# 代码重构
“如果尿布臭了,就换掉它。”-语出 Beck 奶奶,论抚养小孩的哲学。重构,绝对是软件开发写程序过程中最重要的事之一。没有重构的项目随着时间的推移必然会成为老太太的裹脚布 ---- 又臭又长。
下面,我们按以下几步对当前项目进行重构,以使得整个项目看起来更易懂,修改其来更容易。
## 建立实体类
建立实体类(接口)将后台API交互过程中对返回的数据进行了规范。这种规范使得团队成员再与后台交互时完全的规避拼写错误,即使错了,整个项目对某个字段的修改也仅仅需要一次。
> 实体类:由于该类的作用是对应后台返回的**实体entity**,所以我们把这种类称为实体类。
本节在新建班级时,并没有创建对应的班级实体类,实虽然并不影响当前功能实现,但明显**班级实体**日后还需要在**班级列表**,**班级编辑**中被再次使用。我们来到src/app/entity文件夹,然后执行`ng g class Clazz`命令来生成一新类:
```bash
panjie@panjies-Mac-Pro entity % ng g class clazz
CREATE src/app/entity/clazz.spec.ts (150 bytes)
CREATE src/app/entity/clazz.ts (23 bytes)
```
然后在`clazz`实体类中增加`id`,`name`,`teacher`属性:
```typescript
import {Teacher} from './teacher';
export class Clazz {
id: number;
name: string;
teacher: Teacher;
}
```
然后如下初始化构造函数:
```typescript
constructor(data = {} ① as ② {
id?③: number;
name?③: string;
teacher?③: Teacher;
}) {
this.id = data.id as number; ④
this.name = data.name as string;
this.teacher = data.teacher as Teacher;
}
```
- ① 使用`data = {}`来初始化一个带有默认值的参数。
- ② 使用as来进行转换,规定参数的类型
- ③ ? 号的作用是指该属性可有可无。
- ④ 由于③的存在,`data.id`的值可能是`undefined`,这里使用`as number`防止报类型不匹配的错误。
哪此一来,我们便可以使用如下语句来实例化`Clazz`了:
```typescript
+++ b/first-app/src/app/entity/clazz.spec.ts
@@ -1,10 +1,12 @@
import {Clazz} from './clazz';
+import {Teacher} from './teacher';
describe('Clazz', () => {
it('should create an instance', () => {
expect(new Clazz()).toBeTruthy();
expect(new Clazz({id: 123})).toBeTruthy();
- expect(new Clazz()).toBeTruthy();
- expect(new Clazz()).toBeTruthy();
+ expect(new Clazz({name: 'test'})).toBeTruthy();
+ expect(new Clazz({teacher: {id: 1} as Teacher})).toBeTruthy();
+ expect(new Clazz({id: 123, name: 'test', teacher: {id: 1} as Teacher})).toBeTruthy();
});
});
```
将`it`变更为`fit`,执行单元测试,通过:
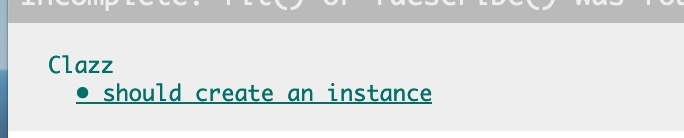
实体类有了,下一步我们将实体类应该到对应的组件中:
```typescript
+++ b/first-app/src/app/clazz/add/add.component.ts
+import {Clazz} from '../../entity/clazz';
onSubmit(): void {
const newClazz = new Clazz({
name: this.clazz.name,
teacher: {
id: this.clazz.teacherId
} as Teacher
});
```
此时如果我们不小心把`name`拼写为`naem`,则编辑器会实时地报告一个错误:

### 重构/src/app/entity/teacher.ts
既然如上的构造函数这么优秀,我们打开`/src/app/entity/teacher.ts`,按上述方法重写一便构造函数:
```typescript
+++ b/first-app/src/app/entity/teacher.ts
@@ -9,12 +9,13 @@ export class Teacher {
sex: boolean;
username: string;
- constructor(id: number, email: string, name: string, password: string, sex: boolean, username: string) {
- this.id = id;
- this.email = email;
- this.name = name;
- this.password = password;
- this.sex = sex;
- this.username = username;
+ constructor(data = {} as {
+ id?: number, email?: string, name?: string, password?: string, sex?: boolean, username?: string}) {
+ this.id = data.id as number;
+ this.email = data.email as string;
+ this.name = data.name as string;
+ this.password = data.password as string;
+ this.sex = data.sex as boolean;
+ this.username = data.username as string;
}
}
```
typescript这个强类型的语言,能够快速发现在重构过程中发生的问题。上述构造函数重构后,在启用`ng t`的控制台将得到如下错误提醒:
```bash
Error: src/app/entity/teacher.spec.ts:5:27 - error TS2554: Expected 0-1 arguments, but got 6.
