# 1. 插值器Interpolator
前面提到过,在`xml`中使用`android:interpolator`用来指定一个插值器,它会影响动画的播放速度。系统常见的插值器有:
* 线性插值器,`LinearInterpolator`。
* 加速减速插值器:`AccelerateDecelerateInterpolator`。
* 减速插值器:`DecelerateInterpolator`。
这里以`LinearInterpolator`为案例:
~~~java
public class LinearInterpolator extends BaseInterpolator implements NativeInterpolator {
public LinearInterpolator() {
}
public LinearInterpolator(Context context, AttributeSet attrs) {
}
public float getInterpolation(float input) {
return input;
}
/** @hide */
@Override
public long createNativeInterpolator() {
return NativeInterpolatorFactory.createLinearInterpolator();
}
}
~~~
从代码可以知道输入和输出都是`input`,也就是说斜率为常数。这里举一个案例,在`40ms`内,使用线性插值器将`View`的`x`从`0`变换到`40`。动画的刷新频率为`10ms/帧`,那么我们可以得出下面几个刷新时刻:
| 时间段 | 0 | 1 |2|3|4|
| --- | --- |--- |--- |--- |--- |
| t | 0ms | 10ms | 20ms | 30ms| 40ms |
具体的`x`变为了什么值,这里就需要使用估值器,比如:
~~~
public class IntEvaluator implements TypeEvaluator<Integer> {
/**
* This function returns the result of linearly interpolating the start and end values, with
* <code>fraction</code> representing the proportion between the start and end values. The
* calculation is a simple parametric calculation: <code>result = x0 + t * (x1 - x0)</code>,
* where <code>x0</code> is <code>startValue</code>, <code>x1</code> is <code>endValue</code>,
* and <code>t</code> is <code>fraction</code>.
*
* @param fraction The fraction from the starting to the ending values
* @param startValue The start value; should be of type <code>int</code> or
* <code>Integer</code>
* @param endValue The end value; should be of type <code>int</code> or <code>Integer</code>
* @return A linear interpolation between the start and end values, given the
* <code>fraction</code> parameter.
*/
public Integer evaluate(float fraction, Integer startValue, Integer endValue) {
int startInt = startValue;
return (int)(startInt + fraction * (endValue - startInt));
}
}
~~~
也就是传入当前的百分比,得到估值。计算公式为:
```
result = x0 + t * (x1 - x0)
```
简单理解一下也就是起始位置`x0`加上传入的比例`t`根据其移动距离转化后的长度值。
那么,类似的这里简单摘要一下`AccelerateDecelerateInterpolator`的源码:
~~~
public class AccelerateDecelerateInterpolator extends BaseInterpolator
implements NativeInterpolator {
public AccelerateDecelerateInterpolator() {
}
@SuppressWarnings({"UnusedDeclaration"})
public AccelerateDecelerateInterpolator(Context context, AttributeSet attrs) {
}
public float getInterpolation(float input) {
return (float)(Math.cos((input + 1) * Math.PI) / 2.0f) + 0.5f;
}
}
~~~
其计算也就是:
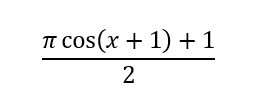
当然,我们也可以定义自己的插值器。
# 2. 估值器Evaluator
前面我们使用过`ArgbEvaluator`,主要用来计算改变后的百分比对应的值。比如:`IntEvaluator`、`FloatEvaluator`和`ArgbEvaluator`。
上面介绍了`IntEvaluator`,我们知道其计算也就是根据比例来计算值。那么对应的`FloatEvaluator`这里也简单看下:
~~~
public class FloatEvaluator implements TypeEvaluator<Number> {
public Float evaluate(float fraction, Number startValue, Number endValue) {
float startFloat = startValue.floatValue();
return startFloat + fraction * (endValue.floatValue() - startFloat);
}
}
~~~
可以看到这里的计算和`IntEvaluator`保持一致,只是类型不同。
同样的,也可以自定义一个估值器来计算。
- 介绍
- UI
- MaterialButton
- MaterialButtonToggleGroup
- 字体相关设置
- Material Design
- Toolbar
- 下拉刷新
- 可折叠式标题栏
- 悬浮按钮
- 滑动菜单DrawerLayout
- NavigationView
- 可交互提示
- CoordinatorLayout
- 卡片式布局
- 搜索框SearchView
- 自定义View
- 简单封装单选
- RecyclerView
- xml设置点击样式
- adb
- 连接真机
- 小技巧
- 通过字符串ID获取资源
- 自定义View组件
- 使用系统控件重新组合
- 旋转菜单
- 轮播图
- 下拉输入框
- 自定义VIew
- 图片组合的开关按钮
- 自定义ViewPager
- 联系人快速索引案例
- 使用ListView定义侧滑菜单
- 下拉粘黏效果
- 滑动冲突
- 滑动冲突之非同向冲突
- onMeasure
- 绘制字体
- 设置画笔Paint
- 贝赛尔曲线
- Invalidate和PostInvalidate
- super.onTouchEvent(event)?
- setShadowLayer与阴影效果
- Shader
- ImageView的scaleType属性
- 渐变
- LinearGradient
- 图像混合模式
- PorterDuffXfermode
- 橡皮擦效果
- Matrix
- 离屏绘制
- Canvas和图层
- Canvas简介
- Canvas中常用操作总结
- Shape
- 圆角属性
- Android常见动画
- Android动画简介
- View动画
- 自定义View动画
- View动画的特殊使用场景
- LayoutAnimation
- Activity的切换转场效果
- 属性动画
- 帧动画
- 属性动画监听
- 插值器和估值器
- 工具
- dp和px的转换
- 获取屏幕宽高
- JNI
- javah命令
- C和Java相互调用
- WebView
- Android Studio快捷键
- Bitmap和Drawable图像
- Bitmap简要介绍
- 图片缩放和裁剪效果
- 创建指定颜色的Bitmap图像
- Gradle本地仓库
- Gradle小技巧
- RxJava+Okhttp+Retrofit构建网络模块
- 服务器相关配置
- node环境配置
- 3D特效