很少有项目是仅仅有一个模块组成的。我们当前的前台`app`目录如下:
```
.
├── app-routing.module.ts
├── app.component.html
├── app.component.sass
├── app.component.spec.ts
├── app.component.ts
├── app.module.ts
├── teacher-add.component.html
├── teacher-add.component.ts
├── teacher-edit.component.html
└── teacher-edit.component.ts
```
如果再有其它的模块(比如我们马上要进行开发的班级管理模块),则会面临如下问题:
* 教师管理模块占用了入口组件AppComponent,其它模块没有地方安置。
* 教师的增加、编辑生了四个新文件,随着模块数量的增加,该目录面临文件数过多。
* 直接使用`/add`路径来增加教师,语义没有`/teacher/add`明确。
* 路由配置文件app-routing.module单一,多人开发时容易产生冲突。
* 路由配置文件app-routing.module单一,随着模块的增多,将文件内容会过长而变得无法维护。
引入班级管理后,我们希望看到如下的路由:
| URL | 组件 | 功能 |
| ---- | ---- | ---- |
| <空> | App | 首页,显示欢迎界面,显示导航栏 |
| `/teacher` | TeacherIndex | 教师列表页 |
| `/teacher/add` | TeacherAdd | 增加教师 |
| `/teacher/edit/<id>` | TeacherEdit | 编辑教师 |
| `/klass` | KlassIndex | 班级列表页 |
| `/klass/add` | KlassAdd | 增加班级 |
| `/Klass/edit/<id>` | KlassEdit | 编辑班级 |
# 文件夹化
对项目文件进行重构最简单有效的方法便是划分文件夹,比如我们把教师管理的文件全部放到`app/teacher`文件夹下,把班级管理的文件全部放到`app/klass`文件夹下:
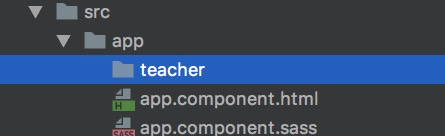
然后借助于`webstorm`来完成迁移:
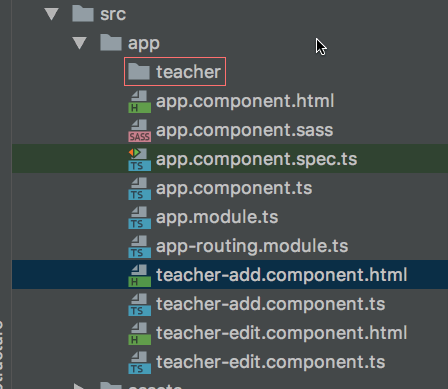
> **注意:** 不能批量选中迁移否则`webstorm`无法为我们自动处理引用路径的问题。
迁移后目录如下:
```
.
├── app-routing.module.ts
├── app.component.html
├── app.component.sass
├── app.component.spec.ts
├── app.component.ts
├── app.module.ts
└── teacher
├── teacher-add.component.html
├── teacher-add.component.ts
├── teacher-edit.component.html
└── teacher-edit.component.ts
```
## 新建TeacherIndex组件
新建TeacherIndex组件,并复制原App组件中相关的代码至:
teacher/teacher-index.component.ts
```
import {Component, OnInit} from '@angular/core';
import {HttpClient} from '@angular/common/http';
@Component({
templateUrl: './teacher-index.component.html',
})
export class TeacherIndexComponent implements OnInit {
// 定义教师数组
teachers = new Array();
constructor(private httpClient: HttpClient) {
}
/**
* 该方法将在组件准备完毕后被调用
*/
ngOnInit() {
/* 后台数据的请求地址,如果变量定义后不再重新赋值,则应该使用const来定义 */
const url = 'http://localhost:8080/Teacher/';
/* 使用get方法请求url,请求一旦成功后,将调用传入的第一个方法;如果请求失败,将调用传入的第二个方法 */
this.httpClient.get(url)
.subscribe((response: any) => {
console.log(response);
this.teachers = response;
}, (response) => {
console.log(response);
console.error('请求出错');
});
}
/**
* 点击删除按钮时删除对应的教师
* @param teacher 要删除的教师
*/
onDelete(teacher: { id: string }): void {
const url = 'http://localhost:8080/Teacher/' + teacher.id;
