# TypeScript类
本节我们共同完成上节中的作业:新建Teacher类。
## 实体类
在团队项中,我们更愿意将与后台返回数据对应的类称为实体Entity类,表示此类与后台返回的数据格式一致。教师数据便是典型的由后台获取的数据,所以我们在`app`文件夹下新下`entity`文件夹:
```bash
panjie@panjies-Mac-Pro app % pwd
/Users/panjie/github/mengyunzhi/angular11-guild/first-app/src/app
panjie@panjies-Mac-Pro app % tree -L 1
.
├── add
├── app-routing.module.ts
├── app.component.css
├── app.component.html
├── app.component.spec.ts
├── app.component.ts
├── app.module.ts
├── edit
├── entity 👈
├── index
└── login
5 directories, 6 files
```
然后进入该文件夹,使用angular cli自动创建一个普通的teacher类:
```bash
panjie@panjies-Mac-Pro entity % pwd
/Users/panjie/github/mengyunzhi/angular11-guild/first-app/src/app/entity
panjie@panjies-Mac-Pro entity % ng g class teacher
CREATE src/app/entity/teacher.spec.ts (158 bytes)
CREATE src/app/entity/teacher.ts (25 bytes)
```
Angualr cli将自动创建一个Teacher类及该类对应的测试文件。
## class Teacher
此时我们便可以定义Teacher的属性了:
```typescript
+++ b/first-app/src/app/entity/teacher.ts
@@ -1,2 +1,11 @@
+/**
+ * 教师(用户)
+ */
export class Teacher {
+ id: number;
+ email: string;
+ name: string;
+ password: string;
+ sex: boolean;
+ username: string;
}
```
接着定义构造函数:
```typescript
+++ b/first-app/src/app/entity/teacher.ts
@@ -7,5 +7,14 @@ export class Teacher {
name: string;
password: string;
sex: boolean;
username: string;
+
+ constructor(id: number, email: string, name: string, password: string, sex: boolean, username: string) {
+ this.id = id;
+ this.email = email;
+ this.name = name;
+ this.password = password;
+ this.sex = sex;
+ this.username = username;
+ }
}
```
接下来,我们便可以在Login的相关功能上使用该类型了。
### 测试
```typescript
+++ b/first-app/src/app/entity/teacher.spec.ts
@@ -2,6 +2,6 @@ import { Teacher } from './teacher';
describe('Teacher', () => {
it('should create an instance', () => {
- expect(new Teacher()).toBeTruthy();
+ expect(new Teacher(1, 'email', 'name', 'password', true, 'username')).toBeTruthy();
});
});
```
## 规定类型
使用Teacher类重写登录组件:
```typescript
+++ b/first-app/src/app/login/login.component.ts
@@ -1,5 +1,6 @@
import {Component, EventEmitter, OnInit, Output} from '@angular/core';
import {HttpClient, HttpHeaders} from '@angular/common/http';
+import {Teacher} from '../entity/teacher';
@Component({
selector: 'app-login',
@@ -7,13 +8,10 @@ import {HttpClient, HttpHeaders} from '@angular/common/http';
styleUrls: ['./login.component.css']
})
export class LoginComponent implements OnInit {
- teacher = {} as {
- username: string,
- password: string
- };
+ teacher = {} as Teacher;
@Output()
- beLogin = new EventEmitter<{ username: string, name: string, email: string, sex: boolean }>();
+ beLogin = new EventEmitter<Teacher>();
constructor(private httpClient: HttpClient) {
}
@@ -31,7 +29,7 @@ export class LoginComponent implements OnInit {
httpHeaders = httpHeaders.append('Authorization', 'Basic ' + authToken);
this.httpClient
- .get<any>(
+ .get<Teacher>(
