本小节进行StudentService.page方法的功能开发。打开src/app/service文件夹并找到student.service.ts及student.service.spec.ts。
# 功能代码
```
import {HttpClient, HttpParams} from '@angular/common/http';
...
export class StudentService {
...
/**
* 分页
* @param params name:名称,sno:学号,klassId:班级ID,page:第几页,size:每页大小
*/
page(params: { name?: string, sno?: string, klassId?: number, page?: number, size?: number}):
Observable<{ totalPages: number, content: Array<Student> }> {
const url = 'http://localhost:8080/Student';
/* 设置默认值① */
if (params.page === undefined) {
params.page = 0;
}
if (params.size === undefined) {
params.size = 10;
}
/* 初始化查询参数 */
const queryParams = new HttpParams()➊
.➋set('name', params.name ? params.name : '')➌
.set('sno', params.sno ? params.sno : ''②)
.set('klassId', params.klassId ? params.klassId.toString() : '')
.set('page', params.page.toString())
.set('size', params.size.toString());
console.log(queryParams);★
return this.httpClient.get<{ totalPages: number, content: Array<Student> ③}>(url, {params: queryParams})➍;
}
```
* ① 设置默认值的一种方式
* ➊ 初始化参数类型为HttpParams
* ➋ 使用连续操作来添加查询参数
* ➌ set(string, string),发起http查询时参数的类型必须为字符串(或字符串数组)
* ② 设置默认值的另一种方式
* ★ 对某个类型还不熟悉的时候,将对象打印到控制台很关键
* ③ 定义get方法返回值的格式
* ➍ `HttpClient.get(请求地址, 其它选项)`,需要传入查询参数则使用`HttpClient.get(请求地址, {params: HttpParams})`。
# 测试代码
在功能代码第一次使用HttpClient的带有参数的get方法,在正式的进行mock测试之前,我们先来看看它会发起什么样的真实请求。此时,单元测试中引入的HttpClientTestingModule便不符合我们此时的需求了。我们将其暂时删除掉,引入会发起真实http请求的HttpClientModule。
```
describe('service -> StudentService', () => {
let service: StudentService;
beforeEach(() => TestBed.configureTestingModule({
imports: [
HttpClientTestingModule, ✘
HttpClientModule, ✚
]
}));
```
然后如下编写page单元测试,并在chrome中打开控制台。
```
describe('service -> StudentService', () => {
...
/* 分页测试 */
fit('page', () => {
service.page({}).subscribe();
service.page({name: 'name'}).subscribe();
service.page({page: 2}).subscribe();
});
```
观察控制台,的确发起了我们预期的请求信息:
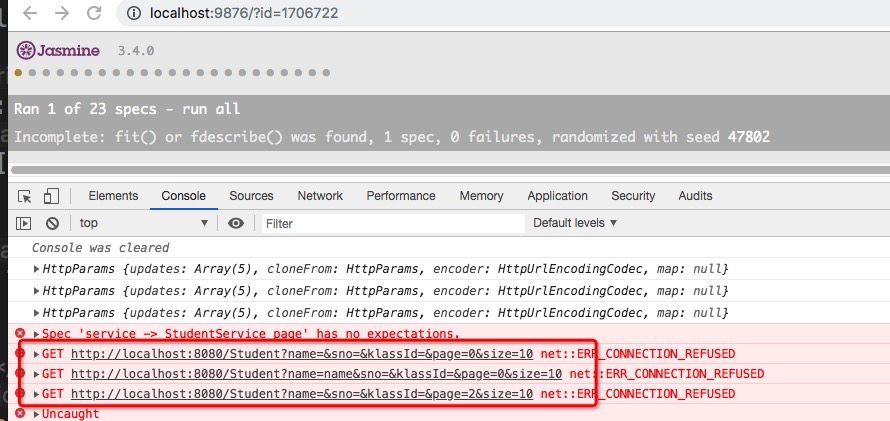
当传入空对象时,以默认值发起GET请求;当传入name值时,以正确的name值发起的GET请求;当传入page时,以正确的page值发起请求。
## MockHttp
查看完发起的真正请求后,继续切换为模拟的Http请求模块来进行进一步测试:
```
describe('service -> StudentService', () => {
let service: StudentService;
beforeEach(() => TestBed.configureTestingModule({
imports: [
HttpClientTestingModule, ✚
HttpClientModule, ✘
]
}));
```
测试的思路与save方法相同:①构造模拟返回值 ②以特定的参数值调用测试方法 ③断言发起了预期的http请求 ④断言接收到了预期的数据。
```
/* 分页测试 */
fit('page', () => {
/* 模拟返回数据 */