5 expect(new Teacher(1, 'email', 'name', 'password', true, 'username')).toBeTruthy();
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
```
打开相应的错误文件,修正如下:
```typescript
+++ b/first-app/src/app/entity/teacher.spec.ts
@@ -1,7 +1,14 @@
-import { Teacher } from './teacher';
+import {Teacher} from './teacher';
describe('Teacher', () => {
it('should create an instance', () => {
- expect(new Teacher(1, 'email', 'name', 'password', true, 'username')).toBeTruthy();
+ expect(new Teacher({
+ id: 1,
+ email: 'email',
+ name: 'name',
+ password: 'password',
+ sex: true,
+ username: 'username'
+ })).toBeTruthy();
});
});
```
新的构造函数有了,历史上一些不太好的写法终于可心退出舞台了:
```typescript
+++ b/first-app/src/app/clazz/add/add.component.ts
@@ -29,9 +29,7 @@ export class AddComponent implements OnInit {
onSubmit(): void {
const newClazz = new Clazz({
name: this.clazz.name,
- teacher: {
- id: this.clazz.teacherId
- } as Teacher
+ teacher: new Teacher({id: this.clazz.teacherId})
});
this.httpClient.post(this.url, newClazz)
.subscribe(clazz => console.log('保存成功', clazz),
```
```typescript
+++ b/first-app/src/app/login/login.component.ts
@@ -8,7 +8,7 @@ import {Teacher} from '../entity/teacher';
styleUrls: ['./login.component.css']
})
export class LoginComponent implements OnInit {
- teacher = {} as Teacher;
+ teacher = new Teacher();
@Output()
beLogin = new EventEmitter<Teacher>();
```
## 剥离测试类
在进行MockApi的过程中,我们在`clazz/add/add.component.mock-api.spec.ts`建立了两个模拟API用的内部类`ClazzMockApi`、 `TeacherMockApi`,其它成员不会关注到此内部类的存在,同时将模拟班级与模拟教师放在一起,也不利于管理。
为此,我们在src/app下新建mock-api文件夹,然后将两个内部类移动过去:
```bash
panjie@panjies-Mac-Pro app % pwd
/Users/panjie/github/mengyunzhi/angular11-guild/first-app/src/app
panjie@panjies-Mac-Pro app % tree mock-api
mock-api
├── clazz.mock.api.ts
└── teacher.mock.api.ts
0 directories, 2 files
```
clazz.mock.api.ts内容如下:
```typescript
import {ApiInjector, MockApiInterface, randomNumber, RequestOptions} from '@yunzhi/ng-mock-api';
/**
* 班级模拟API
*/
export 👈 class ClazzMockApi implements MockApiInterface {
getInjectors(): ApiInjector<any>[] {
return [
{
method: 'POST',
url: 'clazz',
result: (urlMatches: string[], options: RequestOptions) => {
console.log('接收到了数据请求,请求主体的内容为:', options.body);
const clazz = options.body;
if (!clazz.name || clazz.name === '') {
throw new Error('班级名称未定义或为空');
}
if (!clazz.teacher || !clazz.teacher.id) {
throw new Error('班主任ID未定义');
}
return {
id: randomNumber(),
name: '保存的班级名称',
createTime: new Date().getTime(),
teacher: {
id: clazz.teacher.id,
name: '教师姓名'
}
};
}
}
];
}
}
```
该类需要被本文件以外的文件`import`,所以必须使用`export`关键字 👈 。
teacher.mock.api.ts内容如下:
```typescript
import {ApiInjector, MockApiInterface, randomNumber} from '@yunzhi/ng-mock-api';
/**
* 教师模拟API
*/
export 👈 class TeacherMockApi implements MockApiInterface {
getInjectors(): ApiInjector<any>[] {
return [{
// 获取所有教师
method: 'GET',
url: 'teacher',
result: [
{
id: randomNumber(),
name: '教师姓名1'
},
{
id: randomNumber(),
name: '教师姓名2'
}
]
}];
}
}
```
最后对原`clazz/add/add.component.mock-api.spec.ts`重构:
```typescript
+++ b/first-app/src/app/clazz/add/add.component.mock-api.spec.ts
@@ -3,6 +3,8 @@ import {ComponentFixture, TestBed} from '@angular/core/testing';
import {HTTP_INTERCEPTORS, HttpClientModule} from '@angular/common/http';
import {ApiInjector, MockApiInterceptor, MockApiInterface, randomNumber, RequestOptions} from '@yunzhi/ng-mock-api';
import {FormsModule} from '@angular/forms';
+import {ClazzMockApi} from '../../mock-api/clazz.mock.api';
+import {TeacherMockApi} from '../../mock-api/teacher.mock.api';
describe('clazz add with mockapi', () => {
let component: AddComponent;
@@ -38,61 +40,3 @@ describe('clazz add with mockapi', () => {
fixture.autoDetectChanges();
});
});
-
-/**
- * 班级模拟API
- */
-class ClazzMockApi implements MockApiInterface {
-请将本行及以下代码全部删除,限于篇幅,略过.