'http://angular.api.codedemo.club:81/teacher/login',
{headers: httpHeaders})
.subscribe(teacher => this.beLogin.emit(teacher),
```
重写Index 组件:
```typescript
+++ b/first-app/src/app/index/index.component.ts
@@ -1,4 +1,5 @@
import {Component, OnInit} from '@angular/core';
+import {Teacher} from '../entity/teacher';
@Component({
selector: 'app-index',
@@ -15,7 +16,7 @@ export class IndexComponent implements OnInit {
ngOnInit(): void {
}
- onLogin(teacher: { username: string, name: string, email: string, sex: boolean }): void {
+ onLogin(teacher: Teacher): void {
console.log(new Date().toTimeString(), '子组件进行了数据弹射', teacher);
this.login = true;
}
```
重写测试
```typescript
+++ b/first-app/src/app/login/login.component.spec.ts
@@ -3,6 +3,7 @@ import {ComponentFixture, TestBed} from '@angular/core/testing';
import {LoginComponent} from './login.component';
import {FormsModule} from '@angular/forms';
import {HttpClientModule} from '@angular/common/http';
+import {Teacher} from '../entity/teacher';
describe('LoginComponent', () => {
let component: LoginComponent;
@@ -45,7 +46,7 @@ describe('LoginComponent', () => {
it('onSubmit 用户登录', () => {
// 启动自动变更检测
fixture.autoDetectChanges();
- component.teacher = {username: '张三', password: 'codedemo.club'};
+ component.teacher = {username: '张三', password: 'codedemo.club'} as Teacher;
component.onSubmit();
});
});
```
## 验证
是时候展示伟大的TypeScript以及`ng t`的伟大魅力了!在日常的开发工作中,我们常常因为不敢重构而放弃重构的想法,特别是一些弱类型的语言,肯定动一动某个属性的名称都会给我们带来恶梦。而有了TypeScript加之Angular为我们准备的`ng t`,我们再也不惧怕重构了。
此时我们可以使用`ng t`来验证本次重构是否成功。在使用`ng t`前,我们需要将所有的`fdescribe`、`fit`进行复原,以使得所有的测试文件均得到执行。又由于我们为每个组件都定义了对应的测试文件,所以若测试的代码全部成功的执行,则说明此次重构没有任何问题。
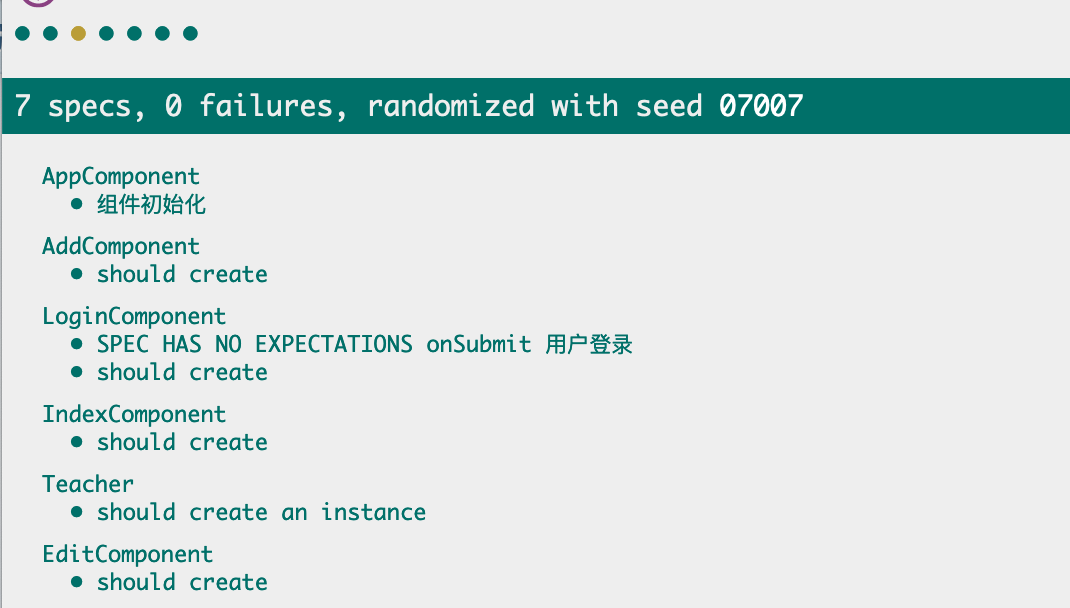
## 本节作业
我们在Teacher对应的测试文件中如下实例化Teacher:
```typescript
+++ b/first-app/src/app/entity/teacher.spec.ts
describe('Teacher', () => {
it('should create an instance', () => {
expect(new Teacher(1, 'email', 'name', 'password', true, 'username')).toBeTruthy();
});
});
```
而如果我们少填写一个参数,或是将对应参数的值输入undefined则会抛出异常:


请**尝试**通过自己的能力使Teacher的构造函数支持默认值,使以下代码正常执行:
```typescript
describe('Teacher', () => {
it('should create an instance', () => {
expect(new Teacher()).toBeTruthy();
const id = 123;
expect(new Teacher(id).toBeTruthy();
const email = 'zhangsan@codedemo.club';
expect(new Teacher(id, email).toBeTruthy();
});
});
```
| 名称 | 地址 | 备注 |
| --------------- | ------------------------------------------------------------ | ---- |
| TypeScript 类 | [https://www.tslang.cn/docs/handbook/classes.html](https://www.tslang.cn/docs/handbook/classes.html) | |
| TypeScript 类型 | [https://www.tslang.cn/docs/handbook/advanced-types.html](https://www.tslang.cn/docs/handbook/advanced-types.html) | |
| 本节源码 | [https://github.com/mengyunzhi/angular11-guild/archive/step3.6.zip](https://github.com/mengyunzhi/angular11-guild/archive/step3.6.zip) | |
- 序言
- 第一章 Hello World
- 1.1 环境安装
- 1.2 Hello Angular
- 1.3 Hello World!