const mockResult = {
totalPages: 10,
content: new Array(new Student({}), new Student({}))
};
/* 进行订阅,发送数据后将called置true */
let called = false;
service.page({}).subscribe((success: { totalPages: number, content: Array<Student> }) => {
called = true;②
expect(success.totalPages).toEqual(10);
expect(success.content.length).toBe(2);
});
/* 断言发起了http请求,方法为get;请求参数值符合预期 */
const req = TestBed.get(HttpTestingController).expectOne((request: HttpRequest<any>) => {
return request.url === 'http://localhost:8080/Student'; ➋
});➊
expect(req.request.method).toEqual('GET');
expect(req.request.params.get('name')).toEqual(''); ➌
expect(req.request.params.get('sno')).toEqual('');
expect(req.request.params.get('klassId')).toEqual('');
expect(req.request.params.get('page')).toEqual('0');
expect(req.request.params.get('size')).toEqual('10');
req.flush(mockResult); ①
expect(called).toBe(true);③
});
```
* ➊ expectOne(matchFn) matchFn为匹配函数,该函数被调用时传入值类型为HttpRequest。
* ➋ 返回值为true表示匹配成功
* ➌ 断言返回了默认的请求参数
* ①②③ 顺序执行,断言接收到了返回数据且返回数据符合预期
## 断言参数
本着测试粒度最小化原则,再新建一个测试用例:
```
/* 分页参数测试 */
fit('page params test', () => {
service.page({name: 'name', sno: 'sno', klassId: 1, page: 2, size: 20}).subscribe();
/* 断言发起了http请求,方法为get;请求参数值符合预期 */
const req = TestBed.get(HttpTestingController).expectOne(
request => request.url === 'http://localhost:8080/Student'
); ➊
expect(req.request.method).toEqual('GET');
expect(req.request.params.get('name')).toEqual('name'); ①
expect(req.request.params.get('sno')).toEqual('sno');
expect(req.request.params.get('klassId')).toEqual('1');
expect(req.request.params.get('page')).toEqual('2');
expect(req.request.params.get('size')).toEqual('20');
req.flush({}); ➋
});
```
* ➊ 当箭头函数中仅有一行代码且使用return返回时,则可以简写为此形式
* ① 断言使用了使用的参数发起了http请求
* ➋ 由于本例的测试内容为**参数是否正确传入**,所以可以完全忽略请求返回值,直接将`{}`做为返回值即可
# 参考文档
| 名称 | 链接 | 预计学习时长(分) |
| --- | --- | --- |
| 源码地址 | [https://github.com/mengyunzhi/spring-boot-and-angular-guild/releases/tag/step4.6.7](https://github.com/mengyunzhi/spring-boot-and-angular-guild/releases/tag/step4.6.7) | - |
| HttpClient GET | [https://www.angular.cn/api/common/http/HttpClient#get](https://www.angular.cn/api/common/http/HttpClient#get) | - |
- 序言
- 第一章:Hello World
- 第一节:Angular准备工作
- 1 Node.js
- 2 npm
- 3 WebStorm
- 第二节:Hello Angular
- 第三节:Spring Boot准备工作
- 1 JDK
- 2 MAVEN
- 3 IDEA
- 第四节:Hello Spring Boot
- 1 Spring Initializr
- 2 Hello Spring Boot!