```
好了,终于不那么过分的不合格了,就到这里。
## 本节作业
项目中仍然存在待重构的`Teacher`,请尝试找到它们并完成重构。
| 名称 | 地址 |
| ---------------- | ------------------------------------------------------------ |
| TypeScript模块 | [https://www.tslang.cn/docs/handbook/modules.html](https://www.tslang.cn/docs/handbook/modules.html) |
| 本节源码(含答案) | [https://github.com/mengyunzhi/angular11-guild/archive/step6.1.7.zip](https://github.com/mengyunzhi/angular11-guild/archive/step6.1.7.zip) |
- 序言
- 第一章 Hello World
- 1.1 环境安装
- 1.2 Hello Angular
- 1.3 Hello World!
- 第二章 教师管理
- 2.1 教师列表
- 2.1.1 初始化原型
- 2.1.2 组件生命周期之初始化
- 2.1.3 ngFor
- 2.1.4 ngIf、ngTemplate
- 2.1.5 引用 Bootstrap
- 2.2 请求后台数据
- 2.2.1 HttpClient
- 2.2.2 请求数据
- 2.2.3 模块与依赖注入
- 2.2.4 异步与回调函数
- 2.2.5 集成测试
- 2.2.6 本章小节
- 2.3 新增教师
- 2.3.1 组件初始化
- 2.3.2 [(ngModel)]
- 2.3.3 对接后台
- 2.3.4 路由
- 2.4 编辑教师
- 2.4.1 组件初始化
- 2.4.2 获取路由参数
- 2.4.3 插值与模板表达式
- 2.4.4 初识泛型
- 2.4.5 更新教师
- 2.4.6 测试中的路由
- 2.5 删除教师
- 2.6 收尾工作
- 2.6.1 RouterLink
- 2.6.2 fontawesome图标库
- 2.6.3 firefox
- 2.7 总结
- 第三章 用户登录
- 3.1 初识单元测试
- 3.2 http概述
- 3.3 Basic access authentication
- 3.4 着陆组件
- 3.5 @Output
- 3.6 TypeScript 类
- 3.7 浏览器缓存
- 3.8 总结
- 第四章 个人中心
- 4.1 原型
- 4.2 管道
- 4.3 对接后台
- 4.4 x-auth-token认证
- 4.5 拦截器
- 4.6 小结
- 第五章 系统菜单
- 5.1 延迟及测试
- 5.2 手动创建组件
- 5.3 隐藏测试信息
- 5.4 规划路由
- 5.5 定义菜单
- 5.6 注销
- 5.7 小结
- 第六章 班级管理
- 6.1 新增班级
- 6.1.1 组件初始化
- 6.1.2 MockApi 新建班级
- 6.1.3 ApiInterceptor
- 6.1.4 数据验证
- 6.1.5 教师选择列表
- 6.1.6 MockApi 教师列表
- 6.1.7 代码重构
- 6.1.8 小结
- 6.2 教师列表组件
- 6.2.1 初始化
- 6.2.2 响应式表单
- 6.2.3 getTestScheduler()
- 6.2.4 应用组件
- 6.2.5 小结
- 6.3 班级列表
- 6.3.1 原型设计
- 6.3.2 初始化分页
- 6.3.3 MockApi
- 6.3.4 静态分页
- 6.3.5 动态分页
- 6.3.6 @Input()
- 6.4 编辑班级
- 6.4.1 测试模块
- 6.4.2 响应式表单验证
- 6.4.3 @Input()
- 6.4.4 FormGroup
- 6.4.5 自定义FormControl
- 6.4.6 代码重构
- 6.4.7 小结
- 6.5 删除班级
- 6.6 集成测试
- 6.6.1 惰性加载
- 6.6.2 API拦截器
- 6.6.3 路由与跳转
- 6.6.4 ngStyle
- 6.7 初识Service
- 6.7.1 catchError
- 6.7.2 单例服务
- 6.7.3 单元测试
- 6.8 小结
- 第七章 学生管理
- 7.1 班级列表组件
- 7.2 新增学生
- 7.2.1 exports
- 7.2.2 自定义验证器
- 7.2.3 异步验证器
- 7.2.4 再识DI
- 7.2.5 属性型指令
- 7.2.6 完成功能
- 7.2.7 小结
- 7.3 单元测试进阶
- 7.4 学生列表
- 7.4.1 JSON对象与对象
- 7.4.2 单元测试
- 7.4.3 分页模块
- 7.4.4 子组件测试
- 7.4.5 重构分页
- 7.5 删除学生
- 7.5.1 第三方dialog
- 7.5.2 批量删除
- 7.5.3 面向对象
- 7.6 集成测试
- 7.7 编辑学生
- 7.7.1 初始化
- 7.7.2 自定义provider
- 7.7.3 更新学生
- 7.7.4 集成测试
- 7.7.5 可订阅的路由参数
- 7.7.6 小结
- 7.8 总结
- 第八章 其它
- 8.1 打包构建
- 8.2 发布部署
- 第九章 总结