- 第二章 教师管理
- 2.1 教师列表
- 2.1.1 初始化原型
- 2.1.2 组件生命周期之初始化
- 2.1.3 ngFor
- 2.1.4 ngIf、ngTemplate
- 2.1.5 引用 Bootstrap
- 2.2 请求后台数据
- 2.2.1 HttpClient
- 2.2.2 请求数据
- 2.2.3 模块与依赖注入
- 2.2.4 异步与回调函数
- 2.2.5 集成测试
- 2.2.6 本章小节
- 2.3 新增教师
- 2.3.1 组件初始化
- 2.3.2 [(ngModel)]
- 2.3.3 对接后台
- 2.3.4 路由
- 2.4 编辑教师
- 2.4.1 组件初始化
- 2.4.2 获取路由参数
- 2.4.3 插值与模板表达式
- 2.4.4 初识泛型
- 2.4.5 更新教师
- 2.4.6 测试中的路由
- 2.5 删除教师
- 2.6 收尾工作
- 2.6.1 RouterLink
- 2.6.2 fontawesome图标库
- 2.6.3 firefox
- 2.7 总结
- 第三章 用户登录
- 3.1 初识单元测试
- 3.2 http概述
- 3.3 Basic access authentication
- 3.4 着陆组件
- 3.5 @Output
- 3.6 TypeScript 类
- 3.7 浏览器缓存
- 3.8 总结
- 第四章 个人中心
- 4.1 原型
- 4.2 管道
- 4.3 对接后台
- 4.4 x-auth-token认证
- 4.5 拦截器
- 4.6 小结
- 第五章 系统菜单
- 5.1 延迟及测试
- 5.2 手动创建组件
- 5.3 隐藏测试信息
- 5.4 规划路由
- 5.5 定义菜单
- 5.6 注销
- 5.7 小结
- 第六章 班级管理
- 6.1 新增班级
- 6.1.1 组件初始化
- 6.1.2 MockApi 新建班级
- 6.1.3 ApiInterceptor
- 6.1.4 数据验证
- 6.1.5 教师选择列表
- 6.1.6 MockApi 教师列表
- 6.1.7 代码重构
- 6.1.8 小结
- 6.2 教师列表组件
- 6.2.1 初始化
- 6.2.2 响应式表单
- 6.2.3 getTestScheduler()
- 6.2.4 应用组件
- 6.2.5 小结
- 6.3 班级列表
- 6.3.1 原型设计
- 6.3.2 初始化分页
- 6.3.3 MockApi
- 6.3.4 静态分页
- 6.3.5 动态分页
- 6.3.6 @Input()
- 6.4 编辑班级
- 6.4.1 测试模块
- 6.4.2 响应式表单验证
- 6.4.3 @Input()
- 6.4.4 FormGroup
- 6.4.5 自定义FormControl
- 6.4.6 代码重构
- 6.4.7 小结
- 6.5 删除班级
- 6.6 集成测试
- 6.6.1 惰性加载
- 6.6.2 API拦截器
- 6.6.3 路由与跳转
- 6.6.4 ngStyle
- 6.7 初识Service
- 6.7.1 catchError
- 6.7.2 单例服务
- 6.7.3 单元测试
- 6.8 小结
- 第七章 学生管理
- 7.1 班级列表组件
- 7.2 新增学生
- 7.2.1 exports
- 7.2.2 自定义验证器
- 7.2.3 异步验证器
- 7.2.4 再识DI
- 7.2.5 属性型指令
- 7.2.6 完成功能
- 7.2.7 小结
- 7.3 单元测试进阶
- 7.4 学生列表
- 7.4.1 JSON对象与对象
- 7.4.2 单元测试
- 7.4.3 分页模块
- 7.4.4 子组件测试
- 7.4.5 重构分页
- 7.5 删除学生
- 7.5.1 第三方dialog
- 7.5.2 批量删除
- 7.5.3 面向对象
- 7.6 集成测试
- 7.7 编辑学生
- 7.7.1 初始化
- 7.7.2 自定义provider
- 7.7.3 更新学生
- 7.7.4 集成测试
- 7.7.5 可订阅的路由参数
- 7.7.6 小结
- 7.8 总结
- 第八章 其它
- 8.1 打包构建
- 8.2 发布部署
- 第九章 总结