- 4.15 操作cookie
- 4.16 调用JavaScript
- 4.17 处理HTML5视频播放
- 4.18 滑动解锁
- 4.19 窗口截图
- 第5章 自动化测试模型
- 5.3 模块化与参数化
- 5.4 读取数据文件
- 第6章 unittest单元测试框架
- 6.1 认识unittest