- 3 maven国内源配置
- 4 package与import
- 第五节:Hello Spring Boot + Angular
- 1 依赖注入【前】
- 2 HttpClient获取数据【前】
- 3 数据绑定【前】
- 4 回调函数【选学】
- 第二章 教师管理
- 第一节 数据库初始化
- 第二节 CRUD之R查数据
- 1 原型初始化【前】
- 2 连接数据库【后】
- 3 使用JDBC读取数据【后】
- 4 前后台对接
- 5 ng-if【前】
- 6 日期管道【前】
- 第三节 CRUD之C增数据
- 1 新建组件并映射路由【前】
- 2 模板驱动表单【前】
- 3 httpClient post请求【前】
- 4 保存数据【后】
- 5 组件间调用【前】
- 第四节 CRUD之U改数据
- 1 路由参数【前】
- 2 请求映射【后】
- 3 前后台对接【前】
- 4 更新数据【前】
- 5 更新某个教师【后】
- 6 路由器链接【前】
- 7 观察者模式【前】
- 第五节 CRUD之D删数据
- 1 绑定到用户输入事件【前】
- 2 删除某个教师【后】
- 第六节 代码重构
- 1 文件夹化【前】
- 2 优化交互体验【前】
- 3 相对与绝对地址【前】
- 第三章 班级管理
- 第一节 JPA初始化数据表
- 第二节 班级列表
- 1 新建模块【前】
- 2 初识单元测试【前】
- 3 初始化原型【前】
- 4 面向对象【前】
- 5 测试HTTP请求【前】
- 6 测试INPUT【前】
- 7 测试BUTTON【前】
- 8 @RequestParam【后】
- 9 Repository【后】
- 10 前后台对接【前】
- 第三节 新增班级
- 1 初始化【前】
- 2 响应式表单【前】
- 3 测试POST请求【前】
- 4 JPA插入数据【后】
- 5 单元测试【后】
- 6 惰性加载【前】
- 7 对接【前】
- 第四节 编辑班级
- 1 FormGroup【前】
- 2 x、[x]、{{x}}与(x)【前】
- 3 模拟路由服务【前】
- 4 测试间谍spy【前】
- 5 使用JPA更新数据【后】
- 6 分层开发【后】
- 7 前后台对接
- 8 深入imports【前】
- 9 深入exports【前】
- 第五节 选择教师组件
- 1 初始化【前】
- 2 动态数据绑定【前】
- 3 初识泛型
- 4 @Output()【前】
- 5 @Input()【前】
- 6 再识单元测试【前】
- 7 其它问题
- 第六节 删除班级
- 1 TDD【前】
- 2 TDD【后】
- 3 前后台对接
- 第四章 学生管理
- 第一节 引入Bootstrap【前】
- 第二节 NAV导航组件【前】
- 1 初始化
- 2 Bootstrap格式化
- 3 RouterLinkActive
- 第三节 footer组件【前】
- 第四节 欢迎界面【前】
- 第五节 新增学生
- 1 初始化【前】
- 2 选择班级组件【前】
- 3 复用选择组件【前】
- 4 完善功能【前】
- 5 MVC【前】
- 6 非NULL校验【后】
- 7 唯一性校验【后】
- 8 @PrePersist【后】
- 9 CM层开发【后】
- 10 集成测试
- 第六节 学生列表
- 1 分页【后】
- 2 HashMap与LinkedHashMap
- 3 初识综合查询【后】
- 4 综合查询进阶【后】
- 5 小试综合查询【后】
- 6 初始化【前】
- 7 M层【前】
- 8 单元测试与分页【前】
- 9 单选与多选【前】
- 10 集成测试
- 第七节 编辑学生
- 1 初始化【前】
- 2 嵌套组件测试【前】
- 3 功能开发【前】
- 4 JsonPath【后】
- 5 spyOn【后】
- 6 集成测试
- 7 @Input 异步传值【前】
- 8 值传递与引入传递
- 9 @PreUpdate【后】
- 10 表单验证【前】
- 第八节 删除学生
- 1 CSS选择器【前】
- 2 confirm【前】
- 3 功能开发与测试【后】
- 4 集成测试
- 5 定制提示框【前】
- 6 引入图标库【前】
- 第九节 集成测试
- 第五章 登录与注销
- 第一节:普通登录
- 1 原型【前】
- 2 功能设计【前】
- 3 功能设计【后】
- 4 应用登录组件【前】
- 5 注销【前】
- 6 保留登录状态【前】
- 第二节:你是谁
- 1 过滤器【后】
- 2 令牌机制【后】
- 3 装饰器模式【后】
- 4 拦截器【前】
- 5 RxJS操作符【前】
- 6 用户登录与注销【后】
- 7 个人中心【前】
- 8 拦截器【后】
- 9 集成测试
- 10 单例模式
- 第六章 课程管理
- 第一节 新增课程
- 1 初始化【前】
- 2 嵌套组件测试【前】
- 3 async管道【前】
- 4 优雅的测试【前】
- 5 功能开发【前】
- 6 实体监听器【后】
- 7 @ManyToMany【后】
- 8 集成测试【前】
- 9 异步验证器【前】
- 10 详解CORS【前】
- 第二节 课程列表
- 第三节 果断
- 1 初始化【前】
- 2 分页组件【前】
- 2 分页组件【前】
- 3 综合查询【前】
- 4 综合查询【后】
- 4 综合查询【后】
- 第节 班级列表
- 第节 教师列表
- 第节 编辑课程
- TODO返回机制【前】
- 4 弹出框组件【前】
- 5 多路由出口【前】
- 第节 删除课程
- 第七章 权限管理
- 第一节 AOP
- 总结
- 开发规范
- 备用