this.httpClient.delete(url)
.subscribe(() => {
console.log('删除成功');
this.ngOnInit();
}, () => {
console.log('删除失败');
});
}
}
```
将app组件的V层转移至teacher/teacher-index.component.html
```
<router-outlet></router-outlet>
<table>
<tr>
<th>序号</th>
<th>姓名</th>
<th>用户名</th>
<th>邮箱</th>
<th>性别</th>
<th>注册时间</th>
<th>操作</th>
</tr>
<tr *ngFor="let teacher of teachers">
<td>{{teacher.id}}</td>
<td>{{teacher.name}}</td>
<td>{{teacher.username}}</td>
<td>{{teacher.email}}</td>
<td>
<span *ngIf="teacher.sex">女</span>
<span *ngIf="!teacher.sex">男</span>
</td>
<td>{{teacher.createTime | date: 'yyyy-MM-dd'}}</td>
<td><a [routerLink]="['/edit', teacher.id]">编辑</a> <button (click)="onDelete(teacher)">删除</button></td>
</tr>
</table>
```
## 去冗余代码
此时教师列表已经被我们转到了新的TeacherIndex组件中,我们恢复下App组件的代码:
app.component.html
```
<h2>欢迎使用河北工业大学教务管理系统</h2>
<router-outlet></router-outlet>
```
app.component
```
import {Component} from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.sass']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
}
}
```
## 新组件添加至模块
app.module
```
import {TeacherIndexComponent} from './teacher/teacher-index.component'; ①
@NgModule({
declarations: [
AppComponent,
TeacherAddComponent,
TeacherEditComponent,
TeacherIndexComponent ②
],
```
## 测试
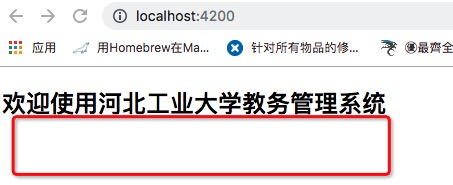
测试我们发现,教师列表没有了。
这是由于在重构前我们使用了`App`组件来显示教师列表;重构以后`App`组件仅显示欢迎信息,当然教师列表就随着不存在了。下面使用定义空路由路径的方法来默认显示教师列表组件。
## 重新定义路由
app-routing.module
```
import {TeacherIndexComponent} from './teacher/teacher-index.component';
const routes: Routes = [
{
path: '', ➊
component: TeacherIndexComponent ➋
},
{
path: 'add',
component: TeacherAddComponent
},
{
path: 'edit/:id',
component: TeacherEditComponent
}
];
```
* ➊路径为空时,匹配➋TeacherIndexComponent组件
此时当访问[http://localhost:4200/](http://localhost:4200/)时,则默认匹配上了空路径,进而将显示教师列表组件。
# 测试
打开[http://localhost:4200](http://localhost:4200)及控制台。查看功能是否正常。
# 参考文档
| 名称 | 链接 | 预计学习时长(分) |
| --- | --- | --- |
| 源码地址 | [https://github.com/mengyunzhi/spring-boot-and-angular-guild/releases/tag/step2.6.1](https://github.com/mengyunzhi/spring-boot-and-angular-guild/releases/tag/step2.6.1) | - |
- 序言
- 第一章:Hello World
- 第一节:Angular准备工作
- 1 Node.js
- 2 npm
- 3 WebStorm
- 第二节:Hello Angular
- 第三节:Spring Boot准备工作
- 1 JDK
- 2 MAVEN
- 3 IDEA
- 第四节:Hello Spring Boot
- 1 Spring Initializr
- 2 Hello Spring Boot!
