## 一、构建项目
setup语法糖带来的好处:
* 定于的数据和方法,无需return。
* 引入的组件无需(components:{})注册,不在用写name属性了。
前置知识:https://github.com/vuejs/rfcs/tree/master/active-rfcs
setup语法糖:https://github.com/vuejs/rfcs/blob/master/active-rfcs/0040-script-setup.md
### 1. 运行命令
npm init vite@latest
选择vue3
选择ts
### 2. 配置端口,解决在终端启动时,出现Netword: use --host to expose问题
在vite.config.ts文件中配置以下信息
```
server: {
host: '0.0.0.0',
port: 8080,
open: true
}
```
### 3. vite配置别名
参考地址:https://vitejs.cn/config/#config-file-resolving
npm install @types/node --save-dev
```
import { resolve } from 'path'
// ...
resolve: {
alias: [{
find: '@',
replacement: resolve(__dirname, 'src')
}]
}
```
### 4. VSCode插件
关闭Vetur,安装Vue Language Features (Volar)
配置代码快速生成
在VsCode中,文件-首选项-用户片段- vue3.json.code-snippets(global),新建一个vue3.ts
### 5. 安装路由
npm install vue-router@4 --save
参考:https://router.vuejs.org/zh/installation.html
新建路由文件`router/index.ts`
``` ts
import { createRouter, createWebHistory, RouteRecordRaw } from 'vue-router'
import Layout from "@/layout/Index.vue"
const routes: Array<RouteRecordRaw> = [
{
path: '/',
name: 'Layout',
redirect: '/home',
component: Layout,
children: [
{
path: '/home',
name: 'Home',
component: () => import('@/view/home/index.vue'),
meta: {
title: '首页',
icon: 'IceCream'
}
}
]
},
{
path: '/system',
name: 'System',
component: Layout,
meta:{
title: '系统管理',
icon: 'IceCream'
},
children: [
{
path: '/menu',
name: 'Menu',
component: () => import('@/view/system/menu/index.vue'),
meta: {
title: '菜单管理',
icon: 'IceCream'
}
},
{
path: '/role',
name: 'Role',
component: () => import('@/view/system/role/index.vue'),
meta: {
title: '角色管理',
icon: 'IceCream'
}
},
{
path: '/user',
name: 'User',
component: () => import('@/view/system/user/index.vue'),
meta: {
title: '用户管理',
icon: 'IceCream'
}
}
]
}
]
// 创建路由
const router = createRouter({
history: createWebHistory(),
routes
})
export default router
```
在main.ts文件中引入,即可(测试是否生效,在页面中通过 `<router-view />`)
```
import router from './router/index'
createApp(App).use(router).mount('#app')
```
### 6. 安装Vuex4.x
cnpm install vuex@next --save
ts支持参考:https://vuex.vuejs.org/zh/guide/typescript-support.html
新建store/index.ts文件:
``` ts
import { InjectionKey } from 'vue'
import { createStore, useStore as baseUseStore, Store } from 'vuex'
export interface State {
count: number
}
export const key: InjectionKey<Store<State>> = Symbol()
export const store = createStore<State>({
state: {
count: 1
},
mutations: {
setCount(state: State, count: number) {
state.count = count
}
},
getters: {
getCount(state: State) {
return state.count
}
}
})
// 注意此处我们 自定义useStore方法
export function useStore() {
return baseUseStore(key)
}
```
在mian.ts中引入
```
import { store, key } from './store/index'
createApp(App).use(store, key).mount('#app')
```
在组件中验证
```ts
import { computed } from 'vue'
import { useStore } from '../store'
// ...
const showCount = computed(()=>{
return store.getters.getCount
})
const addCount = () => {
store.commit('setCount', ++count.value)
}
```
解决在组件中,如main.ts中 `@/...`报错问题,在 `tsconfig.json`文件中
```
// ...
"esModuleInterop": true,
"skipLibCheck": true,// 解决打包可能出现的报错问题
"lib": ["esnext", "dom"],
"baseUrl": ".",
"paths": {
"@/*": [
"src/*"
]
}
```
### 7. 配置eslint
npm install eslint eslint-plugin-vue --save-dev
根目录新建 `.eslintrc.js`文件:
```
module.exports = {
root: true,
parserOptions: {
sourceType: 'module'
},
parser: 'vue-eslint-parser',
extends: ['plugin:vue/vue3-essential', 'plugin:vue/vue3-strongly-recommended', 'plugin:vue/vue3-recommended'],
env: {
browser: true,
node: true,
es6: true
},
rules: {
'no-console': 'off',
'comma-dangle': [2, 'never'],
// ... 更多配置...
}
}
```
### 8. 添加css预处理器sass
npm install sass sass-loader --save-dev
深层样式修改:v-deep、:deep等方式。
### 9. 引入element-plus
cnpm install element-plus --save
图标:cnpm install @element-plus/icons-vue --save
在main.ts中引入,有两种方式,选其一即可。
* 方式一:配合 `<component :is="iconStr"></component> `使用,iconStr字符串开头字母可小写
* 方式二:配合`<Icon :icon="iconStr"> </Icon>`使用,iconStr字符串**开头必须大写,且驼峰命名**,和Icon图标名称保持一致。
* 注:在tsconfig.json文件中,配置`"suppressImplicitAnyIndexErrors": true,`可解决`Icons[icon]`的问题
```
import ElementPlus from 'element-plus'
import 'element-plus/dist/index.css'
import * as Icons from '@element-plus/icons-vue'
// ...
const app = createApp(App).use(router).use(store, key).use(ElementPlus)
// 方式一:配合 <component :is="icon"></component> 使用,icon字符串开头字母可小写
// 全局注册图标,暂时用于el-menu中菜单自定义(动态组件)图标
Object.keys(Icons).forEach(key=>{
app.component(key, Icons[key as keyof typeof Icons])
})
// 方式二:
const Icon = (props: { icon: string }) => {
const { icon } = props
return createVNode(Icons[icon as keyof typeof Icons])
}
app.component('Icon', Icon)
app.mount('#app')
```
## 二、布局
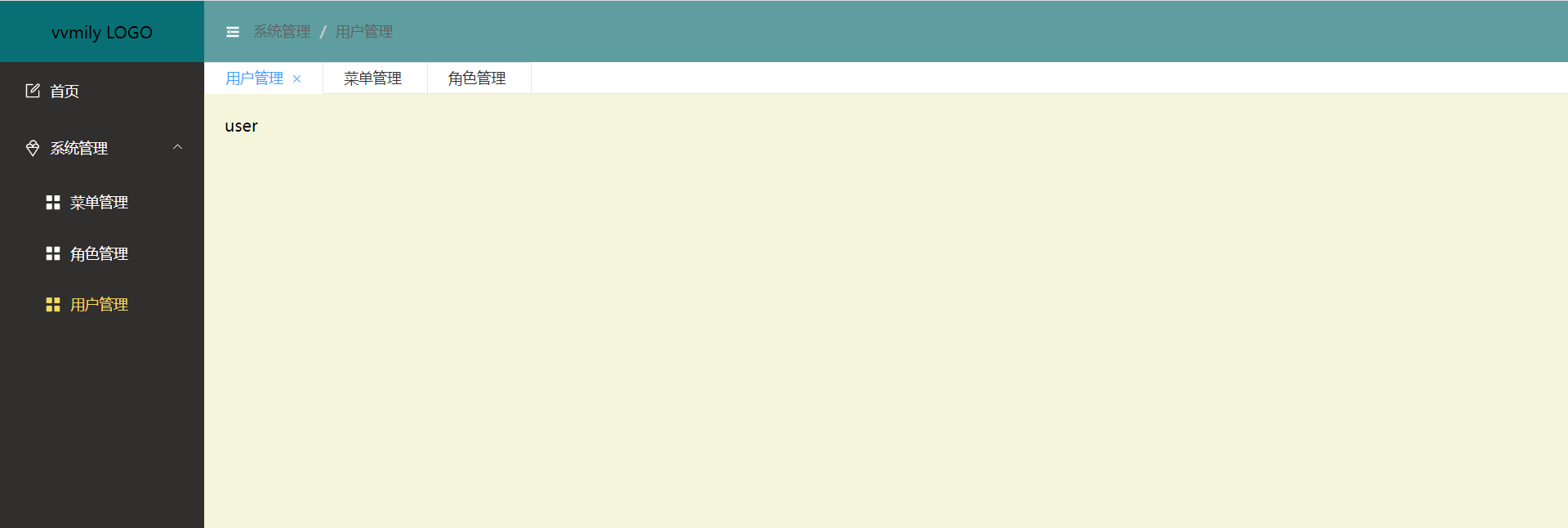
* 文件目录
```
layout
---Index.vue
---aside
------Index.vue
------MenuItem.vue
---header
------Index.vue
------Breadcrumb.vue
---tabs
------Index.vue
```
* layout/index.vue
```
<template>
<el-container class="vv-container">