- 3 maven国内源配置
- 4 package与import
- 第五节:Hello Spring Boot + Angular
- 1 依赖注入【前】
- 2 HttpClient获取数据【前】
- 3 数据绑定【前】
- 4 回调函数【选学】
- 第二章 教师管理
- 第一节 数据库初始化
- 第二节 CRUD之R查数据
- 1 原型初始化【前】
- 2 连接数据库【后】
- 3 使用JDBC读取数据【后】
- 4 前后台对接
- 5 ng-if【前】
- 6 日期管道【前】
- 第三节 CRUD之C增数据
- 1 新建组件并映射路由【前】
- 2 模板驱动表单【前】
- 3 httpClient post请求【前】
- 4 保存数据【后】
- 5 组件间调用【前】
- 第四节 CRUD之U改数据
- 1 路由参数【前】
- 2 请求映射【后】
- 3 前后台对接【前】
- 4 更新数据【前】
- 5 更新某个教师【后】
- 6 路由器链接【前】
- 7 观察者模式【前】
- 第五节 CRUD之D删数据
- 1 绑定到用户输入事件【前】
- 2 删除某个教师【后】
- 第六节 代码重构
- 1 文件夹化【前】
- 2 优化交互体验【前】
- 3 相对与绝对地址【前】
- 第三章 班级管理
- 第一节 JPA初始化数据表
- 第二节 班级列表
- 1 新建模块【前】
- 2 初识单元测试【前】
- 3 初始化原型【前】
- 4 面向对象【前】
- 5 测试HTTP请求【前】
- 6 测试INPUT【前】
- 7 测试BUTTON【前】
- 8 @RequestParam【后】
- 9 Repository【后】
- 10 前后台对接【前】
- 第三节 新增班级
- 1 初始化【前】
- 2 响应式表单【前】
- 3 测试POST请求【前】
- 4 JPA插入数据【后】
- 5 单元测试【后】
- 6 惰性加载【前】
- 7 对接【前】
- 第四节 编辑班级
- 1 FormGroup【前】
- 2 x、[x]、{{x}}与(x)【前】
- 3 模拟路由服务【前】
- 4 测试间谍spy【前】
- 5 使用JPA更新数据【后】
- 6 分层开发【后】
- 7 前后台对接
- 8 深入imports【前】
- 9 深入exports【前】
- 第五节 选择教师组件
- 1 初始化【前】
- 2 动态数据绑定【前】
- 3 初识泛型
- 4 @Output()【前】
- 5 @Input()【前】
- 6 再识单元测试【前】
- 7 其它问题
- 第六节 删除班级
- 1 TDD【前】
- 2 TDD【后】
- 3 前后台对接
- 第四章 学生管理
- 第一节 引入Bootstrap【前】
- 第二节 NAV导航组件【前】
- 1 初始化
- 2 Bootstrap格式化
- 3 RouterLinkActive
- 第三节 footer组件【前】
- 第四节 欢迎界面【前】
- 第五节 新增学生
- 1 初始化【前】
- 2 选择班级组件【前】
- 3 复用选择组件【前】
- 4 完善功能【前】
- 5 MVC【前】
- 6 非NULL校验【后】
- 7 唯一性校验【后】
- 8 @PrePersist【后】
- 9 CM层开发【后】
- 10 集成测试
- 第六节 学生列表
- 1 分页【后】
- 2 HashMap与LinkedHashMap
- 3 初识综合查询【后】
- 4 综合查询进阶【后】
- 5 小试综合查询【后】
- 6 初始化【前】
- 7 M层【前】
- 8 单元测试与分页【前】
- 9 单选与多选【前】
- 10 集成测试
- 第七节 编辑学生
- 1 初始化【前】
- 2 嵌套组件测试【前】
- 3 功能开发【前】
- 4 JsonPath【后】
- 5 spyOn【后】
- 6 集成测试
- 7 @Input 异步传值【前】
- 8 值传递与引入传递
- 9 @PreUpdate【后】
- 10 表单验证【前】
- 第八节 删除学生
- 1 CSS选择器【前】
- 2 confirm【前】
- 3 功能开发与测试【后】
- 4 集成测试
- 5 定制提示框【前】
- 6 引入图标库【前】
- 第九节 集成测试
- 第五章 登录与注销
- 第一节:普通登录
- 1 原型【前】
- 2 功能设计【前】
- 3 功能设计【后】
- 4 应用登录组件【前】
- 5 注销【前】
- 6 保留登录状态【前】
- 第二节:你是谁
- 1 过滤器【后】
- 2 令牌机制【后】
- 3 装饰器模式【后】
- 4 拦截器【前】
- 5 RxJS操作符【前】
- 6 用户登录与注销【后】
- 7 个人中心【前】
- 8 拦截器【后】
- 9 集成测试
- 10 单例模式
- 第六章 课程管理
- 第一节 新增课程
- 1 初始化【前】
- 2 嵌套组件测试【前】
- 3 async管道【前】
- 4 优雅的测试【前】
- 5 功能开发【前】
- 6 实体监听器【后】
- 7 @ManyToMany【后】
- 8 集成测试【前】
- 9 异步验证器【前】
- 10 详解CORS【前】
- 第二节 课程列表
- 第三节 果断
- 1 初始化【前】
- 2 分页组件【前】
- 2 分页组件【前】
- 3 综合查询【前】
- 4 综合查询【后】
- 4 综合查询【后】
- 第节 班级列表
- 第节 教师列表
- 第节 编辑课程
- TODO返回机制【前】
- 4 弹出框组件【前】
- 5 多路由出口【前】
- 第节 删除课程
- 第七章 权限管理
- 第一节 AOP
- 总结
- 开发规范
- 备用