<el-aside width="auto" class="vv-aside">
<div class="logo">{{ isCollapse ? "vvmily" : "vvmily LOGO" }}</div>
<Aside />
</el-aside>
<el-container>
<el-header class="vv-header">
<Header />
</el-header>
<Tabs />
<el-main class="vv-main">
<router-view />
</el-main>
</el-container>
</el-container>
</template>
// ... 省略
```
在此el-container布局中,在这里重点关注一下左侧菜单`<Aside />`实现以及 `collapse`(配合 `vuex`)的控制,各个文件请[点击前往查看仓库](https://gitee.com/ming112/vvmily-vite-vue3-admin)。好了,到这里大体不居已经出来,剩下文字等细节样式问题,可自行补充。
- 首页
- 2021年
- 基础知识
- 同源策略
- 跨域
- css
- less
- scss
- reset
- 超出文本显示省略号
- 默认滚动条
- 清除浮动
- line-height与vertical-align
- box-sizing
- 动画
- 布局
- JavaScript
- 设计模式
- 深浅拷贝
- 排序
- canvas
- 防抖节流
- 获取屏幕/可视区域宽高
- 正则
- 重绘重排
- rem换算
- 手写算法
- apply、call和bind原理与实现
- this的理解-普通函数、箭头函数
- node
- nodejs
- express
- koa
- egg
- 基于nodeJS的全栈项目
- 小程序
- 常见问题
- ec-canvas之横竖屏切换重绘
- 公众号后台基本配置
- 小程序发布协议更新
- 小程序引入iconfont字体
- Uni-app
- 环境搭建
- 项目搭建
- 数据库
- MySQL数据库安装
- 数据库图形化界面常用命令行
- cmd命令行操作数据库
- Redis安装
- APP
- 控制缩放meta
- GIT
- 常用命令
- vsCode
- 常用插件
- Ajax
- axios-services
- 文章
- 如何让代码更加优雅
- 虚拟滚动
- 网站收藏
- 防抖节流之定时器清除问题
- 号称破解全网会员的脚本
- 资料笔记
- 资料笔记2
- 公司面试题
- 服务器相关
- 前端自动化部署-jenkins
- nginx.conf配置
- https添加证书
- shell基本命令
- 微型ssh-deploy前端部署插件
- webpack
- 深入理解loader
- 深入理解plugin
- webpack注意事项
- vite和webpack区别
- React
- react+antd搭建
- Vue
- vue-cli
- vue.config.js
- 面板分割左右拖动
- vvmily-admin-template
- v-if与v-for那个优先级高?
- 下载excel
- 导入excel
- Echart-China-Map
- vue-xlsx(解析excel)
- 给elementUI的el-table添加骨架
- cdn引入配置
- Vue2.x之defineProperty应用
- 彻底弄懂diff算法的key作用
- 复制模板内容
- 表格操作按钮太多
- element常用组件二次封装
- Vue3.x
- Vue3快速上手(第一天)
- Vue3.x快速上手(第二天)
- Vue3.x快速上手(第三天)
- vue3+element-plus搭建项目
- vue3
- 脚手架
- vvmily-cli
- TS
- ts笔记
- common
- Date
- utils
- axios封装
- 2022年
- HTML
- CSS基础
- JavaScript 基础
- 前端框架Vue
- 计算机网络
- 浏览器相关
- 性能优化
- js手写代码
- 前端安全
- 前端算法
- 前端构建与编译
- 操作系统
- Node.js
- 一些开放问题、智力题